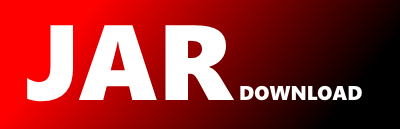
com.oracle.truffle.sl.nodes.access.SLReadPropertyNodeGen Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of truffle-sl Show documentation
Show all versions of truffle-sl Show documentation
Truffle SL is an example language implemented using the Truffle API.
The newest version!
// CheckStyle: start generated
package com.oracle.truffle.sl.nodes.access;
import com.oracle.truffle.api.CompilerDirectives;
import com.oracle.truffle.api.CompilerDirectives.CompilationFinal;
import com.oracle.truffle.api.dsl.GeneratedBy;
import com.oracle.truffle.api.frame.VirtualFrame;
import com.oracle.truffle.api.interop.Message;
import com.oracle.truffle.api.interop.TruffleObject;
import com.oracle.truffle.api.nodes.Node;
import com.oracle.truffle.api.nodes.NodeCost;
import com.oracle.truffle.api.object.DynamicObject;
import com.oracle.truffle.sl.nodes.SLExpressionNode;
import com.oracle.truffle.sl.nodes.interop.SLForeignToSLTypeNode;
import com.oracle.truffle.sl.runtime.SLContext;
import java.util.concurrent.locks.Lock;
@GeneratedBy(SLReadPropertyNode.class)
public final class SLReadPropertyNodeGen extends SLReadPropertyNode {
@Child private SLExpressionNode receiverNode_;
@Child private SLExpressionNode nameNode_;
@CompilationFinal private int state_;
@Child private SLReadPropertyCacheNode read_readNode_;
@Child private Node readForeign_foreignReadNode_;
@Child private SLForeignToSLTypeNode readForeign_toSLTypeNode_;
private SLReadPropertyNodeGen(SLExpressionNode receiverNode, SLExpressionNode nameNode) {
this.receiverNode_ = receiverNode;
this.nameNode_ = nameNode;
}
@Override
public Object executeGeneric(VirtualFrame frameValue) {
int state = state_;
Object receiverNodeValue_ = this.receiverNode_.executeGeneric(frameValue);
Object nameNodeValue_ = this.nameNode_.executeGeneric(frameValue);
if (state != 0 /* is-active read(DynamicObject, Object, SLReadPropertyCacheNode) || readForeign(TruffleObject, Object, Node, SLForeignToSLTypeNode) || typeError(Object, Object) */) {
if ((state & 0b1) != 0 /* is-active read(DynamicObject, Object, SLReadPropertyCacheNode) */ && receiverNodeValue_ instanceof DynamicObject) {
DynamicObject receiverNodeValue__ = (DynamicObject) receiverNodeValue_;
if ((SLContext.isSLObject(receiverNodeValue__))) {
return read(receiverNodeValue__, nameNodeValue_, this.read_readNode_);
}
}
if ((state & 0b10) != 0 /* is-active readForeign(TruffleObject, Object, Node, SLForeignToSLTypeNode) */ && receiverNodeValue_ instanceof TruffleObject) {
TruffleObject receiverNodeValue__ = (TruffleObject) receiverNodeValue_;
if ((!(SLContext.isSLObject(receiverNodeValue__)))) {
return readForeign(receiverNodeValue__, nameNodeValue_, this.readForeign_foreignReadNode_, this.readForeign_toSLTypeNode_);
}
}
if ((state & 0b100) != 0 /* is-active typeError(Object, Object) */) {
if (fallbackGuard_(receiverNodeValue_, nameNodeValue_)) {
return typeError(receiverNodeValue_, nameNodeValue_);
}
}
}
CompilerDirectives.transferToInterpreterAndInvalidate();
return executeAndSpecialize(receiverNodeValue_, nameNodeValue_);
}
@Override
public void executeVoid(VirtualFrame frameValue) {
executeGeneric(frameValue);
return;
}
private Object executeAndSpecialize(Object receiverNodeValue, Object nameNodeValue) {
Lock lock = getLock();
boolean hasLock = true;
lock.lock();
int state = state_;
int oldState = state;
try {
if (receiverNodeValue instanceof DynamicObject) {
DynamicObject receiverNodeValue_ = (DynamicObject) receiverNodeValue;
if ((SLContext.isSLObject(receiverNodeValue_))) {
this.read_readNode_ = super.insert((SLReadPropertyCacheNode.create()));
this.state_ = state = state | 0b1 /* add-active read(DynamicObject, Object, SLReadPropertyCacheNode) */;
lock.unlock();
hasLock = false;
return read(receiverNodeValue_, nameNodeValue, this.read_readNode_);
}
}
if (receiverNodeValue instanceof TruffleObject) {
TruffleObject receiverNodeValue_ = (TruffleObject) receiverNodeValue;
if ((!(SLContext.isSLObject(receiverNodeValue_)))) {
this.readForeign_foreignReadNode_ = super.insert((Message.READ.createNode()));
this.readForeign_toSLTypeNode_ = super.insert((SLForeignToSLTypeNode.create()));
this.state_ = state = state | 0b10 /* add-active readForeign(TruffleObject, Object, Node, SLForeignToSLTypeNode) */;
lock.unlock();
hasLock = false;
return readForeign(receiverNodeValue_, nameNodeValue, this.readForeign_foreignReadNode_, this.readForeign_toSLTypeNode_);
}
}
this.state_ = state = state | 0b100 /* add-active typeError(Object, Object) */;
lock.unlock();
hasLock = false;
return typeError(receiverNodeValue, nameNodeValue);
} finally {
if (oldState != 0) {
checkForPolymorphicSpecialize(oldState);
}
if (hasLock) {
lock.unlock();
}
}
}
private void checkForPolymorphicSpecialize(int oldState) {
int newState = this.state_;
if ((oldState ^ newState) != 0) {
this.reportPolymorphicSpecialize();
}
}
@Override
public NodeCost getCost() {
int state = state_;
if (state == 0b0) {
return NodeCost.UNINITIALIZED;
} else if ((state & (state - 1)) == 0 /* is-single-active */) {
return NodeCost.MONOMORPHIC;
}
return NodeCost.POLYMORPHIC;
}
@SuppressWarnings("unused")
private static boolean fallbackGuard_(Object receiverNodeValue, Object nameNodeValue) {
if (receiverNodeValue instanceof DynamicObject) {
DynamicObject receiverNodeValue_ = (DynamicObject) receiverNodeValue;
if ((SLContext.isSLObject(receiverNodeValue_))) {
return false;
}
}
if (receiverNodeValue instanceof TruffleObject) {
TruffleObject receiverNodeValue_ = (TruffleObject) receiverNodeValue;
if ((!(SLContext.isSLObject(receiverNodeValue_)))) {
return false;
}
}
return true;
}
public static SLReadPropertyNode create(SLExpressionNode receiverNode, SLExpressionNode nameNode) {
return new SLReadPropertyNodeGen(receiverNode, nameNode);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy