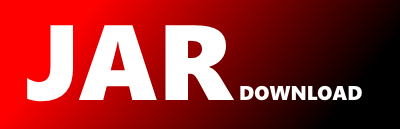
com.ovhcloud.pulumi.ovh.CloudProject.RegionStoragePresignArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RegionStoragePresignArgs extends com.pulumi.resources.ResourceArgs {
public static final RegionStoragePresignArgs Empty = new RegionStoragePresignArgs();
/**
* Define, in seconds, for how long your URL will be valid.
*
*/
@Import(name="expire", required=true)
private Output expire;
/**
* @return Define, in seconds, for how long your URL will be valid.
*
*/
public Output expire() {
return this.expire;
}
/**
* The method you want to use to interact with your object. Can be either 'GET' or 'PUT'.
*
*/
@Import(name="method", required=true)
private Output method;
/**
* @return The method you want to use to interact with your object. Can be either 'GET' or 'PUT'.
*
*/
public Output method() {
return this.method;
}
/**
* The name of your S3 storage container/bucket.
*
*/
@Import(name="name")
private @Nullable Output name;
/**
* @return The name of your S3 storage container/bucket.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy