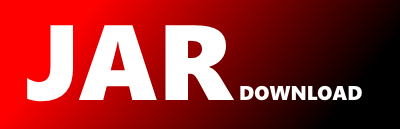
com.ovhcloud.pulumi.ovh.CloudProject.WorkflowBackupArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class WorkflowBackupArgs extends com.pulumi.resources.ResourceArgs {
public static final WorkflowBackupArgs Empty = new WorkflowBackupArgs();
/**
* The name of the backup files that are created. If empty, the `name` attribute is used.
*
*/
@Import(name="backupName")
private @Nullable Output backupName;
/**
* @return The name of the backup files that are created. If empty, the `name` attribute is used.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy