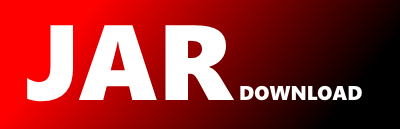
com.ovh.ws.jsonizer.api.base.OvhObject Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2012, OVH. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and limitations under the License.
*/
package com.ovh.ws.jsonizer.api.base;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.Arrays;
public abstract class OvhObject {
public static boolean equal(Object a, Object b) {
return a == b || a != null && a.equals(b);
}
/**
* Generates a hash code for multiple values. The hash code is generated by
* calling {@link Arrays#hashCode(Object[])}.
*/
public static int hashCode(Object... objects) {
return Arrays.hashCode(objects);
}
/**
* Ensures that an object reference passed as a parameter to the calling
* method is not null.
*
* @param reference
* an object reference
* @param errorMessageTemplate
* the exception message to use if the check fails; will
* be converted to a string using {@link String#valueOf(Object)}
* @return the non-null reference that was validated
* @throws IllegalArgumentException
* if {@code reference} is null
*/
public static T checkNotNull(T reference, String errorMessageTemplate, Object... errorMessageArgs) {
if (reference == null) {
throw new IllegalArgumentException(String.format(errorMessageTemplate, errorMessageArgs));
}
return reference;
}
/**
* Returns {@code true} if the given string is null or is the empty string.
*/
public static boolean isNullOrEmpty(String string) {
return string == null || string.length() == 0; // string.isEmpty() in Java 6
}
public static URL newUrl(String url) {
try {
checkNotNull(url, "Url is required");
return new URL(url);
} catch (MalformedURLException e) {
throw new IllegalArgumentException(e);
}
}
public static ToStringHelper toStringHelper(Object self) {
return new ToStringHelper(simpleName(self.getClass()));
}
private static String simpleName(Class> clazz) {
String name = clazz.getName();
// the nth anonymous class has a class name ending in "Outer$n"
// and local inner classes have names ending in "Outer.$1Inner"
name = name.replaceAll("\\$[0-9]+", "\\$");
// we want the name of the inner class all by its lonesome
int start = name.lastIndexOf('$');
// if this isn't an inner class, just find the start of the
// top level class name.
if (start == -1) {
start = name.lastIndexOf('.');
}
return name.substring(start + 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy