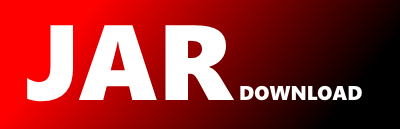
com.ovhcloud.pulumi.ovh.IpLoadBalancing.TcpFrontend Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.IpLoadBalancing;
import com.ovhcloud.pulumi.ovh.IpLoadBalancing.TcpFrontendArgs;
import com.ovhcloud.pulumi.ovh.IpLoadBalancing.inputs.TcpFrontendState;
import com.ovhcloud.pulumi.ovh.Utilities;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Creates a backend server group (frontend) to be used by loadbalancing frontend(s)
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.ovh.IpLoadBalancing.IpLoadBalancingFunctions;
* import com.pulumi.ovh.IpLoadBalancing.inputs.GetIpLoadBalancingArgs;
* import com.pulumi.ovh.IpLoadBalancing.TcpFarm;
* import com.pulumi.ovh.IpLoadBalancing.TcpFarmArgs;
* import com.pulumi.ovh.IpLoadBalancing.TcpFrontend;
* import com.pulumi.ovh.IpLoadBalancing.TcpFrontendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var lb = IpLoadBalancingFunctions.getIpLoadBalancing(GetIpLoadBalancingArgs.builder()
* .serviceName("ip-1.2.3.4")
* .state("ok")
* .build());
*
* var farm80 = new TcpFarm("farm80", TcpFarmArgs.builder()
* .displayName("ingress-8080-gra")
* .port(80)
* .serviceName(lb.applyValue(getIpLoadBalancingResult -> getIpLoadBalancingResult.serviceName()))
* .zone("all")
* .build());
*
* var testfrontend = new TcpFrontend("testfrontend", TcpFrontendArgs.builder()
* .defaultFarmId(farm80.id())
* .displayName("ingress-8080-gra")
* .port("80,443")
* .serviceName(lb.applyValue(getIpLoadBalancingResult -> getIpLoadBalancingResult.serviceName()))
* .zone("all")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* TCP frontend can be imported using the following format `service_name` and the `id` of the frontend separated by "/" e.g.
*
*/
@ResourceType(type="ovh:IpLoadBalancing/tcpFrontend:TcpFrontend")
public class TcpFrontend extends com.pulumi.resources.CustomResource {
/**
* Restrict IP Load Balancing access to these ip block. No restriction if null. List of IP blocks.
*
*/
@Export(name="allowedSources", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> allowedSources;
/**
* @return Restrict IP Load Balancing access to these ip block. No restriction if null. List of IP blocks.
*
*/
public Output>> allowedSources() {
return Codegen.optional(this.allowedSources);
}
/**
* Only attach frontend on these ip. No restriction if null. List of Ip blocks.
*
*/
@Export(name="dedicatedIpfos", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> dedicatedIpfos;
/**
* @return Only attach frontend on these ip. No restriction if null. List of Ip blocks.
*
*/
public Output>> dedicatedIpfos() {
return Codegen.optional(this.dedicatedIpfos);
}
/**
* Default TCP Farm of your frontend
*
*/
@Export(name="defaultFarmId", refs={Integer.class}, tree="[0]")
private Output defaultFarmId;
/**
* @return Default TCP Farm of your frontend
*
*/
public Output defaultFarmId() {
return this.defaultFarmId;
}
/**
* Default ssl served to your customer
*
*/
@Export(name="defaultSslId", refs={Integer.class}, tree="[0]")
private Output defaultSslId;
/**
* @return Default ssl served to your customer
*
*/
public Output defaultSslId() {
return this.defaultSslId;
}
/**
* Deny IP Load Balancing access to these ip block. No restriction if null. You cannot specify both `allowed_source` and `denied_source` at the same time. List of IP blocks.
*
*/
@Export(name="deniedSources", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> deniedSources;
/**
* @return Deny IP Load Balancing access to these ip block. No restriction if null. You cannot specify both `allowed_source` and `denied_source` at the same time. List of IP blocks.
*
*/
public Output>> deniedSources() {
return Codegen.optional(this.deniedSources);
}
/**
* Disable your frontend. Default: 'false'
*
*/
@Export(name="disabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disabled;
/**
* @return Disable your frontend. Default: 'false'
*
*/
public Output> disabled() {
return Codegen.optional(this.disabled);
}
/**
* Human readable name for your frontend, this field is for you
*
*/
@Export(name="displayName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> displayName;
/**
* @return Human readable name for your frontend, this field is for you
*
*/
public Output> displayName() {
return Codegen.optional(this.displayName);
}
/**
* Port(s) attached to your frontend. Supports single port (numerical value),
* range (2 dash-delimited increasing ports) and comma-separated list of 'single port'
* and/or 'range'. Each port must be in the [1;49151] range
*
*/
@Export(name="port", refs={String.class}, tree="[0]")
private Output port;
/**
* @return Port(s) attached to your frontend. Supports single port (numerical value),
* range (2 dash-delimited increasing ports) and comma-separated list of 'single port'
* and/or 'range'. Each port must be in the [1;49151] range
*
*/
public Output port() {
return this.port;
}
/**
* The internal name of your IP load balancing
*
*/
@Export(name="serviceName", refs={String.class}, tree="[0]")
private Output serviceName;
/**
* @return The internal name of your IP load balancing
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* SSL deciphering. Default: 'false'
*
*/
@Export(name="ssl", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> ssl;
/**
* @return SSL deciphering. Default: 'false'
*
*/
public Output> ssl() {
return Codegen.optional(this.ssl);
}
/**
* Zone where the frontend will be defined (ie. `gra`, `bhs` also supports `all`)
*
*/
@Export(name="zone", refs={String.class}, tree="[0]")
private Output zone;
/**
* @return Zone where the frontend will be defined (ie. `gra`, `bhs` also supports `all`)
*
*/
public Output zone() {
return this.zone;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public TcpFrontend(java.lang.String name) {
this(name, TcpFrontendArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public TcpFrontend(java.lang.String name, TcpFrontendArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public TcpFrontend(java.lang.String name, TcpFrontendArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:IpLoadBalancing/tcpFrontend:TcpFrontend", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private TcpFrontend(java.lang.String name, Output id, @Nullable TcpFrontendState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("ovh:IpLoadBalancing/tcpFrontend:TcpFrontend", name, state, makeResourceOptions(options, id), false);
}
private static TcpFrontendArgs makeArgs(TcpFrontendArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? TcpFrontendArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static TcpFrontend get(java.lang.String name, Output id, @Nullable TcpFrontendState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new TcpFrontend(name, id, state, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy