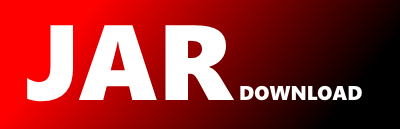
org.eclipse.jetty.spdy.IStream Maven / Gradle / Ivy
//
// ========================================================================
// Copyright (c) 1995-2013 Mort Bay Consulting Pty. Ltd.
// ------------------------------------------------------------------------
// All rights reserved. This program and the accompanying materials
// are made available under the terms of the Eclipse Public License v1.0
// and Apache License v2.0 which accompanies this distribution.
//
// The Eclipse Public License is available at
// http://www.eclipse.org/legal/epl-v10.html
//
// The Apache License v2.0 is available at
// http://www.opensource.org/licenses/apache2.0.php
//
// You may elect to redistribute this code under either of these licenses.
// ========================================================================
//
package org.eclipse.jetty.spdy;
import org.eclipse.jetty.spdy.api.DataInfo;
import org.eclipse.jetty.spdy.api.SessionFrameListener;
import org.eclipse.jetty.spdy.api.Stream;
import org.eclipse.jetty.spdy.api.StreamFrameListener;
import org.eclipse.jetty.spdy.api.SynInfo;
import org.eclipse.jetty.spdy.frames.ControlFrame;
import org.eclipse.jetty.util.Callback;
/**
* The internal interface that represents a stream.
* {@link IStream} contains additional methods used by a SPDY
* implementation (and not by an application).
*/
public interface IStream extends Stream, Callback
{
/**
* Senders of data frames need to know the current window size
* to determine whether they can send more data.
*
* @return the current window size for this stream.
* @see #updateWindowSize(int)
*/
public int getWindowSize();
/**
* Updates the window size for this stream by the given amount,
* that can be positive or negative.
* Senders and recipients of data frames update the window size,
* respectively, with negative values and positive values.
*
* @param delta the signed amount the window size needs to be updated
* @see #getWindowSize()
*/
public void updateWindowSize(int delta);
/**
* @param listener the stream frame listener associated to this stream
* as returned by {@link SessionFrameListener#onSyn(Stream, SynInfo)}
*/
public void setStreamFrameListener(StreamFrameListener listener);
//TODO: javadoc thomas
public StreamFrameListener getStreamFrameListener();
/**
* A stream can be open, {@link #isHalfClosed() half closed} or
* {@link #isClosed() closed} and this method updates the close state
* of this stream.
* If the stream is open, calling this method with a value of true
* puts the stream into half closed state.
* If the stream is half closed, calling this method with a value
* of true puts the stream into closed state.
*
* @param close whether the close state should be updated
* @param local whether the close is local or remote
*/
public void updateCloseState(boolean close, boolean local);
/**
* Processes the given control frame,
* for example by updating the stream's state or by calling listeners.
*
* @param frame the control frame to process
* @see #process(DataInfo)
*/
public void process(ControlFrame frame);
/**
* Processes the given {@code dataInfo},
* for example by updating the stream's state or by calling listeners.
*
* @param dataInfo the DataInfo to process
* @see #process(ControlFrame)
*/
public void process(DataInfo dataInfo);
/**
* Associate the given {@link IStream} to this {@link IStream}.
*
* @param stream the stream to associate with this stream
*/
public void associate(IStream stream);
/**
* remove the given associated {@link IStream} from this stream
*
* @param stream the stream to be removed
*/
public void disassociate(IStream stream);
/**
* Overrides Stream.getAssociatedStream() to return an instance of IStream instead of Stream
*
* @see Stream#getAssociatedStream()
*/
@Override
public IStream getAssociatedStream();
}