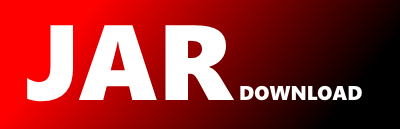
com.ovhcloud.pulumi.ovh.Iam.outputs.GetPermissionsGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Iam.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetPermissionsGroupResult {
private @Nullable List allows;
private String createdAt;
private @Nullable List denies;
private @Nullable String description;
private @Nullable List excepts;
private String id;
private String name;
private String owner;
private String updatedAt;
private String urn;
private GetPermissionsGroupResult() {}
public List allows() {
return this.allows == null ? List.of() : this.allows;
}
public String createdAt() {
return this.createdAt;
}
public List denies() {
return this.denies == null ? List.of() : this.denies;
}
public Optional description() {
return Optional.ofNullable(this.description);
}
public List excepts() {
return this.excepts == null ? List.of() : this.excepts;
}
public String id() {
return this.id;
}
public String name() {
return this.name;
}
public String owner() {
return this.owner;
}
public String updatedAt() {
return this.updatedAt;
}
public String urn() {
return this.urn;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetPermissionsGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List allows;
private String createdAt;
private @Nullable List denies;
private @Nullable String description;
private @Nullable List excepts;
private String id;
private String name;
private String owner;
private String updatedAt;
private String urn;
public Builder() {}
public Builder(GetPermissionsGroupResult defaults) {
Objects.requireNonNull(defaults);
this.allows = defaults.allows;
this.createdAt = defaults.createdAt;
this.denies = defaults.denies;
this.description = defaults.description;
this.excepts = defaults.excepts;
this.id = defaults.id;
this.name = defaults.name;
this.owner = defaults.owner;
this.updatedAt = defaults.updatedAt;
this.urn = defaults.urn;
}
@CustomType.Setter
public Builder allows(@Nullable List allows) {
this.allows = allows;
return this;
}
public Builder allows(String... allows) {
return allows(List.of(allows));
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder denies(@Nullable List denies) {
this.denies = denies;
return this;
}
public Builder denies(String... denies) {
return denies(List.of(denies));
}
@CustomType.Setter
public Builder description(@Nullable String description) {
this.description = description;
return this;
}
@CustomType.Setter
public Builder excepts(@Nullable List excepts) {
this.excepts = excepts;
return this;
}
public Builder excepts(String... excepts) {
return excepts(List.of(excepts));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder owner(String owner) {
if (owner == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "owner");
}
this.owner = owner;
return this;
}
@CustomType.Setter
public Builder updatedAt(String updatedAt) {
if (updatedAt == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "updatedAt");
}
this.updatedAt = updatedAt;
return this;
}
@CustomType.Setter
public Builder urn(String urn) {
if (urn == null) {
throw new MissingRequiredPropertyException("GetPermissionsGroupResult", "urn");
}
this.urn = urn;
return this;
}
public GetPermissionsGroupResult build() {
final var _resultValue = new GetPermissionsGroupResult();
_resultValue.allows = allows;
_resultValue.createdAt = createdAt;
_resultValue.denies = denies;
_resultValue.description = description;
_resultValue.excepts = excepts;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.owner = owner;
_resultValue.updatedAt = updatedAt;
_resultValue.urn = urn;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy