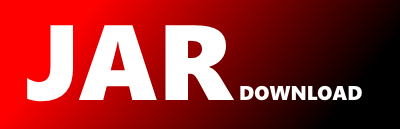
com.ovhcloud.pulumi.ovh.CloudProject.inputs.KubeCustomizationKubeProxyIpvsArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.CloudProject.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KubeCustomizationKubeProxyIpvsArgs extends com.pulumi.resources.ResourceArgs {
public static final KubeCustomizationKubeProxyIpvsArgs Empty = new KubeCustomizationKubeProxyIpvsArgs();
/**
* Minimum period that IPVS rules are refreshed in [RFC3339](https://www.rfc-editor.org/rfc/rfc3339) duration (e.g. `PT60S`).
*
*/
@Import(name="minSyncPeriod")
private @Nullable Output minSyncPeriod;
/**
* @return Minimum period that IPVS rules are refreshed in [RFC3339](https://www.rfc-editor.org/rfc/rfc3339) duration (e.g. `PT60S`).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy