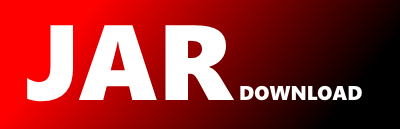
com.ovhcloud.pulumi.ovh.Vps.outputs.VpsModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-ovh Show documentation
Show all versions of pulumi-ovh Show documentation
A Pulumi package for creating and managing OVH resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.ovhcloud.pulumi.ovh.Vps.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VpsModel {
/**
* @return All options the VPS can have (additionalDisk┃automatedBackup┃cpanel┃ftpbackup┃plesk┃snapshot┃veeam┃windows)
*
*/
private @Nullable List availableOptions;
/**
* @return Datacenters where this model is available
*
*/
private @Nullable List datacenters;
/**
* @return Disk capacity of this VPS
*
*/
private @Nullable Double disk;
/**
* @return Maximum number of additional IPs
*
*/
private @Nullable Double maximumAdditionnalIp;
/**
* @return RAM of the VPS
*
*/
private @Nullable Double memory;
/**
* @return Name of the VPS
*
*/
private @Nullable String name;
/**
* @return Description of this VPS offer
*
*/
private @Nullable String offer;
/**
* @return Number of vcores
*
*/
private @Nullable Double vcore;
/**
* @return All versions that VPS can have (2013v1┃2014v1┃2015v1┃2017v1┃2017v2┃2017v3┃2018v1┃2018v2┃2019v1)
*
*/
private @Nullable String version;
private VpsModel() {}
/**
* @return All options the VPS can have (additionalDisk┃automatedBackup┃cpanel┃ftpbackup┃plesk┃snapshot┃veeam┃windows)
*
*/
public List availableOptions() {
return this.availableOptions == null ? List.of() : this.availableOptions;
}
/**
* @return Datacenters where this model is available
*
*/
public List datacenters() {
return this.datacenters == null ? List.of() : this.datacenters;
}
/**
* @return Disk capacity of this VPS
*
*/
public Optional disk() {
return Optional.ofNullable(this.disk);
}
/**
* @return Maximum number of additional IPs
*
*/
public Optional maximumAdditionnalIp() {
return Optional.ofNullable(this.maximumAdditionnalIp);
}
/**
* @return RAM of the VPS
*
*/
public Optional memory() {
return Optional.ofNullable(this.memory);
}
/**
* @return Name of the VPS
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Description of this VPS offer
*
*/
public Optional offer() {
return Optional.ofNullable(this.offer);
}
/**
* @return Number of vcores
*
*/
public Optional vcore() {
return Optional.ofNullable(this.vcore);
}
/**
* @return All versions that VPS can have (2013v1┃2014v1┃2015v1┃2017v1┃2017v2┃2017v3┃2018v1┃2018v2┃2019v1)
*
*/
public Optional version() {
return Optional.ofNullable(this.version);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VpsModel defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List availableOptions;
private @Nullable List datacenters;
private @Nullable Double disk;
private @Nullable Double maximumAdditionnalIp;
private @Nullable Double memory;
private @Nullable String name;
private @Nullable String offer;
private @Nullable Double vcore;
private @Nullable String version;
public Builder() {}
public Builder(VpsModel defaults) {
Objects.requireNonNull(defaults);
this.availableOptions = defaults.availableOptions;
this.datacenters = defaults.datacenters;
this.disk = defaults.disk;
this.maximumAdditionnalIp = defaults.maximumAdditionnalIp;
this.memory = defaults.memory;
this.name = defaults.name;
this.offer = defaults.offer;
this.vcore = defaults.vcore;
this.version = defaults.version;
}
@CustomType.Setter
public Builder availableOptions(@Nullable List availableOptions) {
this.availableOptions = availableOptions;
return this;
}
public Builder availableOptions(String... availableOptions) {
return availableOptions(List.of(availableOptions));
}
@CustomType.Setter
public Builder datacenters(@Nullable List datacenters) {
this.datacenters = datacenters;
return this;
}
public Builder datacenters(String... datacenters) {
return datacenters(List.of(datacenters));
}
@CustomType.Setter
public Builder disk(@Nullable Double disk) {
this.disk = disk;
return this;
}
@CustomType.Setter
public Builder maximumAdditionnalIp(@Nullable Double maximumAdditionnalIp) {
this.maximumAdditionnalIp = maximumAdditionnalIp;
return this;
}
@CustomType.Setter
public Builder memory(@Nullable Double memory) {
this.memory = memory;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder offer(@Nullable String offer) {
this.offer = offer;
return this;
}
@CustomType.Setter
public Builder vcore(@Nullable Double vcore) {
this.vcore = vcore;
return this;
}
@CustomType.Setter
public Builder version(@Nullable String version) {
this.version = version;
return this;
}
public VpsModel build() {
final var _resultValue = new VpsModel();
_resultValue.availableOptions = availableOptions;
_resultValue.datacenters = datacenters;
_resultValue.disk = disk;
_resultValue.maximumAdditionnalIp = maximumAdditionnalIp;
_resultValue.memory = memory;
_resultValue.name = name;
_resultValue.offer = offer;
_resultValue.vcore = vcore;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy