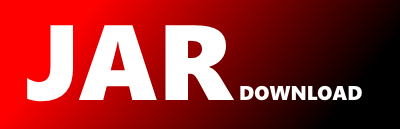
com.palantir.timelock.history.PaxosLogWithAcceptedAndLearnedValues Maven / Gradle / Ivy
package com.palantir.timelock.history;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonSetter;
import com.fasterxml.jackson.annotation.Nulls;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.palantir.logsafe.Preconditions;
import com.palantir.logsafe.SafeArg;
import com.palantir.logsafe.exceptions.SafeIllegalArgumentException;
import com.palantir.paxos.PaxosValue;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
import javax.annotation.Generated;
import javax.annotation.Nonnull;
@JsonDeserialize(builder = PaxosLogWithAcceptedAndLearnedValues.Builder.class)
@Generated("com.palantir.conjure.java.types.BeanGenerator")
public final class PaxosLogWithAcceptedAndLearnedValues {
private final Optional acceptedState;
private final Optional paxosValue;
private final long seq;
private int memoizedHashCode;
private PaxosLogWithAcceptedAndLearnedValues(
Optional acceptedState, Optional paxosValue, long seq) {
validateFields(acceptedState, paxosValue);
this.acceptedState = acceptedState;
this.paxosValue = paxosValue;
this.seq = seq;
}
@JsonProperty("acceptedState")
public Optional getAcceptedState() {
return this.acceptedState;
}
@JsonProperty("paxosValue")
public Optional getPaxosValue() {
return this.paxosValue;
}
@JsonProperty("seq")
public long getSeq() {
return this.seq;
}
@Override
public boolean equals(Object other) {
return this == other
|| (other instanceof PaxosLogWithAcceptedAndLearnedValues
&& equalTo((PaxosLogWithAcceptedAndLearnedValues) other));
}
private boolean equalTo(PaxosLogWithAcceptedAndLearnedValues other) {
return this.acceptedState.equals(other.acceptedState)
&& this.paxosValue.equals(other.paxosValue)
&& this.seq == other.seq;
}
@Override
public int hashCode() {
int result = memoizedHashCode;
if (result == 0) {
result = Objects.hash(this.acceptedState, this.paxosValue, this.seq);
memoizedHashCode = result;
}
return result;
}
@Override
public String toString() {
return "PaxosLogWithAcceptedAndLearnedValues{acceptedState: " + acceptedState + ", paxosValue: " + paxosValue
+ ", seq: " + seq + '}';
}
public static PaxosLogWithAcceptedAndLearnedValues of(
PaxosAcceptorData acceptedState, PaxosValue paxosValue, long seq) {
return builder()
.seq(seq)
.acceptedState(Optional.of(acceptedState))
.paxosValue(Optional.of(paxosValue))
.build();
}
private static void validateFields(Optional acceptedState, Optional paxosValue) {
List missingFields = null;
missingFields = addFieldIfMissing(missingFields, acceptedState, "acceptedState");
missingFields = addFieldIfMissing(missingFields, paxosValue, "paxosValue");
if (missingFields != null) {
throw new SafeIllegalArgumentException(
"Some required fields have not been set", SafeArg.of("missingFields", missingFields));
}
}
private static List addFieldIfMissing(List prev, Object fieldValue, String fieldName) {
List missingFields = prev;
if (fieldValue == null) {
if (missingFields == null) {
missingFields = new ArrayList<>(2);
}
missingFields.add(fieldName);
}
return missingFields;
}
public static Builder builder() {
return new Builder();
}
@Generated("com.palantir.conjure.java.types.BeanBuilderGenerator")
@JsonIgnoreProperties(ignoreUnknown = true)
public static final class Builder {
boolean _buildInvoked;
private Optional acceptedState = Optional.empty();
private Optional paxosValue = Optional.empty();
private long seq;
private boolean _seqInitialized = false;
private Builder() {}
public Builder from(PaxosLogWithAcceptedAndLearnedValues other) {
checkNotBuilt();
acceptedState(other.getAcceptedState());
paxosValue(other.getPaxosValue());
seq(other.getSeq());
return this;
}
@JsonSetter(value = "acceptedState", nulls = Nulls.SKIP)
public Builder acceptedState(@Nonnull Optional acceptedState) {
checkNotBuilt();
this.acceptedState = Preconditions.checkNotNull(acceptedState, "acceptedState cannot be null");
return this;
}
public Builder acceptedState(@Nonnull PaxosAcceptorData acceptedState) {
checkNotBuilt();
this.acceptedState = Optional.of(Preconditions.checkNotNull(acceptedState, "acceptedState cannot be null"));
return this;
}
@JsonSetter(value = "paxosValue", nulls = Nulls.SKIP)
public Builder paxosValue(@Nonnull Optional extends PaxosValue> paxosValue) {
checkNotBuilt();
this.paxosValue = Preconditions.checkNotNull(paxosValue, "paxosValue cannot be null")
.map(Function.identity());
return this;
}
public Builder paxosValue(@Nonnull PaxosValue paxosValue) {
checkNotBuilt();
this.paxosValue = Optional.of(Preconditions.checkNotNull(paxosValue, "paxosValue cannot be null"));
return this;
}
@JsonSetter("seq")
public Builder seq(long seq) {
checkNotBuilt();
this.seq = seq;
this._seqInitialized = true;
return this;
}
private void validatePrimitiveFieldsHaveBeenInitialized() {
List missingFields = null;
missingFields = addFieldIfMissing(missingFields, _seqInitialized, "seq");
if (missingFields != null) {
throw new SafeIllegalArgumentException(
"Some required fields have not been set", SafeArg.of("missingFields", missingFields));
}
}
private static List addFieldIfMissing(List prev, boolean initialized, String fieldName) {
List missingFields = prev;
if (!initialized) {
if (missingFields == null) {
missingFields = new ArrayList<>(1);
}
missingFields.add(fieldName);
}
return missingFields;
}
public PaxosLogWithAcceptedAndLearnedValues build() {
checkNotBuilt();
this._buildInvoked = true;
validatePrimitiveFieldsHaveBeenInitialized();
return new PaxosLogWithAcceptedAndLearnedValues(acceptedState, paxosValue, seq);
}
private void checkNotBuilt() {
Preconditions.checkState(!_buildInvoked, "Build has already been called");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy