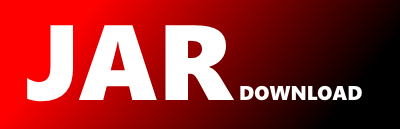
com.ovhcloud.pulumi.ovh.Me.inputs.InstallationTemplatePartitionSchemePartitionState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Me.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class InstallationTemplatePartitionSchemePartitionState extends com.pulumi.resources.ResourceArgs {
public static final InstallationTemplatePartitionSchemePartitionState Empty = new InstallationTemplatePartitionSchemePartitionState();
/**
* Partition filesystem. Enum with possibles values:
* - btrfs
* - ext3
* - ext4
* - ntfs
* - reiserfs
* - swap
* - ufs
* - xfs
* - zfs
*
*/
@Import(name="filesystem")
private @Nullable Output filesystem;
/**
* @return Partition filesystem. Enum with possibles values:
* - btrfs
* - ext3
* - ext4
* - ntfs
* - reiserfs
* - swap
* - ufs
* - xfs
* - zfs
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy