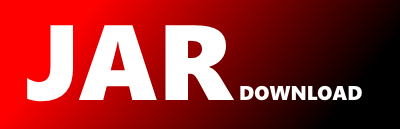
com.ovhcloud.pulumi.ovh.Me.outputs.GetInstallationTemplateResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Me.outputs;
import com.ovhcloud.pulumi.ovh.Me.outputs.GetInstallationTemplateCustomization;
import com.ovhcloud.pulumi.ovh.Me.outputs.GetInstallationTemplateInput;
import com.ovhcloud.pulumi.ovh.Me.outputs.GetInstallationTemplatePartitionScheme;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetInstallationTemplateResult {
/**
* @return Template bit format (32 or 64).
*
*/
private Integer bitFormat;
/**
* @return Category of this template (informative only).
*
*/
private String category;
private List customizations;
/**
* @return Information about this template.
*
*/
private String description;
/**
* @return Distribution this template is based on.
*
*/
private String distribution;
/**
* @return End of install date of the template.
*
*/
private String endOfInstall;
/**
* @return Template family type (bsd,linux,solaris,windows).
*
*/
private String family;
/**
* @return Filesystems available.
*
*/
private List filesystems;
/**
* @return Distribution supports hardware raid configuration through the OVHcloud API.
*
* @deprecated
* This will be deprecated in the next release
*
*/
@Deprecated /* This will be deprecated in the next release */
private Boolean hardRaidConfiguration;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Represents the questions of the expected answers in the userMetadata field.
*
*/
private List inputs;
/**
* @return Whether this template supports LVM.
*
*/
private Boolean lvmReady;
/**
* @return Partitioning customization is not available for this OS template.
*
*/
private Boolean noPartitioning;
private List partitionSchemes;
/**
* @return Template supports RAID0 and RAID1 on 2 disks.
*
*/
private Boolean softRaidOnlyMirroring;
/**
* @return Subfamily of the template.
*
*/
private String subfamily;
private String templateName;
private GetInstallationTemplateResult() {}
/**
* @return Template bit format (32 or 64).
*
*/
public Integer bitFormat() {
return this.bitFormat;
}
/**
* @return Category of this template (informative only).
*
*/
public String category() {
return this.category;
}
public List customizations() {
return this.customizations;
}
/**
* @return Information about this template.
*
*/
public String description() {
return this.description;
}
/**
* @return Distribution this template is based on.
*
*/
public String distribution() {
return this.distribution;
}
/**
* @return End of install date of the template.
*
*/
public String endOfInstall() {
return this.endOfInstall;
}
/**
* @return Template family type (bsd,linux,solaris,windows).
*
*/
public String family() {
return this.family;
}
/**
* @return Filesystems available.
*
*/
public List filesystems() {
return this.filesystems;
}
/**
* @return Distribution supports hardware raid configuration through the OVHcloud API.
*
* @deprecated
* This will be deprecated in the next release
*
*/
@Deprecated /* This will be deprecated in the next release */
public Boolean hardRaidConfiguration() {
return this.hardRaidConfiguration;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Represents the questions of the expected answers in the userMetadata field.
*
*/
public List inputs() {
return this.inputs;
}
/**
* @return Whether this template supports LVM.
*
*/
public Boolean lvmReady() {
return this.lvmReady;
}
/**
* @return Partitioning customization is not available for this OS template.
*
*/
public Boolean noPartitioning() {
return this.noPartitioning;
}
public List partitionSchemes() {
return this.partitionSchemes;
}
/**
* @return Template supports RAID0 and RAID1 on 2 disks.
*
*/
public Boolean softRaidOnlyMirroring() {
return this.softRaidOnlyMirroring;
}
/**
* @return Subfamily of the template.
*
*/
public String subfamily() {
return this.subfamily;
}
public String templateName() {
return this.templateName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetInstallationTemplateResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer bitFormat;
private String category;
private List customizations;
private String description;
private String distribution;
private String endOfInstall;
private String family;
private List filesystems;
private Boolean hardRaidConfiguration;
private String id;
private List inputs;
private Boolean lvmReady;
private Boolean noPartitioning;
private List partitionSchemes;
private Boolean softRaidOnlyMirroring;
private String subfamily;
private String templateName;
public Builder() {}
public Builder(GetInstallationTemplateResult defaults) {
Objects.requireNonNull(defaults);
this.bitFormat = defaults.bitFormat;
this.category = defaults.category;
this.customizations = defaults.customizations;
this.description = defaults.description;
this.distribution = defaults.distribution;
this.endOfInstall = defaults.endOfInstall;
this.family = defaults.family;
this.filesystems = defaults.filesystems;
this.hardRaidConfiguration = defaults.hardRaidConfiguration;
this.id = defaults.id;
this.inputs = defaults.inputs;
this.lvmReady = defaults.lvmReady;
this.noPartitioning = defaults.noPartitioning;
this.partitionSchemes = defaults.partitionSchemes;
this.softRaidOnlyMirroring = defaults.softRaidOnlyMirroring;
this.subfamily = defaults.subfamily;
this.templateName = defaults.templateName;
}
@CustomType.Setter
public Builder bitFormat(Integer bitFormat) {
if (bitFormat == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "bitFormat");
}
this.bitFormat = bitFormat;
return this;
}
@CustomType.Setter
public Builder category(String category) {
if (category == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "category");
}
this.category = category;
return this;
}
@CustomType.Setter
public Builder customizations(List customizations) {
if (customizations == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "customizations");
}
this.customizations = customizations;
return this;
}
public Builder customizations(GetInstallationTemplateCustomization... customizations) {
return customizations(List.of(customizations));
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder distribution(String distribution) {
if (distribution == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "distribution");
}
this.distribution = distribution;
return this;
}
@CustomType.Setter
public Builder endOfInstall(String endOfInstall) {
if (endOfInstall == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "endOfInstall");
}
this.endOfInstall = endOfInstall;
return this;
}
@CustomType.Setter
public Builder family(String family) {
if (family == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "family");
}
this.family = family;
return this;
}
@CustomType.Setter
public Builder filesystems(List filesystems) {
if (filesystems == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "filesystems");
}
this.filesystems = filesystems;
return this;
}
public Builder filesystems(String... filesystems) {
return filesystems(List.of(filesystems));
}
@CustomType.Setter
public Builder hardRaidConfiguration(Boolean hardRaidConfiguration) {
if (hardRaidConfiguration == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "hardRaidConfiguration");
}
this.hardRaidConfiguration = hardRaidConfiguration;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder inputs(List inputs) {
if (inputs == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "inputs");
}
this.inputs = inputs;
return this;
}
public Builder inputs(GetInstallationTemplateInput... inputs) {
return inputs(List.of(inputs));
}
@CustomType.Setter
public Builder lvmReady(Boolean lvmReady) {
if (lvmReady == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "lvmReady");
}
this.lvmReady = lvmReady;
return this;
}
@CustomType.Setter
public Builder noPartitioning(Boolean noPartitioning) {
if (noPartitioning == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "noPartitioning");
}
this.noPartitioning = noPartitioning;
return this;
}
@CustomType.Setter
public Builder partitionSchemes(List partitionSchemes) {
if (partitionSchemes == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "partitionSchemes");
}
this.partitionSchemes = partitionSchemes;
return this;
}
public Builder partitionSchemes(GetInstallationTemplatePartitionScheme... partitionSchemes) {
return partitionSchemes(List.of(partitionSchemes));
}
@CustomType.Setter
public Builder softRaidOnlyMirroring(Boolean softRaidOnlyMirroring) {
if (softRaidOnlyMirroring == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "softRaidOnlyMirroring");
}
this.softRaidOnlyMirroring = softRaidOnlyMirroring;
return this;
}
@CustomType.Setter
public Builder subfamily(String subfamily) {
if (subfamily == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "subfamily");
}
this.subfamily = subfamily;
return this;
}
@CustomType.Setter
public Builder templateName(String templateName) {
if (templateName == null) {
throw new MissingRequiredPropertyException("GetInstallationTemplateResult", "templateName");
}
this.templateName = templateName;
return this;
}
public GetInstallationTemplateResult build() {
final var _resultValue = new GetInstallationTemplateResult();
_resultValue.bitFormat = bitFormat;
_resultValue.category = category;
_resultValue.customizations = customizations;
_resultValue.description = description;
_resultValue.distribution = distribution;
_resultValue.endOfInstall = endOfInstall;
_resultValue.family = family;
_resultValue.filesystems = filesystems;
_resultValue.hardRaidConfiguration = hardRaidConfiguration;
_resultValue.id = id;
_resultValue.inputs = inputs;
_resultValue.lvmReady = lvmReady;
_resultValue.noPartitioning = noPartitioning;
_resultValue.partitionSchemes = partitionSchemes;
_resultValue.softRaidOnlyMirroring = softRaidOnlyMirroring;
_resultValue.subfamily = subfamily;
_resultValue.templateName = templateName;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy