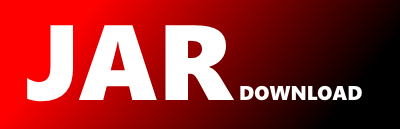
com.ovhcloud.pulumi.ovh.Dbaas.outputs.GetLogsOutputGraylogStreamResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Dbaas.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetLogsOutputGraylogStreamResult {
private Boolean canAlert;
/**
* @return Cold storage compression method
*
*/
private String coldStorageCompression;
/**
* @return ColdStorage content
*
*/
private String coldStorageContent;
/**
* @return Is Cold storage enabled?
*
*/
private Boolean coldStorageEnabled;
/**
* @return Notify on new Cold storage archive
*
*/
private Boolean coldStorageNotifyEnabled;
/**
* @return Cold storage retention in year
*
*/
private Integer coldStorageRetention;
/**
* @return ColdStorage destination
*
*/
private String coldStorageTarget;
/**
* @return Stream creation
*
*/
private String createdAt;
/**
* @return Stream description
*
*/
private String description;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Enable ES indexing
*
*/
private Boolean indexingEnabled;
/**
* @return Maximum indexing size (in GB)
*
*/
private Integer indexingMaxSize;
/**
* @return If set, notify when size is near 80, 90 or 100 % of the maximum configured setting
*
*/
private Boolean indexingNotifyEnabled;
/**
* @return Indicates if you are allowed to edit entry
*
*/
private Boolean isEditable;
/**
* @return Indicates if you are allowed to share entry
*
*/
private Boolean isShareable;
/**
* @return Number of alert condition
*
*/
private Integer nbAlertCondition;
/**
* @return Number of coldstored archives
*
*/
private Integer nbArchive;
/**
* @return Parent stream ID
*
*/
private String parentStreamId;
/**
* @return If set, pause indexing when maximum size is reach
*
*/
private Boolean pauseIndexingOnMaxSize;
/**
* @return Retention ID
*
*/
private String retentionId;
private String serviceName;
/**
* @return Stream ID
*
*/
private String streamId;
private String title;
/**
* @return Stream last update
*
*/
private String updatedAt;
/**
* @return Enable Websocket
*
*/
private Boolean webSocketEnabled;
/**
* @return Write token of the stream (empty if the caller is not the owner of the stream)
*
*/
private String writeToken;
private GetLogsOutputGraylogStreamResult() {}
public Boolean canAlert() {
return this.canAlert;
}
/**
* @return Cold storage compression method
*
*/
public String coldStorageCompression() {
return this.coldStorageCompression;
}
/**
* @return ColdStorage content
*
*/
public String coldStorageContent() {
return this.coldStorageContent;
}
/**
* @return Is Cold storage enabled?
*
*/
public Boolean coldStorageEnabled() {
return this.coldStorageEnabled;
}
/**
* @return Notify on new Cold storage archive
*
*/
public Boolean coldStorageNotifyEnabled() {
return this.coldStorageNotifyEnabled;
}
/**
* @return Cold storage retention in year
*
*/
public Integer coldStorageRetention() {
return this.coldStorageRetention;
}
/**
* @return ColdStorage destination
*
*/
public String coldStorageTarget() {
return this.coldStorageTarget;
}
/**
* @return Stream creation
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return Stream description
*
*/
public String description() {
return this.description;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Enable ES indexing
*
*/
public Boolean indexingEnabled() {
return this.indexingEnabled;
}
/**
* @return Maximum indexing size (in GB)
*
*/
public Integer indexingMaxSize() {
return this.indexingMaxSize;
}
/**
* @return If set, notify when size is near 80, 90 or 100 % of the maximum configured setting
*
*/
public Boolean indexingNotifyEnabled() {
return this.indexingNotifyEnabled;
}
/**
* @return Indicates if you are allowed to edit entry
*
*/
public Boolean isEditable() {
return this.isEditable;
}
/**
* @return Indicates if you are allowed to share entry
*
*/
public Boolean isShareable() {
return this.isShareable;
}
/**
* @return Number of alert condition
*
*/
public Integer nbAlertCondition() {
return this.nbAlertCondition;
}
/**
* @return Number of coldstored archives
*
*/
public Integer nbArchive() {
return this.nbArchive;
}
/**
* @return Parent stream ID
*
*/
public String parentStreamId() {
return this.parentStreamId;
}
/**
* @return If set, pause indexing when maximum size is reach
*
*/
public Boolean pauseIndexingOnMaxSize() {
return this.pauseIndexingOnMaxSize;
}
/**
* @return Retention ID
*
*/
public String retentionId() {
return this.retentionId;
}
public String serviceName() {
return this.serviceName;
}
/**
* @return Stream ID
*
*/
public String streamId() {
return this.streamId;
}
public String title() {
return this.title;
}
/**
* @return Stream last update
*
*/
public String updatedAt() {
return this.updatedAt;
}
/**
* @return Enable Websocket
*
*/
public Boolean webSocketEnabled() {
return this.webSocketEnabled;
}
/**
* @return Write token of the stream (empty if the caller is not the owner of the stream)
*
*/
public String writeToken() {
return this.writeToken;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetLogsOutputGraylogStreamResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean canAlert;
private String coldStorageCompression;
private String coldStorageContent;
private Boolean coldStorageEnabled;
private Boolean coldStorageNotifyEnabled;
private Integer coldStorageRetention;
private String coldStorageTarget;
private String createdAt;
private String description;
private String id;
private Boolean indexingEnabled;
private Integer indexingMaxSize;
private Boolean indexingNotifyEnabled;
private Boolean isEditable;
private Boolean isShareable;
private Integer nbAlertCondition;
private Integer nbArchive;
private String parentStreamId;
private Boolean pauseIndexingOnMaxSize;
private String retentionId;
private String serviceName;
private String streamId;
private String title;
private String updatedAt;
private Boolean webSocketEnabled;
private String writeToken;
public Builder() {}
public Builder(GetLogsOutputGraylogStreamResult defaults) {
Objects.requireNonNull(defaults);
this.canAlert = defaults.canAlert;
this.coldStorageCompression = defaults.coldStorageCompression;
this.coldStorageContent = defaults.coldStorageContent;
this.coldStorageEnabled = defaults.coldStorageEnabled;
this.coldStorageNotifyEnabled = defaults.coldStorageNotifyEnabled;
this.coldStorageRetention = defaults.coldStorageRetention;
this.coldStorageTarget = defaults.coldStorageTarget;
this.createdAt = defaults.createdAt;
this.description = defaults.description;
this.id = defaults.id;
this.indexingEnabled = defaults.indexingEnabled;
this.indexingMaxSize = defaults.indexingMaxSize;
this.indexingNotifyEnabled = defaults.indexingNotifyEnabled;
this.isEditable = defaults.isEditable;
this.isShareable = defaults.isShareable;
this.nbAlertCondition = defaults.nbAlertCondition;
this.nbArchive = defaults.nbArchive;
this.parentStreamId = defaults.parentStreamId;
this.pauseIndexingOnMaxSize = defaults.pauseIndexingOnMaxSize;
this.retentionId = defaults.retentionId;
this.serviceName = defaults.serviceName;
this.streamId = defaults.streamId;
this.title = defaults.title;
this.updatedAt = defaults.updatedAt;
this.webSocketEnabled = defaults.webSocketEnabled;
this.writeToken = defaults.writeToken;
}
@CustomType.Setter
public Builder canAlert(Boolean canAlert) {
if (canAlert == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "canAlert");
}
this.canAlert = canAlert;
return this;
}
@CustomType.Setter
public Builder coldStorageCompression(String coldStorageCompression) {
if (coldStorageCompression == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageCompression");
}
this.coldStorageCompression = coldStorageCompression;
return this;
}
@CustomType.Setter
public Builder coldStorageContent(String coldStorageContent) {
if (coldStorageContent == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageContent");
}
this.coldStorageContent = coldStorageContent;
return this;
}
@CustomType.Setter
public Builder coldStorageEnabled(Boolean coldStorageEnabled) {
if (coldStorageEnabled == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageEnabled");
}
this.coldStorageEnabled = coldStorageEnabled;
return this;
}
@CustomType.Setter
public Builder coldStorageNotifyEnabled(Boolean coldStorageNotifyEnabled) {
if (coldStorageNotifyEnabled == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageNotifyEnabled");
}
this.coldStorageNotifyEnabled = coldStorageNotifyEnabled;
return this;
}
@CustomType.Setter
public Builder coldStorageRetention(Integer coldStorageRetention) {
if (coldStorageRetention == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageRetention");
}
this.coldStorageRetention = coldStorageRetention;
return this;
}
@CustomType.Setter
public Builder coldStorageTarget(String coldStorageTarget) {
if (coldStorageTarget == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "coldStorageTarget");
}
this.coldStorageTarget = coldStorageTarget;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder indexingEnabled(Boolean indexingEnabled) {
if (indexingEnabled == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "indexingEnabled");
}
this.indexingEnabled = indexingEnabled;
return this;
}
@CustomType.Setter
public Builder indexingMaxSize(Integer indexingMaxSize) {
if (indexingMaxSize == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "indexingMaxSize");
}
this.indexingMaxSize = indexingMaxSize;
return this;
}
@CustomType.Setter
public Builder indexingNotifyEnabled(Boolean indexingNotifyEnabled) {
if (indexingNotifyEnabled == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "indexingNotifyEnabled");
}
this.indexingNotifyEnabled = indexingNotifyEnabled;
return this;
}
@CustomType.Setter
public Builder isEditable(Boolean isEditable) {
if (isEditable == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "isEditable");
}
this.isEditable = isEditable;
return this;
}
@CustomType.Setter
public Builder isShareable(Boolean isShareable) {
if (isShareable == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "isShareable");
}
this.isShareable = isShareable;
return this;
}
@CustomType.Setter
public Builder nbAlertCondition(Integer nbAlertCondition) {
if (nbAlertCondition == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "nbAlertCondition");
}
this.nbAlertCondition = nbAlertCondition;
return this;
}
@CustomType.Setter
public Builder nbArchive(Integer nbArchive) {
if (nbArchive == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "nbArchive");
}
this.nbArchive = nbArchive;
return this;
}
@CustomType.Setter
public Builder parentStreamId(String parentStreamId) {
if (parentStreamId == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "parentStreamId");
}
this.parentStreamId = parentStreamId;
return this;
}
@CustomType.Setter
public Builder pauseIndexingOnMaxSize(Boolean pauseIndexingOnMaxSize) {
if (pauseIndexingOnMaxSize == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "pauseIndexingOnMaxSize");
}
this.pauseIndexingOnMaxSize = pauseIndexingOnMaxSize;
return this;
}
@CustomType.Setter
public Builder retentionId(String retentionId) {
if (retentionId == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "retentionId");
}
this.retentionId = retentionId;
return this;
}
@CustomType.Setter
public Builder serviceName(String serviceName) {
if (serviceName == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "serviceName");
}
this.serviceName = serviceName;
return this;
}
@CustomType.Setter
public Builder streamId(String streamId) {
if (streamId == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "streamId");
}
this.streamId = streamId;
return this;
}
@CustomType.Setter
public Builder title(String title) {
if (title == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "title");
}
this.title = title;
return this;
}
@CustomType.Setter
public Builder updatedAt(String updatedAt) {
if (updatedAt == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
@CustomType.Setter
public Builder webSocketEnabled(Boolean webSocketEnabled) {
if (webSocketEnabled == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "webSocketEnabled");
}
this.webSocketEnabled = webSocketEnabled;
return this;
}
@CustomType.Setter
public Builder writeToken(String writeToken) {
if (writeToken == null) {
throw new MissingRequiredPropertyException("GetLogsOutputGraylogStreamResult", "writeToken");
}
this.writeToken = writeToken;
return this;
}
public GetLogsOutputGraylogStreamResult build() {
final var _resultValue = new GetLogsOutputGraylogStreamResult();
_resultValue.canAlert = canAlert;
_resultValue.coldStorageCompression = coldStorageCompression;
_resultValue.coldStorageContent = coldStorageContent;
_resultValue.coldStorageEnabled = coldStorageEnabled;
_resultValue.coldStorageNotifyEnabled = coldStorageNotifyEnabled;
_resultValue.coldStorageRetention = coldStorageRetention;
_resultValue.coldStorageTarget = coldStorageTarget;
_resultValue.createdAt = createdAt;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.indexingEnabled = indexingEnabled;
_resultValue.indexingMaxSize = indexingMaxSize;
_resultValue.indexingNotifyEnabled = indexingNotifyEnabled;
_resultValue.isEditable = isEditable;
_resultValue.isShareable = isShareable;
_resultValue.nbAlertCondition = nbAlertCondition;
_resultValue.nbArchive = nbArchive;
_resultValue.parentStreamId = parentStreamId;
_resultValue.pauseIndexingOnMaxSize = pauseIndexingOnMaxSize;
_resultValue.retentionId = retentionId;
_resultValue.serviceName = serviceName;
_resultValue.streamId = streamId;
_resultValue.title = title;
_resultValue.updatedAt = updatedAt;
_resultValue.webSocketEnabled = webSocketEnabled;
_resultValue.writeToken = writeToken;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy