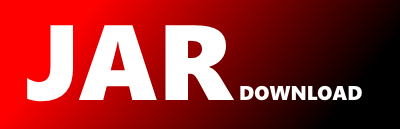
com.ovh.ws.jsonizer.api.http.HttpRequester Maven / Gradle / Ivy
The newest version!
/**
* Copyright 2012, OVH. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and limitations under the License.
*/
package com.ovh.ws.jsonizer.api.http;
import static com.ovh.ws.jsonizer.api.base.OvhObject.isNullOrEmpty;
import java.net.URI;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import com.ovh.ws.api.OvhWsException;
import com.ovh.ws.api.auth.AuthProvider;
import com.ovh.ws.api.http.RequestSigner;
import com.ovh.ws.jsonizer.api.JsonizerConfig;
import com.ovh.ws.jsonizer.api.request.RestRequest;
public class HttpRequester {
private static final String SESSION_PARAM = "X-OVH-SESSION";
private final JsonizerConfig config;
private final RequestSigner requestSigner;
private HttpClient httpClient;
public HttpRequester(JsonizerConfig config) {
this.config = config;
requestSigner = new RequestSigner();
}
public String execute(RestRequest request) throws OvhWsException {
SyncWebResource resource = getHttpClient().resource(request.toUri());
prepareResource(resource, request);
return resource.execute();
}
public void execute(RestRequest request, HttpResponseHandler responseHandler) {
try {
AsyncWebResource asyncResource = getHttpClient().asyncResource(request.toUri());
prepareResource(asyncResource, request);
asyncResource.execute(responseHandler);
} catch (OvhWsException caught) {
responseHandler.onFailure(caught);
}
}
private void prepareResource(WebResource resource, RestRequest> request) throws OvhWsException {
String paramsValue = serializeParams(request.getParams());
Map headers = new HashMap();
headers.putAll(getHttpClient().getHeaders());
AuthProvider authProvider = config.getAuthProvider();
if (authProvider != null) {
addSessionHeader(headers, authProvider);
headers.putAll(signingIfNecessary(request.toUri(), paramsValue, authProvider));
}
resource.headers(headers);
Map params = buildParams(paramsValue);
resource.queryParams(params);
}
private String serializeParams(Map params) throws OvhWsException {
return !params.isEmpty() ? config.getParser().writeValue(params) : "";
}
private void addSessionHeader(Map headers, AuthProvider authProvider) {
if (authProvider.getSessionId() != null) {
headers.put(SESSION_PARAM, authProvider.getSessionId());
}
}
private Map signingIfNecessary(URI url, String paramsValue, AuthProvider authProvider)
throws OvhWsException {
return requestSigner.getSigningHeaders(url.toString(), paramsValue, authProvider.getToken());
}
private Map buildParams(String paramsValue) {
Map params;
if (isNullOrEmpty(paramsValue)) {
params = Collections.emptyMap();
} else {
params = Collections.singletonMap("params", paramsValue);
}
return params;
}
public HttpClient getHttpClient() {
if (httpClient == null) {
return config.getHttpClient();
}
return httpClient;
}
public void setHttpClient(HttpClient httpClient) {
this.httpClient = httpClient;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy