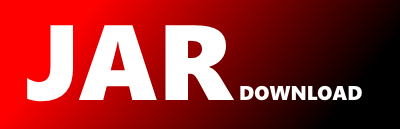
spice.util.BufferQueue.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spice-core_3 Show documentation
Show all versions of spice-core_3 Show documentation
Core functionality leveraged and shared by most other sub-projects of YouI.
package spice.util
import cats.effect.IO
import java.util.concurrent.ConcurrentLinkedQueue
import java.util.concurrent.atomic.AtomicInteger
import scala.annotation.tailrec
case class BufferQueue[T](manager: BufferManager, handler: List[T] => IO[Unit]) {
private val queue = new ConcurrentLinkedQueue[T]
private val size = new AtomicInteger(0)
def enqueue(item: T): Int = {
queue.add(item)
size.incrementAndGet()
}
def enqueue(items: List[T]): Int = items.map(enqueue).last
def ready: Boolean = size.get() > manager.triggerAfter
def isEmpty: Boolean = queue.isEmpty
def nonEmpty: Boolean = !isEmpty
def process(): IO[Unit] = {
val items = pollQueue(Nil, manager.maxPerBatch)
if (manager.sendEmpty || items.nonEmpty) {
handler(items)
} else {
IO.unit
}
}
@tailrec
private def pollQueue(list: List[T], remaining: Int): List[T] = if (remaining <= 0 || queue.isEmpty) {
list.reverse
} else {
val item = queue.poll()
size.decrementAndGet()
pollQueue(item :: list, remaining - 1)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy