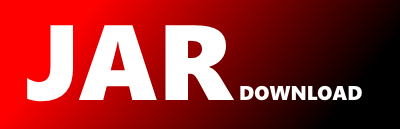
com.ovhcloud.pulumi.ovh.Dbaas.inputs.LogsOutputGraylogStreamState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Dbaas.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LogsOutputGraylogStreamState extends com.pulumi.resources.ResourceArgs {
public static final LogsOutputGraylogStreamState Empty = new LogsOutputGraylogStreamState();
/**
* Indicates if the current user can create alert on the stream
*
*/
@Import(name="canAlert")
private @Nullable Output canAlert;
/**
* @return Indicates if the current user can create alert on the stream
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy