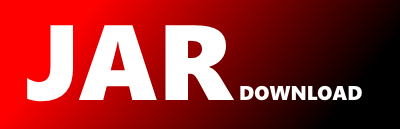
com.google.ortools.constraintsolver.RoutingSearchParameters Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/constraint_solver/routing_parameters.proto
package com.google.ortools.constraintsolver;
/**
*
* Parameters defining the search used to solve vehicle routing problems.
* If a parameter is unset (or, equivalently, set to its default value),
* then the routing library will pick its preferred value for that parameter
* automatically: this should be the case for most parameters.
* To see those "default" parameters, call GetDefaultRoutingSearchParameters().
* Next ID: 35
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters}
*/
public final class RoutingSearchParameters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.RoutingSearchParameters)
RoutingSearchParametersOrBuilder {
private static final long serialVersionUID = 0L;
// Use RoutingSearchParameters.newBuilder() to construct.
private RoutingSearchParameters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RoutingSearchParameters() {
firstSolutionStrategy_ = 0;
localSearchMetaheuristic_ = 0;
useCp_ = 0;
useCpSat_ = 0;
continuousSchedulingSolver_ = 0;
mixedIntegerSchedulingSolver_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RoutingSearchParameters();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RoutingSearchParameters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
firstSolutionStrategy_ = rawValue;
break;
}
case 16: {
useUnfilteredFirstSolutionStrategy_ = input.readBool();
break;
}
case 26: {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder subBuilder = null;
if (localSearchOperators_ != null) {
subBuilder = localSearchOperators_.toBuilder();
}
localSearchOperators_ = input.readMessage(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(localSearchOperators_);
localSearchOperators_ = subBuilder.buildPartial();
}
break;
}
case 32: {
int rawValue = input.readEnum();
localSearchMetaheuristic_ = rawValue;
break;
}
case 41: {
guidedLocalSearchLambdaCoefficient_ = input.readDouble();
break;
}
case 48: {
useDepthFirstSearch_ = input.readBool();
break;
}
case 57: {
optimizationStep_ = input.readDouble();
break;
}
case 64: {
solutionLimit_ = input.readInt64();
break;
}
case 74: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (timeLimit_ != null) {
subBuilder = timeLimit_.toBuilder();
}
timeLimit_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(timeLimit_);
timeLimit_ = subBuilder.buildPartial();
}
break;
}
case 82: {
com.google.protobuf.Duration.Builder subBuilder = null;
if (lnsTimeLimit_ != null) {
subBuilder = lnsTimeLimit_.toBuilder();
}
lnsTimeLimit_ = input.readMessage(com.google.protobuf.Duration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(lnsTimeLimit_);
lnsTimeLimit_ = subBuilder.buildPartial();
}
break;
}
case 88: {
useFullPropagation_ = input.readBool();
break;
}
case 104: {
logSearch_ = input.readBool();
break;
}
case 113: {
savingsNeighborsRatio_ = input.readDouble();
break;
}
case 120: {
savingsAddReverseArcs_ = input.readBool();
break;
}
case 129: {
cheapestInsertionFarthestSeedsRatio_ = input.readDouble();
break;
}
case 136: {
numberOfSolutionsToCollect_ = input.readInt32();
break;
}
case 145: {
savingsArcCoefficient_ = input.readDouble();
break;
}
case 152: {
savingsParallelRoutes_ = input.readBool();
break;
}
case 160: {
relocateExpensiveChainNumArcsToConsider_ = input.readInt32();
break;
}
case 169: {
cheapestInsertionFirstSolutionNeighborsRatio_ = input.readDouble();
break;
}
case 177: {
logCostScalingFactor_ = input.readDouble();
break;
}
case 185: {
savingsMaxMemoryUsageBytes_ = input.readDouble();
break;
}
case 216: {
int rawValue = input.readEnum();
useCpSat_ = rawValue;
break;
}
case 224: {
int rawValue = input.readEnum();
useCp_ = rawValue;
break;
}
case 233: {
logCostOffset_ = input.readDouble();
break;
}
case 240: {
christofidesUseMinimumMatching_ = input.readBool();
break;
}
case 249: {
cheapestInsertionLsOperatorNeighborsRatio_ = input.readDouble();
break;
}
case 256: {
heuristicExpensiveChainLnsNumArcsToConsider_ = input.readInt32();
break;
}
case 264: {
int rawValue = input.readEnum();
continuousSchedulingSolver_ = rawValue;
break;
}
case 272: {
int rawValue = input.readEnum();
mixedIntegerSchedulingSolver_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.class, com.google.ortools.constraintsolver.RoutingSearchParameters.Builder.class);
}
/**
*
* Underlying solver to use in dimension scheduling, respectively for
* continuous and mixed models.
*
*
* Protobuf enum {@code operations_research.RoutingSearchParameters.SchedulingSolver}
*/
public enum SchedulingSolver
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNSET = 0;
*/
UNSET(0),
/**
* GLOP = 1;
*/
GLOP(1),
/**
* CP_SAT = 2;
*/
CP_SAT(2),
UNRECOGNIZED(-1),
;
/**
* UNSET = 0;
*/
public static final int UNSET_VALUE = 0;
/**
* GLOP = 1;
*/
public static final int GLOP_VALUE = 1;
/**
* CP_SAT = 2;
*/
public static final int CP_SAT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SchedulingSolver valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static SchedulingSolver forNumber(int value) {
switch (value) {
case 0: return UNSET;
case 1: return GLOP;
case 2: return CP_SAT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SchedulingSolver> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SchedulingSolver findValueByNumber(int number) {
return SchedulingSolver.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingSearchParameters.getDescriptor().getEnumTypes().get(0);
}
private static final SchedulingSolver[] VALUES = values();
public static SchedulingSolver valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SchedulingSolver(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:operations_research.RoutingSearchParameters.SchedulingSolver)
}
public interface LocalSearchNeighborhoodOperatorsOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
com.google.protobuf.MessageOrBuilder {
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The enum numeric value on the wire for useRelocate.
*/
int getUseRelocateValue();
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The useRelocate.
*/
com.google.ortools.util.OptionalBoolean getUseRelocate();
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The enum numeric value on the wire for useRelocatePair.
*/
int getUseRelocatePairValue();
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The useRelocatePair.
*/
com.google.ortools.util.OptionalBoolean getUseRelocatePair();
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The enum numeric value on the wire for useLightRelocatePair.
*/
int getUseLightRelocatePairValue();
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The useLightRelocatePair.
*/
com.google.ortools.util.OptionalBoolean getUseLightRelocatePair();
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The enum numeric value on the wire for useRelocateNeighbors.
*/
int getUseRelocateNeighborsValue();
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The useRelocateNeighbors.
*/
com.google.ortools.util.OptionalBoolean getUseRelocateNeighbors();
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The enum numeric value on the wire for useRelocateSubtrip.
*/
int getUseRelocateSubtripValue();
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The useRelocateSubtrip.
*/
com.google.ortools.util.OptionalBoolean getUseRelocateSubtrip();
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The enum numeric value on the wire for useExchange.
*/
int getUseExchangeValue();
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The useExchange.
*/
com.google.ortools.util.OptionalBoolean getUseExchange();
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The enum numeric value on the wire for useExchangePair.
*/
int getUseExchangePairValue();
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The useExchangePair.
*/
com.google.ortools.util.OptionalBoolean getUseExchangePair();
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The enum numeric value on the wire for useExchangeSubtrip.
*/
int getUseExchangeSubtripValue();
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The useExchangeSubtrip.
*/
com.google.ortools.util.OptionalBoolean getUseExchangeSubtrip();
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The enum numeric value on the wire for useCross.
*/
int getUseCrossValue();
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The useCross.
*/
com.google.ortools.util.OptionalBoolean getUseCross();
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The enum numeric value on the wire for useCrossExchange.
*/
int getUseCrossExchangeValue();
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The useCrossExchange.
*/
com.google.ortools.util.OptionalBoolean getUseCrossExchange();
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The enum numeric value on the wire for useRelocateExpensiveChain.
*/
int getUseRelocateExpensiveChainValue();
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The useRelocateExpensiveChain.
*/
com.google.ortools.util.OptionalBoolean getUseRelocateExpensiveChain();
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The enum numeric value on the wire for useTwoOpt.
*/
int getUseTwoOptValue();
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The useTwoOpt.
*/
com.google.ortools.util.OptionalBoolean getUseTwoOpt();
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The enum numeric value on the wire for useOrOpt.
*/
int getUseOrOptValue();
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The useOrOpt.
*/
com.google.ortools.util.OptionalBoolean getUseOrOpt();
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The enum numeric value on the wire for useLinKernighan.
*/
int getUseLinKernighanValue();
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The useLinKernighan.
*/
com.google.ortools.util.OptionalBoolean getUseLinKernighan();
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The enum numeric value on the wire for useTspOpt.
*/
int getUseTspOptValue();
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The useTspOpt.
*/
com.google.ortools.util.OptionalBoolean getUseTspOpt();
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The enum numeric value on the wire for useMakeActive.
*/
int getUseMakeActiveValue();
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The useMakeActive.
*/
com.google.ortools.util.OptionalBoolean getUseMakeActive();
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The enum numeric value on the wire for useRelocateAndMakeActive.
*/
int getUseRelocateAndMakeActiveValue();
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The useRelocateAndMakeActive.
*/
com.google.ortools.util.OptionalBoolean getUseRelocateAndMakeActive();
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The enum numeric value on the wire for useMakeInactive.
*/
int getUseMakeInactiveValue();
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The useMakeInactive.
*/
com.google.ortools.util.OptionalBoolean getUseMakeInactive();
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The enum numeric value on the wire for useMakeChainInactive.
*/
int getUseMakeChainInactiveValue();
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The useMakeChainInactive.
*/
com.google.ortools.util.OptionalBoolean getUseMakeChainInactive();
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The enum numeric value on the wire for useSwapActive.
*/
int getUseSwapActiveValue();
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The useSwapActive.
*/
com.google.ortools.util.OptionalBoolean getUseSwapActive();
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The enum numeric value on the wire for useExtendedSwapActive.
*/
int getUseExtendedSwapActiveValue();
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The useExtendedSwapActive.
*/
com.google.ortools.util.OptionalBoolean getUseExtendedSwapActive();
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The enum numeric value on the wire for useNodePairSwapActive.
*/
int getUseNodePairSwapActiveValue();
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The useNodePairSwapActive.
*/
com.google.ortools.util.OptionalBoolean getUseNodePairSwapActive();
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The enum numeric value on the wire for usePathLns.
*/
int getUsePathLnsValue();
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The usePathLns.
*/
com.google.ortools.util.OptionalBoolean getUsePathLns();
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The enum numeric value on the wire for useFullPathLns.
*/
int getUseFullPathLnsValue();
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The useFullPathLns.
*/
com.google.ortools.util.OptionalBoolean getUseFullPathLns();
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The enum numeric value on the wire for useTspLns.
*/
int getUseTspLnsValue();
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The useTspLns.
*/
com.google.ortools.util.OptionalBoolean getUseTspLns();
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The enum numeric value on the wire for useInactiveLns.
*/
int getUseInactiveLnsValue();
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The useInactiveLns.
*/
com.google.ortools.util.OptionalBoolean getUseInactiveLns();
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionPathLns.
*/
int getUseGlobalCheapestInsertionPathLnsValue();
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The useGlobalCheapestInsertionPathLns.
*/
com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionPathLns();
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The enum numeric value on the wire for useLocalCheapestInsertionPathLns.
*/
int getUseLocalCheapestInsertionPathLnsValue();
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The useLocalCheapestInsertionPathLns.
*/
com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionPathLns();
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionExpensiveChainLns.
*/
int getUseGlobalCheapestInsertionExpensiveChainLnsValue();
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The useGlobalCheapestInsertionExpensiveChainLns.
*/
com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionExpensiveChainLns();
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The enum numeric value on the wire for useLocalCheapestInsertionExpensiveChainLns.
*/
int getUseLocalCheapestInsertionExpensiveChainLnsValue();
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The useLocalCheapestInsertionExpensiveChainLns.
*/
com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionExpensiveChainLns();
}
/**
*
* Local search neighborhood operators used to build a solutions neighborhood.
* Next ID: 31
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators}
*/
public static final class LocalSearchNeighborhoodOperators extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
LocalSearchNeighborhoodOperatorsOrBuilder {
private static final long serialVersionUID = 0L;
// Use LocalSearchNeighborhoodOperators.newBuilder() to construct.
private LocalSearchNeighborhoodOperators(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private LocalSearchNeighborhoodOperators() {
useRelocate_ = 0;
useRelocatePair_ = 0;
useLightRelocatePair_ = 0;
useRelocateNeighbors_ = 0;
useRelocateSubtrip_ = 0;
useExchange_ = 0;
useExchangePair_ = 0;
useExchangeSubtrip_ = 0;
useCross_ = 0;
useCrossExchange_ = 0;
useRelocateExpensiveChain_ = 0;
useTwoOpt_ = 0;
useOrOpt_ = 0;
useLinKernighan_ = 0;
useTspOpt_ = 0;
useMakeActive_ = 0;
useRelocateAndMakeActive_ = 0;
useMakeInactive_ = 0;
useMakeChainInactive_ = 0;
useSwapActive_ = 0;
useExtendedSwapActive_ = 0;
useNodePairSwapActive_ = 0;
usePathLns_ = 0;
useFullPathLns_ = 0;
useTspLns_ = 0;
useInactiveLns_ = 0;
useGlobalCheapestInsertionPathLns_ = 0;
useLocalCheapestInsertionPathLns_ = 0;
useGlobalCheapestInsertionExpensiveChainLns_ = 0;
useLocalCheapestInsertionExpensiveChainLns_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new LocalSearchNeighborhoodOperators();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private LocalSearchNeighborhoodOperators(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
useRelocate_ = rawValue;
break;
}
case 16: {
int rawValue = input.readEnum();
useRelocatePair_ = rawValue;
break;
}
case 24: {
int rawValue = input.readEnum();
useRelocateNeighbors_ = rawValue;
break;
}
case 32: {
int rawValue = input.readEnum();
useExchange_ = rawValue;
break;
}
case 40: {
int rawValue = input.readEnum();
useCross_ = rawValue;
break;
}
case 48: {
int rawValue = input.readEnum();
useCrossExchange_ = rawValue;
break;
}
case 56: {
int rawValue = input.readEnum();
useTwoOpt_ = rawValue;
break;
}
case 64: {
int rawValue = input.readEnum();
useOrOpt_ = rawValue;
break;
}
case 72: {
int rawValue = input.readEnum();
useLinKernighan_ = rawValue;
break;
}
case 80: {
int rawValue = input.readEnum();
useTspOpt_ = rawValue;
break;
}
case 88: {
int rawValue = input.readEnum();
useMakeActive_ = rawValue;
break;
}
case 96: {
int rawValue = input.readEnum();
useMakeInactive_ = rawValue;
break;
}
case 104: {
int rawValue = input.readEnum();
useMakeChainInactive_ = rawValue;
break;
}
case 112: {
int rawValue = input.readEnum();
useSwapActive_ = rawValue;
break;
}
case 120: {
int rawValue = input.readEnum();
useExtendedSwapActive_ = rawValue;
break;
}
case 128: {
int rawValue = input.readEnum();
usePathLns_ = rawValue;
break;
}
case 136: {
int rawValue = input.readEnum();
useFullPathLns_ = rawValue;
break;
}
case 144: {
int rawValue = input.readEnum();
useTspLns_ = rawValue;
break;
}
case 152: {
int rawValue = input.readEnum();
useInactiveLns_ = rawValue;
break;
}
case 160: {
int rawValue = input.readEnum();
useNodePairSwapActive_ = rawValue;
break;
}
case 168: {
int rawValue = input.readEnum();
useRelocateAndMakeActive_ = rawValue;
break;
}
case 176: {
int rawValue = input.readEnum();
useExchangePair_ = rawValue;
break;
}
case 184: {
int rawValue = input.readEnum();
useRelocateExpensiveChain_ = rawValue;
break;
}
case 192: {
int rawValue = input.readEnum();
useLightRelocatePair_ = rawValue;
break;
}
case 200: {
int rawValue = input.readEnum();
useRelocateSubtrip_ = rawValue;
break;
}
case 208: {
int rawValue = input.readEnum();
useExchangeSubtrip_ = rawValue;
break;
}
case 216: {
int rawValue = input.readEnum();
useGlobalCheapestInsertionPathLns_ = rawValue;
break;
}
case 224: {
int rawValue = input.readEnum();
useLocalCheapestInsertionPathLns_ = rawValue;
break;
}
case 232: {
int rawValue = input.readEnum();
useGlobalCheapestInsertionExpensiveChainLns_ = rawValue;
break;
}
case 240: {
int rawValue = input.readEnum();
useLocalCheapestInsertionExpensiveChainLns_ = rawValue;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.class, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder.class);
}
public static final int USE_RELOCATE_FIELD_NUMBER = 1;
private int useRelocate_;
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The enum numeric value on the wire for useRelocate.
*/
@java.lang.Override public int getUseRelocateValue() {
return useRelocate_;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The useRelocate.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocate() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocate_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_RELOCATE_PAIR_FIELD_NUMBER = 2;
private int useRelocatePair_;
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The enum numeric value on the wire for useRelocatePair.
*/
@java.lang.Override public int getUseRelocatePairValue() {
return useRelocatePair_;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The useRelocatePair.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocatePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocatePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_LIGHT_RELOCATE_PAIR_FIELD_NUMBER = 24;
private int useLightRelocatePair_;
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The enum numeric value on the wire for useLightRelocatePair.
*/
@java.lang.Override public int getUseLightRelocatePairValue() {
return useLightRelocatePair_;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The useLightRelocatePair.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseLightRelocatePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLightRelocatePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_RELOCATE_NEIGHBORS_FIELD_NUMBER = 3;
private int useRelocateNeighbors_;
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The enum numeric value on the wire for useRelocateNeighbors.
*/
@java.lang.Override public int getUseRelocateNeighborsValue() {
return useRelocateNeighbors_;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The useRelocateNeighbors.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocateNeighbors() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateNeighbors_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_RELOCATE_SUBTRIP_FIELD_NUMBER = 25;
private int useRelocateSubtrip_;
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The enum numeric value on the wire for useRelocateSubtrip.
*/
@java.lang.Override public int getUseRelocateSubtripValue() {
return useRelocateSubtrip_;
}
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The useRelocateSubtrip.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocateSubtrip() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateSubtrip_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_EXCHANGE_FIELD_NUMBER = 4;
private int useExchange_;
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The enum numeric value on the wire for useExchange.
*/
@java.lang.Override public int getUseExchangeValue() {
return useExchange_;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The useExchange.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseExchange() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchange_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_EXCHANGE_PAIR_FIELD_NUMBER = 22;
private int useExchangePair_;
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The enum numeric value on the wire for useExchangePair.
*/
@java.lang.Override public int getUseExchangePairValue() {
return useExchangePair_;
}
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The useExchangePair.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseExchangePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchangePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_EXCHANGE_SUBTRIP_FIELD_NUMBER = 26;
private int useExchangeSubtrip_;
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The enum numeric value on the wire for useExchangeSubtrip.
*/
@java.lang.Override public int getUseExchangeSubtripValue() {
return useExchangeSubtrip_;
}
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The useExchangeSubtrip.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseExchangeSubtrip() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchangeSubtrip_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_CROSS_FIELD_NUMBER = 5;
private int useCross_;
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The enum numeric value on the wire for useCross.
*/
@java.lang.Override public int getUseCrossValue() {
return useCross_;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The useCross.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseCross() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCross_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_CROSS_EXCHANGE_FIELD_NUMBER = 6;
private int useCrossExchange_;
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The enum numeric value on the wire for useCrossExchange.
*/
@java.lang.Override public int getUseCrossExchangeValue() {
return useCrossExchange_;
}
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The useCrossExchange.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseCrossExchange() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCrossExchange_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_RELOCATE_EXPENSIVE_CHAIN_FIELD_NUMBER = 23;
private int useRelocateExpensiveChain_;
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The enum numeric value on the wire for useRelocateExpensiveChain.
*/
@java.lang.Override public int getUseRelocateExpensiveChainValue() {
return useRelocateExpensiveChain_;
}
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The useRelocateExpensiveChain.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocateExpensiveChain() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateExpensiveChain_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_TWO_OPT_FIELD_NUMBER = 7;
private int useTwoOpt_;
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The enum numeric value on the wire for useTwoOpt.
*/
@java.lang.Override public int getUseTwoOptValue() {
return useTwoOpt_;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The useTwoOpt.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseTwoOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTwoOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_OR_OPT_FIELD_NUMBER = 8;
private int useOrOpt_;
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The enum numeric value on the wire for useOrOpt.
*/
@java.lang.Override public int getUseOrOptValue() {
return useOrOpt_;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The useOrOpt.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseOrOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useOrOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_LIN_KERNIGHAN_FIELD_NUMBER = 9;
private int useLinKernighan_;
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The enum numeric value on the wire for useLinKernighan.
*/
@java.lang.Override public int getUseLinKernighanValue() {
return useLinKernighan_;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The useLinKernighan.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseLinKernighan() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLinKernighan_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_TSP_OPT_FIELD_NUMBER = 10;
private int useTspOpt_;
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The enum numeric value on the wire for useTspOpt.
*/
@java.lang.Override public int getUseTspOptValue() {
return useTspOpt_;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The useTspOpt.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseTspOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTspOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_MAKE_ACTIVE_FIELD_NUMBER = 11;
private int useMakeActive_;
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The enum numeric value on the wire for useMakeActive.
*/
@java.lang.Override public int getUseMakeActiveValue() {
return useMakeActive_;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The useMakeActive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseMakeActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_RELOCATE_AND_MAKE_ACTIVE_FIELD_NUMBER = 21;
private int useRelocateAndMakeActive_;
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The enum numeric value on the wire for useRelocateAndMakeActive.
*/
@java.lang.Override public int getUseRelocateAndMakeActiveValue() {
return useRelocateAndMakeActive_;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The useRelocateAndMakeActive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseRelocateAndMakeActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateAndMakeActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_MAKE_INACTIVE_FIELD_NUMBER = 12;
private int useMakeInactive_;
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The enum numeric value on the wire for useMakeInactive.
*/
@java.lang.Override public int getUseMakeInactiveValue() {
return useMakeInactive_;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The useMakeInactive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseMakeInactive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeInactive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_MAKE_CHAIN_INACTIVE_FIELD_NUMBER = 13;
private int useMakeChainInactive_;
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The enum numeric value on the wire for useMakeChainInactive.
*/
@java.lang.Override public int getUseMakeChainInactiveValue() {
return useMakeChainInactive_;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The useMakeChainInactive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseMakeChainInactive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeChainInactive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_SWAP_ACTIVE_FIELD_NUMBER = 14;
private int useSwapActive_;
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The enum numeric value on the wire for useSwapActive.
*/
@java.lang.Override public int getUseSwapActiveValue() {
return useSwapActive_;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The useSwapActive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_EXTENDED_SWAP_ACTIVE_FIELD_NUMBER = 15;
private int useExtendedSwapActive_;
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The enum numeric value on the wire for useExtendedSwapActive.
*/
@java.lang.Override public int getUseExtendedSwapActiveValue() {
return useExtendedSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The useExtendedSwapActive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseExtendedSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExtendedSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_NODE_PAIR_SWAP_ACTIVE_FIELD_NUMBER = 20;
private int useNodePairSwapActive_;
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The enum numeric value on the wire for useNodePairSwapActive.
*/
@java.lang.Override public int getUseNodePairSwapActiveValue() {
return useNodePairSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The useNodePairSwapActive.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseNodePairSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useNodePairSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_PATH_LNS_FIELD_NUMBER = 16;
private int usePathLns_;
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The enum numeric value on the wire for usePathLns.
*/
@java.lang.Override public int getUsePathLnsValue() {
return usePathLns_;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The usePathLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUsePathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(usePathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_FULL_PATH_LNS_FIELD_NUMBER = 17;
private int useFullPathLns_;
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The enum numeric value on the wire for useFullPathLns.
*/
@java.lang.Override public int getUseFullPathLnsValue() {
return useFullPathLns_;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The useFullPathLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseFullPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useFullPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_TSP_LNS_FIELD_NUMBER = 18;
private int useTspLns_;
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The enum numeric value on the wire for useTspLns.
*/
@java.lang.Override public int getUseTspLnsValue() {
return useTspLns_;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The useTspLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseTspLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTspLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_INACTIVE_LNS_FIELD_NUMBER = 19;
private int useInactiveLns_;
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The enum numeric value on the wire for useInactiveLns.
*/
@java.lang.Override public int getUseInactiveLnsValue() {
return useInactiveLns_;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The useInactiveLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseInactiveLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useInactiveLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_GLOBAL_CHEAPEST_INSERTION_PATH_LNS_FIELD_NUMBER = 27;
private int useGlobalCheapestInsertionPathLns_;
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionPathLns.
*/
@java.lang.Override public int getUseGlobalCheapestInsertionPathLnsValue() {
return useGlobalCheapestInsertionPathLns_;
}
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The useGlobalCheapestInsertionPathLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useGlobalCheapestInsertionPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_LOCAL_CHEAPEST_INSERTION_PATH_LNS_FIELD_NUMBER = 28;
private int useLocalCheapestInsertionPathLns_;
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The enum numeric value on the wire for useLocalCheapestInsertionPathLns.
*/
@java.lang.Override public int getUseLocalCheapestInsertionPathLnsValue() {
return useLocalCheapestInsertionPathLns_;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The useLocalCheapestInsertionPathLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLocalCheapestInsertionPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_GLOBAL_CHEAPEST_INSERTION_EXPENSIVE_CHAIN_LNS_FIELD_NUMBER = 29;
private int useGlobalCheapestInsertionExpensiveChainLns_;
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public int getUseGlobalCheapestInsertionExpensiveChainLnsValue() {
return useGlobalCheapestInsertionExpensiveChainLns_;
}
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The useGlobalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionExpensiveChainLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useGlobalCheapestInsertionExpensiveChainLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_LOCAL_CHEAPEST_INSERTION_EXPENSIVE_CHAIN_LNS_FIELD_NUMBER = 30;
private int useLocalCheapestInsertionExpensiveChainLns_;
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The enum numeric value on the wire for useLocalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public int getUseLocalCheapestInsertionExpensiveChainLnsValue() {
return useLocalCheapestInsertionExpensiveChainLns_;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The useLocalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionExpensiveChainLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLocalCheapestInsertionExpensiveChainLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (useRelocate_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(1, useRelocate_);
}
if (useRelocatePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(2, useRelocatePair_);
}
if (useRelocateNeighbors_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(3, useRelocateNeighbors_);
}
if (useExchange_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(4, useExchange_);
}
if (useCross_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(5, useCross_);
}
if (useCrossExchange_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(6, useCrossExchange_);
}
if (useTwoOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(7, useTwoOpt_);
}
if (useOrOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(8, useOrOpt_);
}
if (useLinKernighan_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(9, useLinKernighan_);
}
if (useTspOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(10, useTspOpt_);
}
if (useMakeActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(11, useMakeActive_);
}
if (useMakeInactive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(12, useMakeInactive_);
}
if (useMakeChainInactive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(13, useMakeChainInactive_);
}
if (useSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(14, useSwapActive_);
}
if (useExtendedSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(15, useExtendedSwapActive_);
}
if (usePathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(16, usePathLns_);
}
if (useFullPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(17, useFullPathLns_);
}
if (useTspLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(18, useTspLns_);
}
if (useInactiveLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(19, useInactiveLns_);
}
if (useNodePairSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(20, useNodePairSwapActive_);
}
if (useRelocateAndMakeActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(21, useRelocateAndMakeActive_);
}
if (useExchangePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(22, useExchangePair_);
}
if (useRelocateExpensiveChain_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(23, useRelocateExpensiveChain_);
}
if (useLightRelocatePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(24, useLightRelocatePair_);
}
if (useRelocateSubtrip_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(25, useRelocateSubtrip_);
}
if (useExchangeSubtrip_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(26, useExchangeSubtrip_);
}
if (useGlobalCheapestInsertionPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(27, useGlobalCheapestInsertionPathLns_);
}
if (useLocalCheapestInsertionPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(28, useLocalCheapestInsertionPathLns_);
}
if (useGlobalCheapestInsertionExpensiveChainLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(29, useGlobalCheapestInsertionExpensiveChainLns_);
}
if (useLocalCheapestInsertionExpensiveChainLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(30, useLocalCheapestInsertionExpensiveChainLns_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (useRelocate_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, useRelocate_);
}
if (useRelocatePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, useRelocatePair_);
}
if (useRelocateNeighbors_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, useRelocateNeighbors_);
}
if (useExchange_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, useExchange_);
}
if (useCross_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, useCross_);
}
if (useCrossExchange_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, useCrossExchange_);
}
if (useTwoOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, useTwoOpt_);
}
if (useOrOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(8, useOrOpt_);
}
if (useLinKernighan_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(9, useLinKernighan_);
}
if (useTspOpt_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(10, useTspOpt_);
}
if (useMakeActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(11, useMakeActive_);
}
if (useMakeInactive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(12, useMakeInactive_);
}
if (useMakeChainInactive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, useMakeChainInactive_);
}
if (useSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(14, useSwapActive_);
}
if (useExtendedSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(15, useExtendedSwapActive_);
}
if (usePathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(16, usePathLns_);
}
if (useFullPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(17, useFullPathLns_);
}
if (useTspLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(18, useTspLns_);
}
if (useInactiveLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(19, useInactiveLns_);
}
if (useNodePairSwapActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(20, useNodePairSwapActive_);
}
if (useRelocateAndMakeActive_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(21, useRelocateAndMakeActive_);
}
if (useExchangePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, useExchangePair_);
}
if (useRelocateExpensiveChain_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(23, useRelocateExpensiveChain_);
}
if (useLightRelocatePair_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(24, useLightRelocatePair_);
}
if (useRelocateSubtrip_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(25, useRelocateSubtrip_);
}
if (useExchangeSubtrip_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(26, useExchangeSubtrip_);
}
if (useGlobalCheapestInsertionPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(27, useGlobalCheapestInsertionPathLns_);
}
if (useLocalCheapestInsertionPathLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(28, useLocalCheapestInsertionPathLns_);
}
if (useGlobalCheapestInsertionExpensiveChainLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(29, useGlobalCheapestInsertionExpensiveChainLns_);
}
if (useLocalCheapestInsertionExpensiveChainLns_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(30, useLocalCheapestInsertionExpensiveChainLns_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators)) {
return super.equals(obj);
}
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators other = (com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) obj;
if (useRelocate_ != other.useRelocate_) return false;
if (useRelocatePair_ != other.useRelocatePair_) return false;
if (useLightRelocatePair_ != other.useLightRelocatePair_) return false;
if (useRelocateNeighbors_ != other.useRelocateNeighbors_) return false;
if (useRelocateSubtrip_ != other.useRelocateSubtrip_) return false;
if (useExchange_ != other.useExchange_) return false;
if (useExchangePair_ != other.useExchangePair_) return false;
if (useExchangeSubtrip_ != other.useExchangeSubtrip_) return false;
if (useCross_ != other.useCross_) return false;
if (useCrossExchange_ != other.useCrossExchange_) return false;
if (useRelocateExpensiveChain_ != other.useRelocateExpensiveChain_) return false;
if (useTwoOpt_ != other.useTwoOpt_) return false;
if (useOrOpt_ != other.useOrOpt_) return false;
if (useLinKernighan_ != other.useLinKernighan_) return false;
if (useTspOpt_ != other.useTspOpt_) return false;
if (useMakeActive_ != other.useMakeActive_) return false;
if (useRelocateAndMakeActive_ != other.useRelocateAndMakeActive_) return false;
if (useMakeInactive_ != other.useMakeInactive_) return false;
if (useMakeChainInactive_ != other.useMakeChainInactive_) return false;
if (useSwapActive_ != other.useSwapActive_) return false;
if (useExtendedSwapActive_ != other.useExtendedSwapActive_) return false;
if (useNodePairSwapActive_ != other.useNodePairSwapActive_) return false;
if (usePathLns_ != other.usePathLns_) return false;
if (useFullPathLns_ != other.useFullPathLns_) return false;
if (useTspLns_ != other.useTspLns_) return false;
if (useInactiveLns_ != other.useInactiveLns_) return false;
if (useGlobalCheapestInsertionPathLns_ != other.useGlobalCheapestInsertionPathLns_) return false;
if (useLocalCheapestInsertionPathLns_ != other.useLocalCheapestInsertionPathLns_) return false;
if (useGlobalCheapestInsertionExpensiveChainLns_ != other.useGlobalCheapestInsertionExpensiveChainLns_) return false;
if (useLocalCheapestInsertionExpensiveChainLns_ != other.useLocalCheapestInsertionExpensiveChainLns_) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + USE_RELOCATE_FIELD_NUMBER;
hash = (53 * hash) + useRelocate_;
hash = (37 * hash) + USE_RELOCATE_PAIR_FIELD_NUMBER;
hash = (53 * hash) + useRelocatePair_;
hash = (37 * hash) + USE_LIGHT_RELOCATE_PAIR_FIELD_NUMBER;
hash = (53 * hash) + useLightRelocatePair_;
hash = (37 * hash) + USE_RELOCATE_NEIGHBORS_FIELD_NUMBER;
hash = (53 * hash) + useRelocateNeighbors_;
hash = (37 * hash) + USE_RELOCATE_SUBTRIP_FIELD_NUMBER;
hash = (53 * hash) + useRelocateSubtrip_;
hash = (37 * hash) + USE_EXCHANGE_FIELD_NUMBER;
hash = (53 * hash) + useExchange_;
hash = (37 * hash) + USE_EXCHANGE_PAIR_FIELD_NUMBER;
hash = (53 * hash) + useExchangePair_;
hash = (37 * hash) + USE_EXCHANGE_SUBTRIP_FIELD_NUMBER;
hash = (53 * hash) + useExchangeSubtrip_;
hash = (37 * hash) + USE_CROSS_FIELD_NUMBER;
hash = (53 * hash) + useCross_;
hash = (37 * hash) + USE_CROSS_EXCHANGE_FIELD_NUMBER;
hash = (53 * hash) + useCrossExchange_;
hash = (37 * hash) + USE_RELOCATE_EXPENSIVE_CHAIN_FIELD_NUMBER;
hash = (53 * hash) + useRelocateExpensiveChain_;
hash = (37 * hash) + USE_TWO_OPT_FIELD_NUMBER;
hash = (53 * hash) + useTwoOpt_;
hash = (37 * hash) + USE_OR_OPT_FIELD_NUMBER;
hash = (53 * hash) + useOrOpt_;
hash = (37 * hash) + USE_LIN_KERNIGHAN_FIELD_NUMBER;
hash = (53 * hash) + useLinKernighan_;
hash = (37 * hash) + USE_TSP_OPT_FIELD_NUMBER;
hash = (53 * hash) + useTspOpt_;
hash = (37 * hash) + USE_MAKE_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useMakeActive_;
hash = (37 * hash) + USE_RELOCATE_AND_MAKE_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useRelocateAndMakeActive_;
hash = (37 * hash) + USE_MAKE_INACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useMakeInactive_;
hash = (37 * hash) + USE_MAKE_CHAIN_INACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useMakeChainInactive_;
hash = (37 * hash) + USE_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useSwapActive_;
hash = (37 * hash) + USE_EXTENDED_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useExtendedSwapActive_;
hash = (37 * hash) + USE_NODE_PAIR_SWAP_ACTIVE_FIELD_NUMBER;
hash = (53 * hash) + useNodePairSwapActive_;
hash = (37 * hash) + USE_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + usePathLns_;
hash = (37 * hash) + USE_FULL_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + useFullPathLns_;
hash = (37 * hash) + USE_TSP_LNS_FIELD_NUMBER;
hash = (53 * hash) + useTspLns_;
hash = (37 * hash) + USE_INACTIVE_LNS_FIELD_NUMBER;
hash = (53 * hash) + useInactiveLns_;
hash = (37 * hash) + USE_GLOBAL_CHEAPEST_INSERTION_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + useGlobalCheapestInsertionPathLns_;
hash = (37 * hash) + USE_LOCAL_CHEAPEST_INSERTION_PATH_LNS_FIELD_NUMBER;
hash = (53 * hash) + useLocalCheapestInsertionPathLns_;
hash = (37 * hash) + USE_GLOBAL_CHEAPEST_INSERTION_EXPENSIVE_CHAIN_LNS_FIELD_NUMBER;
hash = (53 * hash) + useGlobalCheapestInsertionExpensiveChainLns_;
hash = (37 * hash) + USE_LOCAL_CHEAPEST_INSERTION_EXPENSIVE_CHAIN_LNS_FIELD_NUMBER;
hash = (53 * hash) + useLocalCheapestInsertionExpensiveChainLns_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Local search neighborhood operators used to build a solutions neighborhood.
* Next ID: 31
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.class, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder.class);
}
// Construct using com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
useRelocate_ = 0;
useRelocatePair_ = 0;
useLightRelocatePair_ = 0;
useRelocateNeighbors_ = 0;
useRelocateSubtrip_ = 0;
useExchange_ = 0;
useExchangePair_ = 0;
useExchangeSubtrip_ = 0;
useCross_ = 0;
useCrossExchange_ = 0;
useRelocateExpensiveChain_ = 0;
useTwoOpt_ = 0;
useOrOpt_ = 0;
useLinKernighan_ = 0;
useTspOpt_ = 0;
useMakeActive_ = 0;
useRelocateAndMakeActive_ = 0;
useMakeInactive_ = 0;
useMakeChainInactive_ = 0;
useSwapActive_ = 0;
useExtendedSwapActive_ = 0;
useNodePairSwapActive_ = 0;
usePathLns_ = 0;
useFullPathLns_ = 0;
useTspLns_ = 0;
useInactiveLns_ = 0;
useGlobalCheapestInsertionPathLns_ = 0;
useLocalCheapestInsertionPathLns_ = 0;
useGlobalCheapestInsertionExpensiveChainLns_ = 0;
useLocalCheapestInsertionExpensiveChainLns_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_LocalSearchNeighborhoodOperators_descriptor;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstanceForType() {
return com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance();
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators build() {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators buildPartial() {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators result = new com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators(this);
result.useRelocate_ = useRelocate_;
result.useRelocatePair_ = useRelocatePair_;
result.useLightRelocatePair_ = useLightRelocatePair_;
result.useRelocateNeighbors_ = useRelocateNeighbors_;
result.useRelocateSubtrip_ = useRelocateSubtrip_;
result.useExchange_ = useExchange_;
result.useExchangePair_ = useExchangePair_;
result.useExchangeSubtrip_ = useExchangeSubtrip_;
result.useCross_ = useCross_;
result.useCrossExchange_ = useCrossExchange_;
result.useRelocateExpensiveChain_ = useRelocateExpensiveChain_;
result.useTwoOpt_ = useTwoOpt_;
result.useOrOpt_ = useOrOpt_;
result.useLinKernighan_ = useLinKernighan_;
result.useTspOpt_ = useTspOpt_;
result.useMakeActive_ = useMakeActive_;
result.useRelocateAndMakeActive_ = useRelocateAndMakeActive_;
result.useMakeInactive_ = useMakeInactive_;
result.useMakeChainInactive_ = useMakeChainInactive_;
result.useSwapActive_ = useSwapActive_;
result.useExtendedSwapActive_ = useExtendedSwapActive_;
result.useNodePairSwapActive_ = useNodePairSwapActive_;
result.usePathLns_ = usePathLns_;
result.useFullPathLns_ = useFullPathLns_;
result.useTspLns_ = useTspLns_;
result.useInactiveLns_ = useInactiveLns_;
result.useGlobalCheapestInsertionPathLns_ = useGlobalCheapestInsertionPathLns_;
result.useLocalCheapestInsertionPathLns_ = useLocalCheapestInsertionPathLns_;
result.useGlobalCheapestInsertionExpensiveChainLns_ = useGlobalCheapestInsertionExpensiveChainLns_;
result.useLocalCheapestInsertionExpensiveChainLns_ = useLocalCheapestInsertionExpensiveChainLns_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) {
return mergeFrom((com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators other) {
if (other == com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance()) return this;
if (other.useRelocate_ != 0) {
setUseRelocateValue(other.getUseRelocateValue());
}
if (other.useRelocatePair_ != 0) {
setUseRelocatePairValue(other.getUseRelocatePairValue());
}
if (other.useLightRelocatePair_ != 0) {
setUseLightRelocatePairValue(other.getUseLightRelocatePairValue());
}
if (other.useRelocateNeighbors_ != 0) {
setUseRelocateNeighborsValue(other.getUseRelocateNeighborsValue());
}
if (other.useRelocateSubtrip_ != 0) {
setUseRelocateSubtripValue(other.getUseRelocateSubtripValue());
}
if (other.useExchange_ != 0) {
setUseExchangeValue(other.getUseExchangeValue());
}
if (other.useExchangePair_ != 0) {
setUseExchangePairValue(other.getUseExchangePairValue());
}
if (other.useExchangeSubtrip_ != 0) {
setUseExchangeSubtripValue(other.getUseExchangeSubtripValue());
}
if (other.useCross_ != 0) {
setUseCrossValue(other.getUseCrossValue());
}
if (other.useCrossExchange_ != 0) {
setUseCrossExchangeValue(other.getUseCrossExchangeValue());
}
if (other.useRelocateExpensiveChain_ != 0) {
setUseRelocateExpensiveChainValue(other.getUseRelocateExpensiveChainValue());
}
if (other.useTwoOpt_ != 0) {
setUseTwoOptValue(other.getUseTwoOptValue());
}
if (other.useOrOpt_ != 0) {
setUseOrOptValue(other.getUseOrOptValue());
}
if (other.useLinKernighan_ != 0) {
setUseLinKernighanValue(other.getUseLinKernighanValue());
}
if (other.useTspOpt_ != 0) {
setUseTspOptValue(other.getUseTspOptValue());
}
if (other.useMakeActive_ != 0) {
setUseMakeActiveValue(other.getUseMakeActiveValue());
}
if (other.useRelocateAndMakeActive_ != 0) {
setUseRelocateAndMakeActiveValue(other.getUseRelocateAndMakeActiveValue());
}
if (other.useMakeInactive_ != 0) {
setUseMakeInactiveValue(other.getUseMakeInactiveValue());
}
if (other.useMakeChainInactive_ != 0) {
setUseMakeChainInactiveValue(other.getUseMakeChainInactiveValue());
}
if (other.useSwapActive_ != 0) {
setUseSwapActiveValue(other.getUseSwapActiveValue());
}
if (other.useExtendedSwapActive_ != 0) {
setUseExtendedSwapActiveValue(other.getUseExtendedSwapActiveValue());
}
if (other.useNodePairSwapActive_ != 0) {
setUseNodePairSwapActiveValue(other.getUseNodePairSwapActiveValue());
}
if (other.usePathLns_ != 0) {
setUsePathLnsValue(other.getUsePathLnsValue());
}
if (other.useFullPathLns_ != 0) {
setUseFullPathLnsValue(other.getUseFullPathLnsValue());
}
if (other.useTspLns_ != 0) {
setUseTspLnsValue(other.getUseTspLnsValue());
}
if (other.useInactiveLns_ != 0) {
setUseInactiveLnsValue(other.getUseInactiveLnsValue());
}
if (other.useGlobalCheapestInsertionPathLns_ != 0) {
setUseGlobalCheapestInsertionPathLnsValue(other.getUseGlobalCheapestInsertionPathLnsValue());
}
if (other.useLocalCheapestInsertionPathLns_ != 0) {
setUseLocalCheapestInsertionPathLnsValue(other.getUseLocalCheapestInsertionPathLnsValue());
}
if (other.useGlobalCheapestInsertionExpensiveChainLns_ != 0) {
setUseGlobalCheapestInsertionExpensiveChainLnsValue(other.getUseGlobalCheapestInsertionExpensiveChainLnsValue());
}
if (other.useLocalCheapestInsertionExpensiveChainLns_ != 0) {
setUseLocalCheapestInsertionExpensiveChainLnsValue(other.getUseLocalCheapestInsertionExpensiveChainLnsValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int useRelocate_ = 0;
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The enum numeric value on the wire for useRelocate.
*/
@java.lang.Override public int getUseRelocateValue() {
return useRelocate_;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @param value The enum numeric value on the wire for useRelocate to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateValue(int value) {
useRelocate_ = value;
onChanged();
return this;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return The useRelocate.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocate() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocate_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @param value The useRelocate to set.
* @return This builder for chaining.
*/
public Builder setUseRelocate(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocate_ = value.getNumber();
onChanged();
return this;
}
/**
*
* --- Inter-route operators ---
* Operator which moves a single node to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> 3 -> [2] -> 4 -> 5
* 1 -> 3 -> 4 -> [2] -> 5
* 1 -> 2 -> 4 -> [3] -> 5
* 1 -> [4] -> 2 -> 3 -> 5
*
*
* .operations_research.OptionalBoolean use_relocate = 1;
* @return This builder for chaining.
*/
public Builder clearUseRelocate() {
useRelocate_ = 0;
onChanged();
return this;
}
private int useRelocatePair_ = 0;
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The enum numeric value on the wire for useRelocatePair.
*/
@java.lang.Override public int getUseRelocatePairValue() {
return useRelocatePair_;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @param value The enum numeric value on the wire for useRelocatePair to set.
* @return This builder for chaining.
*/
public Builder setUseRelocatePairValue(int value) {
useRelocatePair_ = value;
onChanged();
return this;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return The useRelocatePair.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocatePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocatePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @param value The useRelocatePair to set.
* @return This builder for chaining.
*/
public Builder setUseRelocatePair(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocatePair_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes to another
* position where the first node of the pair must be before the second node
* on the same path. Compared to the light_relocate_pair operator, tries all
* possible positions of insertion of a pair (not only after another pair).
* Possible neighbors for the path 1 -> A -> B -> 2 -> 3 (where (1, 3) are
* first and last nodes of the path and can therefore not be moved, and
* (A, B) is a pair of nodes):
* 1 -> [A] -> 2 -> [B] -> 3
* 1 -> 2 -> [A] -> [B] -> 3
*
*
* .operations_research.OptionalBoolean use_relocate_pair = 2;
* @return This builder for chaining.
*/
public Builder clearUseRelocatePair() {
useRelocatePair_ = 0;
onChanged();
return this;
}
private int useLightRelocatePair_ = 0;
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The enum numeric value on the wire for useLightRelocatePair.
*/
@java.lang.Override public int getUseLightRelocatePairValue() {
return useLightRelocatePair_;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @param value The enum numeric value on the wire for useLightRelocatePair to set.
* @return This builder for chaining.
*/
public Builder setUseLightRelocatePairValue(int value) {
useLightRelocatePair_ = value;
onChanged();
return this;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return The useLightRelocatePair.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseLightRelocatePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLightRelocatePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @param value The useLightRelocatePair to set.
* @return This builder for chaining.
*/
public Builder setUseLightRelocatePair(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useLightRelocatePair_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which moves a pair of pickup and delivery nodes after another
* pair.
* Possible neighbors for paths 1 -> A -> B -> 2, 3 -> C -> D -> 4 (where
* (1, 2) and (3, 4) are first and last nodes of paths and can therefore not
* be moved, and (A, B) and (C, D) are pair of nodes):
* 1 -> 2, 3 -> C -> [A] -> D -> [B] -> 4
* 1 -> A -> [C] -> B -> [D] -> 2, 3 -> 4
*
*
* .operations_research.OptionalBoolean use_light_relocate_pair = 24;
* @return This builder for chaining.
*/
public Builder clearUseLightRelocatePair() {
useLightRelocatePair_ = 0;
onChanged();
return this;
}
private int useRelocateNeighbors_ = 0;
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The enum numeric value on the wire for useRelocateNeighbors.
*/
@java.lang.Override public int getUseRelocateNeighborsValue() {
return useRelocateNeighbors_;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @param value The enum numeric value on the wire for useRelocateNeighbors to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateNeighborsValue(int value) {
useRelocateNeighbors_ = value;
onChanged();
return this;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return The useRelocateNeighbors.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocateNeighbors() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateNeighbors_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @param value The useRelocateNeighbors to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateNeighbors(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocateNeighbors_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Relocate neighborhood which moves chains of neighbors.
* The operator starts by relocating a node n after a node m, then continues
* moving nodes which were after n as long as the "cost" added is less than
* the "cost" of the arc (m, n). If the new chain doesn't respect the domain
* of next variables, it will try reordering the nodes until it finds a
* valid path.
* Possible neighbors for path 1 -> A -> B -> C -> D -> E -> 2 (where (1, 2)
* are first and last nodes of the path and can therefore not be moved, A
* must be performed before B, and A, D and E are located at the same
* place):
* 1 -> A -> C -> [B] -> D -> E -> 2
* 1 -> A -> C -> D -> [B] -> E -> 2
* 1 -> A -> C -> D -> E -> [B] -> 2
* 1 -> A -> B -> D -> [C] -> E -> 2
* 1 -> A -> B -> D -> E -> [C] -> 2
* 1 -> A -> [D] -> [E] -> B -> C -> 2
* 1 -> A -> B -> [D] -> [E] -> C -> 2
* 1 -> A -> [E] -> B -> C -> D -> 2
* 1 -> A -> B -> [E] -> C -> D -> 2
* 1 -> A -> B -> C -> [E] -> D -> 2
* This operator is extremelly useful to move chains of nodes which are
* located at the same place (for instance nodes part of a same stop).
*
*
* .operations_research.OptionalBoolean use_relocate_neighbors = 3;
* @return This builder for chaining.
*/
public Builder clearUseRelocateNeighbors() {
useRelocateNeighbors_ = 0;
onChanged();
return this;
}
private int useRelocateSubtrip_ = 0;
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The enum numeric value on the wire for useRelocateSubtrip.
*/
@java.lang.Override public int getUseRelocateSubtripValue() {
return useRelocateSubtrip_;
}
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @param value The enum numeric value on the wire for useRelocateSubtrip to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateSubtripValue(int value) {
useRelocateSubtrip_ = value;
onChanged();
return this;
}
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return The useRelocateSubtrip.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocateSubtrip() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateSubtrip_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @param value The useRelocateSubtrip to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateSubtrip(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocateSubtrip_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Relocate neighborhood that moves subpaths all pickup and delivery
* pairs have both pickup and delivery inside the subpath or both outside
* the subpath. For instance, for given paths:
* 0 -> A -> B -> A' -> B' -> 5 -> 6 -> 8
* 7 -> 9
* Pairs (A,A') and (B,B') are interleaved, so the expected neighbors are:
* 0 -> 5 -> A -> B -> A' -> B' -> 6 -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> A -> B -> A' -> B' -> 8
* 7 -> 9
* 0 -> 5 -> 6 -> 8
* 7 -> A -> B -> A' -> B' -> 9
*
*
* .operations_research.OptionalBoolean use_relocate_subtrip = 25;
* @return This builder for chaining.
*/
public Builder clearUseRelocateSubtrip() {
useRelocateSubtrip_ = 0;
onChanged();
return this;
}
private int useExchange_ = 0;
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The enum numeric value on the wire for useExchange.
*/
@java.lang.Override public int getUseExchangeValue() {
return useExchange_;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @param value The enum numeric value on the wire for useExchange to set.
* @return This builder for chaining.
*/
public Builder setUseExchangeValue(int value) {
useExchange_ = value;
onChanged();
return this;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return The useExchange.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseExchange() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchange_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @param value The useExchange to set.
* @return This builder for chaining.
*/
public Builder setUseExchange(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useExchange_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which exchanges the positions of two nodes.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3] -> [2] -> 4 -> 5
* 1 -> [4] -> 3 -> [2] -> 5
* 1 -> 2 -> [4] -> [3] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange = 4;
* @return This builder for chaining.
*/
public Builder clearUseExchange() {
useExchange_ = 0;
onChanged();
return this;
}
private int useExchangePair_ = 0;
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The enum numeric value on the wire for useExchangePair.
*/
@java.lang.Override public int getUseExchangePairValue() {
return useExchangePair_;
}
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @param value The enum numeric value on the wire for useExchangePair to set.
* @return This builder for chaining.
*/
public Builder setUseExchangePairValue(int value) {
useExchangePair_ = value;
onChanged();
return this;
}
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return The useExchangePair.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseExchangePair() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchangePair_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @param value The useExchangePair to set.
* @return This builder for chaining.
*/
public Builder setUseExchangePair(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useExchangePair_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which exchanges the positions of two pair of nodes. Pairs
* correspond to the pickup and delivery pairs defined in the routing model.
* Possible neighbor for the paths
* 1 -> A -> B -> 2 -> 3 and 4 -> C -> D -> 5
* (where (1, 3) and (4, 5) are first and last nodes of the paths and can
* therefore not be moved, and (A, B) and (C,D) are pairs of nodes):
* 1 -> [C] -> [D] -> 2 -> 3, 4 -> [A] -> [B] -> 5
*
*
* .operations_research.OptionalBoolean use_exchange_pair = 22;
* @return This builder for chaining.
*/
public Builder clearUseExchangePair() {
useExchangePair_ = 0;
onChanged();
return this;
}
private int useExchangeSubtrip_ = 0;
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The enum numeric value on the wire for useExchangeSubtrip.
*/
@java.lang.Override public int getUseExchangeSubtripValue() {
return useExchangeSubtrip_;
}
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @param value The enum numeric value on the wire for useExchangeSubtrip to set.
* @return This builder for chaining.
*/
public Builder setUseExchangeSubtripValue(int value) {
useExchangeSubtrip_ = value;
onChanged();
return this;
}
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return The useExchangeSubtrip.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseExchangeSubtrip() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExchangeSubtrip_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @param value The useExchangeSubtrip to set.
* @return This builder for chaining.
*/
public Builder setUseExchangeSubtrip(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useExchangeSubtrip_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which exchanges subtrips associated to two pairs of nodes,
* see use_relocate_subtrip for a definition of subtrips.
*
*
* .operations_research.OptionalBoolean use_exchange_subtrip = 26;
* @return This builder for chaining.
*/
public Builder clearUseExchangeSubtrip() {
useExchangeSubtrip_ = 0;
onChanged();
return this;
}
private int useCross_ = 0;
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The enum numeric value on the wire for useCross.
*/
@java.lang.Override public int getUseCrossValue() {
return useCross_;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @param value The enum numeric value on the wire for useCross to set.
* @return This builder for chaining.
*/
public Builder setUseCrossValue(int value) {
useCross_ = value;
onChanged();
return this;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return The useCross.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseCross() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCross_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @param value The useCross to set.
* @return This builder for chaining.
*/
public Builder setUseCross(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useCross_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which cross exchanges the starting chains of 2 paths, including
* exchanging the whole paths.
* First and last nodes are not moved.
* Possible neighbors for the paths 1 -> 2 -> 3 -> 4 -> 5 and 6 -> 7 -> 8
* (where (1, 5) and (6, 8) are first and last nodes of the paths and can
* therefore not be moved):
* 1 -> [7] -> 3 -> 4 -> 5 6 -> [2] -> 8
* 1 -> [7] -> 4 -> 5 6 -> [2 -> 3] -> 8
* 1 -> [7] -> 5 6 -> [2 -> 3 -> 4] -> 8
*
*
* .operations_research.OptionalBoolean use_cross = 5;
* @return This builder for chaining.
*/
public Builder clearUseCross() {
useCross_ = 0;
onChanged();
return this;
}
private int useCrossExchange_ = 0;
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The enum numeric value on the wire for useCrossExchange.
*/
@java.lang.Override public int getUseCrossExchangeValue() {
return useCrossExchange_;
}
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @param value The enum numeric value on the wire for useCrossExchange to set.
* @return This builder for chaining.
*/
public Builder setUseCrossExchangeValue(int value) {
useCrossExchange_ = value;
onChanged();
return this;
}
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return The useCrossExchange.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseCrossExchange() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCrossExchange_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @param value The useCrossExchange to set.
* @return This builder for chaining.
*/
public Builder setUseCrossExchange(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useCrossExchange_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Not implemented yet. TODO(b/68128619): Implement.
*
*
* .operations_research.OptionalBoolean use_cross_exchange = 6;
* @return This builder for chaining.
*/
public Builder clearUseCrossExchange() {
useCrossExchange_ = 0;
onChanged();
return this;
}
private int useRelocateExpensiveChain_ = 0;
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The enum numeric value on the wire for useRelocateExpensiveChain.
*/
@java.lang.Override public int getUseRelocateExpensiveChainValue() {
return useRelocateExpensiveChain_;
}
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @param value The enum numeric value on the wire for useRelocateExpensiveChain to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateExpensiveChainValue(int value) {
useRelocateExpensiveChain_ = value;
onChanged();
return this;
}
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return The useRelocateExpensiveChain.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocateExpensiveChain() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateExpensiveChain_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @param value The useRelocateExpensiveChain to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateExpensiveChain(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocateExpensiveChain_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which detects the relocate_expensive_chain_num_arcs_to_consider
* most expensive arcs on a path, and moves the chain resulting from cutting
* pairs of arcs among these to another position.
* Possible neighbors for paths 1 -> 2 (empty) and
* 3 -> A ------> B --> C -----> D -> 4 (where A -> B and C -> D are the 2
* most expensive arcs, and the chain resulting from breaking them is
* B -> C):
* 1 -> [B -> C] -> 2 3 -> A -> D -> 4
* 1 -> 2 3 -> [B -> C] -> A -> D -> 4
* 1 -> 2 3 -> A -> D -> [B -> C] -> 4
*
*
* .operations_research.OptionalBoolean use_relocate_expensive_chain = 23;
* @return This builder for chaining.
*/
public Builder clearUseRelocateExpensiveChain() {
useRelocateExpensiveChain_ = 0;
onChanged();
return this;
}
private int useTwoOpt_ = 0;
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The enum numeric value on the wire for useTwoOpt.
*/
@java.lang.Override public int getUseTwoOptValue() {
return useTwoOpt_;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @param value The enum numeric value on the wire for useTwoOpt to set.
* @return This builder for chaining.
*/
public Builder setUseTwoOptValue(int value) {
useTwoOpt_ = value;
onChanged();
return this;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return The useTwoOpt.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseTwoOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTwoOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @param value The useTwoOpt to set.
* @return This builder for chaining.
*/
public Builder setUseTwoOpt(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useTwoOpt_ = value.getNumber();
onChanged();
return this;
}
/**
*
* --- Intra-route operators ---
* Operator which reverves a sub-chain of a path. It is called TwoOpt
* because it breaks two arcs on the path; resulting paths are called
* two-optimal.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5
* (where (1, 5) are first and last nodes of the path and can therefore not
* be moved):
* 1 -> [3 -> 2] -> 4 -> 5
* 1 -> [4 -> 3 -> 2] -> 5
* 1 -> 2 -> [4 -> 3] -> 5
*
*
* .operations_research.OptionalBoolean use_two_opt = 7;
* @return This builder for chaining.
*/
public Builder clearUseTwoOpt() {
useTwoOpt_ = 0;
onChanged();
return this;
}
private int useOrOpt_ = 0;
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The enum numeric value on the wire for useOrOpt.
*/
@java.lang.Override public int getUseOrOptValue() {
return useOrOpt_;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @param value The enum numeric value on the wire for useOrOpt to set.
* @return This builder for chaining.
*/
public Builder setUseOrOptValue(int value) {
useOrOpt_ = value;
onChanged();
return this;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return The useOrOpt.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseOrOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useOrOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @param value The useOrOpt to set.
* @return This builder for chaining.
*/
public Builder setUseOrOpt(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useOrOpt_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which moves sub-chains of a path of length 1, 2 and 3 to another
* position in the same path.
* When the length of the sub-chain is 1, the operator simply moves a node
* to another position.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 -> 5, for a sub-chain
* length of 2 (where (1, 5) are first and last nodes of the path and can
* therefore not be moved):
* 1 -> 4 -> [2 -> 3] -> 5
* 1 -> [3 -> 4] -> 2 -> 5
* The OR_OPT operator is a limited version of 3-Opt (breaks 3 arcs on a
* path).
*
*
* .operations_research.OptionalBoolean use_or_opt = 8;
* @return This builder for chaining.
*/
public Builder clearUseOrOpt() {
useOrOpt_ = 0;
onChanged();
return this;
}
private int useLinKernighan_ = 0;
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The enum numeric value on the wire for useLinKernighan.
*/
@java.lang.Override public int getUseLinKernighanValue() {
return useLinKernighan_;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @param value The enum numeric value on the wire for useLinKernighan to set.
* @return This builder for chaining.
*/
public Builder setUseLinKernighanValue(int value) {
useLinKernighan_ = value;
onChanged();
return this;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return The useLinKernighan.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseLinKernighan() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLinKernighan_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @param value The useLinKernighan to set.
* @return This builder for chaining.
*/
public Builder setUseLinKernighan(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useLinKernighan_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Lin-Kernighan operator.
* While the accumulated local gain is positive, performs a 2-OPT or a 3-OPT
* move followed by a series of 2-OPT moves. Returns a neighbor for which
* the global gain is positive.
*
*
* .operations_research.OptionalBoolean use_lin_kernighan = 9;
* @return This builder for chaining.
*/
public Builder clearUseLinKernighan() {
useLinKernighan_ = 0;
onChanged();
return this;
}
private int useTspOpt_ = 0;
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The enum numeric value on the wire for useTspOpt.
*/
@java.lang.Override public int getUseTspOptValue() {
return useTspOpt_;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @param value The enum numeric value on the wire for useTspOpt to set.
* @return This builder for chaining.
*/
public Builder setUseTspOptValue(int value) {
useTspOpt_ = value;
onChanged();
return this;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return The useTspOpt.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseTspOpt() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTspOpt_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @param value The useTspOpt to set.
* @return This builder for chaining.
*/
public Builder setUseTspOpt(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useTspOpt_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Sliding TSP operator.
* Uses an exact dynamic programming algorithm to solve the TSP
* corresponding to path sub-chains.
* For a subchain 1 -> 2 -> 3 -> 4 -> 5 -> 6, solves the TSP on
* nodes A, 2, 3, 4, 5, where A is a merger of nodes 1 and 6 such that
* cost(A,i) = cost(1,i) and cost(i,A) = cost(i,6).
*
*
* .operations_research.OptionalBoolean use_tsp_opt = 10;
* @return This builder for chaining.
*/
public Builder clearUseTspOpt() {
useTspOpt_ = 0;
onChanged();
return this;
}
private int useMakeActive_ = 0;
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The enum numeric value on the wire for useMakeActive.
*/
@java.lang.Override public int getUseMakeActiveValue() {
return useMakeActive_;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @param value The enum numeric value on the wire for useMakeActive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeActiveValue(int value) {
useMakeActive_ = value;
onChanged();
return this;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return The useMakeActive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseMakeActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @param value The useMakeActive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeActive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useMakeActive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* --- Operators on inactive nodes ---
* Operator which inserts an inactive node into a path.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 2 -> 3 -> 4
* 1 -> 2 -> [5] -> 3 -> 4
* 1 -> 2 -> 3 -> [5] -> 4
*
*
* .operations_research.OptionalBoolean use_make_active = 11;
* @return This builder for chaining.
*/
public Builder clearUseMakeActive() {
useMakeActive_ = 0;
onChanged();
return this;
}
private int useRelocateAndMakeActive_ = 0;
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The enum numeric value on the wire for useRelocateAndMakeActive.
*/
@java.lang.Override public int getUseRelocateAndMakeActiveValue() {
return useRelocateAndMakeActive_;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @param value The enum numeric value on the wire for useRelocateAndMakeActive to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateAndMakeActiveValue(int value) {
useRelocateAndMakeActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return The useRelocateAndMakeActive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseRelocateAndMakeActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useRelocateAndMakeActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @param value The useRelocateAndMakeActive to set.
* @return This builder for chaining.
*/
public Builder setUseRelocateAndMakeActive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useRelocateAndMakeActive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which relocates a node while making an inactive one active.
* As of 3/2017, the operator is limited to two kinds of moves:
* - Relocating a node and replacing it by an inactive node.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 3 -> 5, 2 -> 4 -> 6.
* - Relocating a node and inserting an inactive node next to it.
* Possible neighbor for path 1 -> 5, 2 -> 3 -> 6 and 4 inactive
* (where 1,2 and 5,6 are first and last nodes of paths) is:
* 1 -> 4 -> 3 -> 5, 2 -> 6.
*
*
* .operations_research.OptionalBoolean use_relocate_and_make_active = 21;
* @return This builder for chaining.
*/
public Builder clearUseRelocateAndMakeActive() {
useRelocateAndMakeActive_ = 0;
onChanged();
return this;
}
private int useMakeInactive_ = 0;
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The enum numeric value on the wire for useMakeInactive.
*/
@java.lang.Override public int getUseMakeInactiveValue() {
return useMakeInactive_;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @param value The enum numeric value on the wire for useMakeInactive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeInactiveValue(int value) {
useMakeInactive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return The useMakeInactive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseMakeInactive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeInactive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @param value The useMakeInactive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeInactive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useMakeInactive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which makes path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_inactive = 12;
* @return This builder for chaining.
*/
public Builder clearUseMakeInactive() {
useMakeInactive_ = 0;
onChanged();
return this;
}
private int useMakeChainInactive_ = 0;
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The enum numeric value on the wire for useMakeChainInactive.
*/
@java.lang.Override public int getUseMakeChainInactiveValue() {
return useMakeChainInactive_;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @param value The enum numeric value on the wire for useMakeChainInactive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeChainInactiveValue(int value) {
useMakeChainInactive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return The useMakeChainInactive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseMakeChainInactive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useMakeChainInactive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @param value The useMakeChainInactive to set.
* @return This builder for chaining.
*/
public Builder setUseMakeChainInactive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useMakeChainInactive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which makes a "chain" of path nodes inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 (where 1 and 4 are first
* and last nodes of the path) are:
* 1 -> 3 -> 4 with 2 inactive
* 1 -> 2 -> 4 with 3 inactive
* 1 -> 4 with 2 and 3 inactive
*
*
* .operations_research.OptionalBoolean use_make_chain_inactive = 13;
* @return This builder for chaining.
*/
public Builder clearUseMakeChainInactive() {
useMakeChainInactive_ = 0;
onChanged();
return this;
}
private int useSwapActive_ = 0;
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The enum numeric value on the wire for useSwapActive.
*/
@java.lang.Override public int getUseSwapActiveValue() {
return useSwapActive_;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @param value The enum numeric value on the wire for useSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseSwapActiveValue(int value) {
useSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return The useSwapActive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @param value The useSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseSwapActive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useSwapActive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which replaces an active node by an inactive one.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_swap_active = 14;
* @return This builder for chaining.
*/
public Builder clearUseSwapActive() {
useSwapActive_ = 0;
onChanged();
return this;
}
private int useExtendedSwapActive_ = 0;
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The enum numeric value on the wire for useExtendedSwapActive.
*/
@java.lang.Override public int getUseExtendedSwapActiveValue() {
return useExtendedSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @param value The enum numeric value on the wire for useExtendedSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseExtendedSwapActiveValue(int value) {
useExtendedSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return The useExtendedSwapActive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseExtendedSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useExtendedSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @param value The useExtendedSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseExtendedSwapActive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useExtendedSwapActive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active one inactive.
* It is similar to SwapActiveOperator excepts that it tries to insert the
* inactive node in all possible positions instead of just the position of
* the node made inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path) are:
* 1 -> [5] -> 3 -> 4 with 2 inactive
* 1 -> 3 -> [5] -> 4 with 2 inactive
* 1 -> [5] -> 2 -> 4 with 3 inactive
* 1 -> 2 -> [5] -> 4 with 3 inactive
*
*
* .operations_research.OptionalBoolean use_extended_swap_active = 15;
* @return This builder for chaining.
*/
public Builder clearUseExtendedSwapActive() {
useExtendedSwapActive_ = 0;
onChanged();
return this;
}
private int useNodePairSwapActive_ = 0;
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The enum numeric value on the wire for useNodePairSwapActive.
*/
@java.lang.Override public int getUseNodePairSwapActiveValue() {
return useNodePairSwapActive_;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @param value The enum numeric value on the wire for useNodePairSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseNodePairSwapActiveValue(int value) {
useNodePairSwapActive_ = value;
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return The useNodePairSwapActive.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseNodePairSwapActive() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useNodePairSwapActive_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @param value The useNodePairSwapActive to set.
* @return This builder for chaining.
*/
public Builder setUseNodePairSwapActive(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useNodePairSwapActive_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which makes an inactive node active and an active pair of nodes
* inactive OR makes an inactive pair of nodes active and an active node
* inactive.
* Possible neighbors for the path 1 -> 2 -> 3 -> 4 with 5 inactive
* (where 1 and 4 are first and last nodes of the path and (2,3) is a pair
* of nodes) are:
* 1 -> [5] -> 4 with (2,3) inactive
* Possible neighbors for the path 1 -> 2 -> 3 with (4,5) inactive
* (where 1 and 3 are first and last nodes of the path and (4,5) is a pair
* of nodes) are:
* 1 -> [4] -> [5] -> 3 with 2 inactive
*
*
* .operations_research.OptionalBoolean use_node_pair_swap_active = 20;
* @return This builder for chaining.
*/
public Builder clearUseNodePairSwapActive() {
useNodePairSwapActive_ = 0;
onChanged();
return this;
}
private int usePathLns_ = 0;
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The enum numeric value on the wire for usePathLns.
*/
@java.lang.Override public int getUsePathLnsValue() {
return usePathLns_;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @param value The enum numeric value on the wire for usePathLns to set.
* @return This builder for chaining.
*/
public Builder setUsePathLnsValue(int value) {
usePathLns_ = value;
onChanged();
return this;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return The usePathLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUsePathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(usePathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @param value The usePathLns to set.
* @return This builder for chaining.
*/
public Builder setUsePathLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
usePathLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* --- Large neighborhood search operators ---
* Operator which relaxes two sub-chains of three consecutive arcs each.
* Each sub-chain is defined by a start node and the next three arcs. Those
* six arcs are relaxed to build a new neighbor.
* PATH_LNS explores all possible pairs of starting nodes and so defines
* n^2 neighbors, n being the number of nodes.
* Note that the two sub-chains can be part of the same path; they even may
* overlap.
*
*
* .operations_research.OptionalBoolean use_path_lns = 16;
* @return This builder for chaining.
*/
public Builder clearUsePathLns() {
usePathLns_ = 0;
onChanged();
return this;
}
private int useFullPathLns_ = 0;
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The enum numeric value on the wire for useFullPathLns.
*/
@java.lang.Override public int getUseFullPathLnsValue() {
return useFullPathLns_;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @param value The enum numeric value on the wire for useFullPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseFullPathLnsValue(int value) {
useFullPathLns_ = value;
onChanged();
return this;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return The useFullPathLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseFullPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useFullPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @param value The useFullPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseFullPathLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useFullPathLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which relaxes one entire path and all unactive nodes.
*
*
* .operations_research.OptionalBoolean use_full_path_lns = 17;
* @return This builder for chaining.
*/
public Builder clearUseFullPathLns() {
useFullPathLns_ = 0;
onChanged();
return this;
}
private int useTspLns_ = 0;
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The enum numeric value on the wire for useTspLns.
*/
@java.lang.Override public int getUseTspLnsValue() {
return useTspLns_;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @param value The enum numeric value on the wire for useTspLns to set.
* @return This builder for chaining.
*/
public Builder setUseTspLnsValue(int value) {
useTspLns_ = value;
onChanged();
return this;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return The useTspLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseTspLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useTspLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @param value The useTspLns to set.
* @return This builder for chaining.
*/
public Builder setUseTspLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useTspLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* TSP-base LNS.
* Randomly merges consecutive nodes until n "meta"-nodes remain and solves
* the corresponding TSP.
* This defines an "unlimited" neighborhood which must be stopped by search
* limits. To force diversification, the operator iteratively forces each
* node to serve as base of a meta-node.
*
*
* .operations_research.OptionalBoolean use_tsp_lns = 18;
* @return This builder for chaining.
*/
public Builder clearUseTspLns() {
useTspLns_ = 0;
onChanged();
return this;
}
private int useInactiveLns_ = 0;
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The enum numeric value on the wire for useInactiveLns.
*/
@java.lang.Override public int getUseInactiveLnsValue() {
return useInactiveLns_;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @param value The enum numeric value on the wire for useInactiveLns to set.
* @return This builder for chaining.
*/
public Builder setUseInactiveLnsValue(int value) {
useInactiveLns_ = value;
onChanged();
return this;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return The useInactiveLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseInactiveLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useInactiveLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @param value The useInactiveLns to set.
* @return This builder for chaining.
*/
public Builder setUseInactiveLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useInactiveLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Operator which relaxes all inactive nodes and one sub-chain of six
* consecutive arcs. That way the path can be improved by inserting inactive
* nodes or swaping arcs.
*
*
* .operations_research.OptionalBoolean use_inactive_lns = 19;
* @return This builder for chaining.
*/
public Builder clearUseInactiveLns() {
useInactiveLns_ = 0;
onChanged();
return this;
}
private int useGlobalCheapestInsertionPathLns_ = 0;
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionPathLns.
*/
@java.lang.Override public int getUseGlobalCheapestInsertionPathLnsValue() {
return useGlobalCheapestInsertionPathLns_;
}
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @param value The enum numeric value on the wire for useGlobalCheapestInsertionPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseGlobalCheapestInsertionPathLnsValue(int value) {
useGlobalCheapestInsertionPathLns_ = value;
onChanged();
return this;
}
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return The useGlobalCheapestInsertionPathLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useGlobalCheapestInsertionPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @param value The useGlobalCheapestInsertionPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseGlobalCheapestInsertionPathLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useGlobalCheapestInsertionPathLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* --- LNS-like large neighborhood search operators using heuristics ---
* Operator which makes all nodes on a route unperformed, and reinserts them
* using the GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_path_lns = 27;
* @return This builder for chaining.
*/
public Builder clearUseGlobalCheapestInsertionPathLns() {
useGlobalCheapestInsertionPathLns_ = 0;
onChanged();
return this;
}
private int useLocalCheapestInsertionPathLns_ = 0;
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The enum numeric value on the wire for useLocalCheapestInsertionPathLns.
*/
@java.lang.Override public int getUseLocalCheapestInsertionPathLnsValue() {
return useLocalCheapestInsertionPathLns_;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @param value The enum numeric value on the wire for useLocalCheapestInsertionPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseLocalCheapestInsertionPathLnsValue(int value) {
useLocalCheapestInsertionPathLns_ = value;
onChanged();
return this;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return The useLocalCheapestInsertionPathLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionPathLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLocalCheapestInsertionPathLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @param value The useLocalCheapestInsertionPathLns to set.
* @return This builder for chaining.
*/
public Builder setUseLocalCheapestInsertionPathLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useLocalCheapestInsertionPathLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_path_lns = 28;
* @return This builder for chaining.
*/
public Builder clearUseLocalCheapestInsertionPathLns() {
useLocalCheapestInsertionPathLns_ = 0;
onChanged();
return this;
}
private int useGlobalCheapestInsertionExpensiveChainLns_ = 0;
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The enum numeric value on the wire for useGlobalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public int getUseGlobalCheapestInsertionExpensiveChainLnsValue() {
return useGlobalCheapestInsertionExpensiveChainLns_;
}
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @param value The enum numeric value on the wire for useGlobalCheapestInsertionExpensiveChainLns to set.
* @return This builder for chaining.
*/
public Builder setUseGlobalCheapestInsertionExpensiveChainLnsValue(int value) {
useGlobalCheapestInsertionExpensiveChainLns_ = value;
onChanged();
return this;
}
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return The useGlobalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseGlobalCheapestInsertionExpensiveChainLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useGlobalCheapestInsertionExpensiveChainLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @param value The useGlobalCheapestInsertionExpensiveChainLns to set.
* @return This builder for chaining.
*/
public Builder setUseGlobalCheapestInsertionExpensiveChainLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useGlobalCheapestInsertionExpensiveChainLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* This operator finds heuristic_expensive_chain_lns_num_arcs_to_consider
* most expensive arcs on a route, makes the nodes in between pairs of these
* expensive arcs unperformed, and reinserts them using the
* GlobalCheapestInsertion heuristic.
*
*
* .operations_research.OptionalBoolean use_global_cheapest_insertion_expensive_chain_lns = 29;
* @return This builder for chaining.
*/
public Builder clearUseGlobalCheapestInsertionExpensiveChainLns() {
useGlobalCheapestInsertionExpensiveChainLns_ = 0;
onChanged();
return this;
}
private int useLocalCheapestInsertionExpensiveChainLns_ = 0;
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The enum numeric value on the wire for useLocalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override public int getUseLocalCheapestInsertionExpensiveChainLnsValue() {
return useLocalCheapestInsertionExpensiveChainLns_;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @param value The enum numeric value on the wire for useLocalCheapestInsertionExpensiveChainLns to set.
* @return This builder for chaining.
*/
public Builder setUseLocalCheapestInsertionExpensiveChainLnsValue(int value) {
useLocalCheapestInsertionExpensiveChainLns_ = value;
onChanged();
return this;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return The useLocalCheapestInsertionExpensiveChainLns.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseLocalCheapestInsertionExpensiveChainLns() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useLocalCheapestInsertionExpensiveChainLns_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @param value The useLocalCheapestInsertionExpensiveChainLns to set.
* @return This builder for chaining.
*/
public Builder setUseLocalCheapestInsertionExpensiveChainLns(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useLocalCheapestInsertionExpensiveChainLns_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Same as above but using LocalCheapestInsertion as a heuristic for
* insertion.
*
*
* .operations_research.OptionalBoolean use_local_cheapest_insertion_expensive_chain_lns = 30;
* @return This builder for chaining.
*/
public Builder clearUseLocalCheapestInsertionExpensiveChainLns() {
useLocalCheapestInsertionExpensiveChainLns_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
}
// @@protoc_insertion_point(class_scope:operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators)
private static final com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators();
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public LocalSearchNeighborhoodOperators parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new LocalSearchNeighborhoodOperators(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FIRST_SOLUTION_STRATEGY_FIELD_NUMBER = 1;
private int firstSolutionStrategy_;
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The enum numeric value on the wire for firstSolutionStrategy.
*/
@java.lang.Override public int getFirstSolutionStrategyValue() {
return firstSolutionStrategy_;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The firstSolutionStrategy.
*/
@java.lang.Override public com.google.ortools.constraintsolver.FirstSolutionStrategy.Value getFirstSolutionStrategy() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.FirstSolutionStrategy.Value result = com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.valueOf(firstSolutionStrategy_);
return result == null ? com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNRECOGNIZED : result;
}
public static final int USE_UNFILTERED_FIRST_SOLUTION_STRATEGY_FIELD_NUMBER = 2;
private boolean useUnfilteredFirstSolutionStrategy_;
/**
*
* --- Advanced first solutions strategy settings ---
* Don't touch these unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_unfiltered_first_solution_strategy = 2;
* @return The useUnfilteredFirstSolutionStrategy.
*/
@java.lang.Override
public boolean getUseUnfilteredFirstSolutionStrategy() {
return useUnfilteredFirstSolutionStrategy_;
}
public static final int SAVINGS_NEIGHBORS_RATIO_FIELD_NUMBER = 14;
private double savingsNeighborsRatio_;
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (in ]0, 1]) of neighbors to consider for each node when constructing
* the savings. If unspecified, its value is considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
* @return The savingsNeighborsRatio.
*/
@java.lang.Override
public double getSavingsNeighborsRatio() {
return savingsNeighborsRatio_;
}
public static final int SAVINGS_MAX_MEMORY_USAGE_BYTES_FIELD_NUMBER = 23;
private double savingsMaxMemoryUsageBytes_;
/**
*
* The number of neighbors considered for each node in the Savings heuristic
* is chosen so that the space used to store the savings doesn't exceed
* savings_max_memory_usage_bytes, which must be in ]0, 1e10].
* NOTE: If both savings_neighbors_ratio and savings_max_memory_usage_bytes
* are specified, the number of neighbors considered for each node will be the
* minimum of the two numbers determined by these parameters.
*
*
* double savings_max_memory_usage_bytes = 23;
* @return The savingsMaxMemoryUsageBytes.
*/
@java.lang.Override
public double getSavingsMaxMemoryUsageBytes() {
return savingsMaxMemoryUsageBytes_;
}
public static final int SAVINGS_ADD_REVERSE_ARCS_FIELD_NUMBER = 15;
private boolean savingsAddReverseArcs_;
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
* @return The savingsAddReverseArcs.
*/
@java.lang.Override
public boolean getSavingsAddReverseArcs() {
return savingsAddReverseArcs_;
}
public static final int SAVINGS_ARC_COEFFICIENT_FIELD_NUMBER = 18;
private double savingsArcCoefficient_;
/**
*
* Coefficient of the cost of the arc for which the saving value is being
* computed:
* Saving(a-->b) = Cost(a-->end) + Cost(start-->b)
* - savings_arc_coefficient * Cost(a-->b)
* This parameter must be greater than 0, and its default value is 1.
*
*
* double savings_arc_coefficient = 18;
* @return The savingsArcCoefficient.
*/
@java.lang.Override
public double getSavingsArcCoefficient() {
return savingsArcCoefficient_;
}
public static final int SAVINGS_PARALLEL_ROUTES_FIELD_NUMBER = 19;
private boolean savingsParallelRoutes_;
/**
*
* When true, the routes are built in parallel, sequentially otherwise.
*
*
* bool savings_parallel_routes = 19;
* @return The savingsParallelRoutes.
*/
@java.lang.Override
public boolean getSavingsParallelRoutes() {
return savingsParallelRoutes_;
}
public static final int CHEAPEST_INSERTION_FARTHEST_SEEDS_RATIO_FIELD_NUMBER = 16;
private double cheapestInsertionFarthestSeedsRatio_;
/**
*
* Ratio (between 0 and 1) of available vehicles in the model on which
* farthest nodes of the model are inserted as seeds in the
* GlobalCheapestInsertion first solution heuristic.
*
*
* double cheapest_insertion_farthest_seeds_ratio = 16;
* @return The cheapestInsertionFarthestSeedsRatio.
*/
@java.lang.Override
public double getCheapestInsertionFarthestSeedsRatio() {
return cheapestInsertionFarthestSeedsRatio_;
}
public static final int CHEAPEST_INSERTION_FIRST_SOLUTION_NEIGHBORS_RATIO_FIELD_NUMBER = 21;
private double cheapestInsertionFirstSolutionNeighborsRatio_;
/**
*
* Ratio (in ]0, 1]) of neighbors to consider for each node when creating
* new insertions in the parallel/sequential cheapest insertion heuristic.
* If not overridden, its default value is 1, meaning all neighbors will be
* considered.
* Neighbors ratio for the first solution heuristic.
*
*
* double cheapest_insertion_first_solution_neighbors_ratio = 21;
* @return The cheapestInsertionFirstSolutionNeighborsRatio.
*/
@java.lang.Override
public double getCheapestInsertionFirstSolutionNeighborsRatio() {
return cheapestInsertionFirstSolutionNeighborsRatio_;
}
public static final int CHEAPEST_INSERTION_LS_OPERATOR_NEIGHBORS_RATIO_FIELD_NUMBER = 31;
private double cheapestInsertionLsOperatorNeighborsRatio_;
/**
*
* Neighbors ratio for the heuristic when used in a local search operator (see
* local_search_operators.use_global_cheapest_insertion_path_lns and
* local_search_operators.use_global_cheapest_insertion_chain_lns below).
*
*
* double cheapest_insertion_ls_operator_neighbors_ratio = 31;
* @return The cheapestInsertionLsOperatorNeighborsRatio.
*/
@java.lang.Override
public double getCheapestInsertionLsOperatorNeighborsRatio() {
return cheapestInsertionLsOperatorNeighborsRatio_;
}
public static final int CHRISTOFIDES_USE_MINIMUM_MATCHING_FIELD_NUMBER = 30;
private boolean christofidesUseMinimumMatching_;
/**
*
* If true use minimum matching instead of minimal matching in the
* Christofides algorithm.
*
*
* bool christofides_use_minimum_matching = 30;
* @return The christofidesUseMinimumMatching.
*/
@java.lang.Override
public boolean getChristofidesUseMinimumMatching() {
return christofidesUseMinimumMatching_;
}
public static final int LOCAL_SEARCH_OPERATORS_FIELD_NUMBER = 3;
private com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators localSearchOperators_;
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return Whether the localSearchOperators field is set.
*/
@java.lang.Override
public boolean hasLocalSearchOperators() {
return localSearchOperators_ != null;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return The localSearchOperators.
*/
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getLocalSearchOperators() {
return localSearchOperators_ == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder getLocalSearchOperatorsOrBuilder() {
return getLocalSearchOperators();
}
public static final int RELOCATE_EXPENSIVE_CHAIN_NUM_ARCS_TO_CONSIDER_FIELD_NUMBER = 20;
private int relocateExpensiveChainNumArcsToConsider_;
/**
*
* Number of expensive arcs to consider cutting in the RelocateExpensiveChain
* neighborhood operator (see
* LocalSearchNeighborhoodOperators.use_relocate_expensive_chain()).
* This parameter must be greater than 2.
* NOTE(user): The number of neighbors generated by the operator for
* relocate_expensive_chain_num_arcs_to_consider = K is around
* K*(K-1)/2 * number_of_routes * number_of_nodes.
*
*
* int32 relocate_expensive_chain_num_arcs_to_consider = 20;
* @return The relocateExpensiveChainNumArcsToConsider.
*/
@java.lang.Override
public int getRelocateExpensiveChainNumArcsToConsider() {
return relocateExpensiveChainNumArcsToConsider_;
}
public static final int HEURISTIC_EXPENSIVE_CHAIN_LNS_NUM_ARCS_TO_CONSIDER_FIELD_NUMBER = 32;
private int heuristicExpensiveChainLnsNumArcsToConsider_;
/**
*
* Number of expensive arcs to consider cutting in the
* FilteredHeuristicExpensiveChainLNSOperator operator.
*
*
* int32 heuristic_expensive_chain_lns_num_arcs_to_consider = 32;
* @return The heuristicExpensiveChainLnsNumArcsToConsider.
*/
@java.lang.Override
public int getHeuristicExpensiveChainLnsNumArcsToConsider() {
return heuristicExpensiveChainLnsNumArcsToConsider_;
}
public static final int LOCAL_SEARCH_METAHEURISTIC_FIELD_NUMBER = 4;
private int localSearchMetaheuristic_;
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The enum numeric value on the wire for localSearchMetaheuristic.
*/
@java.lang.Override public int getLocalSearchMetaheuristicValue() {
return localSearchMetaheuristic_;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The localSearchMetaheuristic.
*/
@java.lang.Override public com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value getLocalSearchMetaheuristic() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value result = com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.valueOf(localSearchMetaheuristic_);
return result == null ? com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNRECOGNIZED : result;
}
public static final int GUIDED_LOCAL_SEARCH_LAMBDA_COEFFICIENT_FIELD_NUMBER = 5;
private double guidedLocalSearchLambdaCoefficient_;
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used. Must be positive.
*
*
* double guided_local_search_lambda_coefficient = 5;
* @return The guidedLocalSearchLambdaCoefficient.
*/
@java.lang.Override
public double getGuidedLocalSearchLambdaCoefficient() {
return guidedLocalSearchLambdaCoefficient_;
}
public static final int USE_DEPTH_FIRST_SEARCH_FIELD_NUMBER = 6;
private boolean useDepthFirstSearch_;
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
* @return The useDepthFirstSearch.
*/
@java.lang.Override
public boolean getUseDepthFirstSearch() {
return useDepthFirstSearch_;
}
public static final int USE_CP_FIELD_NUMBER = 28;
private int useCp_;
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The enum numeric value on the wire for useCp.
*/
@java.lang.Override public int getUseCpValue() {
return useCp_;
}
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The useCp.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseCp() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCp_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int USE_CP_SAT_FIELD_NUMBER = 27;
private int useCpSat_;
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The enum numeric value on the wire for useCpSat.
*/
@java.lang.Override public int getUseCpSatValue() {
return useCpSat_;
}
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The useCpSat.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getUseCpSat() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCpSat_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
public static final int CONTINUOUS_SCHEDULING_SOLVER_FIELD_NUMBER = 33;
private int continuousSchedulingSolver_;
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The enum numeric value on the wire for continuousSchedulingSolver.
*/
@java.lang.Override public int getContinuousSchedulingSolverValue() {
return continuousSchedulingSolver_;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The continuousSchedulingSolver.
*/
@java.lang.Override public com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getContinuousSchedulingSolver() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver result = com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.valueOf(continuousSchedulingSolver_);
return result == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNRECOGNIZED : result;
}
public static final int MIXED_INTEGER_SCHEDULING_SOLVER_FIELD_NUMBER = 34;
private int mixedIntegerSchedulingSolver_;
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The enum numeric value on the wire for mixedIntegerSchedulingSolver.
*/
@java.lang.Override public int getMixedIntegerSchedulingSolverValue() {
return mixedIntegerSchedulingSolver_;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The mixedIntegerSchedulingSolver.
*/
@java.lang.Override public com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getMixedIntegerSchedulingSolver() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver result = com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.valueOf(mixedIntegerSchedulingSolver_);
return result == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNRECOGNIZED : result;
}
public static final int OPTIMIZATION_STEP_FIELD_NUMBER = 7;
private double optimizationStep_;
/**
*
* Minimum step by which the solution must be improved in local search. 0
* means "unspecified". If this value is fractional, it will get rounded to
* the nearest integer.
*
*
* double optimization_step = 7;
* @return The optimizationStep.
*/
@java.lang.Override
public double getOptimizationStep() {
return optimizationStep_;
}
public static final int NUMBER_OF_SOLUTIONS_TO_COLLECT_FIELD_NUMBER = 17;
private int numberOfSolutionsToCollect_;
/**
*
* Number of solutions to collect during the search. Corresponds to the best
* solutions found during the search. 0 means "unspecified".
*
*
* int32 number_of_solutions_to_collect = 17;
* @return The numberOfSolutionsToCollect.
*/
@java.lang.Override
public int getNumberOfSolutionsToCollect() {
return numberOfSolutionsToCollect_;
}
public static final int SOLUTION_LIMIT_FIELD_NUMBER = 8;
private long solutionLimit_;
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search. 0 means
* "unspecified".
*
*
* int64 solution_limit = 8;
* @return The solutionLimit.
*/
@java.lang.Override
public long getSolutionLimit() {
return solutionLimit_;
}
public static final int TIME_LIMIT_FIELD_NUMBER = 9;
private com.google.protobuf.Duration timeLimit_;
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return Whether the timeLimit field is set.
*/
@java.lang.Override
public boolean hasTimeLimit() {
return timeLimit_ != null;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return The timeLimit.
*/
@java.lang.Override
public com.google.protobuf.Duration getTimeLimit() {
return timeLimit_ == null ? com.google.protobuf.Duration.getDefaultInstance() : timeLimit_;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getTimeLimitOrBuilder() {
return getTimeLimit();
}
public static final int LNS_TIME_LIMIT_FIELD_NUMBER = 10;
private com.google.protobuf.Duration lnsTimeLimit_;
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return Whether the lnsTimeLimit field is set.
*/
@java.lang.Override
public boolean hasLnsTimeLimit() {
return lnsTimeLimit_ != null;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return The lnsTimeLimit.
*/
@java.lang.Override
public com.google.protobuf.Duration getLnsTimeLimit() {
return lnsTimeLimit_ == null ? com.google.protobuf.Duration.getDefaultInstance() : lnsTimeLimit_;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
@java.lang.Override
public com.google.protobuf.DurationOrBuilder getLnsTimeLimitOrBuilder() {
return getLnsTimeLimit();
}
public static final int USE_FULL_PROPAGATION_FIELD_NUMBER = 11;
private boolean useFullPropagation_;
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with full propagation in routing model (instead of 'light'
* propagation only). Full propagation is only necessary when using
* depth-first search or for models which require strong propagation to
* finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_full_propagation = 11;
* @return The useFullPropagation.
*/
@java.lang.Override
public boolean getUseFullPropagation() {
return useFullPropagation_;
}
public static final int LOG_SEARCH_FIELD_NUMBER = 13;
private boolean logSearch_;
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
* @return The logSearch.
*/
@java.lang.Override
public boolean getLogSearch() {
return logSearch_;
}
public static final int LOG_COST_SCALING_FACTOR_FIELD_NUMBER = 22;
private double logCostScalingFactor_;
/**
*
* In logs, cost values will be scaled and offset by the given values in the
* following way: log_cost_scaling_factor * (cost + log_cost_offset)
*
*
* double log_cost_scaling_factor = 22;
* @return The logCostScalingFactor.
*/
@java.lang.Override
public double getLogCostScalingFactor() {
return logCostScalingFactor_;
}
public static final int LOG_COST_OFFSET_FIELD_NUMBER = 29;
private double logCostOffset_;
/**
* double log_cost_offset = 29;
* @return The logCostOffset.
*/
@java.lang.Override
public double getLogCostOffset() {
return logCostOffset_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (firstSolutionStrategy_ != com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNSET.getNumber()) {
output.writeEnum(1, firstSolutionStrategy_);
}
if (useUnfilteredFirstSolutionStrategy_ != false) {
output.writeBool(2, useUnfilteredFirstSolutionStrategy_);
}
if (localSearchOperators_ != null) {
output.writeMessage(3, getLocalSearchOperators());
}
if (localSearchMetaheuristic_ != com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNSET.getNumber()) {
output.writeEnum(4, localSearchMetaheuristic_);
}
if (guidedLocalSearchLambdaCoefficient_ != 0D) {
output.writeDouble(5, guidedLocalSearchLambdaCoefficient_);
}
if (useDepthFirstSearch_ != false) {
output.writeBool(6, useDepthFirstSearch_);
}
if (optimizationStep_ != 0D) {
output.writeDouble(7, optimizationStep_);
}
if (solutionLimit_ != 0L) {
output.writeInt64(8, solutionLimit_);
}
if (timeLimit_ != null) {
output.writeMessage(9, getTimeLimit());
}
if (lnsTimeLimit_ != null) {
output.writeMessage(10, getLnsTimeLimit());
}
if (useFullPropagation_ != false) {
output.writeBool(11, useFullPropagation_);
}
if (logSearch_ != false) {
output.writeBool(13, logSearch_);
}
if (savingsNeighborsRatio_ != 0D) {
output.writeDouble(14, savingsNeighborsRatio_);
}
if (savingsAddReverseArcs_ != false) {
output.writeBool(15, savingsAddReverseArcs_);
}
if (cheapestInsertionFarthestSeedsRatio_ != 0D) {
output.writeDouble(16, cheapestInsertionFarthestSeedsRatio_);
}
if (numberOfSolutionsToCollect_ != 0) {
output.writeInt32(17, numberOfSolutionsToCollect_);
}
if (savingsArcCoefficient_ != 0D) {
output.writeDouble(18, savingsArcCoefficient_);
}
if (savingsParallelRoutes_ != false) {
output.writeBool(19, savingsParallelRoutes_);
}
if (relocateExpensiveChainNumArcsToConsider_ != 0) {
output.writeInt32(20, relocateExpensiveChainNumArcsToConsider_);
}
if (cheapestInsertionFirstSolutionNeighborsRatio_ != 0D) {
output.writeDouble(21, cheapestInsertionFirstSolutionNeighborsRatio_);
}
if (logCostScalingFactor_ != 0D) {
output.writeDouble(22, logCostScalingFactor_);
}
if (savingsMaxMemoryUsageBytes_ != 0D) {
output.writeDouble(23, savingsMaxMemoryUsageBytes_);
}
if (useCpSat_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(27, useCpSat_);
}
if (useCp_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
output.writeEnum(28, useCp_);
}
if (logCostOffset_ != 0D) {
output.writeDouble(29, logCostOffset_);
}
if (christofidesUseMinimumMatching_ != false) {
output.writeBool(30, christofidesUseMinimumMatching_);
}
if (cheapestInsertionLsOperatorNeighborsRatio_ != 0D) {
output.writeDouble(31, cheapestInsertionLsOperatorNeighborsRatio_);
}
if (heuristicExpensiveChainLnsNumArcsToConsider_ != 0) {
output.writeInt32(32, heuristicExpensiveChainLnsNumArcsToConsider_);
}
if (continuousSchedulingSolver_ != com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNSET.getNumber()) {
output.writeEnum(33, continuousSchedulingSolver_);
}
if (mixedIntegerSchedulingSolver_ != com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNSET.getNumber()) {
output.writeEnum(34, mixedIntegerSchedulingSolver_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (firstSolutionStrategy_ != com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, firstSolutionStrategy_);
}
if (useUnfilteredFirstSolutionStrategy_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, useUnfilteredFirstSolutionStrategy_);
}
if (localSearchOperators_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getLocalSearchOperators());
}
if (localSearchMetaheuristic_ != com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, localSearchMetaheuristic_);
}
if (guidedLocalSearchLambdaCoefficient_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(5, guidedLocalSearchLambdaCoefficient_);
}
if (useDepthFirstSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(6, useDepthFirstSearch_);
}
if (optimizationStep_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(7, optimizationStep_);
}
if (solutionLimit_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, solutionLimit_);
}
if (timeLimit_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, getTimeLimit());
}
if (lnsTimeLimit_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, getLnsTimeLimit());
}
if (useFullPropagation_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(11, useFullPropagation_);
}
if (logSearch_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(13, logSearch_);
}
if (savingsNeighborsRatio_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(14, savingsNeighborsRatio_);
}
if (savingsAddReverseArcs_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, savingsAddReverseArcs_);
}
if (cheapestInsertionFarthestSeedsRatio_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(16, cheapestInsertionFarthestSeedsRatio_);
}
if (numberOfSolutionsToCollect_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(17, numberOfSolutionsToCollect_);
}
if (savingsArcCoefficient_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(18, savingsArcCoefficient_);
}
if (savingsParallelRoutes_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(19, savingsParallelRoutes_);
}
if (relocateExpensiveChainNumArcsToConsider_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(20, relocateExpensiveChainNumArcsToConsider_);
}
if (cheapestInsertionFirstSolutionNeighborsRatio_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(21, cheapestInsertionFirstSolutionNeighborsRatio_);
}
if (logCostScalingFactor_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(22, logCostScalingFactor_);
}
if (savingsMaxMemoryUsageBytes_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(23, savingsMaxMemoryUsageBytes_);
}
if (useCpSat_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(27, useCpSat_);
}
if (useCp_ != com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(28, useCp_);
}
if (logCostOffset_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(29, logCostOffset_);
}
if (christofidesUseMinimumMatching_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(30, christofidesUseMinimumMatching_);
}
if (cheapestInsertionLsOperatorNeighborsRatio_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(31, cheapestInsertionLsOperatorNeighborsRatio_);
}
if (heuristicExpensiveChainLnsNumArcsToConsider_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(32, heuristicExpensiveChainLnsNumArcsToConsider_);
}
if (continuousSchedulingSolver_ != com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(33, continuousSchedulingSolver_);
}
if (mixedIntegerSchedulingSolver_ != com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNSET.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(34, mixedIntegerSchedulingSolver_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.constraintsolver.RoutingSearchParameters)) {
return super.equals(obj);
}
com.google.ortools.constraintsolver.RoutingSearchParameters other = (com.google.ortools.constraintsolver.RoutingSearchParameters) obj;
if (firstSolutionStrategy_ != other.firstSolutionStrategy_) return false;
if (getUseUnfilteredFirstSolutionStrategy()
!= other.getUseUnfilteredFirstSolutionStrategy()) return false;
if (java.lang.Double.doubleToLongBits(getSavingsNeighborsRatio())
!= java.lang.Double.doubleToLongBits(
other.getSavingsNeighborsRatio())) return false;
if (java.lang.Double.doubleToLongBits(getSavingsMaxMemoryUsageBytes())
!= java.lang.Double.doubleToLongBits(
other.getSavingsMaxMemoryUsageBytes())) return false;
if (getSavingsAddReverseArcs()
!= other.getSavingsAddReverseArcs()) return false;
if (java.lang.Double.doubleToLongBits(getSavingsArcCoefficient())
!= java.lang.Double.doubleToLongBits(
other.getSavingsArcCoefficient())) return false;
if (getSavingsParallelRoutes()
!= other.getSavingsParallelRoutes()) return false;
if (java.lang.Double.doubleToLongBits(getCheapestInsertionFarthestSeedsRatio())
!= java.lang.Double.doubleToLongBits(
other.getCheapestInsertionFarthestSeedsRatio())) return false;
if (java.lang.Double.doubleToLongBits(getCheapestInsertionFirstSolutionNeighborsRatio())
!= java.lang.Double.doubleToLongBits(
other.getCheapestInsertionFirstSolutionNeighborsRatio())) return false;
if (java.lang.Double.doubleToLongBits(getCheapestInsertionLsOperatorNeighborsRatio())
!= java.lang.Double.doubleToLongBits(
other.getCheapestInsertionLsOperatorNeighborsRatio())) return false;
if (getChristofidesUseMinimumMatching()
!= other.getChristofidesUseMinimumMatching()) return false;
if (hasLocalSearchOperators() != other.hasLocalSearchOperators()) return false;
if (hasLocalSearchOperators()) {
if (!getLocalSearchOperators()
.equals(other.getLocalSearchOperators())) return false;
}
if (getRelocateExpensiveChainNumArcsToConsider()
!= other.getRelocateExpensiveChainNumArcsToConsider()) return false;
if (getHeuristicExpensiveChainLnsNumArcsToConsider()
!= other.getHeuristicExpensiveChainLnsNumArcsToConsider()) return false;
if (localSearchMetaheuristic_ != other.localSearchMetaheuristic_) return false;
if (java.lang.Double.doubleToLongBits(getGuidedLocalSearchLambdaCoefficient())
!= java.lang.Double.doubleToLongBits(
other.getGuidedLocalSearchLambdaCoefficient())) return false;
if (getUseDepthFirstSearch()
!= other.getUseDepthFirstSearch()) return false;
if (useCp_ != other.useCp_) return false;
if (useCpSat_ != other.useCpSat_) return false;
if (continuousSchedulingSolver_ != other.continuousSchedulingSolver_) return false;
if (mixedIntegerSchedulingSolver_ != other.mixedIntegerSchedulingSolver_) return false;
if (java.lang.Double.doubleToLongBits(getOptimizationStep())
!= java.lang.Double.doubleToLongBits(
other.getOptimizationStep())) return false;
if (getNumberOfSolutionsToCollect()
!= other.getNumberOfSolutionsToCollect()) return false;
if (getSolutionLimit()
!= other.getSolutionLimit()) return false;
if (hasTimeLimit() != other.hasTimeLimit()) return false;
if (hasTimeLimit()) {
if (!getTimeLimit()
.equals(other.getTimeLimit())) return false;
}
if (hasLnsTimeLimit() != other.hasLnsTimeLimit()) return false;
if (hasLnsTimeLimit()) {
if (!getLnsTimeLimit()
.equals(other.getLnsTimeLimit())) return false;
}
if (getUseFullPropagation()
!= other.getUseFullPropagation()) return false;
if (getLogSearch()
!= other.getLogSearch()) return false;
if (java.lang.Double.doubleToLongBits(getLogCostScalingFactor())
!= java.lang.Double.doubleToLongBits(
other.getLogCostScalingFactor())) return false;
if (java.lang.Double.doubleToLongBits(getLogCostOffset())
!= java.lang.Double.doubleToLongBits(
other.getLogCostOffset())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FIRST_SOLUTION_STRATEGY_FIELD_NUMBER;
hash = (53 * hash) + firstSolutionStrategy_;
hash = (37 * hash) + USE_UNFILTERED_FIRST_SOLUTION_STRATEGY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseUnfilteredFirstSolutionStrategy());
hash = (37 * hash) + SAVINGS_NEIGHBORS_RATIO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSavingsNeighborsRatio()));
hash = (37 * hash) + SAVINGS_MAX_MEMORY_USAGE_BYTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSavingsMaxMemoryUsageBytes()));
hash = (37 * hash) + SAVINGS_ADD_REVERSE_ARCS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSavingsAddReverseArcs());
hash = (37 * hash) + SAVINGS_ARC_COEFFICIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getSavingsArcCoefficient()));
hash = (37 * hash) + SAVINGS_PARALLEL_ROUTES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSavingsParallelRoutes());
hash = (37 * hash) + CHEAPEST_INSERTION_FARTHEST_SEEDS_RATIO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCheapestInsertionFarthestSeedsRatio()));
hash = (37 * hash) + CHEAPEST_INSERTION_FIRST_SOLUTION_NEIGHBORS_RATIO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCheapestInsertionFirstSolutionNeighborsRatio()));
hash = (37 * hash) + CHEAPEST_INSERTION_LS_OPERATOR_NEIGHBORS_RATIO_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getCheapestInsertionLsOperatorNeighborsRatio()));
hash = (37 * hash) + CHRISTOFIDES_USE_MINIMUM_MATCHING_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getChristofidesUseMinimumMatching());
if (hasLocalSearchOperators()) {
hash = (37 * hash) + LOCAL_SEARCH_OPERATORS_FIELD_NUMBER;
hash = (53 * hash) + getLocalSearchOperators().hashCode();
}
hash = (37 * hash) + RELOCATE_EXPENSIVE_CHAIN_NUM_ARCS_TO_CONSIDER_FIELD_NUMBER;
hash = (53 * hash) + getRelocateExpensiveChainNumArcsToConsider();
hash = (37 * hash) + HEURISTIC_EXPENSIVE_CHAIN_LNS_NUM_ARCS_TO_CONSIDER_FIELD_NUMBER;
hash = (53 * hash) + getHeuristicExpensiveChainLnsNumArcsToConsider();
hash = (37 * hash) + LOCAL_SEARCH_METAHEURISTIC_FIELD_NUMBER;
hash = (53 * hash) + localSearchMetaheuristic_;
hash = (37 * hash) + GUIDED_LOCAL_SEARCH_LAMBDA_COEFFICIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getGuidedLocalSearchLambdaCoefficient()));
hash = (37 * hash) + USE_DEPTH_FIRST_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseDepthFirstSearch());
hash = (37 * hash) + USE_CP_FIELD_NUMBER;
hash = (53 * hash) + useCp_;
hash = (37 * hash) + USE_CP_SAT_FIELD_NUMBER;
hash = (53 * hash) + useCpSat_;
hash = (37 * hash) + CONTINUOUS_SCHEDULING_SOLVER_FIELD_NUMBER;
hash = (53 * hash) + continuousSchedulingSolver_;
hash = (37 * hash) + MIXED_INTEGER_SCHEDULING_SOLVER_FIELD_NUMBER;
hash = (53 * hash) + mixedIntegerSchedulingSolver_;
hash = (37 * hash) + OPTIMIZATION_STEP_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getOptimizationStep()));
hash = (37 * hash) + NUMBER_OF_SOLUTIONS_TO_COLLECT_FIELD_NUMBER;
hash = (53 * hash) + getNumberOfSolutionsToCollect();
hash = (37 * hash) + SOLUTION_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSolutionLimit());
if (hasTimeLimit()) {
hash = (37 * hash) + TIME_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getTimeLimit().hashCode();
}
if (hasLnsTimeLimit()) {
hash = (37 * hash) + LNS_TIME_LIMIT_FIELD_NUMBER;
hash = (53 * hash) + getLnsTimeLimit().hashCode();
}
hash = (37 * hash) + USE_FULL_PROPAGATION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseFullPropagation());
hash = (37 * hash) + LOG_SEARCH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getLogSearch());
hash = (37 * hash) + LOG_COST_SCALING_FACTOR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLogCostScalingFactor()));
hash = (37 * hash) + LOG_COST_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getLogCostOffset()));
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.constraintsolver.RoutingSearchParameters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Parameters defining the search used to solve vehicle routing problems.
* If a parameter is unset (or, equivalently, set to its default value),
* then the routing library will pick its preferred value for that parameter
* automatically: this should be the case for most parameters.
* To see those "default" parameters, call GetDefaultRoutingSearchParameters().
* Next ID: 35
*
*
* Protobuf type {@code operations_research.RoutingSearchParameters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.RoutingSearchParameters)
com.google.ortools.constraintsolver.RoutingSearchParametersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.constraintsolver.RoutingSearchParameters.class, com.google.ortools.constraintsolver.RoutingSearchParameters.Builder.class);
}
// Construct using com.google.ortools.constraintsolver.RoutingSearchParameters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
firstSolutionStrategy_ = 0;
useUnfilteredFirstSolutionStrategy_ = false;
savingsNeighborsRatio_ = 0D;
savingsMaxMemoryUsageBytes_ = 0D;
savingsAddReverseArcs_ = false;
savingsArcCoefficient_ = 0D;
savingsParallelRoutes_ = false;
cheapestInsertionFarthestSeedsRatio_ = 0D;
cheapestInsertionFirstSolutionNeighborsRatio_ = 0D;
cheapestInsertionLsOperatorNeighborsRatio_ = 0D;
christofidesUseMinimumMatching_ = false;
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = null;
} else {
localSearchOperators_ = null;
localSearchOperatorsBuilder_ = null;
}
relocateExpensiveChainNumArcsToConsider_ = 0;
heuristicExpensiveChainLnsNumArcsToConsider_ = 0;
localSearchMetaheuristic_ = 0;
guidedLocalSearchLambdaCoefficient_ = 0D;
useDepthFirstSearch_ = false;
useCp_ = 0;
useCpSat_ = 0;
continuousSchedulingSolver_ = 0;
mixedIntegerSchedulingSolver_ = 0;
optimizationStep_ = 0D;
numberOfSolutionsToCollect_ = 0;
solutionLimit_ = 0L;
if (timeLimitBuilder_ == null) {
timeLimit_ = null;
} else {
timeLimit_ = null;
timeLimitBuilder_ = null;
}
if (lnsTimeLimitBuilder_ == null) {
lnsTimeLimit_ = null;
} else {
lnsTimeLimit_ = null;
lnsTimeLimitBuilder_ = null;
}
useFullPropagation_ = false;
logSearch_ = false;
logCostScalingFactor_ = 0D;
logCostOffset_ = 0D;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.constraintsolver.RoutingParameters.internal_static_operations_research_RoutingSearchParameters_descriptor;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstanceForType() {
return com.google.ortools.constraintsolver.RoutingSearchParameters.getDefaultInstance();
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters build() {
com.google.ortools.constraintsolver.RoutingSearchParameters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters buildPartial() {
com.google.ortools.constraintsolver.RoutingSearchParameters result = new com.google.ortools.constraintsolver.RoutingSearchParameters(this);
result.firstSolutionStrategy_ = firstSolutionStrategy_;
result.useUnfilteredFirstSolutionStrategy_ = useUnfilteredFirstSolutionStrategy_;
result.savingsNeighborsRatio_ = savingsNeighborsRatio_;
result.savingsMaxMemoryUsageBytes_ = savingsMaxMemoryUsageBytes_;
result.savingsAddReverseArcs_ = savingsAddReverseArcs_;
result.savingsArcCoefficient_ = savingsArcCoefficient_;
result.savingsParallelRoutes_ = savingsParallelRoutes_;
result.cheapestInsertionFarthestSeedsRatio_ = cheapestInsertionFarthestSeedsRatio_;
result.cheapestInsertionFirstSolutionNeighborsRatio_ = cheapestInsertionFirstSolutionNeighborsRatio_;
result.cheapestInsertionLsOperatorNeighborsRatio_ = cheapestInsertionLsOperatorNeighborsRatio_;
result.christofidesUseMinimumMatching_ = christofidesUseMinimumMatching_;
if (localSearchOperatorsBuilder_ == null) {
result.localSearchOperators_ = localSearchOperators_;
} else {
result.localSearchOperators_ = localSearchOperatorsBuilder_.build();
}
result.relocateExpensiveChainNumArcsToConsider_ = relocateExpensiveChainNumArcsToConsider_;
result.heuristicExpensiveChainLnsNumArcsToConsider_ = heuristicExpensiveChainLnsNumArcsToConsider_;
result.localSearchMetaheuristic_ = localSearchMetaheuristic_;
result.guidedLocalSearchLambdaCoefficient_ = guidedLocalSearchLambdaCoefficient_;
result.useDepthFirstSearch_ = useDepthFirstSearch_;
result.useCp_ = useCp_;
result.useCpSat_ = useCpSat_;
result.continuousSchedulingSolver_ = continuousSchedulingSolver_;
result.mixedIntegerSchedulingSolver_ = mixedIntegerSchedulingSolver_;
result.optimizationStep_ = optimizationStep_;
result.numberOfSolutionsToCollect_ = numberOfSolutionsToCollect_;
result.solutionLimit_ = solutionLimit_;
if (timeLimitBuilder_ == null) {
result.timeLimit_ = timeLimit_;
} else {
result.timeLimit_ = timeLimitBuilder_.build();
}
if (lnsTimeLimitBuilder_ == null) {
result.lnsTimeLimit_ = lnsTimeLimit_;
} else {
result.lnsTimeLimit_ = lnsTimeLimitBuilder_.build();
}
result.useFullPropagation_ = useFullPropagation_;
result.logSearch_ = logSearch_;
result.logCostScalingFactor_ = logCostScalingFactor_;
result.logCostOffset_ = logCostOffset_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.constraintsolver.RoutingSearchParameters) {
return mergeFrom((com.google.ortools.constraintsolver.RoutingSearchParameters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.constraintsolver.RoutingSearchParameters other) {
if (other == com.google.ortools.constraintsolver.RoutingSearchParameters.getDefaultInstance()) return this;
if (other.firstSolutionStrategy_ != 0) {
setFirstSolutionStrategyValue(other.getFirstSolutionStrategyValue());
}
if (other.getUseUnfilteredFirstSolutionStrategy() != false) {
setUseUnfilteredFirstSolutionStrategy(other.getUseUnfilteredFirstSolutionStrategy());
}
if (other.getSavingsNeighborsRatio() != 0D) {
setSavingsNeighborsRatio(other.getSavingsNeighborsRatio());
}
if (other.getSavingsMaxMemoryUsageBytes() != 0D) {
setSavingsMaxMemoryUsageBytes(other.getSavingsMaxMemoryUsageBytes());
}
if (other.getSavingsAddReverseArcs() != false) {
setSavingsAddReverseArcs(other.getSavingsAddReverseArcs());
}
if (other.getSavingsArcCoefficient() != 0D) {
setSavingsArcCoefficient(other.getSavingsArcCoefficient());
}
if (other.getSavingsParallelRoutes() != false) {
setSavingsParallelRoutes(other.getSavingsParallelRoutes());
}
if (other.getCheapestInsertionFarthestSeedsRatio() != 0D) {
setCheapestInsertionFarthestSeedsRatio(other.getCheapestInsertionFarthestSeedsRatio());
}
if (other.getCheapestInsertionFirstSolutionNeighborsRatio() != 0D) {
setCheapestInsertionFirstSolutionNeighborsRatio(other.getCheapestInsertionFirstSolutionNeighborsRatio());
}
if (other.getCheapestInsertionLsOperatorNeighborsRatio() != 0D) {
setCheapestInsertionLsOperatorNeighborsRatio(other.getCheapestInsertionLsOperatorNeighborsRatio());
}
if (other.getChristofidesUseMinimumMatching() != false) {
setChristofidesUseMinimumMatching(other.getChristofidesUseMinimumMatching());
}
if (other.hasLocalSearchOperators()) {
mergeLocalSearchOperators(other.getLocalSearchOperators());
}
if (other.getRelocateExpensiveChainNumArcsToConsider() != 0) {
setRelocateExpensiveChainNumArcsToConsider(other.getRelocateExpensiveChainNumArcsToConsider());
}
if (other.getHeuristicExpensiveChainLnsNumArcsToConsider() != 0) {
setHeuristicExpensiveChainLnsNumArcsToConsider(other.getHeuristicExpensiveChainLnsNumArcsToConsider());
}
if (other.localSearchMetaheuristic_ != 0) {
setLocalSearchMetaheuristicValue(other.getLocalSearchMetaheuristicValue());
}
if (other.getGuidedLocalSearchLambdaCoefficient() != 0D) {
setGuidedLocalSearchLambdaCoefficient(other.getGuidedLocalSearchLambdaCoefficient());
}
if (other.getUseDepthFirstSearch() != false) {
setUseDepthFirstSearch(other.getUseDepthFirstSearch());
}
if (other.useCp_ != 0) {
setUseCpValue(other.getUseCpValue());
}
if (other.useCpSat_ != 0) {
setUseCpSatValue(other.getUseCpSatValue());
}
if (other.continuousSchedulingSolver_ != 0) {
setContinuousSchedulingSolverValue(other.getContinuousSchedulingSolverValue());
}
if (other.mixedIntegerSchedulingSolver_ != 0) {
setMixedIntegerSchedulingSolverValue(other.getMixedIntegerSchedulingSolverValue());
}
if (other.getOptimizationStep() != 0D) {
setOptimizationStep(other.getOptimizationStep());
}
if (other.getNumberOfSolutionsToCollect() != 0) {
setNumberOfSolutionsToCollect(other.getNumberOfSolutionsToCollect());
}
if (other.getSolutionLimit() != 0L) {
setSolutionLimit(other.getSolutionLimit());
}
if (other.hasTimeLimit()) {
mergeTimeLimit(other.getTimeLimit());
}
if (other.hasLnsTimeLimit()) {
mergeLnsTimeLimit(other.getLnsTimeLimit());
}
if (other.getUseFullPropagation() != false) {
setUseFullPropagation(other.getUseFullPropagation());
}
if (other.getLogSearch() != false) {
setLogSearch(other.getLogSearch());
}
if (other.getLogCostScalingFactor() != 0D) {
setLogCostScalingFactor(other.getLogCostScalingFactor());
}
if (other.getLogCostOffset() != 0D) {
setLogCostOffset(other.getLogCostOffset());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.constraintsolver.RoutingSearchParameters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.constraintsolver.RoutingSearchParameters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int firstSolutionStrategy_ = 0;
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The enum numeric value on the wire for firstSolutionStrategy.
*/
@java.lang.Override public int getFirstSolutionStrategyValue() {
return firstSolutionStrategy_;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @param value The enum numeric value on the wire for firstSolutionStrategy to set.
* @return This builder for chaining.
*/
public Builder setFirstSolutionStrategyValue(int value) {
firstSolutionStrategy_ = value;
onChanged();
return this;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The firstSolutionStrategy.
*/
@java.lang.Override
public com.google.ortools.constraintsolver.FirstSolutionStrategy.Value getFirstSolutionStrategy() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.FirstSolutionStrategy.Value result = com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.valueOf(firstSolutionStrategy_);
return result == null ? com.google.ortools.constraintsolver.FirstSolutionStrategy.Value.UNRECOGNIZED : result;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @param value The firstSolutionStrategy to set.
* @return This builder for chaining.
*/
public Builder setFirstSolutionStrategy(com.google.ortools.constraintsolver.FirstSolutionStrategy.Value value) {
if (value == null) {
throw new NullPointerException();
}
firstSolutionStrategy_ = value.getNumber();
onChanged();
return this;
}
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return This builder for chaining.
*/
public Builder clearFirstSolutionStrategy() {
firstSolutionStrategy_ = 0;
onChanged();
return this;
}
private boolean useUnfilteredFirstSolutionStrategy_ ;
/**
*
* --- Advanced first solutions strategy settings ---
* Don't touch these unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_unfiltered_first_solution_strategy = 2;
* @return The useUnfilteredFirstSolutionStrategy.
*/
@java.lang.Override
public boolean getUseUnfilteredFirstSolutionStrategy() {
return useUnfilteredFirstSolutionStrategy_;
}
/**
*
* --- Advanced first solutions strategy settings ---
* Don't touch these unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_unfiltered_first_solution_strategy = 2;
* @param value The useUnfilteredFirstSolutionStrategy to set.
* @return This builder for chaining.
*/
public Builder setUseUnfilteredFirstSolutionStrategy(boolean value) {
useUnfilteredFirstSolutionStrategy_ = value;
onChanged();
return this;
}
/**
*
* --- Advanced first solutions strategy settings ---
* Don't touch these unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_unfiltered_first_solution_strategy = 2;
* @return This builder for chaining.
*/
public Builder clearUseUnfilteredFirstSolutionStrategy() {
useUnfilteredFirstSolutionStrategy_ = false;
onChanged();
return this;
}
private double savingsNeighborsRatio_ ;
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (in ]0, 1]) of neighbors to consider for each node when constructing
* the savings. If unspecified, its value is considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
* @return The savingsNeighborsRatio.
*/
@java.lang.Override
public double getSavingsNeighborsRatio() {
return savingsNeighborsRatio_;
}
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (in ]0, 1]) of neighbors to consider for each node when constructing
* the savings. If unspecified, its value is considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
* @param value The savingsNeighborsRatio to set.
* @return This builder for chaining.
*/
public Builder setSavingsNeighborsRatio(double value) {
savingsNeighborsRatio_ = value;
onChanged();
return this;
}
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (in ]0, 1]) of neighbors to consider for each node when constructing
* the savings. If unspecified, its value is considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
* @return This builder for chaining.
*/
public Builder clearSavingsNeighborsRatio() {
savingsNeighborsRatio_ = 0D;
onChanged();
return this;
}
private double savingsMaxMemoryUsageBytes_ ;
/**
*
* The number of neighbors considered for each node in the Savings heuristic
* is chosen so that the space used to store the savings doesn't exceed
* savings_max_memory_usage_bytes, which must be in ]0, 1e10].
* NOTE: If both savings_neighbors_ratio and savings_max_memory_usage_bytes
* are specified, the number of neighbors considered for each node will be the
* minimum of the two numbers determined by these parameters.
*
*
* double savings_max_memory_usage_bytes = 23;
* @return The savingsMaxMemoryUsageBytes.
*/
@java.lang.Override
public double getSavingsMaxMemoryUsageBytes() {
return savingsMaxMemoryUsageBytes_;
}
/**
*
* The number of neighbors considered for each node in the Savings heuristic
* is chosen so that the space used to store the savings doesn't exceed
* savings_max_memory_usage_bytes, which must be in ]0, 1e10].
* NOTE: If both savings_neighbors_ratio and savings_max_memory_usage_bytes
* are specified, the number of neighbors considered for each node will be the
* minimum of the two numbers determined by these parameters.
*
*
* double savings_max_memory_usage_bytes = 23;
* @param value The savingsMaxMemoryUsageBytes to set.
* @return This builder for chaining.
*/
public Builder setSavingsMaxMemoryUsageBytes(double value) {
savingsMaxMemoryUsageBytes_ = value;
onChanged();
return this;
}
/**
*
* The number of neighbors considered for each node in the Savings heuristic
* is chosen so that the space used to store the savings doesn't exceed
* savings_max_memory_usage_bytes, which must be in ]0, 1e10].
* NOTE: If both savings_neighbors_ratio and savings_max_memory_usage_bytes
* are specified, the number of neighbors considered for each node will be the
* minimum of the two numbers determined by these parameters.
*
*
* double savings_max_memory_usage_bytes = 23;
* @return This builder for chaining.
*/
public Builder clearSavingsMaxMemoryUsageBytes() {
savingsMaxMemoryUsageBytes_ = 0D;
onChanged();
return this;
}
private boolean savingsAddReverseArcs_ ;
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
* @return The savingsAddReverseArcs.
*/
@java.lang.Override
public boolean getSavingsAddReverseArcs() {
return savingsAddReverseArcs_;
}
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
* @param value The savingsAddReverseArcs to set.
* @return This builder for chaining.
*/
public Builder setSavingsAddReverseArcs(boolean value) {
savingsAddReverseArcs_ = value;
onChanged();
return this;
}
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
* @return This builder for chaining.
*/
public Builder clearSavingsAddReverseArcs() {
savingsAddReverseArcs_ = false;
onChanged();
return this;
}
private double savingsArcCoefficient_ ;
/**
*
* Coefficient of the cost of the arc for which the saving value is being
* computed:
* Saving(a-->b) = Cost(a-->end) + Cost(start-->b)
* - savings_arc_coefficient * Cost(a-->b)
* This parameter must be greater than 0, and its default value is 1.
*
*
* double savings_arc_coefficient = 18;
* @return The savingsArcCoefficient.
*/
@java.lang.Override
public double getSavingsArcCoefficient() {
return savingsArcCoefficient_;
}
/**
*
* Coefficient of the cost of the arc for which the saving value is being
* computed:
* Saving(a-->b) = Cost(a-->end) + Cost(start-->b)
* - savings_arc_coefficient * Cost(a-->b)
* This parameter must be greater than 0, and its default value is 1.
*
*
* double savings_arc_coefficient = 18;
* @param value The savingsArcCoefficient to set.
* @return This builder for chaining.
*/
public Builder setSavingsArcCoefficient(double value) {
savingsArcCoefficient_ = value;
onChanged();
return this;
}
/**
*
* Coefficient of the cost of the arc for which the saving value is being
* computed:
* Saving(a-->b) = Cost(a-->end) + Cost(start-->b)
* - savings_arc_coefficient * Cost(a-->b)
* This parameter must be greater than 0, and its default value is 1.
*
*
* double savings_arc_coefficient = 18;
* @return This builder for chaining.
*/
public Builder clearSavingsArcCoefficient() {
savingsArcCoefficient_ = 0D;
onChanged();
return this;
}
private boolean savingsParallelRoutes_ ;
/**
*
* When true, the routes are built in parallel, sequentially otherwise.
*
*
* bool savings_parallel_routes = 19;
* @return The savingsParallelRoutes.
*/
@java.lang.Override
public boolean getSavingsParallelRoutes() {
return savingsParallelRoutes_;
}
/**
*
* When true, the routes are built in parallel, sequentially otherwise.
*
*
* bool savings_parallel_routes = 19;
* @param value The savingsParallelRoutes to set.
* @return This builder for chaining.
*/
public Builder setSavingsParallelRoutes(boolean value) {
savingsParallelRoutes_ = value;
onChanged();
return this;
}
/**
*
* When true, the routes are built in parallel, sequentially otherwise.
*
*
* bool savings_parallel_routes = 19;
* @return This builder for chaining.
*/
public Builder clearSavingsParallelRoutes() {
savingsParallelRoutes_ = false;
onChanged();
return this;
}
private double cheapestInsertionFarthestSeedsRatio_ ;
/**
*
* Ratio (between 0 and 1) of available vehicles in the model on which
* farthest nodes of the model are inserted as seeds in the
* GlobalCheapestInsertion first solution heuristic.
*
*
* double cheapest_insertion_farthest_seeds_ratio = 16;
* @return The cheapestInsertionFarthestSeedsRatio.
*/
@java.lang.Override
public double getCheapestInsertionFarthestSeedsRatio() {
return cheapestInsertionFarthestSeedsRatio_;
}
/**
*
* Ratio (between 0 and 1) of available vehicles in the model on which
* farthest nodes of the model are inserted as seeds in the
* GlobalCheapestInsertion first solution heuristic.
*
*
* double cheapest_insertion_farthest_seeds_ratio = 16;
* @param value The cheapestInsertionFarthestSeedsRatio to set.
* @return This builder for chaining.
*/
public Builder setCheapestInsertionFarthestSeedsRatio(double value) {
cheapestInsertionFarthestSeedsRatio_ = value;
onChanged();
return this;
}
/**
*
* Ratio (between 0 and 1) of available vehicles in the model on which
* farthest nodes of the model are inserted as seeds in the
* GlobalCheapestInsertion first solution heuristic.
*
*
* double cheapest_insertion_farthest_seeds_ratio = 16;
* @return This builder for chaining.
*/
public Builder clearCheapestInsertionFarthestSeedsRatio() {
cheapestInsertionFarthestSeedsRatio_ = 0D;
onChanged();
return this;
}
private double cheapestInsertionFirstSolutionNeighborsRatio_ ;
/**
*
* Ratio (in ]0, 1]) of neighbors to consider for each node when creating
* new insertions in the parallel/sequential cheapest insertion heuristic.
* If not overridden, its default value is 1, meaning all neighbors will be
* considered.
* Neighbors ratio for the first solution heuristic.
*
*
* double cheapest_insertion_first_solution_neighbors_ratio = 21;
* @return The cheapestInsertionFirstSolutionNeighborsRatio.
*/
@java.lang.Override
public double getCheapestInsertionFirstSolutionNeighborsRatio() {
return cheapestInsertionFirstSolutionNeighborsRatio_;
}
/**
*
* Ratio (in ]0, 1]) of neighbors to consider for each node when creating
* new insertions in the parallel/sequential cheapest insertion heuristic.
* If not overridden, its default value is 1, meaning all neighbors will be
* considered.
* Neighbors ratio for the first solution heuristic.
*
*
* double cheapest_insertion_first_solution_neighbors_ratio = 21;
* @param value The cheapestInsertionFirstSolutionNeighborsRatio to set.
* @return This builder for chaining.
*/
public Builder setCheapestInsertionFirstSolutionNeighborsRatio(double value) {
cheapestInsertionFirstSolutionNeighborsRatio_ = value;
onChanged();
return this;
}
/**
*
* Ratio (in ]0, 1]) of neighbors to consider for each node when creating
* new insertions in the parallel/sequential cheapest insertion heuristic.
* If not overridden, its default value is 1, meaning all neighbors will be
* considered.
* Neighbors ratio for the first solution heuristic.
*
*
* double cheapest_insertion_first_solution_neighbors_ratio = 21;
* @return This builder for chaining.
*/
public Builder clearCheapestInsertionFirstSolutionNeighborsRatio() {
cheapestInsertionFirstSolutionNeighborsRatio_ = 0D;
onChanged();
return this;
}
private double cheapestInsertionLsOperatorNeighborsRatio_ ;
/**
*
* Neighbors ratio for the heuristic when used in a local search operator (see
* local_search_operators.use_global_cheapest_insertion_path_lns and
* local_search_operators.use_global_cheapest_insertion_chain_lns below).
*
*
* double cheapest_insertion_ls_operator_neighbors_ratio = 31;
* @return The cheapestInsertionLsOperatorNeighborsRatio.
*/
@java.lang.Override
public double getCheapestInsertionLsOperatorNeighborsRatio() {
return cheapestInsertionLsOperatorNeighborsRatio_;
}
/**
*
* Neighbors ratio for the heuristic when used in a local search operator (see
* local_search_operators.use_global_cheapest_insertion_path_lns and
* local_search_operators.use_global_cheapest_insertion_chain_lns below).
*
*
* double cheapest_insertion_ls_operator_neighbors_ratio = 31;
* @param value The cheapestInsertionLsOperatorNeighborsRatio to set.
* @return This builder for chaining.
*/
public Builder setCheapestInsertionLsOperatorNeighborsRatio(double value) {
cheapestInsertionLsOperatorNeighborsRatio_ = value;
onChanged();
return this;
}
/**
*
* Neighbors ratio for the heuristic when used in a local search operator (see
* local_search_operators.use_global_cheapest_insertion_path_lns and
* local_search_operators.use_global_cheapest_insertion_chain_lns below).
*
*
* double cheapest_insertion_ls_operator_neighbors_ratio = 31;
* @return This builder for chaining.
*/
public Builder clearCheapestInsertionLsOperatorNeighborsRatio() {
cheapestInsertionLsOperatorNeighborsRatio_ = 0D;
onChanged();
return this;
}
private boolean christofidesUseMinimumMatching_ ;
/**
*
* If true use minimum matching instead of minimal matching in the
* Christofides algorithm.
*
*
* bool christofides_use_minimum_matching = 30;
* @return The christofidesUseMinimumMatching.
*/
@java.lang.Override
public boolean getChristofidesUseMinimumMatching() {
return christofidesUseMinimumMatching_;
}
/**
*
* If true use minimum matching instead of minimal matching in the
* Christofides algorithm.
*
*
* bool christofides_use_minimum_matching = 30;
* @param value The christofidesUseMinimumMatching to set.
* @return This builder for chaining.
*/
public Builder setChristofidesUseMinimumMatching(boolean value) {
christofidesUseMinimumMatching_ = value;
onChanged();
return this;
}
/**
*
* If true use minimum matching instead of minimal matching in the
* Christofides algorithm.
*
*
* bool christofides_use_minimum_matching = 30;
* @return This builder for chaining.
*/
public Builder clearChristofidesUseMinimumMatching() {
christofidesUseMinimumMatching_ = false;
onChanged();
return this;
}
private com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators localSearchOperators_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder> localSearchOperatorsBuilder_;
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return Whether the localSearchOperators field is set.
*/
public boolean hasLocalSearchOperators() {
return localSearchOperatorsBuilder_ != null || localSearchOperators_ != null;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return The localSearchOperators.
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getLocalSearchOperators() {
if (localSearchOperatorsBuilder_ == null) {
return localSearchOperators_ == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
} else {
return localSearchOperatorsBuilder_.getMessage();
}
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder setLocalSearchOperators(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators value) {
if (localSearchOperatorsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
localSearchOperators_ = value;
onChanged();
} else {
localSearchOperatorsBuilder_.setMessage(value);
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder setLocalSearchOperators(
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder builderForValue) {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = builderForValue.build();
onChanged();
} else {
localSearchOperatorsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder mergeLocalSearchOperators(com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators value) {
if (localSearchOperatorsBuilder_ == null) {
if (localSearchOperators_ != null) {
localSearchOperators_ =
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.newBuilder(localSearchOperators_).mergeFrom(value).buildPartial();
} else {
localSearchOperators_ = value;
}
onChanged();
} else {
localSearchOperatorsBuilder_.mergeFrom(value);
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public Builder clearLocalSearchOperators() {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperators_ = null;
onChanged();
} else {
localSearchOperators_ = null;
localSearchOperatorsBuilder_ = null;
}
return this;
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder getLocalSearchOperatorsBuilder() {
onChanged();
return getLocalSearchOperatorsFieldBuilder().getBuilder();
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
public com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder getLocalSearchOperatorsOrBuilder() {
if (localSearchOperatorsBuilder_ != null) {
return localSearchOperatorsBuilder_.getMessageOrBuilder();
} else {
return localSearchOperators_ == null ?
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.getDefaultInstance() : localSearchOperators_;
}
}
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder>
getLocalSearchOperatorsFieldBuilder() {
if (localSearchOperatorsBuilder_ == null) {
localSearchOperatorsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators.Builder, com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder>(
getLocalSearchOperators(),
getParentForChildren(),
isClean());
localSearchOperators_ = null;
}
return localSearchOperatorsBuilder_;
}
private int relocateExpensiveChainNumArcsToConsider_ ;
/**
*
* Number of expensive arcs to consider cutting in the RelocateExpensiveChain
* neighborhood operator (see
* LocalSearchNeighborhoodOperators.use_relocate_expensive_chain()).
* This parameter must be greater than 2.
* NOTE(user): The number of neighbors generated by the operator for
* relocate_expensive_chain_num_arcs_to_consider = K is around
* K*(K-1)/2 * number_of_routes * number_of_nodes.
*
*
* int32 relocate_expensive_chain_num_arcs_to_consider = 20;
* @return The relocateExpensiveChainNumArcsToConsider.
*/
@java.lang.Override
public int getRelocateExpensiveChainNumArcsToConsider() {
return relocateExpensiveChainNumArcsToConsider_;
}
/**
*
* Number of expensive arcs to consider cutting in the RelocateExpensiveChain
* neighborhood operator (see
* LocalSearchNeighborhoodOperators.use_relocate_expensive_chain()).
* This parameter must be greater than 2.
* NOTE(user): The number of neighbors generated by the operator for
* relocate_expensive_chain_num_arcs_to_consider = K is around
* K*(K-1)/2 * number_of_routes * number_of_nodes.
*
*
* int32 relocate_expensive_chain_num_arcs_to_consider = 20;
* @param value The relocateExpensiveChainNumArcsToConsider to set.
* @return This builder for chaining.
*/
public Builder setRelocateExpensiveChainNumArcsToConsider(int value) {
relocateExpensiveChainNumArcsToConsider_ = value;
onChanged();
return this;
}
/**
*
* Number of expensive arcs to consider cutting in the RelocateExpensiveChain
* neighborhood operator (see
* LocalSearchNeighborhoodOperators.use_relocate_expensive_chain()).
* This parameter must be greater than 2.
* NOTE(user): The number of neighbors generated by the operator for
* relocate_expensive_chain_num_arcs_to_consider = K is around
* K*(K-1)/2 * number_of_routes * number_of_nodes.
*
*
* int32 relocate_expensive_chain_num_arcs_to_consider = 20;
* @return This builder for chaining.
*/
public Builder clearRelocateExpensiveChainNumArcsToConsider() {
relocateExpensiveChainNumArcsToConsider_ = 0;
onChanged();
return this;
}
private int heuristicExpensiveChainLnsNumArcsToConsider_ ;
/**
*
* Number of expensive arcs to consider cutting in the
* FilteredHeuristicExpensiveChainLNSOperator operator.
*
*
* int32 heuristic_expensive_chain_lns_num_arcs_to_consider = 32;
* @return The heuristicExpensiveChainLnsNumArcsToConsider.
*/
@java.lang.Override
public int getHeuristicExpensiveChainLnsNumArcsToConsider() {
return heuristicExpensiveChainLnsNumArcsToConsider_;
}
/**
*
* Number of expensive arcs to consider cutting in the
* FilteredHeuristicExpensiveChainLNSOperator operator.
*
*
* int32 heuristic_expensive_chain_lns_num_arcs_to_consider = 32;
* @param value The heuristicExpensiveChainLnsNumArcsToConsider to set.
* @return This builder for chaining.
*/
public Builder setHeuristicExpensiveChainLnsNumArcsToConsider(int value) {
heuristicExpensiveChainLnsNumArcsToConsider_ = value;
onChanged();
return this;
}
/**
*
* Number of expensive arcs to consider cutting in the
* FilteredHeuristicExpensiveChainLNSOperator operator.
*
*
* int32 heuristic_expensive_chain_lns_num_arcs_to_consider = 32;
* @return This builder for chaining.
*/
public Builder clearHeuristicExpensiveChainLnsNumArcsToConsider() {
heuristicExpensiveChainLnsNumArcsToConsider_ = 0;
onChanged();
return this;
}
private int localSearchMetaheuristic_ = 0;
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The enum numeric value on the wire for localSearchMetaheuristic.
*/
@java.lang.Override public int getLocalSearchMetaheuristicValue() {
return localSearchMetaheuristic_;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @param value The enum numeric value on the wire for localSearchMetaheuristic to set.
* @return This builder for chaining.
*/
public Builder setLocalSearchMetaheuristicValue(int value) {
localSearchMetaheuristic_ = value;
onChanged();
return this;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The localSearchMetaheuristic.
*/
@java.lang.Override
public com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value getLocalSearchMetaheuristic() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value result = com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.valueOf(localSearchMetaheuristic_);
return result == null ? com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value.UNRECOGNIZED : result;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @param value The localSearchMetaheuristic to set.
* @return This builder for chaining.
*/
public Builder setLocalSearchMetaheuristic(com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value value) {
if (value == null) {
throw new NullPointerException();
}
localSearchMetaheuristic_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return This builder for chaining.
*/
public Builder clearLocalSearchMetaheuristic() {
localSearchMetaheuristic_ = 0;
onChanged();
return this;
}
private double guidedLocalSearchLambdaCoefficient_ ;
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used. Must be positive.
*
*
* double guided_local_search_lambda_coefficient = 5;
* @return The guidedLocalSearchLambdaCoefficient.
*/
@java.lang.Override
public double getGuidedLocalSearchLambdaCoefficient() {
return guidedLocalSearchLambdaCoefficient_;
}
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used. Must be positive.
*
*
* double guided_local_search_lambda_coefficient = 5;
* @param value The guidedLocalSearchLambdaCoefficient to set.
* @return This builder for chaining.
*/
public Builder setGuidedLocalSearchLambdaCoefficient(double value) {
guidedLocalSearchLambdaCoefficient_ = value;
onChanged();
return this;
}
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used. Must be positive.
*
*
* double guided_local_search_lambda_coefficient = 5;
* @return This builder for chaining.
*/
public Builder clearGuidedLocalSearchLambdaCoefficient() {
guidedLocalSearchLambdaCoefficient_ = 0D;
onChanged();
return this;
}
private boolean useDepthFirstSearch_ ;
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
* @return The useDepthFirstSearch.
*/
@java.lang.Override
public boolean getUseDepthFirstSearch() {
return useDepthFirstSearch_;
}
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
* @param value The useDepthFirstSearch to set.
* @return This builder for chaining.
*/
public Builder setUseDepthFirstSearch(boolean value) {
useDepthFirstSearch_ = value;
onChanged();
return this;
}
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
* @return This builder for chaining.
*/
public Builder clearUseDepthFirstSearch() {
useDepthFirstSearch_ = false;
onChanged();
return this;
}
private int useCp_ = 0;
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The enum numeric value on the wire for useCp.
*/
@java.lang.Override public int getUseCpValue() {
return useCp_;
}
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @param value The enum numeric value on the wire for useCp to set.
* @return This builder for chaining.
*/
public Builder setUseCpValue(int value) {
useCp_ = value;
onChanged();
return this;
}
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The useCp.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseCp() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCp_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @param value The useCp to set.
* @return This builder for chaining.
*/
public Builder setUseCp(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useCp_ = value.getNumber();
onChanged();
return this;
}
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return This builder for chaining.
*/
public Builder clearUseCp() {
useCp_ = 0;
onChanged();
return this;
}
private int useCpSat_ = 0;
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The enum numeric value on the wire for useCpSat.
*/
@java.lang.Override public int getUseCpSatValue() {
return useCpSat_;
}
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @param value The enum numeric value on the wire for useCpSat to set.
* @return This builder for chaining.
*/
public Builder setUseCpSatValue(int value) {
useCpSat_ = value;
onChanged();
return this;
}
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The useCpSat.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getUseCpSat() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(useCpSat_);
return result == null ? com.google.ortools.util.OptionalBoolean.UNRECOGNIZED : result;
}
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @param value The useCpSat to set.
* @return This builder for chaining.
*/
public Builder setUseCpSat(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
useCpSat_ = value.getNumber();
onChanged();
return this;
}
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return This builder for chaining.
*/
public Builder clearUseCpSat() {
useCpSat_ = 0;
onChanged();
return this;
}
private int continuousSchedulingSolver_ = 0;
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The enum numeric value on the wire for continuousSchedulingSolver.
*/
@java.lang.Override public int getContinuousSchedulingSolverValue() {
return continuousSchedulingSolver_;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @param value The enum numeric value on the wire for continuousSchedulingSolver to set.
* @return This builder for chaining.
*/
public Builder setContinuousSchedulingSolverValue(int value) {
continuousSchedulingSolver_ = value;
onChanged();
return this;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The continuousSchedulingSolver.
*/
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getContinuousSchedulingSolver() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver result = com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.valueOf(continuousSchedulingSolver_);
return result == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNRECOGNIZED : result;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @param value The continuousSchedulingSolver to set.
* @return This builder for chaining.
*/
public Builder setContinuousSchedulingSolver(com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver value) {
if (value == null) {
throw new NullPointerException();
}
continuousSchedulingSolver_ = value.getNumber();
onChanged();
return this;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return This builder for chaining.
*/
public Builder clearContinuousSchedulingSolver() {
continuousSchedulingSolver_ = 0;
onChanged();
return this;
}
private int mixedIntegerSchedulingSolver_ = 0;
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The enum numeric value on the wire for mixedIntegerSchedulingSolver.
*/
@java.lang.Override public int getMixedIntegerSchedulingSolverValue() {
return mixedIntegerSchedulingSolver_;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @param value The enum numeric value on the wire for mixedIntegerSchedulingSolver to set.
* @return This builder for chaining.
*/
public Builder setMixedIntegerSchedulingSolverValue(int value) {
mixedIntegerSchedulingSolver_ = value;
onChanged();
return this;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The mixedIntegerSchedulingSolver.
*/
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getMixedIntegerSchedulingSolver() {
@SuppressWarnings("deprecation")
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver result = com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.valueOf(mixedIntegerSchedulingSolver_);
return result == null ? com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver.UNRECOGNIZED : result;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @param value The mixedIntegerSchedulingSolver to set.
* @return This builder for chaining.
*/
public Builder setMixedIntegerSchedulingSolver(com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver value) {
if (value == null) {
throw new NullPointerException();
}
mixedIntegerSchedulingSolver_ = value.getNumber();
onChanged();
return this;
}
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return This builder for chaining.
*/
public Builder clearMixedIntegerSchedulingSolver() {
mixedIntegerSchedulingSolver_ = 0;
onChanged();
return this;
}
private double optimizationStep_ ;
/**
*
* Minimum step by which the solution must be improved in local search. 0
* means "unspecified". If this value is fractional, it will get rounded to
* the nearest integer.
*
*
* double optimization_step = 7;
* @return The optimizationStep.
*/
@java.lang.Override
public double getOptimizationStep() {
return optimizationStep_;
}
/**
*
* Minimum step by which the solution must be improved in local search. 0
* means "unspecified". If this value is fractional, it will get rounded to
* the nearest integer.
*
*
* double optimization_step = 7;
* @param value The optimizationStep to set.
* @return This builder for chaining.
*/
public Builder setOptimizationStep(double value) {
optimizationStep_ = value;
onChanged();
return this;
}
/**
*
* Minimum step by which the solution must be improved in local search. 0
* means "unspecified". If this value is fractional, it will get rounded to
* the nearest integer.
*
*
* double optimization_step = 7;
* @return This builder for chaining.
*/
public Builder clearOptimizationStep() {
optimizationStep_ = 0D;
onChanged();
return this;
}
private int numberOfSolutionsToCollect_ ;
/**
*
* Number of solutions to collect during the search. Corresponds to the best
* solutions found during the search. 0 means "unspecified".
*
*
* int32 number_of_solutions_to_collect = 17;
* @return The numberOfSolutionsToCollect.
*/
@java.lang.Override
public int getNumberOfSolutionsToCollect() {
return numberOfSolutionsToCollect_;
}
/**
*
* Number of solutions to collect during the search. Corresponds to the best
* solutions found during the search. 0 means "unspecified".
*
*
* int32 number_of_solutions_to_collect = 17;
* @param value The numberOfSolutionsToCollect to set.
* @return This builder for chaining.
*/
public Builder setNumberOfSolutionsToCollect(int value) {
numberOfSolutionsToCollect_ = value;
onChanged();
return this;
}
/**
*
* Number of solutions to collect during the search. Corresponds to the best
* solutions found during the search. 0 means "unspecified".
*
*
* int32 number_of_solutions_to_collect = 17;
* @return This builder for chaining.
*/
public Builder clearNumberOfSolutionsToCollect() {
numberOfSolutionsToCollect_ = 0;
onChanged();
return this;
}
private long solutionLimit_ ;
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search. 0 means
* "unspecified".
*
*
* int64 solution_limit = 8;
* @return The solutionLimit.
*/
@java.lang.Override
public long getSolutionLimit() {
return solutionLimit_;
}
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search. 0 means
* "unspecified".
*
*
* int64 solution_limit = 8;
* @param value The solutionLimit to set.
* @return This builder for chaining.
*/
public Builder setSolutionLimit(long value) {
solutionLimit_ = value;
onChanged();
return this;
}
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search. 0 means
* "unspecified".
*
*
* int64 solution_limit = 8;
* @return This builder for chaining.
*/
public Builder clearSolutionLimit() {
solutionLimit_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.Duration timeLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> timeLimitBuilder_;
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return Whether the timeLimit field is set.
*/
public boolean hasTimeLimit() {
return timeLimitBuilder_ != null || timeLimit_ != null;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return The timeLimit.
*/
public com.google.protobuf.Duration getTimeLimit() {
if (timeLimitBuilder_ == null) {
return timeLimit_ == null ? com.google.protobuf.Duration.getDefaultInstance() : timeLimit_;
} else {
return timeLimitBuilder_.getMessage();
}
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public Builder setTimeLimit(com.google.protobuf.Duration value) {
if (timeLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
timeLimit_ = value;
onChanged();
} else {
timeLimitBuilder_.setMessage(value);
}
return this;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public Builder setTimeLimit(
com.google.protobuf.Duration.Builder builderForValue) {
if (timeLimitBuilder_ == null) {
timeLimit_ = builderForValue.build();
onChanged();
} else {
timeLimitBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public Builder mergeTimeLimit(com.google.protobuf.Duration value) {
if (timeLimitBuilder_ == null) {
if (timeLimit_ != null) {
timeLimit_ =
com.google.protobuf.Duration.newBuilder(timeLimit_).mergeFrom(value).buildPartial();
} else {
timeLimit_ = value;
}
onChanged();
} else {
timeLimitBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public Builder clearTimeLimit() {
if (timeLimitBuilder_ == null) {
timeLimit_ = null;
onChanged();
} else {
timeLimit_ = null;
timeLimitBuilder_ = null;
}
return this;
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public com.google.protobuf.Duration.Builder getTimeLimitBuilder() {
onChanged();
return getTimeLimitFieldBuilder().getBuilder();
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
public com.google.protobuf.DurationOrBuilder getTimeLimitOrBuilder() {
if (timeLimitBuilder_ != null) {
return timeLimitBuilder_.getMessageOrBuilder();
} else {
return timeLimit_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : timeLimit_;
}
}
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getTimeLimitFieldBuilder() {
if (timeLimitBuilder_ == null) {
timeLimitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getTimeLimit(),
getParentForChildren(),
isClean());
timeLimit_ = null;
}
return timeLimitBuilder_;
}
private com.google.protobuf.Duration lnsTimeLimit_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder> lnsTimeLimitBuilder_;
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return Whether the lnsTimeLimit field is set.
*/
public boolean hasLnsTimeLimit() {
return lnsTimeLimitBuilder_ != null || lnsTimeLimit_ != null;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return The lnsTimeLimit.
*/
public com.google.protobuf.Duration getLnsTimeLimit() {
if (lnsTimeLimitBuilder_ == null) {
return lnsTimeLimit_ == null ? com.google.protobuf.Duration.getDefaultInstance() : lnsTimeLimit_;
} else {
return lnsTimeLimitBuilder_.getMessage();
}
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public Builder setLnsTimeLimit(com.google.protobuf.Duration value) {
if (lnsTimeLimitBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
lnsTimeLimit_ = value;
onChanged();
} else {
lnsTimeLimitBuilder_.setMessage(value);
}
return this;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public Builder setLnsTimeLimit(
com.google.protobuf.Duration.Builder builderForValue) {
if (lnsTimeLimitBuilder_ == null) {
lnsTimeLimit_ = builderForValue.build();
onChanged();
} else {
lnsTimeLimitBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public Builder mergeLnsTimeLimit(com.google.protobuf.Duration value) {
if (lnsTimeLimitBuilder_ == null) {
if (lnsTimeLimit_ != null) {
lnsTimeLimit_ =
com.google.protobuf.Duration.newBuilder(lnsTimeLimit_).mergeFrom(value).buildPartial();
} else {
lnsTimeLimit_ = value;
}
onChanged();
} else {
lnsTimeLimitBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public Builder clearLnsTimeLimit() {
if (lnsTimeLimitBuilder_ == null) {
lnsTimeLimit_ = null;
onChanged();
} else {
lnsTimeLimit_ = null;
lnsTimeLimitBuilder_ = null;
}
return this;
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public com.google.protobuf.Duration.Builder getLnsTimeLimitBuilder() {
onChanged();
return getLnsTimeLimitFieldBuilder().getBuilder();
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
public com.google.protobuf.DurationOrBuilder getLnsTimeLimitOrBuilder() {
if (lnsTimeLimitBuilder_ != null) {
return lnsTimeLimitBuilder_.getMessageOrBuilder();
} else {
return lnsTimeLimit_ == null ?
com.google.protobuf.Duration.getDefaultInstance() : lnsTimeLimit_;
}
}
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>
getLnsTimeLimitFieldBuilder() {
if (lnsTimeLimitBuilder_ == null) {
lnsTimeLimitBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.protobuf.Duration, com.google.protobuf.Duration.Builder, com.google.protobuf.DurationOrBuilder>(
getLnsTimeLimit(),
getParentForChildren(),
isClean());
lnsTimeLimit_ = null;
}
return lnsTimeLimitBuilder_;
}
private boolean useFullPropagation_ ;
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with full propagation in routing model (instead of 'light'
* propagation only). Full propagation is only necessary when using
* depth-first search or for models which require strong propagation to
* finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_full_propagation = 11;
* @return The useFullPropagation.
*/
@java.lang.Override
public boolean getUseFullPropagation() {
return useFullPropagation_;
}
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with full propagation in routing model (instead of 'light'
* propagation only). Full propagation is only necessary when using
* depth-first search or for models which require strong propagation to
* finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_full_propagation = 11;
* @param value The useFullPropagation to set.
* @return This builder for chaining.
*/
public Builder setUseFullPropagation(boolean value) {
useFullPropagation_ = value;
onChanged();
return this;
}
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with full propagation in routing model (instead of 'light'
* propagation only). Full propagation is only necessary when using
* depth-first search or for models which require strong propagation to
* finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_full_propagation = 11;
* @return This builder for chaining.
*/
public Builder clearUseFullPropagation() {
useFullPropagation_ = false;
onChanged();
return this;
}
private boolean logSearch_ ;
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
* @return The logSearch.
*/
@java.lang.Override
public boolean getLogSearch() {
return logSearch_;
}
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
* @param value The logSearch to set.
* @return This builder for chaining.
*/
public Builder setLogSearch(boolean value) {
logSearch_ = value;
onChanged();
return this;
}
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
* @return This builder for chaining.
*/
public Builder clearLogSearch() {
logSearch_ = false;
onChanged();
return this;
}
private double logCostScalingFactor_ ;
/**
*
* In logs, cost values will be scaled and offset by the given values in the
* following way: log_cost_scaling_factor * (cost + log_cost_offset)
*
*
* double log_cost_scaling_factor = 22;
* @return The logCostScalingFactor.
*/
@java.lang.Override
public double getLogCostScalingFactor() {
return logCostScalingFactor_;
}
/**
*
* In logs, cost values will be scaled and offset by the given values in the
* following way: log_cost_scaling_factor * (cost + log_cost_offset)
*
*
* double log_cost_scaling_factor = 22;
* @param value The logCostScalingFactor to set.
* @return This builder for chaining.
*/
public Builder setLogCostScalingFactor(double value) {
logCostScalingFactor_ = value;
onChanged();
return this;
}
/**
*
* In logs, cost values will be scaled and offset by the given values in the
* following way: log_cost_scaling_factor * (cost + log_cost_offset)
*
*
* double log_cost_scaling_factor = 22;
* @return This builder for chaining.
*/
public Builder clearLogCostScalingFactor() {
logCostScalingFactor_ = 0D;
onChanged();
return this;
}
private double logCostOffset_ ;
/**
* double log_cost_offset = 29;
* @return The logCostOffset.
*/
@java.lang.Override
public double getLogCostOffset() {
return logCostOffset_;
}
/**
* double log_cost_offset = 29;
* @param value The logCostOffset to set.
* @return This builder for chaining.
*/
public Builder setLogCostOffset(double value) {
logCostOffset_ = value;
onChanged();
return this;
}
/**
* double log_cost_offset = 29;
* @return This builder for chaining.
*/
public Builder clearLogCostOffset() {
logCostOffset_ = 0D;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.RoutingSearchParameters)
}
// @@protoc_insertion_point(class_scope:operations_research.RoutingSearchParameters)
private static final com.google.ortools.constraintsolver.RoutingSearchParameters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.constraintsolver.RoutingSearchParameters();
}
public static com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RoutingSearchParameters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RoutingSearchParameters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ortools.constraintsolver.RoutingSearchParameters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy