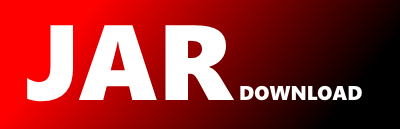
com.ovhcloud.pulumi.ovh.IpLoadBalancing.TcpRouteRuleArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.IpLoadBalancing;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TcpRouteRuleArgs extends com.pulumi.resources.ResourceArgs {
public static final TcpRouteRuleArgs Empty = new TcpRouteRuleArgs();
/**
* Human readable name for your rule, this field is for you
*
*/
@Import(name="displayName")
private @Nullable Output displayName;
/**
* @return Human readable name for your rule, this field is for you
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy