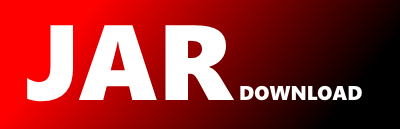
com.orbitz.consul.model.query.ImmutablePreparedQuery Maven / Gradle / Ivy
Show all versions of consul-client Show documentation
package com.orbitz.consul.model.query;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link PreparedQuery}.
*
* Use the builder to create immutable instances:
* {@code ImmutablePreparedQuery.builder()}.
*/
@Generated(from = "PreparedQuery", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
@JsonIgnoreProperties(ignoreUnknown = true)
public final class ImmutablePreparedQuery extends PreparedQuery {
private final @Nullable Template template;
private final String name;
private final @Nullable String session;
private final @Nullable String token;
private final ServiceQuery service;
private final @Nullable DnsQuery dns;
private ImmutablePreparedQuery(
@Nullable Template template,
String name,
@Nullable String session,
@Nullable String token,
ServiceQuery service,
@Nullable DnsQuery dns) {
this.template = template;
this.name = name;
this.session = session;
this.token = token;
this.service = service;
this.dns = dns;
}
/**
* @return The value of the {@code template} attribute
*/
@JsonProperty("Template")
@Override
public Optional