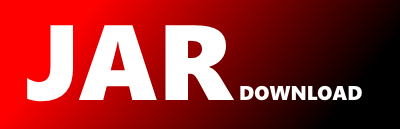
com.ovhcloud.pulumi.ovh.CloudProject.inputs.GetContainerRegistryOIDCPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject.inputs;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetContainerRegistryOIDCPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetContainerRegistryOIDCPlainArgs Empty = new GetContainerRegistryOIDCPlainArgs();
/**
* Specify an OIDC admin group name. All OIDC users in this group will have harbor admin privilege. Keep it blank if you do not want to.
*
*/
@Import(name="oidcAdminGroup")
private @Nullable String oidcAdminGroup;
/**
* @return Specify an OIDC admin group name. All OIDC users in this group will have harbor admin privilege. Keep it blank if you do not want to.
*
*/
public Optional oidcAdminGroup() {
return Optional.ofNullable(this.oidcAdminGroup);
}
/**
* Skip the onboarding screen, so user cannot change its username. Username is provided from ID Token.
*
*/
@Import(name="oidcAutoOnboard")
private @Nullable Boolean oidcAutoOnboard;
/**
* @return Skip the onboarding screen, so user cannot change its username. Username is provided from ID Token.
*
*/
public Optional oidcAutoOnboard() {
return Optional.ofNullable(this.oidcAutoOnboard);
}
/**
* The client ID with which Harbor is registered as client application with the OIDC provider.
*
*/
@Import(name="oidcClientId")
private @Nullable String oidcClientId;
/**
* @return The client ID with which Harbor is registered as client application with the OIDC provider.
*
*/
public Optional oidcClientId() {
return Optional.ofNullable(this.oidcClientId);
}
/**
* The URL of an OIDC-compliant server.
*
*/
@Import(name="oidcEndpoint")
private @Nullable String oidcEndpoint;
/**
* @return The URL of an OIDC-compliant server.
*
*/
public Optional oidcEndpoint() {
return Optional.ofNullable(this.oidcEndpoint);
}
/**
* The name of Claim in the ID token whose value is the list of group names.
*
*/
@Import(name="oidcGroupsClaim")
private @Nullable String oidcGroupsClaim;
/**
* @return The name of Claim in the ID token whose value is the list of group names.
*
*/
public Optional oidcGroupsClaim() {
return Optional.ofNullable(this.oidcGroupsClaim);
}
/**
* The name of the OIDC provider.
*
*/
@Import(name="oidcName")
private @Nullable String oidcName;
/**
* @return The name of the OIDC provider.
*
*/
public Optional oidcName() {
return Optional.ofNullable(this.oidcName);
}
/**
* The scope sent to OIDC server during authentication. It's a comma-separated string that must contain 'openid' and usually also contains 'profile' and 'email'. To obtain refresh tokens it should also contain 'offline_access'.
*
*/
@Import(name="oidcScope")
private @Nullable String oidcScope;
/**
* @return The scope sent to OIDC server during authentication. It's a comma-separated string that must contain 'openid' and usually also contains 'profile' and 'email'. To obtain refresh tokens it should also contain 'offline_access'.
*
*/
public Optional oidcScope() {
return Optional.ofNullable(this.oidcScope);
}
/**
* The name of the claim in the ID Token where the username is retrieved from. If not specified, it will default to 'name' (only useful when automatic Onboarding is enabled).
*
*/
@Import(name="oidcUserClaim")
private @Nullable String oidcUserClaim;
/**
* @return The name of the claim in the ID Token where the username is retrieved from. If not specified, it will default to 'name' (only useful when automatic Onboarding is enabled).
*
*/
public Optional oidcUserClaim() {
return Optional.ofNullable(this.oidcUserClaim);
}
/**
* Set it to `false` if your OIDC server is hosted via self-signed certificate.
*
*/
@Import(name="oidcVerifyCert")
private @Nullable Boolean oidcVerifyCert;
/**
* @return Set it to `false` if your OIDC server is hosted via self-signed certificate.
*
*/
public Optional oidcVerifyCert() {
return Optional.ofNullable(this.oidcVerifyCert);
}
/**
* The id of the Managed Private Registry.
*
*/
@Import(name="registryId", required=true)
private String registryId;
/**
* @return The id of the Managed Private Registry.
*
*/
public String registryId() {
return this.registryId;
}
/**
* The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used.
*
*/
@Import(name="serviceName", required=true)
private String serviceName;
/**
* @return The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used.
*
*/
public String serviceName() {
return this.serviceName;
}
private GetContainerRegistryOIDCPlainArgs() {}
private GetContainerRegistryOIDCPlainArgs(GetContainerRegistryOIDCPlainArgs $) {
this.oidcAdminGroup = $.oidcAdminGroup;
this.oidcAutoOnboard = $.oidcAutoOnboard;
this.oidcClientId = $.oidcClientId;
this.oidcEndpoint = $.oidcEndpoint;
this.oidcGroupsClaim = $.oidcGroupsClaim;
this.oidcName = $.oidcName;
this.oidcScope = $.oidcScope;
this.oidcUserClaim = $.oidcUserClaim;
this.oidcVerifyCert = $.oidcVerifyCert;
this.registryId = $.registryId;
this.serviceName = $.serviceName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetContainerRegistryOIDCPlainArgs defaults) {
return new Builder(defaults);
}
public static final class Builder {
private GetContainerRegistryOIDCPlainArgs $;
public Builder() {
$ = new GetContainerRegistryOIDCPlainArgs();
}
public Builder(GetContainerRegistryOIDCPlainArgs defaults) {
$ = new GetContainerRegistryOIDCPlainArgs(Objects.requireNonNull(defaults));
}
/**
* @param oidcAdminGroup Specify an OIDC admin group name. All OIDC users in this group will have harbor admin privilege. Keep it blank if you do not want to.
*
* @return builder
*
*/
public Builder oidcAdminGroup(@Nullable String oidcAdminGroup) {
$.oidcAdminGroup = oidcAdminGroup;
return this;
}
/**
* @param oidcAutoOnboard Skip the onboarding screen, so user cannot change its username. Username is provided from ID Token.
*
* @return builder
*
*/
public Builder oidcAutoOnboard(@Nullable Boolean oidcAutoOnboard) {
$.oidcAutoOnboard = oidcAutoOnboard;
return this;
}
/**
* @param oidcClientId The client ID with which Harbor is registered as client application with the OIDC provider.
*
* @return builder
*
*/
public Builder oidcClientId(@Nullable String oidcClientId) {
$.oidcClientId = oidcClientId;
return this;
}
/**
* @param oidcEndpoint The URL of an OIDC-compliant server.
*
* @return builder
*
*/
public Builder oidcEndpoint(@Nullable String oidcEndpoint) {
$.oidcEndpoint = oidcEndpoint;
return this;
}
/**
* @param oidcGroupsClaim The name of Claim in the ID token whose value is the list of group names.
*
* @return builder
*
*/
public Builder oidcGroupsClaim(@Nullable String oidcGroupsClaim) {
$.oidcGroupsClaim = oidcGroupsClaim;
return this;
}
/**
* @param oidcName The name of the OIDC provider.
*
* @return builder
*
*/
public Builder oidcName(@Nullable String oidcName) {
$.oidcName = oidcName;
return this;
}
/**
* @param oidcScope The scope sent to OIDC server during authentication. It's a comma-separated string that must contain 'openid' and usually also contains 'profile' and 'email'. To obtain refresh tokens it should also contain 'offline_access'.
*
* @return builder
*
*/
public Builder oidcScope(@Nullable String oidcScope) {
$.oidcScope = oidcScope;
return this;
}
/**
* @param oidcUserClaim The name of the claim in the ID Token where the username is retrieved from. If not specified, it will default to 'name' (only useful when automatic Onboarding is enabled).
*
* @return builder
*
*/
public Builder oidcUserClaim(@Nullable String oidcUserClaim) {
$.oidcUserClaim = oidcUserClaim;
return this;
}
/**
* @param oidcVerifyCert Set it to `false` if your OIDC server is hosted via self-signed certificate.
*
* @return builder
*
*/
public Builder oidcVerifyCert(@Nullable Boolean oidcVerifyCert) {
$.oidcVerifyCert = oidcVerifyCert;
return this;
}
/**
* @param registryId The id of the Managed Private Registry.
*
* @return builder
*
*/
public Builder registryId(String registryId) {
$.registryId = registryId;
return this;
}
/**
* @param serviceName The id of the public cloud project. If omitted, the `OVH_CLOUD_PROJECT_SERVICE` environment variable is used.
*
* @return builder
*
*/
public Builder serviceName(String serviceName) {
$.serviceName = serviceName;
return this;
}
public GetContainerRegistryOIDCPlainArgs build() {
if ($.registryId == null) {
throw new MissingRequiredPropertyException("GetContainerRegistryOIDCPlainArgs", "registryId");
}
if ($.serviceName == null) {
throw new MissingRequiredPropertyException("GetContainerRegistryOIDCPlainArgs", "serviceName");
}
return $;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy