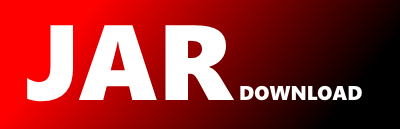
com.ovea.tajin.framework.scheduling.AsyncJobScheduler Maven / Gradle / Ivy
package com.ovea.tajin.framework.scheduling;
import java.util.concurrent.*;
import java.lang.*;
import java.io.*;
import java.net.*;
import java.util.*;
import groovy.lang.*;
import groovy.util.*;
@javax.inject.Singleton() @com.ovea.tajin.framework.jmx.annotation.JmxBean() public class AsyncJobScheduler
extends java.lang.Object implements
com.ovea.tajin.framework.scheduling.JobScheduler, com.ovea.tajin.framework.jmx.JmxSelfNaming, groovy.lang.GroovyObject {
public AsyncJobScheduler
() {}
public groovy.lang.MetaClass getMetaClass() { return (groovy.lang.MetaClass)null;}
public void setMetaClass(groovy.lang.MetaClass mc) { }
public java.lang.Object invokeMethod(java.lang.String method, java.lang.Object arguments) { return null;}
public java.lang.Object getProperty(java.lang.String property) { return null;}
public void setProperty(java.lang.String property, java.lang.Object value) { }
public com.google.common.cache.LoadingCache getExecutors() { return (com.google.common.cache.LoadingCache)null;}
public void setExecutors(com.google.common.cache.LoadingCache value) { }
public com.ovea.tajin.framework.scheduling.JobRepository getRepository() { return (com.ovea.tajin.framework.scheduling.JobRepository)null;}
public void setRepository(com.ovea.tajin.framework.scheduling.JobRepository value) { }
public com.ovea.tajin.framework.util.PropertySettings getSettings() { return (com.ovea.tajin.framework.util.PropertySettings)null;}
public void setSettings(com.ovea.tajin.framework.util.PropertySettings value) { }
public com.ovea.tajin.framework.scheduling.JobScheduler.OnError getOnError() { return (com.ovea.tajin.framework.scheduling.JobScheduler.OnError)null;}
public void setOnError(com.ovea.tajin.framework.scheduling.JobScheduler.OnError value) { }
@com.ovea.tajin.framework.jmx.annotation.JmxProperty() public long getScheduledCount() { return (long)0;}
@com.ovea.tajin.framework.jmx.annotation.JmxProperty() public long getRunningCount() { return (long)0;}
@com.ovea.tajin.framework.jmx.annotation.JmxProperty() public long getTotalExecutionCount() { return (long)0;}
@com.ovea.tajin.framework.jmx.annotation.JmxProperty() public long getFailedCount() { return (long)0;}
@com.ovea.tajin.framework.jmx.annotation.JmxProperty() public java.util.Collection getScheduledJobs() { return (java.util.Collection)null;}
@java.lang.Override() public javax.management.ObjectName getObjectName()throws javax.management.MalformedObjectNameException { return (javax.management.ObjectName)null;}
@javax.annotation.PostConstruct() public void init() { }
@javax.annotation.PreDestroy() public void close() { }
@java.lang.Override() public com.ovea.tajin.framework.scheduling.Job schedule(java.lang.String jobName, java.util.Map data) { return (com.ovea.tajin.framework.scheduling.Job)null;}
@java.lang.Override() public void cancel(java.util.Collection jobIds) { }
@java.lang.Override() public com.ovea.tajin.framework.scheduling.Job schedule(java.lang.String jobName, java.util.Date time, java.util.Map data) { return (com.ovea.tajin.framework.scheduling.Job)null;}
private class JobRunner
extends java.lang.Object implements
java.lang.Runnable, groovy.lang.GroovyObject {
public JobRunner
(com.ovea.tajin.framework.scheduling.Job job) {
super ();
}
public groovy.lang.MetaClass getMetaClass() { return (groovy.lang.MetaClass)null;}
public void setMetaClass(groovy.lang.MetaClass mc) { }
public java.lang.Object invokeMethod(java.lang.String method, java.lang.Object arguments) { return null;}
public java.lang.Object getProperty(java.lang.String property) { return null;}
public void setProperty(java.lang.String property, java.lang.Object value) { }
public final com.ovea.tajin.framework.scheduling.Job getJob() { return (com.ovea.tajin.framework.scheduling.Job)null;}
@java.lang.Override() public void run() { }
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy