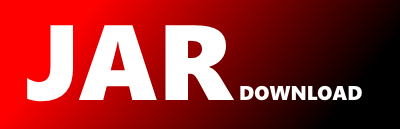
com.palantir.dialogue.core.ClientMetrics Maven / Gradle / Ivy
package com.palantir.dialogue.core;
import com.codahale.metrics.Meter;
import com.codahale.metrics.Timer;
import com.google.errorprone.annotations.CheckReturnValue;
import com.palantir.logsafe.Preconditions;
import com.palantir.logsafe.Safe;
import com.palantir.tritium.metrics.registry.MetricName;
import com.palantir.tritium.metrics.registry.TaggedMetricRegistry;
/**
* General client metrics produced by dialogue. These metrics are meant to be applicable to all conjure clients without being implementation-specific.
*/
final class ClientMetrics {
private static final String JAVA_VERSION = System.getProperty("java.version", "unknown");
private static final String LIBRARY_NAME = "dialogue";
private static final String LIBRARY_VERSION = "4.0.0";
private final TaggedMetricRegistry registry;
private ClientMetrics(TaggedMetricRegistry registry) {
this.registry = registry;
}
static ClientMetrics of(TaggedMetricRegistry registry) {
return new ClientMetrics(Preconditions.checkNotNull(registry, "TaggedMetricRegistry"));
}
/**
* Request time split by status and endpoint. Possible status values are:
*
* - success: 2xx requests, always excludes time spent reading the response body.
* - failure:
*
* - QoS failures (429, 308, 503)
* - 500 requests
* - IOExceptions
*
*
*
*/
@CheckReturnValue
ResponseBuilderChannelNameStage response() {
return new ResponseBuilder();
}
/**
* Rate of deprecated endpoints being invoked.
*/
@CheckReturnValue
Meter deprecations(@Safe String serviceName) {
return registry.meter(deprecationsMetricName(serviceName));
}
static MetricName deprecationsMetricName(@Safe String serviceName) {
return MetricName.builder()
.safeName("client.deprecations")
.putSafeTags("service-name", serviceName)
.putSafeTags("libraryName", LIBRARY_NAME)
.putSafeTags("libraryVersion", LIBRARY_VERSION)
.putSafeTags("javaVersion", JAVA_VERSION)
.build();
}
@Override
public String toString() {
return "ClientMetrics{registry=" + registry + '}';
}
interface ResponseBuildStage {
@CheckReturnValue
Timer build();
@CheckReturnValue
MetricName buildMetricName();
}
interface ResponseBuilderChannelNameStage {
@CheckReturnValue
ResponseBuilderServiceNameStage channelName(@Safe String channelName);
}
interface ResponseBuilderServiceNameStage {
@CheckReturnValue
ResponseBuilderEndpointStage serviceName(@Safe String serviceName);
}
interface ResponseBuilderEndpointStage {
@CheckReturnValue
ResponseBuilderStatusStage endpoint(@Safe String endpoint);
}
interface ResponseBuilderStatusStage {
@CheckReturnValue
ResponseBuildStage status(@Safe String status);
}
private final class ResponseBuilder
implements ResponseBuilderChannelNameStage,
ResponseBuilderServiceNameStage,
ResponseBuilderEndpointStage,
ResponseBuilderStatusStage,
ResponseBuildStage {
private String channelName;
private String serviceName;
private String endpoint;
private String status;
@Override
public ResponseBuilder channelName(@Safe String channelName) {
Preconditions.checkState(this.channelName == null, "channel-name is already set");
this.channelName = Preconditions.checkNotNull(channelName, "channel-name is required");
return this;
}
@Override
public ResponseBuilder serviceName(@Safe String serviceName) {
Preconditions.checkState(this.serviceName == null, "service-name is already set");
this.serviceName = Preconditions.checkNotNull(serviceName, "service-name is required");
return this;
}
@Override
public ResponseBuilder endpoint(@Safe String endpoint) {
Preconditions.checkState(this.endpoint == null, "endpoint is already set");
this.endpoint = Preconditions.checkNotNull(endpoint, "endpoint is required");
return this;
}
@Override
public ResponseBuilder status(@Safe String status) {
Preconditions.checkState(this.status == null, "status is already set");
this.status = Preconditions.checkNotNull(status, "status is required");
return this;
}
@Override
public Timer build() {
return registry.timer(buildMetricName());
}
@Override
public MetricName buildMetricName() {
return MetricName.builder()
.safeName("client.response")
.putSafeTags("channel-name", channelName)
.putSafeTags("service-name", serviceName)
.putSafeTags("endpoint", endpoint)
.putSafeTags("status", status)
.putSafeTags("libraryName", LIBRARY_NAME)
.putSafeTags("libraryVersion", LIBRARY_VERSION)
.putSafeTags("javaVersion", JAVA_VERSION)
.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy