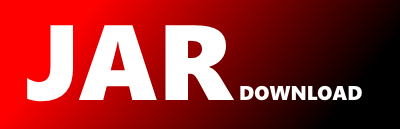
com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Dedicated.inputs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerDetailsArgs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerIamArgs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerOrderArgs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerPlanArgs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerPlanOptionArgs;
import com.ovhcloud.pulumi.ovh.Dedicated.inputs.ServerUserMetadataArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServerState extends com.pulumi.resources.ResourceArgs {
public static final ServerState Empty = new ServerState();
/**
* Dedicated AZ localisation
*
*/
@Import(name="availabilityZone")
private @Nullable Output availabilityZone;
/**
* @return Dedicated AZ localisation
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy