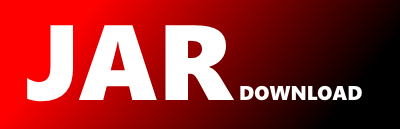
com.ovhcloud.pulumi.ovh.CloudProject.KubeNodePool Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.ovhcloud.pulumi.ovh.CloudProject.KubeNodePoolArgs;
import com.ovhcloud.pulumi.ovh.CloudProject.inputs.KubeNodePoolState;
import com.ovhcloud.pulumi.ovh.CloudProject.outputs.KubeNodePoolTemplate;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a nodepool in a OVHcloud Managed Kubernetes Service cluster.
*
* ## Example Usage
*
* Create a simple node pool in your Kubernetes cluster:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.CloudProject.KubeNodePool;
* import com.pulumi.ovh.CloudProject.KubeNodePoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var nodePool = new KubeNodePool("nodePool", KubeNodePoolArgs.builder()
* .desiredNodes(3)
* .flavorName("b2-7")
* .kubeId("xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
* .maxNodes(3)
* .minNodes(3)
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Create an advanced node pool in your Kubernetes cluster:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.CloudProject.KubeNodePool;
* import com.pulumi.ovh.CloudProject.KubeNodePoolArgs;
* import com.pulumi.ovh.CloudProject.inputs.KubeNodePoolTemplateArgs;
* import com.pulumi.ovh.CloudProject.inputs.KubeNodePoolTemplateMetadataArgs;
* import com.pulumi.ovh.CloudProject.inputs.KubeNodePoolTemplateSpecArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var pool = new KubeNodePool("pool", KubeNodePoolArgs.builder()
* .desiredNodes(3)
* .flavorName("b2-7")
* .kubeId("xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx")
* .maxNodes(3)
* .minNodes(3)
* .serviceName("xxxxxxxxxxxxxxxxxxxxxxxxxxxxxx")
* .template(KubeNodePoolTemplateArgs.builder()
* .metadata(KubeNodePoolTemplateMetadataArgs.builder()
* .annotations(Map.ofEntries(
* Map.entry("k1", "v1"),
* Map.entry("k2", "v2")
* ))
* .finalizers()
* .labels(Map.ofEntries(
* Map.entry("k3", "v3"),
* Map.entry("k4", "v4")
* ))
* .build())
* .spec(KubeNodePoolTemplateSpecArgs.builder()
* .taints(Map.ofEntries(
* Map.entry("effect", "PreferNoSchedule"),
* Map.entry("key", "k"),
* Map.entry("value", "v")
* ))
* .unschedulable(false)
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* OVHcloud Managed Kubernetes Service cluster node pool can be imported using the `service_name`, the `id` of the cluster, and the `id` of the nodepool separated by "/" E.g.,
*
* bash
*
* ```sh
* $ pulumi import ovh:CloudProject/kubeNodePool:KubeNodePool pool service_name/kube_id/poolid
* ```
*
*/
@ResourceType(type="ovh:CloudProject/kubeNodePool:KubeNodePool")
public class KubeNodePool extends com.pulumi.resources.CustomResource {
/**
* should the pool use the anti-affinity feature. Default to `false`. **Changing this value recreates the resource.**
*
*/
@Export(name="antiAffinity", refs={Boolean.class}, tree="[0]")
private Output antiAffinity;
/**
* @return should the pool use the anti-affinity feature. Default to `false`. **Changing this value recreates the resource.**
*
*/
public Output antiAffinity() {
return this.antiAffinity;
}
/**
* Enable auto-scaling for the pool. Default to `false`.
*
*/
@Export(name="autoscale", refs={Boolean.class}, tree="[0]")
private Output autoscale;
/**
* @return Enable auto-scaling for the pool. Default to `false`.
*
*/
public Output autoscale() {
return this.autoscale;
}
/**
* scaleDownUnneededTimeSeconds autoscaling parameter
* How long a node should be unneeded before it is eligible for scale down
*
*/
@Export(name="autoscalingScaleDownUnneededTimeSeconds", refs={Integer.class}, tree="[0]")
private Output autoscalingScaleDownUnneededTimeSeconds;
/**
* @return scaleDownUnneededTimeSeconds autoscaling parameter
* How long a node should be unneeded before it is eligible for scale down
*
*/
public Output autoscalingScaleDownUnneededTimeSeconds() {
return this.autoscalingScaleDownUnneededTimeSeconds;
}
/**
* scaleDownUnreadyTimeSeconds autoscaling parameter
* How long an unready node should be unneeded before it is eligible for scale down
*
*/
@Export(name="autoscalingScaleDownUnreadyTimeSeconds", refs={Integer.class}, tree="[0]")
private Output autoscalingScaleDownUnreadyTimeSeconds;
/**
* @return scaleDownUnreadyTimeSeconds autoscaling parameter
* How long an unready node should be unneeded before it is eligible for scale down
*
*/
public Output autoscalingScaleDownUnreadyTimeSeconds() {
return this.autoscalingScaleDownUnreadyTimeSeconds;
}
/**
* scaleDownUtilizationThreshold autoscaling parameter
* Node utilization level, defined as sum of requested resources divided by capacity, below which a node can be considered for scale down
* * ` template ` - (Optional) Managed Kubernetes nodepool template, which is a complex object constituted by two main nested objects:
*
*/
@Export(name="autoscalingScaleDownUtilizationThreshold", refs={Double.class}, tree="[0]")
private Output autoscalingScaleDownUtilizationThreshold;
/**
* @return scaleDownUtilizationThreshold autoscaling parameter
* Node utilization level, defined as sum of requested resources divided by capacity, below which a node can be considered for scale down
* * ` template ` - (Optional) Managed Kubernetes nodepool template, which is a complex object constituted by two main nested objects:
*
*/
public Output autoscalingScaleDownUtilizationThreshold() {
return this.autoscalingScaleDownUtilizationThreshold;
}
/**
* Number of nodes which are actually ready in the pool
*
*/
@Export(name="availableNodes", refs={Integer.class}, tree="[0]")
private Output availableNodes;
/**
* @return Number of nodes which are actually ready in the pool
*
*/
public Output availableNodes() {
return this.availableNodes;
}
/**
* Creation date
*
*/
@Export(name="createdAt", refs={String.class}, tree="[0]")
private Output createdAt;
/**
* @return Creation date
*
*/
public Output createdAt() {
return this.createdAt;
}
/**
* Number of nodes present in the pool
*
*/
@Export(name="currentNodes", refs={Integer.class}, tree="[0]")
private Output currentNodes;
/**
* @return Number of nodes present in the pool
*
*/
public Output currentNodes() {
return this.currentNodes;
}
/**
* number of nodes to start.
*
*/
@Export(name="desiredNodes", refs={Integer.class}, tree="[0]")
private Output desiredNodes;
/**
* @return number of nodes to start.
*
*/
public Output desiredNodes() {
return this.desiredNodes;
}
/**
* Flavor name
*
*/
@Export(name="flavor", refs={String.class}, tree="[0]")
private Output flavor;
/**
* @return Flavor name
*
*/
public Output flavor() {
return this.flavor;
}
/**
* a valid OVHcloud public cloud flavor ID in which the nodes will be started. Ex: "b2-7". You can find the list of flavor IDs: https://www.ovhcloud.com/fr/public-cloud/prices/.
* **Changing this value recreates the resource.**
*
*/
@Export(name="flavorName", refs={String.class}, tree="[0]")
private Output flavorName;
/**
* @return a valid OVHcloud public cloud flavor ID in which the nodes will be started. Ex: "b2-7". You can find the list of flavor IDs: https://www.ovhcloud.com/fr/public-cloud/prices/.
* **Changing this value recreates the resource.**
*
*/
public Output flavorName() {
return this.flavorName;
}
/**
* The id of the managed kubernetes cluster. **Changing this value recreates the resource.**
*
*/
@Export(name="kubeId", refs={String.class}, tree="[0]")
private Output kubeId;
/**
* @return The id of the managed kubernetes cluster. **Changing this value recreates the resource.**
*
*/
public Output kubeId() {
return this.kubeId;
}
/**
* maximum number of nodes allowed in the pool. Setting `desired_nodes` over this value will raise an error.
*
*/
@Export(name="maxNodes", refs={Integer.class}, tree="[0]")
private Output maxNodes;
/**
* @return maximum number of nodes allowed in the pool. Setting `desired_nodes` over this value will raise an error.
*
*/
public Output maxNodes() {
return this.maxNodes;
}
/**
* minimum number of nodes allowed in the pool. Setting `desired_nodes` under this value will raise an error.
*
*/
@Export(name="minNodes", refs={Integer.class}, tree="[0]")
private Output minNodes;
/**
* @return minimum number of nodes allowed in the pool. Setting `desired_nodes` under this value will raise an error.
*
*/
public Output minNodes() {
return this.minNodes;
}
/**
* should the nodes be billed on a monthly basis. Default to `false`. **Changing this value recreates the resource.**
*
*/
@Export(name="monthlyBilled", refs={Boolean.class}, tree="[0]")
private Output monthlyBilled;
/**
* @return should the nodes be billed on a monthly basis. Default to `false`. **Changing this value recreates the resource.**
*
*/
public Output monthlyBilled() {
return this.monthlyBilled;
}
/**
* The name of the nodepool. Warning: `_` char is not allowed! **Changing this value recreates the resource.**
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the nodepool. Warning: `_` char is not allowed! **Changing this value recreates the resource.**
*
*/
public Output name() {
return this.name;
}
/**
* Project id
*
*/
@Export(name="projectId", refs={String.class}, tree="[0]")
private Output projectId;
/**
* @return Project id
*
*/
public Output projectId() {
return this.projectId;
}
/**
* The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used. **Changing this value recreates the resource.**
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used. **Changing this value recreates the resource.**
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* Status describing the state between number of nodes wanted and available ones
*
*/
@Export(name="sizeStatus", refs={String.class}, tree="[0]")
private Output sizeStatus;
/**
* @return Status describing the state between number of nodes wanted and available ones
*
*/
public Output sizeStatus() {
return this.sizeStatus;
}
/**
* Current status
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return Current status
*
*/
public Output status() {
return this.status;
}
/**
* Node pool template
*
*/
@Export(name="template", refs={KubeNodePoolTemplate.class}, tree="[0]")
private Output* @Nullable */ KubeNodePoolTemplate> template;
/**
* @return Node pool template
*
*/
public Output> template() {
return Codegen.optional(this.template);
}
/**
* Number of nodes with the latest version installed in the pool
*
*/
@Export(name="upToDateNodes", refs={Integer.class}, tree="[0]")
private Output upToDateNodes;
/**
* @return Number of nodes with the latest version installed in the pool
*
*/
public Output upToDateNodes() {
return this.upToDateNodes;
}
/**
* Last update date
*
*/
@Export(name="updatedAt", refs={String.class}, tree="[0]")
private Output updatedAt;
/**
* @return Last update date
*
*/
public Output updatedAt() {
return this.updatedAt;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public KubeNodePool(java.lang.String name) {
this(name, KubeNodePoolArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public KubeNodePool(java.lang.String name, KubeNodePoolArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public KubeNodePool(java.lang.String name, KubeNodePoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/kubeNodePool:KubeNodePool", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private KubeNodePool(java.lang.String name, Output id, @Nullable KubeNodePoolState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:CloudProject/kubeNodePool:KubeNodePool", name, state, makeResourceOptions(options, id), false);
}
private static KubeNodePoolArgs makeArgs(KubeNodePoolArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? KubeNodePoolArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static KubeNodePool get(java.lang.String name, Output id, @Nullable KubeNodePoolState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new KubeNodePool(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy