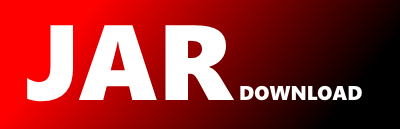
com.google.ortools.constraintsolver.RoutingSearchParametersOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/constraint_solver/routing_parameters.proto
package com.google.ortools.constraintsolver;
public interface RoutingSearchParametersOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.RoutingSearchParameters)
com.google.protobuf.MessageOrBuilder {
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The enum numeric value on the wire for firstSolutionStrategy.
*/
int getFirstSolutionStrategyValue();
/**
*
* First solution strategies, used as starting point of local search.
*
*
* .operations_research.FirstSolutionStrategy.Value first_solution_strategy = 1;
* @return The firstSolutionStrategy.
*/
com.google.ortools.constraintsolver.FirstSolutionStrategy.Value getFirstSolutionStrategy();
/**
*
* --- Advanced first solutions strategy settings ---
* Don't touch these unless you know what you are doing.
* Use filtered version of first solution strategy if available.
*
*
* bool use_unfiltered_first_solution_strategy = 2;
* @return The useUnfilteredFirstSolutionStrategy.
*/
boolean getUseUnfilteredFirstSolutionStrategy();
/**
*
* Parameters specific to the Savings first solution heuristic.
* Ratio (in ]0, 1]) of neighbors to consider for each node when constructing
* the savings. If unspecified, its value is considered to be 1.0.
*
*
* double savings_neighbors_ratio = 14;
* @return The savingsNeighborsRatio.
*/
double getSavingsNeighborsRatio();
/**
*
* The number of neighbors considered for each node in the Savings heuristic
* is chosen so that the space used to store the savings doesn't exceed
* savings_max_memory_usage_bytes, which must be in ]0, 1e10].
* NOTE: If both savings_neighbors_ratio and savings_max_memory_usage_bytes
* are specified, the number of neighbors considered for each node will be the
* minimum of the two numbers determined by these parameters.
*
*
* double savings_max_memory_usage_bytes = 23;
* @return The savingsMaxMemoryUsageBytes.
*/
double getSavingsMaxMemoryUsageBytes();
/**
*
* Add savings related to reverse arcs when finding the nearest neighbors
* of the nodes.
*
*
* bool savings_add_reverse_arcs = 15;
* @return The savingsAddReverseArcs.
*/
boolean getSavingsAddReverseArcs();
/**
*
* Coefficient of the cost of the arc for which the saving value is being
* computed:
* Saving(a-->b) = Cost(a-->end) + Cost(start-->b)
* - savings_arc_coefficient * Cost(a-->b)
* This parameter must be greater than 0, and its default value is 1.
*
*
* double savings_arc_coefficient = 18;
* @return The savingsArcCoefficient.
*/
double getSavingsArcCoefficient();
/**
*
* When true, the routes are built in parallel, sequentially otherwise.
*
*
* bool savings_parallel_routes = 19;
* @return The savingsParallelRoutes.
*/
boolean getSavingsParallelRoutes();
/**
*
* Ratio (between 0 and 1) of available vehicles in the model on which
* farthest nodes of the model are inserted as seeds in the
* GlobalCheapestInsertion first solution heuristic.
*
*
* double cheapest_insertion_farthest_seeds_ratio = 16;
* @return The cheapestInsertionFarthestSeedsRatio.
*/
double getCheapestInsertionFarthestSeedsRatio();
/**
*
* Ratio (in ]0, 1]) of neighbors to consider for each node when creating
* new insertions in the parallel/sequential cheapest insertion heuristic.
* If not overridden, its default value is 1, meaning all neighbors will be
* considered.
* Neighbors ratio for the first solution heuristic.
*
*
* double cheapest_insertion_first_solution_neighbors_ratio = 21;
* @return The cheapestInsertionFirstSolutionNeighborsRatio.
*/
double getCheapestInsertionFirstSolutionNeighborsRatio();
/**
*
* Neighbors ratio for the heuristic when used in a local search operator (see
* local_search_operators.use_global_cheapest_insertion_path_lns and
* local_search_operators.use_global_cheapest_insertion_chain_lns below).
*
*
* double cheapest_insertion_ls_operator_neighbors_ratio = 31;
* @return The cheapestInsertionLsOperatorNeighborsRatio.
*/
double getCheapestInsertionLsOperatorNeighborsRatio();
/**
*
* If true use minimum matching instead of minimal matching in the
* Christofides algorithm.
*
*
* bool christofides_use_minimum_matching = 30;
* @return The christofidesUseMinimumMatching.
*/
boolean getChristofidesUseMinimumMatching();
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return Whether the localSearchOperators field is set.
*/
boolean hasLocalSearchOperators();
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
* @return The localSearchOperators.
*/
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperators getLocalSearchOperators();
/**
* .operations_research.RoutingSearchParameters.LocalSearchNeighborhoodOperators local_search_operators = 3;
*/
com.google.ortools.constraintsolver.RoutingSearchParameters.LocalSearchNeighborhoodOperatorsOrBuilder getLocalSearchOperatorsOrBuilder();
/**
*
* Number of expensive arcs to consider cutting in the RelocateExpensiveChain
* neighborhood operator (see
* LocalSearchNeighborhoodOperators.use_relocate_expensive_chain()).
* This parameter must be greater than 2.
* NOTE(user): The number of neighbors generated by the operator for
* relocate_expensive_chain_num_arcs_to_consider = K is around
* K*(K-1)/2 * number_of_routes * number_of_nodes.
*
*
* int32 relocate_expensive_chain_num_arcs_to_consider = 20;
* @return The relocateExpensiveChainNumArcsToConsider.
*/
int getRelocateExpensiveChainNumArcsToConsider();
/**
*
* Number of expensive arcs to consider cutting in the
* FilteredHeuristicExpensiveChainLNSOperator operator.
*
*
* int32 heuristic_expensive_chain_lns_num_arcs_to_consider = 32;
* @return The heuristicExpensiveChainLnsNumArcsToConsider.
*/
int getHeuristicExpensiveChainLnsNumArcsToConsider();
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The enum numeric value on the wire for localSearchMetaheuristic.
*/
int getLocalSearchMetaheuristicValue();
/**
*
* Local search metaheuristics used to guide the search.
*
*
* .operations_research.LocalSearchMetaheuristic.Value local_search_metaheuristic = 4;
* @return The localSearchMetaheuristic.
*/
com.google.ortools.constraintsolver.LocalSearchMetaheuristic.Value getLocalSearchMetaheuristic();
/**
*
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Lambda coefficient used to penalize arc costs when GUIDED_LOCAL_SEARCH is
* used. Must be positive.
*
*
* double guided_local_search_lambda_coefficient = 5;
* @return The guidedLocalSearchLambdaCoefficient.
*/
double getGuidedLocalSearchLambdaCoefficient();
/**
*
* --- Search control ---
* If true, the solver should use depth-first search rather than local search
* to solve the problem.
*
*
* bool use_depth_first_search = 6;
* @return The useDepthFirstSearch.
*/
boolean getUseDepthFirstSearch();
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The enum numeric value on the wire for useCp.
*/
int getUseCpValue();
/**
*
* If true, use the CP solver to find a solution. Either local or depth-first
* search will be used depending on the value of use_depth_first_search. Will
* be run before the CP-SAT solver (cf. use_cp_sat).
*
*
* .operations_research.OptionalBoolean use_cp = 28;
* @return The useCp.
*/
com.google.ortools.util.OptionalBoolean getUseCp();
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The enum numeric value on the wire for useCpSat.
*/
int getUseCpSatValue();
/**
*
* If true, use the CP-SAT solver to find a solution. If use_cp is also true,
* the CP-SAT solver will be run after the CP solver if there is time
* remaining and will use the CP solution as a hint for the CP-SAT search.
* As of 5/2019, only TSP models can be solved.
*
*
* .operations_research.OptionalBoolean use_cp_sat = 27;
* @return The useCpSat.
*/
com.google.ortools.util.OptionalBoolean getUseCpSat();
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The enum numeric value on the wire for continuousSchedulingSolver.
*/
int getContinuousSchedulingSolverValue();
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver continuous_scheduling_solver = 33;
* @return The continuousSchedulingSolver.
*/
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getContinuousSchedulingSolver();
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The enum numeric value on the wire for mixedIntegerSchedulingSolver.
*/
int getMixedIntegerSchedulingSolverValue();
/**
* .operations_research.RoutingSearchParameters.SchedulingSolver mixed_integer_scheduling_solver = 34;
* @return The mixedIntegerSchedulingSolver.
*/
com.google.ortools.constraintsolver.RoutingSearchParameters.SchedulingSolver getMixedIntegerSchedulingSolver();
/**
*
* Minimum step by which the solution must be improved in local search. 0
* means "unspecified". If this value is fractional, it will get rounded to
* the nearest integer.
*
*
* double optimization_step = 7;
* @return The optimizationStep.
*/
double getOptimizationStep();
/**
*
* Number of solutions to collect during the search. Corresponds to the best
* solutions found during the search. 0 means "unspecified".
*
*
* int32 number_of_solutions_to_collect = 17;
* @return The numberOfSolutionsToCollect.
*/
int getNumberOfSolutionsToCollect();
/**
*
* -- Search limits --
* Limit to the number of solutions generated during the search. 0 means
* "unspecified".
*
*
* int64 solution_limit = 8;
* @return The solutionLimit.
*/
long getSolutionLimit();
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return Whether the timeLimit field is set.
*/
boolean hasTimeLimit();
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
* @return The timeLimit.
*/
com.google.protobuf.Duration getTimeLimit();
/**
*
* Limit to the time spent in the search.
*
*
* .google.protobuf.Duration time_limit = 9;
*/
com.google.protobuf.DurationOrBuilder getTimeLimitOrBuilder();
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return Whether the lnsTimeLimit field is set.
*/
boolean hasLnsTimeLimit();
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
* @return The lnsTimeLimit.
*/
com.google.protobuf.Duration getLnsTimeLimit();
/**
*
* Limit to the time spent in the completion search for each local search
* neighbor.
*
*
* .google.protobuf.Duration lns_time_limit = 10;
*/
com.google.protobuf.DurationOrBuilder getLnsTimeLimitOrBuilder();
/**
*
* --- Propagation control ---
* These are advanced settings which should not be modified unless you know
* what you are doing.
* Use constraints with full propagation in routing model (instead of 'light'
* propagation only). Full propagation is only necessary when using
* depth-first search or for models which require strong propagation to
* finalize the value of secondary variables.
* Changing this setting to true will slow down the search in most cases and
* increase memory consumption in all cases.
*
*
* bool use_full_propagation = 11;
* @return The useFullPropagation.
*/
boolean getUseFullPropagation();
/**
*
* --- Miscellaneous ---
* Some of these are advanced settings which should not be modified unless you
* know what you are doing.
* Activates search logging. For each solution found during the search, the
* following will be displayed: its objective value, the maximum objective
* value since the beginning of the search, the elapsed time since the
* beginning of the search, the number of branches explored in the search
* tree, the number of failures in the search tree, the depth of the search
* tree, the number of local search neighbors explored, the number of local
* search neighbors filtered by local search filters, the number of local
* search neighbors accepted, the total memory used and the percentage of the
* search done.
*
*
* bool log_search = 13;
* @return The logSearch.
*/
boolean getLogSearch();
/**
*
* In logs, cost values will be scaled and offset by the given values in the
* following way: log_cost_scaling_factor * (cost + log_cost_offset)
*
*
* double log_cost_scaling_factor = 22;
* @return The logCostScalingFactor.
*/
double getLogCostScalingFactor();
/**
* double log_cost_offset = 29;
* @return The logCostOffset.
*/
double getLogCostOffset();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy