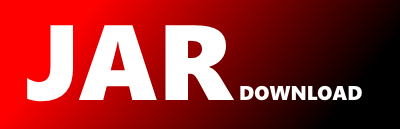
com.ovhcloud.pulumi.ovh.Ip.IpService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Ip;
import com.ovhcloud.pulumi.ovh.Ip.IpServiceArgs;
import com.ovhcloud.pulumi.ovh.Ip.inputs.IpServiceState;
import com.ovhcloud.pulumi.ovh.Ip.outputs.IpServiceOrder;
import com.ovhcloud.pulumi.ovh.Ip.outputs.IpServicePlan;
import com.ovhcloud.pulumi.ovh.Ip.outputs.IpServicePlanOption;
import com.ovhcloud.pulumi.ovh.Ip.outputs.IpServiceRoutedTo;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.Me.MeFunctions;
* import com.pulumi.ovh.Order.OrderFunctions;
* import com.pulumi.ovh.Order.inputs.GetCartArgs;
* import com.pulumi.ovh.Order.inputs.GetCartProductPlanArgs;
* import com.pulumi.ovh.Ip.IpService;
* import com.pulumi.ovh.Ip.IpServiceArgs;
* import com.pulumi.ovh.Ip.inputs.IpServicePlanArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var myaccount = MeFunctions.getMe();
*
* final var mycart = OrderFunctions.getCart(GetCartArgs.builder()
* .ovhSubsidiary("fr")
* .build());
*
* final var ipblockCartProductPlan = OrderFunctions.getCartProductPlan(GetCartProductPlanArgs.builder()
* .cartId(mycart.applyValue(getCartResult -> getCartResult.id()))
* .priceCapacity("renew")
* .product("ip")
* .planCode("ip-v4-s30-ripe")
* .build());
*
* var ipblockIpService = new IpService("ipblockIpService", IpServiceArgs.builder()
* .ovhSubsidiary(mycart.applyValue(getCartResult -> getCartResult.ovhSubsidiary()))
* .description("my ip block")
* .plan(IpServicePlanArgs.builder()
* .duration(ipblockCartProductPlan.applyValue(getCartProductPlanResult -> getCartProductPlanResult.selectedPrices()[0].duration()))
* .planCode(ipblockCartProductPlan.applyValue(getCartProductPlanResult -> getCartProductPlanResult.planCode()))
* .pricingMode(ipblockCartProductPlan.applyValue(getCartProductPlanResult -> getCartProductPlanResult.selectedPrices()[0].pricingMode()))
* .configurations(IpServicePlanConfigurationArgs.builder()
* .label("country")
* .value("FR")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="ovh:Ip/ipService:IpService")
public class IpService extends com.pulumi.resources.CustomResource {
/**
* can be terminated
*
*/
@Export(name="canBeTerminated", refs={Boolean.class}, tree="[0]")
private Output canBeTerminated;
/**
* @return can be terminated
*
*/
public Output canBeTerminated() {
return this.canBeTerminated;
}
/**
* country
*
*/
@Export(name="country", refs={String.class}, tree="[0]")
private Output country;
/**
* @return country
*
*/
public Output country() {
return this.country;
}
/**
* Custom description on your ip.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return Custom description on your ip.
*
*/
public Output description() {
return this.description;
}
/**
* ip block
*
*/
@Export(name="ip", refs={String.class}, tree="[0]")
private Output ip;
/**
* @return ip block
*
*/
public Output ip() {
return this.ip;
}
/**
* Details about an Order
*
*/
@Export(name="orders", refs={List.class,IpServiceOrder.class}, tree="[0,1]")
private Output> orders;
/**
* @return Details about an Order
*
*/
public Output> orders() {
return this.orders;
}
/**
* IP block organisation Id
*
*/
@Export(name="organisationId", refs={String.class}, tree="[0]")
private Output organisationId;
/**
* @return IP block organisation Id
*
*/
public Output organisationId() {
return this.organisationId;
}
/**
* OVHcloud Subsidiary. Country of OVHcloud legal entity you'll be billed by. List of supported subsidiaries available on API at [/1.0/me.json under `models.nichandle.OvhSubsidiaryEnum`](https://eu.api.ovh.com/1.0/me.json)
*
*/
@Export(name="ovhSubsidiary", refs={String.class}, tree="[0]")
private Output ovhSubsidiary;
/**
* @return OVHcloud Subsidiary. Country of OVHcloud legal entity you'll be billed by. List of supported subsidiaries available on API at [/1.0/me.json under `models.nichandle.OvhSubsidiaryEnum`](https://eu.api.ovh.com/1.0/me.json)
*
*/
public Output ovhSubsidiary() {
return this.ovhSubsidiary;
}
/**
* Ovh payment mode
*
* @deprecated
* This field is not anymore used since the API has been deprecated in favor of /payment/mean. Now, the default payment mean is used.
*
*/
@Deprecated /* This field is not anymore used since the API has been deprecated in favor of /payment/mean. Now, the default payment mean is used. */
@Export(name="paymentMean", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> paymentMean;
/**
* @return Ovh payment mode
*
*/
public Output> paymentMean() {
return Codegen.optional(this.paymentMean);
}
/**
* Product Plan to order
*
*/
@Export(name="plan", refs={IpServicePlan.class}, tree="[0]")
private Output plan;
/**
* @return Product Plan to order
*
*/
public Output plan() {
return this.plan;
}
/**
* Product Plan to order
*
*/
@Export(name="planOptions", refs={List.class,IpServicePlanOption.class}, tree="[0,1]")
private Output* @Nullable */ List> planOptions;
/**
* @return Product Plan to order
*
*/
public Output>> planOptions() {
return Codegen.optional(this.planOptions);
}
/**
* Routage information
*
*/
@Export(name="routedTos", refs={List.class,IpServiceRoutedTo.class}, tree="[0,1]")
private Output> routedTos;
/**
* @return Routage information
*
*/
public Output> routedTos() {
return this.routedTos;
}
/**
* service name
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return service name
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* Possible values for ip type
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return Possible values for ip type
*
*/
public Output type() {
return this.type;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public IpService(java.lang.String name) {
this(name, IpServiceArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public IpService(java.lang.String name, IpServiceArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public IpService(java.lang.String name, IpServiceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:Ip/ipService:IpService", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private IpService(java.lang.String name, Output id, @Nullable IpServiceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:Ip/ipService:IpService", name, state, makeResourceOptions(options, id), false);
}
private static IpServiceArgs makeArgs(IpServiceArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? IpServiceArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static IpService get(java.lang.String name, Output id, @Nullable IpServiceState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new IpService(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy