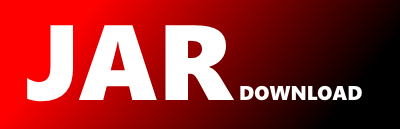
com.palantir.atlasdb.transaction.impl.ForwardingTransaction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of atlasdb-client Show documentation
Show all versions of atlasdb-client Show documentation
Palantir open source project
/*
* (c) Copyright 2018 Palantir Technologies Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.palantir.atlasdb.transaction.impl;
import com.google.common.collect.ForwardingObject;
import com.google.common.util.concurrent.ListenableFuture;
import com.palantir.atlasdb.keyvalue.api.BatchColumnRangeSelection;
import com.palantir.atlasdb.keyvalue.api.Cell;
import com.palantir.atlasdb.keyvalue.api.ColumnRangeSelection;
import com.palantir.atlasdb.keyvalue.api.ColumnSelection;
import com.palantir.atlasdb.keyvalue.api.RangeRequest;
import com.palantir.atlasdb.keyvalue.api.RowResult;
import com.palantir.atlasdb.keyvalue.api.TableReference;
import com.palantir.atlasdb.transaction.api.ConstraintCheckable;
import com.palantir.atlasdb.transaction.api.GetRangesQuery;
import com.palantir.atlasdb.transaction.api.Transaction;
import com.palantir.atlasdb.transaction.api.TransactionFailedException;
import com.palantir.atlasdb.transaction.api.TransactionReadSentinelBehavior;
import com.palantir.atlasdb.transaction.api.ValueAndChangeMetadata;
import com.palantir.atlasdb.transaction.api.annotations.ReviewedRestrictedApiUsage;
import com.palantir.atlasdb.transaction.api.exceptions.MoreCellsPresentThanExpectedException;
import com.palantir.atlasdb.transaction.service.TransactionService;
import com.palantir.common.base.BatchingVisitable;
import com.palantir.lock.watch.ChangeMetadata;
import com.palantir.util.result.Result;
import java.util.Iterator;
import java.util.Map;
import java.util.NavigableMap;
import java.util.Set;
import java.util.function.BiFunction;
import java.util.stream.Stream;
public abstract class ForwardingTransaction extends ForwardingObject implements Transaction {
@Override
public void useTable(TableReference tableRef, ConstraintCheckable table) {
delegate().useTable(tableRef, table);
}
@Override
public abstract Transaction delegate();
@Override
public NavigableMap> getRows(
TableReference tableRef, Iterable rows, ColumnSelection columnSelection) {
return delegate().getRows(tableRef, rows, columnSelection);
}
@Override
public Map>> getRowsColumnRange(
TableReference tableRef, Iterable rows, BatchColumnRangeSelection columnRangeSelection) {
return delegate().getRowsColumnRange(tableRef, rows, columnRangeSelection);
}
@Override
public Map>> getRowsColumnRangeIterator(
TableReference tableRef, Iterable rows, BatchColumnRangeSelection columnRangeSelection) {
return delegate().getRowsColumnRangeIterator(tableRef, rows, columnRangeSelection);
}
@Override
public Iterator> getSortedColumns(
TableReference tableRef, Iterable rows, BatchColumnRangeSelection batchColumnRangeSelection) {
return delegate().getSortedColumns(tableRef, rows, batchColumnRangeSelection);
}
@Override
public Iterator> getRowsColumnRange(
TableReference tableRef, Iterable rows, ColumnRangeSelection columnRangeSelection, int batchHint) {
return delegate().getRowsColumnRange(tableRef, rows, columnRangeSelection, batchHint);
}
@Override
public Map get(TableReference tableRef, Set cells) {
return delegate().get(tableRef, cells);
}
@ReviewedRestrictedApiUsage
@Override
public Result | |
© 2015 - 2025 Weber Informatics LLC | Privacy Policy