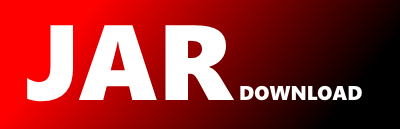
com.ovhcloud.pulumi.ovh.IpLoadBalancing.inputs.TcpFarmServerState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.IpLoadBalancing.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TcpFarmServerState extends com.pulumi.resources.ResourceArgs {
public static final TcpFarmServerState Empty = new TcpFarmServerState();
/**
* Address of the backend server (IP from either internal or OVHcloud network)
*
*/
@Import(name="address")
private @Nullable Output address;
/**
* @return Address of the backend server (IP from either internal or OVHcloud network)
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy