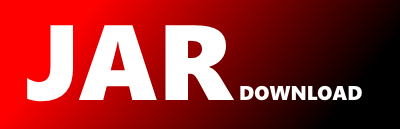
com.ovhcloud.pulumi.ovh.CloudProject.Kube Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.ovhcloud.pulumi.ovh.CloudProject.KubeArgs;
import com.ovhcloud.pulumi.ovh.CloudProject.inputs.KubeState;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubeCustomization;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubeCustomizationApiserver;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubeCustomizationKubeProxy;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubeKubeconfigAttribute;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubePrivateNetworkConfiguration;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Import
*
* OVHcloud Managed Kubernetes Service clusters can be imported using the `service_name` and the `id` of the cluster, separated by "/" E.g.,
*
* bash
*
* ```sh
* $ pulumi import ovh:CloudProject/kube:Kube my_kube_cluster service_name/kube_id
* ```
*
*/
@ResourceType(type="ovh:CloudProject/kube:Kube")
public class Kube extends com.pulumi.resources.CustomResource {
/**
* True if control-plane is up-to-date.
*
*/
@Export(name="controlPlaneIsUpToDate", refs={Boolean.class}, tree="[0]")
private Output controlPlaneIsUpToDate;
/**
* @return True if control-plane is up-to-date.
*
*/
public Output controlPlaneIsUpToDate() {
return this.controlPlaneIsUpToDate;
}
/**
* Kubernetes API server customization
*
*/
@Export(name="customizationApiservers", refs={List.class,KubeCustomizationApiserver.class}, tree="[0,1]")
private Output> customizationApiservers;
/**
* @return Kubernetes API server customization
*
*/
public Output> customizationApiservers() {
return this.customizationApiservers;
}
/**
* Kubernetes kube-proxy customization
*
*/
@Export(name="customizationKubeProxy", refs={KubeCustomizationKubeProxy.class}, tree="[0]")
private Output* @Nullable */ KubeCustomizationKubeProxy> customizationKubeProxy;
/**
* @return Kubernetes kube-proxy customization
*
*/
public Output> customizationKubeProxy() {
return Codegen.optional(this.customizationKubeProxy);
}
/**
* **Deprecated** (Optional) Use `customization_apiserver` and `customization_kube_proxy` instead. Kubernetes cluster customization
*
* @deprecated
* Use customization_apiserver instead
*
*/
@Deprecated /* Use customization_apiserver instead */
@Export(name="customizations", refs={List.class,KubeCustomization.class}, tree="[0,1]")
private Output> customizations;
/**
* @return **Deprecated** (Optional) Use `customization_apiserver` and `customization_kube_proxy` instead. Kubernetes cluster customization
*
*/
public Output> customizations() {
return this.customizations;
}
/**
* True if all nodes and control-plane are up-to-date.
*
*/
@Export(name="isUpToDate", refs={Boolean.class}, tree="[0]")
private Output isUpToDate;
/**
* @return True if all nodes and control-plane are up-to-date.
*
*/
public Output isUpToDate() {
return this.isUpToDate;
}
/**
* Selected mode for kube-proxy. **Changing this value recreates the resource, including ETCD user data.** Defaults to `iptables`.
*
*/
@Export(name="kubeProxyMode", refs={String.class}, tree="[0]")
private Output kubeProxyMode;
/**
* @return Selected mode for kube-proxy. **Changing this value recreates the resource, including ETCD user data.** Defaults to `iptables`.
*
*/
public Output kubeProxyMode() {
return this.kubeProxyMode;
}
/**
* The kubeconfig file. Use this file to connect to your kubernetes cluster.
*
*/
@Export(name="kubeconfig", refs={String.class}, tree="[0]")
private Output kubeconfig;
/**
* @return The kubeconfig file. Use this file to connect to your kubernetes cluster.
*
*/
public Output kubeconfig() {
return this.kubeconfig;
}
/**
* The kubeconfig file attributes.
*
*/
@Export(name="kubeconfigAttributes", refs={List.class,KubeKubeconfigAttribute.class}, tree="[0,1]")
private Output> kubeconfigAttributes;
/**
* @return The kubeconfig file attributes.
*
*/
public Output> kubeconfigAttributes() {
return this.kubeconfigAttributes;
}
/**
* Openstack private network (or vRack) ID to use for load balancers.
*
*/
@Export(name="loadBalancersSubnetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> loadBalancersSubnetId;
/**
* @return Openstack private network (or vRack) ID to use for load balancers.
*
*/
public Output> loadBalancersSubnetId() {
return Codegen.optional(this.loadBalancersSubnetId);
}
/**
* The name of the kubernetes cluster.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the kubernetes cluster.
*
*/
public Output name() {
return this.name;
}
/**
* Kubernetes versions available for upgrade.
*
*/
@Export(name="nextUpgradeVersions", refs={List.class,String.class}, tree="[0,1]")
private Output> nextUpgradeVersions;
/**
* @return Kubernetes versions available for upgrade.
*
*/
public Output> nextUpgradeVersions() {
return this.nextUpgradeVersions;
}
/**
* Openstack private network (or vRack) ID to use for nodes. **Cannot be updated, it can only be used at cluster creation or reset.**
*
*/
@Export(name="nodesSubnetId", refs={String.class}, tree="[0]")
private Output nodesSubnetId;
/**
* @return Openstack private network (or vRack) ID to use for nodes. **Cannot be updated, it can only be used at cluster creation or reset.**
*
*/
public Output nodesSubnetId() {
return this.nodesSubnetId;
}
/**
* Cluster nodes URL.
*
*/
@Export(name="nodesUrl", refs={String.class}, tree="[0]")
private Output nodesUrl;
/**
* @return Cluster nodes URL.
*
*/
public Output nodesUrl() {
return this.nodesUrl;
}
/**
* The private network configuration. If this is set then the 2 parameters below shall be defined.
*
*/
@Export(name="privateNetworkConfiguration", refs={KubePrivateNetworkConfiguration.class}, tree="[0]")
private Output* @Nullable */ KubePrivateNetworkConfiguration> privateNetworkConfiguration;
/**
* @return The private network configuration. If this is set then the 2 parameters below shall be defined.
*
*/
public Output> privateNetworkConfiguration() {
return Codegen.optional(this.privateNetworkConfiguration);
}
/**
* OpenStack private network (or vRack) ID to use. **Changing this value recreates the resource, including ETCD user data.** Defaults - not use private network.
*
* > __WARNING__ Updating the private network ID resets the cluster so that all user data is deleted.
*
*/
@Export(name="privateNetworkId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> privateNetworkId;
/**
* @return OpenStack private network (or vRack) ID to use. **Changing this value recreates the resource, including ETCD user data.** Defaults - not use private network.
*
* > __WARNING__ Updating the private network ID resets the cluster so that all user data is deleted.
*
*/
public Output> privateNetworkId() {
return Codegen.optional(this.privateNetworkId);
}
/**
* a valid OVHcloud public cloud region ID in which the kubernetes cluster will be available. Ex.: "GRA1". Defaults to all public cloud regions. **Changing this value recreates the resource.**
*
*/
@Export(name="region", refs={String.class}, tree="[0]")
private Output region;
/**
* @return a valid OVHcloud public cloud region ID in which the kubernetes cluster will be available. Ex.: "GRA1". Defaults to all public cloud regions. **Changing this value recreates the resource.**
*
*/
public Output region() {
return this.region;
}
/**
* The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used. **Changing this value recreates the resource.**
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used. **Changing this value recreates the resource.**
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* Cluster status. Should be normally set to 'READY'.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Cluster status. Should be normally set to 'READY'.
*
*/
public Output status() {
return this.status;
}
/**
* Cluster update policy. Choose between [ALWAYS_UPDATE, MINIMAL_DOWNTIME, NEVER_UPDATE].
*
*/
@Export(name="updatePolicy", refs={String.class}, tree="[0]")
private Output updatePolicy;
/**
* @return Cluster update policy. Choose between [ALWAYS_UPDATE, MINIMAL_DOWNTIME, NEVER_UPDATE].
*
*/
public Output updatePolicy() {
return this.updatePolicy;
}
/**
* Management URL of your cluster.
*
*/
@Export(name="url", refs={String.class}, tree="[0]")
private Output url;
/**
* @return Management URL of your cluster.
*
*/
public Output url() {
return this.url;
}
/**
* kubernetes version to use. Changing this value updates the resource. Defaults to the latest available.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return kubernetes version to use. Changing this value updates the resource. Defaults to the latest available.
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Kube(java.lang.String name) {
this(name, KubeArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Kube(java.lang.String name, KubeArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Kube(java.lang.String name, KubeArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/kube:Kube", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Kube(java.lang.String name, Output id, @Nullable KubeState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/kube:Kube", name, state, makeResourceOptions(options, id), false);
}
private static KubeArgs makeArgs(KubeArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? KubeArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"kubeconfig",
"kubeconfigAttributes"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Kube get(java.lang.String name, Output id, @Nullable KubeState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Kube(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy