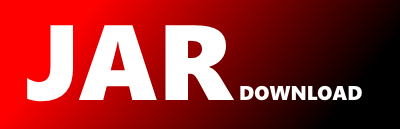
com.ovhcloud.pulumi.ovh.Iam.inputs.PermissionsGroupState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Iam.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PermissionsGroupState extends com.pulumi.resources.ResourceArgs {
public static final PermissionsGroupState Empty = new PermissionsGroupState();
/**
* Set of actions allowed by the permissions group.
*
*/
@Import(name="allows")
private @Nullable Output> allows;
/**
* @return Set of actions allowed by the permissions group.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy