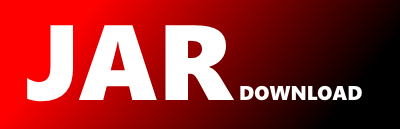
com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Dedicated.outputs;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkBandwidth;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkConnectionVal;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkOla;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkRouting;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkSwitching;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkTraffic;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkVmac;
import com.ovhcloud.pulumi.ovh.Dedicated.outputs.GetServerSpecificationsNetworkVrack;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
@CustomType
public final class GetServerSpecificationsNetworkResult {
/**
* @return vrack bandwidth limitation
*
*/
private GetServerSpecificationsNetworkBandwidth bandwidth;
/**
* @return Network connection flow rate
*
*/
private GetServerSpecificationsNetworkConnectionVal connectionVal;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return OLA details
*
*/
private GetServerSpecificationsNetworkOla ola;
/**
* @return Routing details
*
*/
private GetServerSpecificationsNetworkRouting routing;
private String serviceName;
/**
* @return Switching details
*
*/
private GetServerSpecificationsNetworkSwitching switching;
/**
* @return Traffic details
*
*/
private GetServerSpecificationsNetworkTraffic traffic;
/**
* @return VMAC information for this dedicated server
*
*/
private GetServerSpecificationsNetworkVmac vmac;
/**
* @return vRack details
*
*/
private GetServerSpecificationsNetworkVrack vrack;
private GetServerSpecificationsNetworkResult() {}
/**
* @return vrack bandwidth limitation
*
*/
public GetServerSpecificationsNetworkBandwidth bandwidth() {
return this.bandwidth;
}
/**
* @return Network connection flow rate
*
*/
public GetServerSpecificationsNetworkConnectionVal connectionVal() {
return this.connectionVal;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return OLA details
*
*/
public GetServerSpecificationsNetworkOla ola() {
return this.ola;
}
/**
* @return Routing details
*
*/
public GetServerSpecificationsNetworkRouting routing() {
return this.routing;
}
public String serviceName() {
return this.serviceName;
}
/**
* @return Switching details
*
*/
public GetServerSpecificationsNetworkSwitching switching() {
return this.switching;
}
/**
* @return Traffic details
*
*/
public GetServerSpecificationsNetworkTraffic traffic() {
return this.traffic;
}
/**
* @return VMAC information for this dedicated server
*
*/
public GetServerSpecificationsNetworkVmac vmac() {
return this.vmac;
}
/**
* @return vRack details
*
*/
public GetServerSpecificationsNetworkVrack vrack() {
return this.vrack;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerSpecificationsNetworkResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private GetServerSpecificationsNetworkBandwidth bandwidth;
private GetServerSpecificationsNetworkConnectionVal connectionVal;
private String id;
private GetServerSpecificationsNetworkOla ola;
private GetServerSpecificationsNetworkRouting routing;
private String serviceName;
private GetServerSpecificationsNetworkSwitching switching;
private GetServerSpecificationsNetworkTraffic traffic;
private GetServerSpecificationsNetworkVmac vmac;
private GetServerSpecificationsNetworkVrack vrack;
public Builder() {}
public Builder(GetServerSpecificationsNetworkResult defaults) {
Objects.requireNonNull(defaults);
this.bandwidth = defaults.bandwidth;
this.connectionVal = defaults.connectionVal;
this.id = defaults.id;
this.ola = defaults.ola;
this.routing = defaults.routing;
this.serviceName = defaults.serviceName;
this.switching = defaults.switching;
this.traffic = defaults.traffic;
this.vmac = defaults.vmac;
this.vrack = defaults.vrack;
}
@CustomType.Setter
public Builder bandwidth(GetServerSpecificationsNetworkBandwidth bandwidth) {
if (bandwidth == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "bandwidth");
}
this.bandwidth = bandwidth;
return this;
}
@CustomType.Setter
public Builder connectionVal(GetServerSpecificationsNetworkConnectionVal connectionVal) {
if (connectionVal == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "connectionVal");
}
this.connectionVal = connectionVal;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ola(GetServerSpecificationsNetworkOla ola) {
if (ola == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "ola");
}
this.ola = ola;
return this;
}
@CustomType.Setter
public Builder routing(GetServerSpecificationsNetworkRouting routing) {
if (routing == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "routing");
}
this.routing = routing;
return this;
}
@CustomType.Setter
public Builder serviceName(String serviceName) {
if (serviceName == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "serviceName");
}
this.serviceName = serviceName;
return this;
}
@CustomType.Setter
public Builder switching(GetServerSpecificationsNetworkSwitching switching) {
if (switching == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "switching");
}
this.switching = switching;
return this;
}
@CustomType.Setter
public Builder traffic(GetServerSpecificationsNetworkTraffic traffic) {
if (traffic == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "traffic");
}
this.traffic = traffic;
return this;
}
@CustomType.Setter
public Builder vmac(GetServerSpecificationsNetworkVmac vmac) {
if (vmac == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "vmac");
}
this.vmac = vmac;
return this;
}
@CustomType.Setter
public Builder vrack(GetServerSpecificationsNetworkVrack vrack) {
if (vrack == null) {
throw new MissingRequiredPropertyException("GetServerSpecificationsNetworkResult", "vrack");
}
this.vrack = vrack;
return this;
}
public GetServerSpecificationsNetworkResult build() {
final var _resultValue = new GetServerSpecificationsNetworkResult();
_resultValue.bandwidth = bandwidth;
_resultValue.connectionVal = connectionVal;
_resultValue.id = id;
_resultValue.ola = ola;
_resultValue.routing = routing;
_resultValue.serviceName = serviceName;
_resultValue.switching = switching;
_resultValue.traffic = traffic;
_resultValue.vmac = vmac;
_resultValue.vrack = vrack;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy