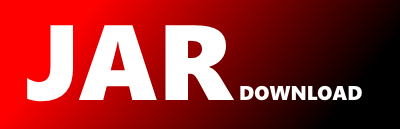
com.ovhcloud.pulumi.ovh.CloudProject.Gateway Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.ovhcloud.pulumi.ovh.CloudProject.GatewayArgs;
import com.ovhcloud.pulumi.ovh.CloudProject.inputs.GatewayState;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.GatewayExternalInformation;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.GatewayInterface;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import javax.annotation.Nullable;
/**
* Create a new Gateway for existing subnet in the specified public cloud project.
*
* ## Import
*
* A gateway can be imported using the `service_name`, `region` and `id` (identifier of the gateway) properties, separated by a `/`.
*
* bash
*
* ```sh
* $ pulumi import ovh:CloudProject/gateway:Gateway gateway service_name/region/id
* ```
*
*/
@ResourceType(type="ovh:CloudProject/gateway:Gateway")
public class Gateway extends com.pulumi.resources.CustomResource {
/**
* List of External Information of the gateway.
*
*/
@Export(name="externalInformations", refs={List.class,GatewayExternalInformation.class}, tree="[0,1]")
private Output> externalInformations;
/**
* @return List of External Information of the gateway.
*
*/
public Output> externalInformations() {
return this.externalInformations;
}
/**
* Interfaces list of the gateway.
*
*/
@Export(name="interfaces", refs={List.class,GatewayInterface.class}, tree="[0,1]")
private Output> interfaces;
/**
* @return Interfaces list of the gateway.
*
*/
public Output> interfaces() {
return this.interfaces;
}
/**
* Model of the gateway.
*
*/
@Export(name="model", refs={String.class}, tree="[0]")
private Output model;
/**
* @return Model of the gateway.
*
*/
public Output model() {
return this.model;
}
/**
* Name of the gateway.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the gateway.
*
*/
public Output name() {
return this.name;
}
/**
* ID of the private network.
*
*/
@Export(name="networkId", refs={String.class}, tree="[0]")
private Output networkId;
/**
* @return ID of the private network.
*
*/
public Output networkId() {
return this.networkId;
}
/**
* Region of the gateway.
*
*/
@Export(name="region", refs={String.class}, tree="[0]")
private Output region;
/**
* @return Region of the gateway.
*
*/
public Output region() {
return this.region;
}
/**
* ID of the private network.
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return ID of the private network.
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* Status of the gateway.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Status of the gateway.
*
*/
public Output status() {
return this.status;
}
/**
* ID of the subnet.
*
*/
@Export(name="subnetId", refs={String.class}, tree="[0]")
private Output subnetId;
/**
* @return ID of the subnet.
*
*/
public Output subnetId() {
return this.subnetId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Gateway(java.lang.String name) {
this(name, GatewayArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Gateway(java.lang.String name, GatewayArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Gateway(java.lang.String name, GatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/gateway:Gateway", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Gateway(java.lang.String name, Output id, @Nullable GatewayState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/gateway:Gateway", name, state, makeResourceOptions(options, id), false);
}
private static GatewayArgs makeArgs(GatewayArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? GatewayArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Gateway get(java.lang.String name, Output id, @Nullable GatewayState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Gateway(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy