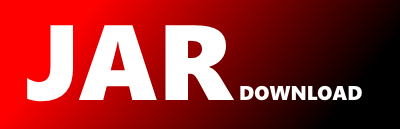
com.ovhcloud.pulumi.ovh.Hosting.PrivateDatabaseArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Hosting;
import com.ovhcloud.pulumi.ovh.Hosting.inputs.PrivateDatabaseOrderArgs;
import com.ovhcloud.pulumi.ovh.Hosting.inputs.PrivateDatabasePlanArgs;
import com.ovhcloud.pulumi.ovh.Hosting.inputs.PrivateDatabasePlanOptionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PrivateDatabaseArgs extends com.pulumi.resources.ResourceArgs {
public static final PrivateDatabaseArgs Empty = new PrivateDatabaseArgs();
/**
* Name displayed in customer panel for your private database
*
*/
@Import(name="displayName")
private @Nullable Output displayName;
/**
* @return Name displayed in customer panel for your private database
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy