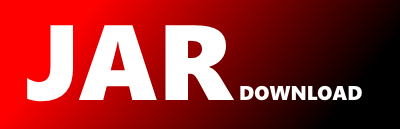
generator.dart.service.template Maven / Gradle / Ivy
import 'dart:convert';
import 'dart:typed_data';
import 'package:file_saver/file_saver.dart';
import 'package:http/http.dart' as http;
import 'package:file_picker/file_picker.dart';
%%IMPORTS%%
// GENERATED CODE: Do not edit!
class Service {
static Uri base = Uri.base;
// Should be updated before a download call
static String downloadFileName = 'download';
static Uri host(String path) => base.replace(path: path);
static Future restful(
String path,
Map request,
Response Function(Map json) convert
) async {
try {
final String body = jsonEncode(request);
final http.Response response = await http.post(
Service.host(path),
headers: {
"Content-Type": "application/json; charset=UTF-8",
"Accept": "application/json"
},
body: body
);
return convert(jsonDecode(response.body));
} on Exception catch(exc) {
String message = exc.toString();
Map json = jsonDecode('{"error": {"message": "$message"} }');
return convert(json);
}
}
static Future multiPart(
String path,
Function(http.MultipartRequest request) build,
Response Function(Map json) convert
) async {
final http.MultipartRequest request = http.MultipartRequest("POST", Service.host(path));
build(request);
final http.StreamedResponse response = await request.send();
final Uint8List responseData = await response.stream.toBytes();
final String jsonString = String.fromCharCodes(responseData);
return convert(jsonDecode(jsonString));
}
static Future restDownload(
String fileName,
String path,
Map request,
) async {
final String body = jsonEncode(request);
final http.Response response = await http.post(
Service.host(path),
headers: {
"Content-Type": "application/json; charset=UTF-8",
"Accept": "application/json"
},
body: body
);
Uint8List bytes = response.bodyBytes;
await FileSaver.instance.saveFile(name: fileName, bytes: bytes);
}
%%SERVICES%%
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy