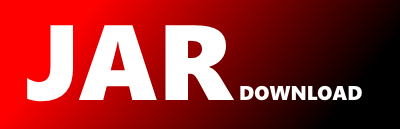
com.ovhcloud.pulumi.ovh.Order.outputs.GetCartProductPlanResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Order.outputs;
import com.ovhcloud.pulumi.ovh.Order.outputs.GetCartProductPlanPrice;
import com.ovhcloud.pulumi.ovh.Order.outputs.GetCartProductPlanSelectedPrice;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetCartProductPlanResult {
private String cartId;
private @Nullable String catalogName;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Product offer identifier
*
*/
private String planCode;
private String priceCapacity;
/**
* @return Prices of the product offer
*
*/
private List prices;
private String product;
/**
* @return Name of the product
*
*/
private String productName;
/**
* @return Product type
*
*/
private String productType;
/**
* @return Selected Price according to capacity
*
*/
private List selectedPrices;
private GetCartProductPlanResult() {}
public String cartId() {
return this.cartId;
}
public Optional catalogName() {
return Optional.ofNullable(this.catalogName);
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Product offer identifier
*
*/
public String planCode() {
return this.planCode;
}
public String priceCapacity() {
return this.priceCapacity;
}
/**
* @return Prices of the product offer
*
*/
public List prices() {
return this.prices;
}
public String product() {
return this.product;
}
/**
* @return Name of the product
*
*/
public String productName() {
return this.productName;
}
/**
* @return Product type
*
*/
public String productType() {
return this.productType;
}
/**
* @return Selected Price according to capacity
*
*/
public List selectedPrices() {
return this.selectedPrices;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCartProductPlanResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String cartId;
private @Nullable String catalogName;
private String id;
private String planCode;
private String priceCapacity;
private List prices;
private String product;
private String productName;
private String productType;
private List selectedPrices;
public Builder() {}
public Builder(GetCartProductPlanResult defaults) {
Objects.requireNonNull(defaults);
this.cartId = defaults.cartId;
this.catalogName = defaults.catalogName;
this.id = defaults.id;
this.planCode = defaults.planCode;
this.priceCapacity = defaults.priceCapacity;
this.prices = defaults.prices;
this.product = defaults.product;
this.productName = defaults.productName;
this.productType = defaults.productType;
this.selectedPrices = defaults.selectedPrices;
}
@CustomType.Setter
public Builder cartId(String cartId) {
if (cartId == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "cartId");
}
this.cartId = cartId;
return this;
}
@CustomType.Setter
public Builder catalogName(@Nullable String catalogName) {
this.catalogName = catalogName;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder planCode(String planCode) {
if (planCode == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "planCode");
}
this.planCode = planCode;
return this;
}
@CustomType.Setter
public Builder priceCapacity(String priceCapacity) {
if (priceCapacity == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "priceCapacity");
}
this.priceCapacity = priceCapacity;
return this;
}
@CustomType.Setter
public Builder prices(List prices) {
if (prices == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "prices");
}
this.prices = prices;
return this;
}
public Builder prices(GetCartProductPlanPrice... prices) {
return prices(List.of(prices));
}
@CustomType.Setter
public Builder product(String product) {
if (product == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "product");
}
this.product = product;
return this;
}
@CustomType.Setter
public Builder productName(String productName) {
if (productName == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "productName");
}
this.productName = productName;
return this;
}
@CustomType.Setter
public Builder productType(String productType) {
if (productType == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "productType");
}
this.productType = productType;
return this;
}
@CustomType.Setter
public Builder selectedPrices(List selectedPrices) {
if (selectedPrices == null) {
throw new MissingRequiredPropertyException("GetCartProductPlanResult", "selectedPrices");
}
this.selectedPrices = selectedPrices;
return this;
}
public Builder selectedPrices(GetCartProductPlanSelectedPrice... selectedPrices) {
return selectedPrices(List.of(selectedPrices));
}
public GetCartProductPlanResult build() {
final var _resultValue = new GetCartProductPlanResult();
_resultValue.cartId = cartId;
_resultValue.catalogName = catalogName;
_resultValue.id = id;
_resultValue.planCode = planCode;
_resultValue.priceCapacity = priceCapacity;
_resultValue.prices = prices;
_resultValue.product = product;
_resultValue.productName = productName;
_resultValue.productType = productType;
_resultValue.selectedPrices = selectedPrices;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy