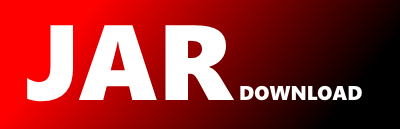
com.palantir.conjure.java.undertow.runtime.TracedEncoding Maven / Gradle / Ivy
/*
* (c) Copyright 2019 Palantir Technologies Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.palantir.conjure.java.undertow.runtime;
import com.google.common.collect.ImmutableMap;
import com.palantir.conjure.java.undertow.lib.Endpoint;
import com.palantir.conjure.java.undertow.lib.TypeMarker;
import com.palantir.tracing.Tracer;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.lang.reflect.Type;
/** Encoding implementation which wraps serialization and deserialization with tracing spans. */
final class TracedEncoding implements Encoding {
static final String DESERIALIZE_OPERATION = "Undertow: deserialize";
static final String SERIALIZE_OPERATION = "Undertow: serialize";
private final Encoding encoding;
private TracedEncoding(Encoding encoding) {
this.encoding = encoding;
}
static Encoding wrap(Encoding encoding) {
return new TracedEncoding(encoding);
}
@Override
public Serializer serializer(TypeMarker type) {
return new TracedSerializer<>(encoding.serializer(type), SERIALIZE_OPERATION, getTags(type));
}
@Override
public Serializer serializer(TypeMarker type, Endpoint endpoint) {
return new TracedSerializer<>(encoding.serializer(type, endpoint), SERIALIZE_OPERATION, getTags(type));
}
@Override
public Deserializer deserializer(TypeMarker type) {
return new TracedDeserializer<>(encoding.deserializer(type), DESERIALIZE_OPERATION, getTags(type));
}
@Override
public Deserializer deserializer(TypeMarker type, Endpoint endpoint) {
return new TracedDeserializer<>(encoding.deserializer(type, endpoint), DESERIALIZE_OPERATION, getTags(type));
}
/**
* Builds a human readable type string. Class types use the classes simple name, however complex types do not have
* this optimization because it is more complex than it's worth for now.
*/
static String toString(TypeMarker> typeMarker) {
Type type = typeMarker.getType();
if (type instanceof Class) {
return ((Class>) type).getSimpleName();
}
return type.toString();
}
@Override
public String getContentType() {
return encoding.getContentType();
}
@Override
public boolean supportsContentType(String contentType) {
return encoding.supportsContentType(contentType);
}
private ImmutableMap getTags(TypeMarker type) {
return ImmutableMap.of("type", toString(type), "contentType", getContentType());
}
private static final class TracedSerializer implements Serializer {
private final Serializer delegate;
private final String operation;
private final ImmutableMap tags;
TracedSerializer(Serializer delegate, String operation, ImmutableMap tags) {
this.delegate = delegate;
this.operation = operation;
this.tags = tags;
}
@Override
public void serialize(T value, OutputStream output) throws IOException {
Tracer.fastStartSpan(operation);
try {
delegate.serialize(value, output);
} finally {
Tracer.fastCompleteSpan(tags);
}
}
}
private static final class TracedDeserializer implements Deserializer {
private final Deserializer delegate;
private final String operation;
private final ImmutableMap tags;
TracedDeserializer(Deserializer delegate, String operation, ImmutableMap tags) {
this.delegate = delegate;
this.operation = operation;
this.tags = tags;
}
@Override
public T deserialize(InputStream input) throws IOException {
Tracer.fastStartSpan(operation);
try {
return delegate.deserialize(input);
} finally {
Tracer.fastCompleteSpan(tags);
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy