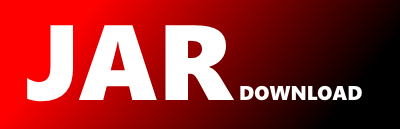
com.ovhcloud.pulumi.ovh.CloudProject.Database Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.ovhcloud.pulumi.ovh.CloudProject.DatabaseArgs;
import com.ovhcloud.pulumi.ovh.CloudProject.inputs.DatabaseState;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.DatabaseEndpoint;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.DatabaseIpRestriction;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.DatabaseNode;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* Minimum settings for each engine (region choice is up to the user):
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.CloudProject.Database;
* import com.pulumi.ovh.CloudProject.DatabaseArgs;
* import com.pulumi.ovh.CloudProject.inputs.DatabaseNodeArgs;
* import com.pulumi.ovh.CloudProject.inputs.DatabaseIpRestrictionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var cassandradb = new Database("cassandradb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-cassandra")
* .engine("cassandra")
* .version("4.0")
* .plan("essential")
* .nodes(
* DatabaseNodeArgs.builder()
* .region("BHS")
* .build(),
* DatabaseNodeArgs.builder()
* .region("BHS")
* .build(),
* DatabaseNodeArgs.builder()
* .region("BHS")
* .build())
* .flavor("db1-4")
* .build());
*
* var kafkadb = new Database("kafkadb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-kafka")
* .engine("kafka")
* .version("3.4")
* .plan("business")
* .kafkaRestApi(true)
* .kafkaSchemaRegistry(true)
* .nodes(
* DatabaseNodeArgs.builder()
* .region("DE")
* .build(),
* DatabaseNodeArgs.builder()
* .region("DE")
* .build(),
* DatabaseNodeArgs.builder()
* .region("DE")
* .build())
* .flavor("db1-4")
* .build());
*
* var m3db = new Database("m3db", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-m3db")
* .engine("m3db")
* .version("1.2")
* .plan("essential")
* .nodes(DatabaseNodeArgs.builder()
* .region("BHS")
* .build())
* .flavor("db1-7")
* .build());
*
* var mongodb = new Database("mongodb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-mongodb")
* .engine("mongodb")
* .version("5.0")
* .plan("discovery")
* .nodes(DatabaseNodeArgs.builder()
* .region("GRA")
* .build())
* .flavor("db1-2")
* .build());
*
* var mysqldb = new Database("mysqldb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-mysql")
* .engine("mysql")
* .version("8")
* .plan("essential")
* .nodes(DatabaseNodeArgs.builder()
* .region("SBG")
* .build())
* .flavor("db1-4")
* .advancedConfiguration(Map.ofEntries(
* Map.entry("mysql.sql_mode", "ANSI,ERROR_FOR_DIVISION_BY_ZERO,NO_ENGINE_SUBSTITUTION,NO_ZERO_DATE,NO_ZERO_IN_DATE,STRICT_ALL_TABLES"),
* Map.entry("mysql.sql_require_primary_key", "true")
* ))
* .build());
*
* var opensearchdb = new Database("opensearchdb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-opensearch")
* .engine("opensearch")
* .version("1")
* .plan("essential")
* .opensearchAclsEnabled(true)
* .nodes(DatabaseNodeArgs.builder()
* .region("UK")
* .build())
* .flavor("db1-4")
* .build());
*
* var pgsqldb = new Database("pgsqldb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-postgresql")
* .engine("postgresql")
* .version("14")
* .plan("essential")
* .nodes(DatabaseNodeArgs.builder()
* .region("WAW")
* .build())
* .flavor("db1-4")
* .ipRestrictions(
* DatabaseIpRestrictionArgs.builder()
* .description("ip 1")
* .ip("178.97.6.0/24")
* .build(),
* DatabaseIpRestrictionArgs.builder()
* .description("ip 2")
* .ip("178.97.7.0/24")
* .build())
* .build());
*
* var redisdb = new Database("redisdb", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-redis")
* .engine("redis")
* .version("6.2")
* .plan("essential")
* .nodes(DatabaseNodeArgs.builder()
* .region("BHS")
* .build())
* .flavor("db1-4")
* .build());
*
* var grafana = new Database("grafana", DatabaseArgs.builder()
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .description("my-first-grafana")
* .engine("grafana")
* .version("9.1")
* .plan("essential")
* .nodes(DatabaseNodeArgs.builder()
* .region("GRA")
* .build())
* .flavor("db1-4")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* To deploy a business PostgreSQL service with two nodes on public network:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.CloudProject.Database;
* import com.pulumi.ovh.CloudProject.DatabaseArgs;
* import com.pulumi.ovh.CloudProject.inputs.DatabaseNodeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var postgresql = new Database("postgresql", DatabaseArgs.builder()
* .description("my-first-postgresql")
* .engine("postgresql")
* .flavor("db1-15")
* .nodes(
* DatabaseNodeArgs.builder()
* .region("GRA")
* .build(),
* DatabaseNodeArgs.builder()
* .region("GRA")
* .build())
* .plan("business")
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .version("14")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* To deploy an enterprise MongoDB service with three nodes on private network:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.CloudProject.Database;
* import com.pulumi.ovh.CloudProject.DatabaseArgs;
* import com.pulumi.ovh.CloudProject.inputs.DatabaseNodeArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var mongodb = new Database("mongodb", DatabaseArgs.builder()
* .description("my-first-mongodb")
* .engine("mongodb")
* .flavor("db1-30")
* .nodes(
* DatabaseNodeArgs.builder()
* .networkId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .region("SBG")
* .subnetId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .build(),
* DatabaseNodeArgs.builder()
* .networkId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .region("SBG")
* .subnetId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .build(),
* DatabaseNodeArgs.builder()
* .networkId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .region("SBG")
* .subnetId("XXXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX")
* .build())
* .plan("production")
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .version("5.0")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* OVHcloud Managed database clusters can be imported using the `service_name`, `engine`, `id` of the cluster, separated by "/" E.g.,
*
* bash
*
* ```sh
* $ pulumi import ovh:CloudProject/database:Database my_database_cluster service_name/engine/id
* ```
*
*/
@ResourceType(type="ovh:CloudProject/database:Database")
public class Database extends com.pulumi.resources.CustomResource {
/**
* Advanced configuration key / value.
*
*/
@Export(name="advancedConfiguration", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy