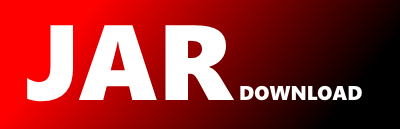
com.oracle.truffle.sl.nodes.access.SLWritePropertyCacheNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.access;
import com.oracle.truffle.api.Assumption;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.dsl.UnsupportedSpecializationException;
import com.oracle.truffle.api.nodes.ExplodeLoop;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.nodes.ExplodeLoop.LoopExplosionKind;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.api.object.Location;
import com.oracle.truffle.api.object.Shape;
import java.util.concurrent.locks.Lock;
@GeneratedBy(SLWritePropertyCacheNode.class)
public final class SLWritePropertyCacheNodeGen extends SLWritePropertyCacheNode {
@CompilationFinal private int state_ = 1;
@CompilationFinal private int exclude_;
@CompilationFinal private WriteExistingPropertyCachedData writeExistingPropertyCached_cache;
@CompilationFinal private WriteNewPropertyCachedData writeNewPropertyCached_cache;
private SLWritePropertyCacheNodeGen() {
}
@ExplodeLoop(kind = LoopExplosionKind.FULL_EXPLODE_UNTIL_RETURN)
@Override
public void executeWrite(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
int state = state_;
if ((state & 0b11110) != 0 /* is-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) || writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) || writeUncached(DynamicObject, Object, Object) || updateShape(DynamicObject, Object, Object) */) {
if ((state & 0b10) != 0 /* is-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) */) {
WriteExistingPropertyCachedData s1_ = this.writeExistingPropertyCached_cache;
while (s1_ != null) {
if (!isValid_(s1_.assumption0_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeWriteExistingPropertyCached_(s1_);
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
if ((s1_.cachedName_.equals(arg1Value)) && (SLPropertyCacheNode.shapeCheck(s1_.shape_, arg0Value))) {
assert (s1_.location_ != null);
if ((SLWritePropertyCacheNode.canSet(s1_.location_, arg2Value))) {
SLWritePropertyCacheNode.writeExistingPropertyCached(arg0Value, arg1Value, arg2Value, s1_.cachedName_, s1_.shape_, s1_.location_);
return;
}
}
s1_ = s1_.next_;
}
}
if ((state & 0b100) != 0 /* is-active writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */) {
WriteNewPropertyCachedData s2_ = this.writeNewPropertyCached_cache;
while (s2_ != null) {
if (!isValid_(s2_.assumption0_) || !isValid_(s2_.assumption1_)) {
CompilerDirectives.transferToInterpreterAndInvalidate();
removeWriteNewPropertyCached_(s2_);
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
if ((SLPropertyCacheNode.namesEqual(s2_.cachedName_, arg1Value)) && (SLPropertyCacheNode.shapeCheck(s2_.oldShape_, arg0Value))) {
assert (s2_.oldLocation_ == null);
if ((SLWritePropertyCacheNode.canStore(s2_.newLocation_, arg2Value))) {
SLWritePropertyCacheNode.writeNewPropertyCached(arg0Value, arg1Value, arg2Value, s2_.cachedName_, s2_.oldShape_, s2_.oldLocation_, s2_.newShape_, s2_.newLocation_);
return;
}
}
s2_ = s2_.next_;
}
}
if ((state & 0b1000) != 0 /* is-active writeUncached(DynamicObject, Object, Object) */) {
if ((arg0Value.getShape().isValid())) {
SLWritePropertyCacheNode.writeUncached(arg0Value, arg1Value, arg2Value);
return;
}
}
if ((state & 0b10000) != 0 /* is-active updateShape(DynamicObject, Object, Object) */) {
if ((!(arg0Value.getShape().isValid()))) {
updateShape(arg0Value, arg1Value, arg2Value);
return;
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
executeAndSpecialize(arg0Value, arg1Value, arg2Value);
return;
}
private void executeAndSpecialize(DynamicObject arg0Value, Object arg1Value, Object arg2Value) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
try {
int state = state_ & 0xfffffffe/* mask-active uninitialized*/;
int exclude = exclude_;
if ((exclude & 0b1) == 0 /* is-not-excluded writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) */) {
int count1_ = 0;
WriteExistingPropertyCachedData s1_ = this.writeExistingPropertyCached_cache;
if ((state & 0b10) != 0 /* is-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) */) {
while (s1_ != null) {
if ((s1_.cachedName_.equals(arg1Value)) && (SLPropertyCacheNode.shapeCheck(s1_.shape_, arg0Value))) {
assert (s1_.location_ != null);
if ((SLWritePropertyCacheNode.canSet(s1_.location_, arg2Value)) && (s1_.assumption0_ == null || isValid_(s1_.assumption0_))) {
break;
}
}
s1_ = s1_.next_;
count1_++;
}
}
if (s1_ == null) {
{
Shape shape__ = (SLPropertyCacheNode.lookupShape(arg0Value));
// assert (s1_.cachedName_.equals(arg1Value));
if ((SLPropertyCacheNode.shapeCheck(shape__, arg0Value))) {
Location location__ = (SLWritePropertyCacheNode.lookupLocation(shape__, arg1Value, arg2Value));
if ((location__ != null) && (SLWritePropertyCacheNode.canSet(location__, arg2Value))) {
Assumption assumption0 = (shape__.getValidAssumption());
if (isValid_(assumption0)) {
if (count1_ < (SLPropertyCacheNode.CACHE_LIMIT)) {
s1_ = new WriteExistingPropertyCachedData(writeExistingPropertyCached_cache);
s1_.cachedName_ = (arg1Value);
s1_.shape_ = shape__;
s1_.location_ = location__;
s1_.assumption0_ = assumption0;
this.writeExistingPropertyCached_cache = s1_;
this.state_ = state = state | 0b10 /* add-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) */;
}
}
}
}
}
}
if (s1_ != null) {
lock.unlock();
hasLock = false;
SLWritePropertyCacheNode.writeExistingPropertyCached(arg0Value, arg1Value, arg2Value, s1_.cachedName_, s1_.shape_, s1_.location_);
return;
}
}
if ((exclude & 0b10) == 0 /* is-not-excluded writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */) {
int count2_ = 0;
WriteNewPropertyCachedData s2_ = this.writeNewPropertyCached_cache;
if ((state & 0b100) != 0 /* is-active writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */) {
while (s2_ != null) {
if ((SLPropertyCacheNode.namesEqual(s2_.cachedName_, arg1Value)) && (SLPropertyCacheNode.shapeCheck(s2_.oldShape_, arg0Value))) {
assert (s2_.oldLocation_ == null);
if ((SLWritePropertyCacheNode.canStore(s2_.newLocation_, arg2Value)) && (s2_.assumption0_ == null || isValid_(s2_.assumption0_)) && (s2_.assumption1_ == null || isValid_(s2_.assumption1_))) {
break;
}
}
s2_ = s2_.next_;
count2_++;
}
}
if (s2_ == null) {
{
Object cachedName__ = (arg1Value);
if ((SLPropertyCacheNode.namesEqual(cachedName__, arg1Value))) {
Shape oldShape__ = (SLPropertyCacheNode.lookupShape(arg0Value));
if ((SLPropertyCacheNode.shapeCheck(oldShape__, arg0Value))) {
Location oldLocation__ = (SLWritePropertyCacheNode.lookupLocation(oldShape__, arg1Value, arg2Value));
if ((oldLocation__ == null)) {
Shape newShape__ = (SLWritePropertyCacheNode.defineProperty(oldShape__, arg1Value, arg2Value));
Location newLocation__ = (SLWritePropertyCacheNode.lookupLocation(newShape__, arg1Value));
if ((SLWritePropertyCacheNode.canStore(newLocation__, arg2Value))) {
Assumption assumption0 = (oldShape__.getValidAssumption());
if (isValid_(assumption0)) {
Assumption assumption1 = (newShape__.getValidAssumption());
if (isValid_(assumption1)) {
if (count2_ < (SLPropertyCacheNode.CACHE_LIMIT)) {
s2_ = new WriteNewPropertyCachedData(writeNewPropertyCached_cache);
s2_.cachedName_ = cachedName__;
s2_.oldShape_ = oldShape__;
s2_.oldLocation_ = oldLocation__;
s2_.newShape_ = newShape__;
s2_.newLocation_ = newLocation__;
s2_.assumption0_ = assumption0;
s2_.assumption1_ = assumption1;
this.writeNewPropertyCached_cache = s2_;
this.state_ = state = state | 0b100 /* add-active writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */;
}
}
}
}
}
}
}
}
}
if (s2_ != null) {
lock.unlock();
hasLock = false;
SLWritePropertyCacheNode.writeNewPropertyCached(arg0Value, arg1Value, arg2Value, s2_.cachedName_, s2_.oldShape_, s2_.oldLocation_, s2_.newShape_, s2_.newLocation_);
return;
}
}
if ((arg0Value.getShape().isValid())) {
this.exclude_ = exclude = exclude | 0b11 /* add-excluded writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location), writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */;
this.writeExistingPropertyCached_cache = null;
this.writeNewPropertyCached_cache = null;
state = state & 0xfffffff9 /* remove-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location), writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */;
this.state_ = state = state | 0b1000 /* add-active writeUncached(DynamicObject, Object, Object) */;
lock.unlock();
hasLock = false;
SLWritePropertyCacheNode.writeUncached(arg0Value, arg1Value, arg2Value);
return;
}
if ((!(arg0Value.getShape().isValid()))) {
this.state_ = state = state | 0b10000 /* add-active updateShape(DynamicObject, Object, Object) */;
lock.unlock();
hasLock = false;
updateShape(arg0Value, arg1Value, arg2Value);
return;
}
CompilerDirectives.transferToInterpreterAndInvalidate();
throw new UnsupportedSpecializationException(this, new Node[] {null, null, null}, arg0Value, arg1Value, arg2Value);
} finally {
if (hasLock) {
lock.unlock();
}
}
}
@Override
public NodeCost getCost() {
int state = state_ & 0xfffffffe/* mask-active uninitialized*/;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if (((state & 0b11110) & ((state & 0b11110) - 1)) == 0 /* is-single-active */) {
WriteExistingPropertyCachedData s1_ = this.writeExistingPropertyCached_cache;
WriteNewPropertyCachedData s2_ = this.writeNewPropertyCached_cache;
if ((s1_ == null || s1_.next_ == null) && (s2_ == null || s2_.next_ == null)) {
return NodeCost.MONOMORPHIC;
}
}
return NodeCost.POLYMORPHIC;
}
void removeWriteExistingPropertyCached_(Object s1_) {
Lock lock = getLock();
lock.lock();
try {
WriteExistingPropertyCachedData prev = null;
WriteExistingPropertyCachedData cur = this.writeExistingPropertyCached_cache;
while (cur != null) {
if (cur == s1_) {
if (prev == null) {
this.writeExistingPropertyCached_cache = cur.next_;
} else {
prev.next_ = cur.next_;
}
break;
}
prev = cur;
cur = cur.next_;
}
if (this.writeExistingPropertyCached_cache == null) {
this.state_ = this.state_ & 0xfffffffd /* remove-active writeExistingPropertyCached(DynamicObject, Object, Object, Object, Shape, Location) */;
}
} finally {
lock.unlock();
}
}
void removeWriteNewPropertyCached_(Object s2_) {
Lock lock = getLock();
lock.lock();
try {
WriteNewPropertyCachedData prev = null;
WriteNewPropertyCachedData cur = this.writeNewPropertyCached_cache;
while (cur != null) {
if (cur == s2_) {
if (prev == null) {
this.writeNewPropertyCached_cache = cur.next_;
} else {
prev.next_ = cur.next_;
}
break;
}
prev = cur;
cur = cur.next_;
}
if (this.writeNewPropertyCached_cache == null) {
this.state_ = this.state_ & 0xfffffffb /* remove-active writeNewPropertyCached(DynamicObject, Object, Object, Object, Shape, Location, Shape, Location) */;
}
} finally {
lock.unlock();
}
}
private static boolean isValid_(Assumption assumption) {
return assumption != null && assumption.isValid();
}
public static SLWritePropertyCacheNode create() {
return new SLWritePropertyCacheNodeGen();
}
@GeneratedBy(SLWritePropertyCacheNode.class)
private static final class WriteExistingPropertyCachedData {
@CompilationFinal WriteExistingPropertyCachedData next_;
@CompilationFinal Object cachedName_;
@CompilationFinal Shape shape_;
@CompilationFinal Location location_;
@CompilationFinal Assumption assumption0_;
WriteExistingPropertyCachedData(WriteExistingPropertyCachedData next_) {
this.next_ = next_;
}
}
@GeneratedBy(SLWritePropertyCacheNode.class)
private static final class WriteNewPropertyCachedData {
@CompilationFinal WriteNewPropertyCachedData next_;
@CompilationFinal Object cachedName_;
@CompilationFinal Shape oldShape_;
@CompilationFinal Location oldLocation_;
@CompilationFinal Shape newShape_;
@CompilationFinal Location newLocation_;
@CompilationFinal Assumption assumption0_;
@CompilationFinal Assumption assumption1_;
WriteNewPropertyCachedData(WriteNewPropertyCachedData next_) {
this.next_ = next_;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy