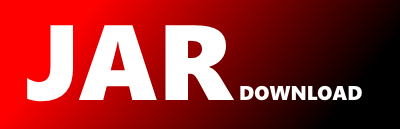
com.orbitz.consul.model.agent.ImmutablePorts Maven / Gradle / Ivy
package com.orbitz.consul.model.agent;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link Ports}.
*
* Use the builder to create immutable instances:
* {@code ImmutablePorts.builder()}.
*/
@Generated(from = "Ports", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutablePorts extends Ports {
private final int dns;
private final int http;
private final int rpc;
private final int serfLan;
private final int serfWan;
private final int server;
private ImmutablePorts(int dns, int http, int rpc, int serfLan, int serfWan, int server) {
this.dns = dns;
this.http = http;
this.rpc = rpc;
this.serfLan = serfLan;
this.serfWan = serfWan;
this.server = server;
}
/**
* @return The value of the {@code dns} attribute
*/
@JsonProperty("DNS")
@Override
public int getDns() {
return dns;
}
/**
* @return The value of the {@code http} attribute
*/
@JsonProperty("HTTP")
@Override
public int getHttp() {
return http;
}
/**
* @return The value of the {@code rpc} attribute
*/
@JsonProperty("RPC")
@Override
public int getRpc() {
return rpc;
}
/**
* @return The value of the {@code serfLan} attribute
*/
@JsonProperty("SerfLan")
@Override
public int getSerfLan() {
return serfLan;
}
/**
* @return The value of the {@code serfWan} attribute
*/
@JsonProperty("SerfWan")
@Override
public int getSerfWan() {
return serfWan;
}
/**
* @return The value of the {@code server} attribute
*/
@JsonProperty("Server")
@Override
public int getServer() {
return server;
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getDns() dns} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for dns
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withDns(int value) {
if (this.dns == value) return this;
return new ImmutablePorts(value, this.http, this.rpc, this.serfLan, this.serfWan, this.server);
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getHttp() http} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for http
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withHttp(int value) {
if (this.http == value) return this;
return new ImmutablePorts(this.dns, value, this.rpc, this.serfLan, this.serfWan, this.server);
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getRpc() rpc} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for rpc
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withRpc(int value) {
if (this.rpc == value) return this;
return new ImmutablePorts(this.dns, this.http, value, this.serfLan, this.serfWan, this.server);
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getSerfLan() serfLan} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for serfLan
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withSerfLan(int value) {
if (this.serfLan == value) return this;
return new ImmutablePorts(this.dns, this.http, this.rpc, value, this.serfWan, this.server);
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getSerfWan() serfWan} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for serfWan
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withSerfWan(int value) {
if (this.serfWan == value) return this;
return new ImmutablePorts(this.dns, this.http, this.rpc, this.serfLan, value, this.server);
}
/**
* Copy the current immutable object by setting a value for the {@link Ports#getServer() server} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for server
* @return A modified copy of the {@code this} object
*/
public final ImmutablePorts withServer(int value) {
if (this.server == value) return this;
return new ImmutablePorts(this.dns, this.http, this.rpc, this.serfLan, this.serfWan, value);
}
/**
* This instance is equal to all instances of {@code ImmutablePorts} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutablePorts
&& equalTo((ImmutablePorts) another);
}
private boolean equalTo(ImmutablePorts another) {
return dns == another.dns
&& http == another.http
&& rpc == another.rpc
&& serfLan == another.serfLan
&& serfWan == another.serfWan
&& server == another.server;
}
/**
* Computes a hash code from attributes: {@code dns}, {@code http}, {@code rpc}, {@code serfLan}, {@code serfWan}, {@code server}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + dns;
h += (h << 5) + http;
h += (h << 5) + rpc;
h += (h << 5) + serfLan;
h += (h << 5) + serfWan;
h += (h << 5) + server;
return h;
}
/**
* Prints the immutable value {@code Ports} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("Ports")
.omitNullValues()
.add("dns", dns)
.add("http", http)
.add("rpc", rpc)
.add("serfLan", serfLan)
.add("serfWan", serfWan)
.add("server", server)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "Ports", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json extends Ports {
int dns;
boolean dnsIsSet;
int http;
boolean httpIsSet;
int rpc;
boolean rpcIsSet;
int serfLan;
boolean serfLanIsSet;
int serfWan;
boolean serfWanIsSet;
int server;
boolean serverIsSet;
@JsonProperty("DNS")
public void setDns(int dns) {
this.dns = dns;
this.dnsIsSet = true;
}
@JsonProperty("HTTP")
public void setHttp(int http) {
this.http = http;
this.httpIsSet = true;
}
@JsonProperty("RPC")
public void setRpc(int rpc) {
this.rpc = rpc;
this.rpcIsSet = true;
}
@JsonProperty("SerfLan")
public void setSerfLan(int serfLan) {
this.serfLan = serfLan;
this.serfLanIsSet = true;
}
@JsonProperty("SerfWan")
public void setSerfWan(int serfWan) {
this.serfWan = serfWan;
this.serfWanIsSet = true;
}
@JsonProperty("Server")
public void setServer(int server) {
this.server = server;
this.serverIsSet = true;
}
@Override
public int getDns() { throw new UnsupportedOperationException(); }
@Override
public int getHttp() { throw new UnsupportedOperationException(); }
@Override
public int getRpc() { throw new UnsupportedOperationException(); }
@Override
public int getSerfLan() { throw new UnsupportedOperationException(); }
@Override
public int getSerfWan() { throw new UnsupportedOperationException(); }
@Override
public int getServer() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutablePorts fromJson(Json json) {
ImmutablePorts.Builder builder = ImmutablePorts.builder();
if (json.dnsIsSet) {
builder.dns(json.dns);
}
if (json.httpIsSet) {
builder.http(json.http);
}
if (json.rpcIsSet) {
builder.rpc(json.rpc);
}
if (json.serfLanIsSet) {
builder.serfLan(json.serfLan);
}
if (json.serfWanIsSet) {
builder.serfWan(json.serfWan);
}
if (json.serverIsSet) {
builder.server(json.server);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link Ports} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable Ports instance
*/
public static ImmutablePorts copyOf(Ports instance) {
if (instance instanceof ImmutablePorts) {
return (ImmutablePorts) instance;
}
return ImmutablePorts.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutablePorts ImmutablePorts}.
*
* ImmutablePorts.builder()
* .dns(int) // required {@link Ports#getDns() dns}
* .http(int) // required {@link Ports#getHttp() http}
* .rpc(int) // required {@link Ports#getRpc() rpc}
* .serfLan(int) // required {@link Ports#getSerfLan() serfLan}
* .serfWan(int) // required {@link Ports#getSerfWan() serfWan}
* .server(int) // required {@link Ports#getServer() server}
* .build();
*
* @return A new ImmutablePorts builder
*/
public static ImmutablePorts.Builder builder() {
return new ImmutablePorts.Builder();
}
/**
* Builds instances of type {@link ImmutablePorts ImmutablePorts}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "Ports", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_DNS = 0x1L;
private static final long INIT_BIT_HTTP = 0x2L;
private static final long INIT_BIT_RPC = 0x4L;
private static final long INIT_BIT_SERF_LAN = 0x8L;
private static final long INIT_BIT_SERF_WAN = 0x10L;
private static final long INIT_BIT_SERVER = 0x20L;
private long initBits = 0x3fL;
private int dns;
private int http;
private int rpc;
private int serfLan;
private int serfWan;
private int server;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code Ports} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(Ports instance) {
Objects.requireNonNull(instance, "instance");
dns(instance.getDns());
http(instance.getHttp());
rpc(instance.getRpc());
serfLan(instance.getSerfLan());
serfWan(instance.getSerfWan());
server(instance.getServer());
return this;
}
/**
* Initializes the value for the {@link Ports#getDns() dns} attribute.
* @param dns The value for dns
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("DNS")
public final Builder dns(int dns) {
this.dns = dns;
initBits &= ~INIT_BIT_DNS;
return this;
}
/**
* Initializes the value for the {@link Ports#getHttp() http} attribute.
* @param http The value for http
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("HTTP")
public final Builder http(int http) {
this.http = http;
initBits &= ~INIT_BIT_HTTP;
return this;
}
/**
* Initializes the value for the {@link Ports#getRpc() rpc} attribute.
* @param rpc The value for rpc
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("RPC")
public final Builder rpc(int rpc) {
this.rpc = rpc;
initBits &= ~INIT_BIT_RPC;
return this;
}
/**
* Initializes the value for the {@link Ports#getSerfLan() serfLan} attribute.
* @param serfLan The value for serfLan
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("SerfLan")
public final Builder serfLan(int serfLan) {
this.serfLan = serfLan;
initBits &= ~INIT_BIT_SERF_LAN;
return this;
}
/**
* Initializes the value for the {@link Ports#getSerfWan() serfWan} attribute.
* @param serfWan The value for serfWan
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("SerfWan")
public final Builder serfWan(int serfWan) {
this.serfWan = serfWan;
initBits &= ~INIT_BIT_SERF_WAN;
return this;
}
/**
* Initializes the value for the {@link Ports#getServer() server} attribute.
* @param server The value for server
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("Server")
public final Builder server(int server) {
this.server = server;
initBits &= ~INIT_BIT_SERVER;
return this;
}
/**
* Builds a new {@link ImmutablePorts ImmutablePorts}.
* @return An immutable instance of Ports
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutablePorts build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutablePorts(dns, http, rpc, serfLan, serfWan, server);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_DNS) != 0) attributes.add("dns");
if ((initBits & INIT_BIT_HTTP) != 0) attributes.add("http");
if ((initBits & INIT_BIT_RPC) != 0) attributes.add("rpc");
if ((initBits & INIT_BIT_SERF_LAN) != 0) attributes.add("serfLan");
if ((initBits & INIT_BIT_SERF_WAN) != 0) attributes.add("serfWan");
if ((initBits & INIT_BIT_SERVER) != 0) attributes.add("server");
return "Cannot build Ports, some of required attributes are not set " + attributes;
}
}
}