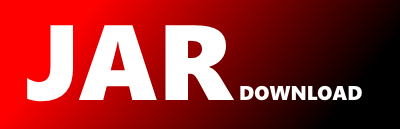
com.orientechnologies.spatial.engine.OLuceneSpatialIndexEngineDelegator Maven / Gradle / Ivy
Show all versions of orientdb-lucene Show documentation
/*
* Copyright 2010-2016 OrientDB LTD (http://orientdb.com)
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS"
* BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language
* governing permissions and limitations under the License.
*
* For more information: http://www.orientdb.com
*/
package com.orientechnologies.spatial.engine;
import com.orientechnologies.common.exception.OException;
import com.orientechnologies.common.util.ORawPair;
import com.orientechnologies.lucene.engine.OLuceneIndexEngine;
import com.orientechnologies.lucene.query.OLuceneQueryContext;
import com.orientechnologies.lucene.tx.OLuceneTxChanges;
import com.orientechnologies.orient.core.config.IndexEngineData;
import com.orientechnologies.orient.core.db.record.OIdentifiable;
import com.orientechnologies.orient.core.id.OContextualRecordId;
import com.orientechnologies.orient.core.id.ORID;
import com.orientechnologies.orient.core.index.OIndexException;
import com.orientechnologies.orient.core.index.OIndexKeyUpdater;
import com.orientechnologies.orient.core.index.OIndexMetadata;
import com.orientechnologies.orient.core.index.engine.IndexEngineValidator;
import com.orientechnologies.orient.core.index.engine.IndexEngineValuesTransformer;
import com.orientechnologies.orient.core.metadata.schema.OClass;
import com.orientechnologies.orient.core.storage.OStorage;
import com.orientechnologies.orient.core.storage.impl.local.paginated.atomicoperations.OAtomicOperation;
import com.orientechnologies.spatial.shape.OShapeFactory;
import java.io.IOException;
import java.util.Set;
import java.util.stream.Stream;
import org.apache.lucene.analysis.Analyzer;
import org.apache.lucene.document.Document;
import org.apache.lucene.search.IndexSearcher;
import org.apache.lucene.search.Query;
import org.apache.lucene.search.ScoreDoc;
import org.apache.lucene.spatial.SpatialStrategy;
/** Created by Enrico Risa on 04/09/15. */
public class OLuceneSpatialIndexEngineDelegator
implements OLuceneIndexEngine, OLuceneSpatialIndexContainer {
private final OStorage storage;
private final String indexName;
private OLuceneSpatialIndexEngineAbstract delegate;
private final int id;
public OLuceneSpatialIndexEngineDelegator(int id, String name, OStorage storage, int version) {
this.id = id;
this.indexName = name;
this.storage = storage;
}
@Override
public int getId() {
return id;
}
@Override
public void init(OIndexMetadata im) {
if (delegate == null) {
if (OClass.INDEX_TYPE.SPATIAL.name().equalsIgnoreCase(im.getType())) {
if (im.getIndexDefinition().getFields().size() > 1) {
delegate =
new OLuceneLegacySpatialIndexEngine(storage, indexName, id, OShapeFactory.INSTANCE);
} else {
delegate =
new OLuceneGeoSpatialIndexEngine(storage, indexName, id, OShapeFactory.INSTANCE);
}
delegate.init(im);
} else {
throw new IllegalStateException("Invalid index type " + im.getType());
}
}
}
@Override
public void flush() {
delegate.flush();
}
public void create(OAtomicOperation atomicOperation, IndexEngineData data) throws IOException {}
@Override
public void delete(OAtomicOperation atomicOperation) {
if (delegate != null) delegate.delete(atomicOperation);
}
@Override
public void load(IndexEngineData data) {
if (delegate != null) {
delegate.load(data);
}
}
@Override
public boolean remove(OAtomicOperation atomicOperation, Object key) {
return delegate.remove(atomicOperation, key);
}
@Override
public void clear(OAtomicOperation atomicOperation) {
delegate.clear(atomicOperation);
}
@Override
public void close() {
delegate.close();
}
@Override
public Object get(Object key) {
return delegate.get(key);
}
@Override
public void put(OAtomicOperation atomicOperation, Object key, Object value) {
try {
delegate.put(atomicOperation, key, value);
} catch (IOException e) {
throw OException.wrapException(
new OIndexException("Error during insertion of key " + key + " in index " + indexName),
e);
}
}
@Override
public void update(
OAtomicOperation atomicOperation, Object key, OIndexKeyUpdater