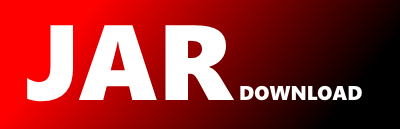
com.orbitz.consul.option.ImmutableQueryParameterOptions Maven / Gradle / Ivy
Show all versions of consul-client Show documentation
package com.orbitz.consul.option;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link QueryParameterOptions}.
*
* Use the builder to create immutable instances:
* {@code ImmutableQueryParameterOptions.builder()}.
*/
@Generated(from = "QueryParameterOptions", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableQueryParameterOptions extends QueryParameterOptions {
private final ImmutableMap toQuery;
private final ImmutableMap toHeaders;
private final @Nullable Boolean replaceExistingChecks;
private final @Nullable Boolean prune;
private ImmutableQueryParameterOptions(
ImmutableMap toQuery,
ImmutableMap toHeaders,
@Nullable Boolean replaceExistingChecks,
@Nullable Boolean prune) {
this.toQuery = toQuery;
this.toHeaders = toHeaders;
this.replaceExistingChecks = replaceExistingChecks;
this.prune = prune;
}
/**
* @return The value of the {@code toQuery} attribute
*/
@Override
public ImmutableMap toQuery() {
return toQuery;
}
/**
* @return The value of the {@code toHeaders} attribute
*/
@Override
public ImmutableMap toHeaders() {
return toHeaders;
}
/**
* @return The value of the {@code replaceExistingChecks} attribute
*/
@Override
public Optional getReplaceExistingChecks() {
return Optional.ofNullable(replaceExistingChecks);
}
/**
* @return The value of the {@code prune} attribute
*/
@Override
public Optional getPrune() {
return Optional.ofNullable(prune);
}
/**
* Copy the current immutable object by replacing the {@link QueryParameterOptions#toQuery() toQuery} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the toQuery map
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withToQuery(Map entries) {
if (this.toQuery == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableQueryParameterOptions(newValue, this.toHeaders, this.replaceExistingChecks, this.prune);
}
/**
* Copy the current immutable object by replacing the {@link QueryParameterOptions#toHeaders() toHeaders} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the toHeaders map
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withToHeaders(Map entries) {
if (this.toHeaders == entries) return this;
ImmutableMap newValue = ImmutableMap.copyOf(entries);
return new ImmutableQueryParameterOptions(this.toQuery, newValue, this.replaceExistingChecks, this.prune);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link QueryParameterOptions#getReplaceExistingChecks() replaceExistingChecks} attribute.
* @param value The value for replaceExistingChecks
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withReplaceExistingChecks(boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.replaceExistingChecks, newValue)) return this;
return new ImmutableQueryParameterOptions(this.toQuery, this.toHeaders, newValue, this.prune);
}
/**
* Copy the current immutable object by setting an optional value for the {@link QueryParameterOptions#getReplaceExistingChecks() replaceExistingChecks} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for replaceExistingChecks
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withReplaceExistingChecks(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.replaceExistingChecks, value)) return this;
return new ImmutableQueryParameterOptions(this.toQuery, this.toHeaders, value, this.prune);
}
/**
* Copy the current immutable object by setting a present value for the optional {@link QueryParameterOptions#getPrune() prune} attribute.
* @param value The value for prune
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withPrune(boolean value) {
@Nullable Boolean newValue = value;
if (Objects.equals(this.prune, newValue)) return this;
return new ImmutableQueryParameterOptions(this.toQuery, this.toHeaders, this.replaceExistingChecks, newValue);
}
/**
* Copy the current immutable object by setting an optional value for the {@link QueryParameterOptions#getPrune() prune} attribute.
* An equality check is used on inner nullable value to prevent copying of the same value by returning {@code this}.
* @param optional A value for prune
* @return A modified copy of {@code this} object
*/
public final ImmutableQueryParameterOptions withPrune(Optional optional) {
@Nullable Boolean value = optional.orElse(null);
if (Objects.equals(this.prune, value)) return this;
return new ImmutableQueryParameterOptions(this.toQuery, this.toHeaders, this.replaceExistingChecks, value);
}
/**
* This instance is equal to all instances of {@code ImmutableQueryParameterOptions} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableQueryParameterOptions
&& equalTo((ImmutableQueryParameterOptions) another);
}
private boolean equalTo(ImmutableQueryParameterOptions another) {
return toQuery.equals(another.toQuery)
&& toHeaders.equals(another.toHeaders)
&& Objects.equals(replaceExistingChecks, another.replaceExistingChecks)
&& Objects.equals(prune, another.prune);
}
/**
* Computes a hash code from attributes: {@code toQuery}, {@code toHeaders}, {@code replaceExistingChecks}, {@code prune}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + toQuery.hashCode();
h += (h << 5) + toHeaders.hashCode();
h += (h << 5) + Objects.hashCode(replaceExistingChecks);
h += (h << 5) + Objects.hashCode(prune);
return h;
}
/**
* Prints the immutable value {@code QueryParameterOptions} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("QueryParameterOptions")
.omitNullValues()
.add("toQuery", toQuery)
.add("toHeaders", toHeaders)
.add("replaceExistingChecks", replaceExistingChecks)
.add("prune", prune)
.toString();
}
/**
* Creates an immutable copy of a {@link QueryParameterOptions} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable QueryParameterOptions instance
*/
public static ImmutableQueryParameterOptions copyOf(QueryParameterOptions instance) {
if (instance instanceof ImmutableQueryParameterOptions) {
return (ImmutableQueryParameterOptions) instance;
}
return ImmutableQueryParameterOptions.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableQueryParameterOptions ImmutableQueryParameterOptions}.
*
* ImmutableQueryParameterOptions.builder()
* .putToQuery|putAllToQuery(String => Object) // {@link QueryParameterOptions#toQuery() toQuery} mappings
* .putToHeaders|putAllToHeaders(String => String) // {@link QueryParameterOptions#toHeaders() toHeaders} mappings
* .replaceExistingChecks(Boolean) // optional {@link QueryParameterOptions#getReplaceExistingChecks() replaceExistingChecks}
* .prune(Boolean) // optional {@link QueryParameterOptions#getPrune() prune}
* .build();
*
* @return A new ImmutableQueryParameterOptions builder
*/
public static ImmutableQueryParameterOptions.Builder builder() {
return new ImmutableQueryParameterOptions.Builder();
}
/**
* Builds instances of type {@link ImmutableQueryParameterOptions ImmutableQueryParameterOptions}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
*
{@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "QueryParameterOptions", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private ImmutableMap.Builder toQuery = ImmutableMap.builder();
private ImmutableMap.Builder toHeaders = ImmutableMap.builder();
private @Nullable Boolean replaceExistingChecks;
private @Nullable Boolean prune;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code com.orbitz.consul.option.QueryParameterOptions} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(QueryParameterOptions instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
/**
* Fill a builder with attribute values from the provided {@code com.orbitz.consul.option.ParamAdder} instance.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(ParamAdder instance) {
Objects.requireNonNull(instance, "instance");
from((Object) instance);
return this;
}
private void from(Object object) {
if (object instanceof QueryParameterOptions) {
QueryParameterOptions instance = (QueryParameterOptions) object;
Optional pruneOptional = instance.getPrune();
if (pruneOptional.isPresent()) {
prune(pruneOptional);
}
Optional replaceExistingChecksOptional = instance.getReplaceExistingChecks();
if (replaceExistingChecksOptional.isPresent()) {
replaceExistingChecks(replaceExistingChecksOptional);
}
}
if (object instanceof ParamAdder) {
ParamAdder instance = (ParamAdder) object;
putAllToQuery(instance.toQuery());
putAllToHeaders(instance.toHeaders());
}
}
/**
* Put one entry to the {@link QueryParameterOptions#toQuery() toQuery} map.
* @param key The key in the toQuery map
* @param value The associated value in the toQuery map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToQuery(String key, Object value) {
this.toQuery.put(key, value);
return this;
}
/**
* Put one entry to the {@link QueryParameterOptions#toQuery() toQuery} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToQuery(Map.Entry entry) {
this.toQuery.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link QueryParameterOptions#toQuery() toQuery} map. Nulls are not permitted
* @param entries The entries that will be added to the toQuery map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toQuery(Map entries) {
this.toQuery = ImmutableMap.builder();
return putAllToQuery(entries);
}
/**
* Put all mappings from the specified map as entries to {@link QueryParameterOptions#toQuery() toQuery} map. Nulls are not permitted
* @param entries The entries that will be added to the toQuery map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllToQuery(Map entries) {
this.toQuery.putAll(entries);
return this;
}
/**
* Put one entry to the {@link QueryParameterOptions#toHeaders() toHeaders} map.
* @param key The key in the toHeaders map
* @param value The associated value in the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(String key, String value) {
this.toHeaders.put(key, value);
return this;
}
/**
* Put one entry to the {@link QueryParameterOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putToHeaders(Map.Entry entry) {
this.toHeaders.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link QueryParameterOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder toHeaders(Map entries) {
this.toHeaders = ImmutableMap.builder();
return putAllToHeaders(entries);
}
/**
* Put all mappings from the specified map as entries to {@link QueryParameterOptions#toHeaders() toHeaders} map. Nulls are not permitted
* @param entries The entries that will be added to the toHeaders map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllToHeaders(Map entries) {
this.toHeaders.putAll(entries);
return this;
}
/**
* Initializes the optional value {@link QueryParameterOptions#getReplaceExistingChecks() replaceExistingChecks} to replaceExistingChecks.
* @param replaceExistingChecks The value for replaceExistingChecks
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder replaceExistingChecks(boolean replaceExistingChecks) {
this.replaceExistingChecks = replaceExistingChecks;
return this;
}
/**
* Initializes the optional value {@link QueryParameterOptions#getReplaceExistingChecks() replaceExistingChecks} to replaceExistingChecks.
* @param replaceExistingChecks The value for replaceExistingChecks
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder replaceExistingChecks(Optional replaceExistingChecks) {
this.replaceExistingChecks = replaceExistingChecks.orElse(null);
return this;
}
/**
* Initializes the optional value {@link QueryParameterOptions#getPrune() prune} to prune.
* @param prune The value for prune
* @return {@code this} builder for chained invocation
*/
@CanIgnoreReturnValue
public final Builder prune(boolean prune) {
this.prune = prune;
return this;
}
/**
* Initializes the optional value {@link QueryParameterOptions#getPrune() prune} to prune.
* @param prune The value for prune
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder prune(Optional prune) {
this.prune = prune.orElse(null);
return this;
}
/**
* Builds a new {@link ImmutableQueryParameterOptions ImmutableQueryParameterOptions}.
* @return An immutable instance of QueryParameterOptions
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableQueryParameterOptions build() {
return new ImmutableQueryParameterOptions(toQuery.build(), toHeaders.build(), replaceExistingChecks, prune);
}
}
}