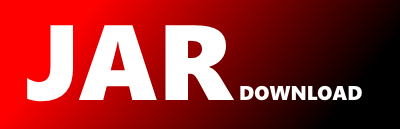
com.palantir.gradle.plugintesting.TestDependencyVersions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of plugin-testing-core Show documentation
Show all versions of plugin-testing-core Show documentation
Palantir open source project
The newest version!
/*
* (c) Copyright 2024 Palantir Technologies Inc. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.palantir.gradle.plugintesting;
import com.google.common.base.Suppliers;
import com.google.common.collect.ImmutableMap;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.util.Map;
import java.util.function.Supplier;
import java.util.stream.Collectors;
/**
* Utility class to keep versions of dependencies referenced in test files up to date with the versions declared in
* the project.
*/
public final class TestDependencyVersions {
static final String TEST_DEPENDENCIES_FILE_SYSTEM_PROPERTY = "TEST_DEPENDENCIES_FILE";
private static final Supplier
© 2015 - 2025 Weber Informatics LLC | Privacy Policy