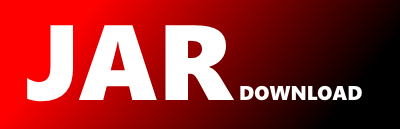
com.google.ortools.linearsolver.MPModelProto Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/linear_solver/linear_solver.proto
package com.google.ortools.linearsolver;
/**
*
* MPModelProto contains all the information for a Linear Programming model.
*
*
* Protobuf type {@code operations_research.MPModelProto}
*/
public final class MPModelProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.MPModelProto)
MPModelProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use MPModelProto.newBuilder() to construct.
private MPModelProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MPModelProto() {
variable_ = java.util.Collections.emptyList();
constraint_ = java.util.Collections.emptyList();
generalConstraint_ = java.util.Collections.emptyList();
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MPModelProto();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MPModelProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
bitField0_ |= 0x00000001;
maximize_ = input.readBool();
break;
}
case 17: {
bitField0_ |= 0x00000002;
objectiveOffset_ = input.readDouble();
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
variable_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
variable_.add(
input.readMessage(com.google.ortools.linearsolver.MPVariableProto.PARSER, extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
constraint_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
constraint_.add(
input.readMessage(com.google.ortools.linearsolver.MPConstraintProto.PARSER, extensionRegistry));
break;
}
case 42: {
com.google.protobuf.ByteString bs = input.readBytes();
bitField0_ |= 0x00000008;
name_ = bs;
break;
}
case 50: {
com.google.ortools.linearsolver.PartialVariableAssignment.Builder subBuilder = null;
if (((bitField0_ & 0x00000010) != 0)) {
subBuilder = solutionHint_.toBuilder();
}
solutionHint_ = input.readMessage(com.google.ortools.linearsolver.PartialVariableAssignment.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(solutionHint_);
solutionHint_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000010;
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
generalConstraint_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
generalConstraint_.add(
input.readMessage(com.google.ortools.linearsolver.MPGeneralConstraintProto.PARSER, extensionRegistry));
break;
}
case 66: {
com.google.ortools.linearsolver.MPQuadraticObjective.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) != 0)) {
subBuilder = quadraticObjective_.toBuilder();
}
quadraticObjective_ = input.readMessage(com.google.ortools.linearsolver.MPQuadraticObjective.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(quadraticObjective_);
quadraticObjective_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
variable_ = java.util.Collections.unmodifiableList(variable_);
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
constraint_ = java.util.Collections.unmodifiableList(constraint_);
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
generalConstraint_ = java.util.Collections.unmodifiableList(generalConstraint_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPModelProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPModelProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.linearsolver.MPModelProto.class, com.google.ortools.linearsolver.MPModelProto.Builder.class);
}
private int bitField0_;
public static final int VARIABLE_FIELD_NUMBER = 3;
private java.util.List variable_;
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
@java.lang.Override
public java.util.List getVariableList() {
return variable_;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
@java.lang.Override
public java.util.List extends com.google.ortools.linearsolver.MPVariableProtoOrBuilder>
getVariableOrBuilderList() {
return variable_;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
@java.lang.Override
public int getVariableCount() {
return variable_.size();
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPVariableProto getVariable(int index) {
return variable_.get(index);
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPVariableProtoOrBuilder getVariableOrBuilder(
int index) {
return variable_.get(index);
}
public static final int CONSTRAINT_FIELD_NUMBER = 4;
private java.util.List constraint_;
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
@java.lang.Override
public java.util.List getConstraintList() {
return constraint_;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
@java.lang.Override
public java.util.List extends com.google.ortools.linearsolver.MPConstraintProtoOrBuilder>
getConstraintOrBuilderList() {
return constraint_;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
@java.lang.Override
public int getConstraintCount() {
return constraint_.size();
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPConstraintProto getConstraint(int index) {
return constraint_.get(index);
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPConstraintProtoOrBuilder getConstraintOrBuilder(
int index) {
return constraint_.get(index);
}
public static final int GENERAL_CONSTRAINT_FIELD_NUMBER = 7;
private java.util.List generalConstraint_;
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
@java.lang.Override
public java.util.List getGeneralConstraintList() {
return generalConstraint_;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
@java.lang.Override
public java.util.List extends com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder>
getGeneralConstraintOrBuilderList() {
return generalConstraint_;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
@java.lang.Override
public int getGeneralConstraintCount() {
return generalConstraint_.size();
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPGeneralConstraintProto getGeneralConstraint(int index) {
return generalConstraint_.get(index);
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder getGeneralConstraintOrBuilder(
int index) {
return generalConstraint_.get(index);
}
public static final int MAXIMIZE_FIELD_NUMBER = 1;
private boolean maximize_;
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @return Whether the maximize field is set.
*/
@java.lang.Override
public boolean hasMaximize() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @return The maximize.
*/
@java.lang.Override
public boolean getMaximize() {
return maximize_;
}
public static final int OBJECTIVE_OFFSET_FIELD_NUMBER = 2;
private double objectiveOffset_;
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @return Whether the objectiveOffset field is set.
*/
@java.lang.Override
public boolean hasObjectiveOffset() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @return The objectiveOffset.
*/
@java.lang.Override
public double getObjectiveOffset() {
return objectiveOffset_;
}
public static final int QUADRATIC_OBJECTIVE_FIELD_NUMBER = 8;
private com.google.ortools.linearsolver.MPQuadraticObjective quadraticObjective_;
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
* @return Whether the quadraticObjective field is set.
*/
@java.lang.Override
public boolean hasQuadraticObjective() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
* @return The quadraticObjective.
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPQuadraticObjective getQuadraticObjective() {
return quadraticObjective_ == null ? com.google.ortools.linearsolver.MPQuadraticObjective.getDefaultInstance() : quadraticObjective_;
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPQuadraticObjectiveOrBuilder getQuadraticObjectiveOrBuilder() {
return quadraticObjective_ == null ? com.google.ortools.linearsolver.MPQuadraticObjective.getDefaultInstance() : quadraticObjective_;
}
public static final int NAME_FIELD_NUMBER = 5;
private volatile java.lang.Object name_;
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return Whether the name field is set.
*/
@java.lang.Override
public boolean hasName() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
}
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SOLUTION_HINT_FIELD_NUMBER = 6;
private com.google.ortools.linearsolver.PartialVariableAssignment solutionHint_;
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
* @return Whether the solutionHint field is set.
*/
@java.lang.Override
public boolean hasSolutionHint() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
* @return The solutionHint.
*/
@java.lang.Override
public com.google.ortools.linearsolver.PartialVariableAssignment getSolutionHint() {
return solutionHint_ == null ? com.google.ortools.linearsolver.PartialVariableAssignment.getDefaultInstance() : solutionHint_;
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
@java.lang.Override
public com.google.ortools.linearsolver.PartialVariableAssignmentOrBuilder getSolutionHintOrBuilder() {
return solutionHint_ == null ? com.google.ortools.linearsolver.PartialVariableAssignment.getDefaultInstance() : solutionHint_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeBool(1, maximize_);
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeDouble(2, objectiveOffset_);
}
for (int i = 0; i < variable_.size(); i++) {
output.writeMessage(3, variable_.get(i));
}
for (int i = 0; i < constraint_.size(); i++) {
output.writeMessage(4, constraint_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, name_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(6, getSolutionHint());
}
for (int i = 0; i < generalConstraint_.size(); i++) {
output.writeMessage(7, generalConstraint_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(8, getQuadraticObjective());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, maximize_);
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(2, objectiveOffset_);
}
for (int i = 0; i < variable_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, variable_.get(i));
}
for (int i = 0; i < constraint_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, constraint_.get(i));
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, name_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getSolutionHint());
}
for (int i = 0; i < generalConstraint_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, generalConstraint_.get(i));
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getQuadraticObjective());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.linearsolver.MPModelProto)) {
return super.equals(obj);
}
com.google.ortools.linearsolver.MPModelProto other = (com.google.ortools.linearsolver.MPModelProto) obj;
if (!getVariableList()
.equals(other.getVariableList())) return false;
if (!getConstraintList()
.equals(other.getConstraintList())) return false;
if (!getGeneralConstraintList()
.equals(other.getGeneralConstraintList())) return false;
if (hasMaximize() != other.hasMaximize()) return false;
if (hasMaximize()) {
if (getMaximize()
!= other.getMaximize()) return false;
}
if (hasObjectiveOffset() != other.hasObjectiveOffset()) return false;
if (hasObjectiveOffset()) {
if (java.lang.Double.doubleToLongBits(getObjectiveOffset())
!= java.lang.Double.doubleToLongBits(
other.getObjectiveOffset())) return false;
}
if (hasQuadraticObjective() != other.hasQuadraticObjective()) return false;
if (hasQuadraticObjective()) {
if (!getQuadraticObjective()
.equals(other.getQuadraticObjective())) return false;
}
if (hasName() != other.hasName()) return false;
if (hasName()) {
if (!getName()
.equals(other.getName())) return false;
}
if (hasSolutionHint() != other.hasSolutionHint()) return false;
if (hasSolutionHint()) {
if (!getSolutionHint()
.equals(other.getSolutionHint())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getVariableCount() > 0) {
hash = (37 * hash) + VARIABLE_FIELD_NUMBER;
hash = (53 * hash) + getVariableList().hashCode();
}
if (getConstraintCount() > 0) {
hash = (37 * hash) + CONSTRAINT_FIELD_NUMBER;
hash = (53 * hash) + getConstraintList().hashCode();
}
if (getGeneralConstraintCount() > 0) {
hash = (37 * hash) + GENERAL_CONSTRAINT_FIELD_NUMBER;
hash = (53 * hash) + getGeneralConstraintList().hashCode();
}
if (hasMaximize()) {
hash = (37 * hash) + MAXIMIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMaximize());
}
if (hasObjectiveOffset()) {
hash = (37 * hash) + OBJECTIVE_OFFSET_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getObjectiveOffset()));
}
if (hasQuadraticObjective()) {
hash = (37 * hash) + QUADRATIC_OBJECTIVE_FIELD_NUMBER;
hash = (53 * hash) + getQuadraticObjective().hashCode();
}
if (hasName()) {
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
}
if (hasSolutionHint()) {
hash = (37 * hash) + SOLUTION_HINT_FIELD_NUMBER;
hash = (53 * hash) + getSolutionHint().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPModelProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPModelProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPModelProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.linearsolver.MPModelProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MPModelProto contains all the information for a Linear Programming model.
*
*
* Protobuf type {@code operations_research.MPModelProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.MPModelProto)
com.google.ortools.linearsolver.MPModelProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPModelProto_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPModelProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.linearsolver.MPModelProto.class, com.google.ortools.linearsolver.MPModelProto.Builder.class);
}
// Construct using com.google.ortools.linearsolver.MPModelProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getVariableFieldBuilder();
getConstraintFieldBuilder();
getGeneralConstraintFieldBuilder();
getQuadraticObjectiveFieldBuilder();
getSolutionHintFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (variableBuilder_ == null) {
variable_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
variableBuilder_.clear();
}
if (constraintBuilder_ == null) {
constraint_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
constraintBuilder_.clear();
}
if (generalConstraintBuilder_ == null) {
generalConstraint_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
generalConstraintBuilder_.clear();
}
maximize_ = false;
bitField0_ = (bitField0_ & ~0x00000008);
objectiveOffset_ = 0D;
bitField0_ = (bitField0_ & ~0x00000010);
if (quadraticObjectiveBuilder_ == null) {
quadraticObjective_ = null;
} else {
quadraticObjectiveBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
name_ = "";
bitField0_ = (bitField0_ & ~0x00000040);
if (solutionHintBuilder_ == null) {
solutionHint_ = null;
} else {
solutionHintBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPModelProto_descriptor;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPModelProto getDefaultInstanceForType() {
return com.google.ortools.linearsolver.MPModelProto.getDefaultInstance();
}
@java.lang.Override
public com.google.ortools.linearsolver.MPModelProto build() {
com.google.ortools.linearsolver.MPModelProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPModelProto buildPartial() {
com.google.ortools.linearsolver.MPModelProto result = new com.google.ortools.linearsolver.MPModelProto(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (variableBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
variable_ = java.util.Collections.unmodifiableList(variable_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.variable_ = variable_;
} else {
result.variable_ = variableBuilder_.build();
}
if (constraintBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0)) {
constraint_ = java.util.Collections.unmodifiableList(constraint_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.constraint_ = constraint_;
} else {
result.constraint_ = constraintBuilder_.build();
}
if (generalConstraintBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0)) {
generalConstraint_ = java.util.Collections.unmodifiableList(generalConstraint_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.generalConstraint_ = generalConstraint_;
} else {
result.generalConstraint_ = generalConstraintBuilder_.build();
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.maximize_ = maximize_;
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.objectiveOffset_ = objectiveOffset_;
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
if (quadraticObjectiveBuilder_ == null) {
result.quadraticObjective_ = quadraticObjective_;
} else {
result.quadraticObjective_ = quadraticObjectiveBuilder_.build();
}
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.name_ = name_;
if (((from_bitField0_ & 0x00000080) != 0)) {
if (solutionHintBuilder_ == null) {
result.solutionHint_ = solutionHint_;
} else {
result.solutionHint_ = solutionHintBuilder_.build();
}
to_bitField0_ |= 0x00000010;
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.linearsolver.MPModelProto) {
return mergeFrom((com.google.ortools.linearsolver.MPModelProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.linearsolver.MPModelProto other) {
if (other == com.google.ortools.linearsolver.MPModelProto.getDefaultInstance()) return this;
if (variableBuilder_ == null) {
if (!other.variable_.isEmpty()) {
if (variable_.isEmpty()) {
variable_ = other.variable_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureVariableIsMutable();
variable_.addAll(other.variable_);
}
onChanged();
}
} else {
if (!other.variable_.isEmpty()) {
if (variableBuilder_.isEmpty()) {
variableBuilder_.dispose();
variableBuilder_ = null;
variable_ = other.variable_;
bitField0_ = (bitField0_ & ~0x00000001);
variableBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getVariableFieldBuilder() : null;
} else {
variableBuilder_.addAllMessages(other.variable_);
}
}
}
if (constraintBuilder_ == null) {
if (!other.constraint_.isEmpty()) {
if (constraint_.isEmpty()) {
constraint_ = other.constraint_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureConstraintIsMutable();
constraint_.addAll(other.constraint_);
}
onChanged();
}
} else {
if (!other.constraint_.isEmpty()) {
if (constraintBuilder_.isEmpty()) {
constraintBuilder_.dispose();
constraintBuilder_ = null;
constraint_ = other.constraint_;
bitField0_ = (bitField0_ & ~0x00000002);
constraintBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConstraintFieldBuilder() : null;
} else {
constraintBuilder_.addAllMessages(other.constraint_);
}
}
}
if (generalConstraintBuilder_ == null) {
if (!other.generalConstraint_.isEmpty()) {
if (generalConstraint_.isEmpty()) {
generalConstraint_ = other.generalConstraint_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureGeneralConstraintIsMutable();
generalConstraint_.addAll(other.generalConstraint_);
}
onChanged();
}
} else {
if (!other.generalConstraint_.isEmpty()) {
if (generalConstraintBuilder_.isEmpty()) {
generalConstraintBuilder_.dispose();
generalConstraintBuilder_ = null;
generalConstraint_ = other.generalConstraint_;
bitField0_ = (bitField0_ & ~0x00000004);
generalConstraintBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getGeneralConstraintFieldBuilder() : null;
} else {
generalConstraintBuilder_.addAllMessages(other.generalConstraint_);
}
}
}
if (other.hasMaximize()) {
setMaximize(other.getMaximize());
}
if (other.hasObjectiveOffset()) {
setObjectiveOffset(other.getObjectiveOffset());
}
if (other.hasQuadraticObjective()) {
mergeQuadraticObjective(other.getQuadraticObjective());
}
if (other.hasName()) {
bitField0_ |= 0x00000040;
name_ = other.name_;
onChanged();
}
if (other.hasSolutionHint()) {
mergeSolutionHint(other.getSolutionHint());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.linearsolver.MPModelProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.linearsolver.MPModelProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List variable_ =
java.util.Collections.emptyList();
private void ensureVariableIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
variable_ = new java.util.ArrayList(variable_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPVariableProto, com.google.ortools.linearsolver.MPVariableProto.Builder, com.google.ortools.linearsolver.MPVariableProtoOrBuilder> variableBuilder_;
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public java.util.List getVariableList() {
if (variableBuilder_ == null) {
return java.util.Collections.unmodifiableList(variable_);
} else {
return variableBuilder_.getMessageList();
}
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public int getVariableCount() {
if (variableBuilder_ == null) {
return variable_.size();
} else {
return variableBuilder_.getCount();
}
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public com.google.ortools.linearsolver.MPVariableProto getVariable(int index) {
if (variableBuilder_ == null) {
return variable_.get(index);
} else {
return variableBuilder_.getMessage(index);
}
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder setVariable(
int index, com.google.ortools.linearsolver.MPVariableProto value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.set(index, value);
onChanged();
} else {
variableBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder setVariable(
int index, com.google.ortools.linearsolver.MPVariableProto.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.set(index, builderForValue.build());
onChanged();
} else {
variableBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder addVariable(com.google.ortools.linearsolver.MPVariableProto value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.add(value);
onChanged();
} else {
variableBuilder_.addMessage(value);
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder addVariable(
int index, com.google.ortools.linearsolver.MPVariableProto value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureVariableIsMutable();
variable_.add(index, value);
onChanged();
} else {
variableBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder addVariable(
com.google.ortools.linearsolver.MPVariableProto.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.add(builderForValue.build());
onChanged();
} else {
variableBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder addVariable(
int index, com.google.ortools.linearsolver.MPVariableProto.Builder builderForValue) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.add(index, builderForValue.build());
onChanged();
} else {
variableBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder addAllVariable(
java.lang.Iterable extends com.google.ortools.linearsolver.MPVariableProto> values) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, variable_);
onChanged();
} else {
variableBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder clearVariable() {
if (variableBuilder_ == null) {
variable_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
variableBuilder_.clear();
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public Builder removeVariable(int index) {
if (variableBuilder_ == null) {
ensureVariableIsMutable();
variable_.remove(index);
onChanged();
} else {
variableBuilder_.remove(index);
}
return this;
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public com.google.ortools.linearsolver.MPVariableProto.Builder getVariableBuilder(
int index) {
return getVariableFieldBuilder().getBuilder(index);
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public com.google.ortools.linearsolver.MPVariableProtoOrBuilder getVariableOrBuilder(
int index) {
if (variableBuilder_ == null) {
return variable_.get(index); } else {
return variableBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public java.util.List extends com.google.ortools.linearsolver.MPVariableProtoOrBuilder>
getVariableOrBuilderList() {
if (variableBuilder_ != null) {
return variableBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(variable_);
}
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public com.google.ortools.linearsolver.MPVariableProto.Builder addVariableBuilder() {
return getVariableFieldBuilder().addBuilder(
com.google.ortools.linearsolver.MPVariableProto.getDefaultInstance());
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public com.google.ortools.linearsolver.MPVariableProto.Builder addVariableBuilder(
int index) {
return getVariableFieldBuilder().addBuilder(
index, com.google.ortools.linearsolver.MPVariableProto.getDefaultInstance());
}
/**
*
* All the variables appearing in the model.
*
*
* repeated .operations_research.MPVariableProto variable = 3;
*/
public java.util.List
getVariableBuilderList() {
return getVariableFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPVariableProto, com.google.ortools.linearsolver.MPVariableProto.Builder, com.google.ortools.linearsolver.MPVariableProtoOrBuilder>
getVariableFieldBuilder() {
if (variableBuilder_ == null) {
variableBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPVariableProto, com.google.ortools.linearsolver.MPVariableProto.Builder, com.google.ortools.linearsolver.MPVariableProtoOrBuilder>(
variable_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
variable_ = null;
}
return variableBuilder_;
}
private java.util.List constraint_ =
java.util.Collections.emptyList();
private void ensureConstraintIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
constraint_ = new java.util.ArrayList(constraint_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPConstraintProto, com.google.ortools.linearsolver.MPConstraintProto.Builder, com.google.ortools.linearsolver.MPConstraintProtoOrBuilder> constraintBuilder_;
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public java.util.List getConstraintList() {
if (constraintBuilder_ == null) {
return java.util.Collections.unmodifiableList(constraint_);
} else {
return constraintBuilder_.getMessageList();
}
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public int getConstraintCount() {
if (constraintBuilder_ == null) {
return constraint_.size();
} else {
return constraintBuilder_.getCount();
}
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public com.google.ortools.linearsolver.MPConstraintProto getConstraint(int index) {
if (constraintBuilder_ == null) {
return constraint_.get(index);
} else {
return constraintBuilder_.getMessage(index);
}
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder setConstraint(
int index, com.google.ortools.linearsolver.MPConstraintProto value) {
if (constraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstraintIsMutable();
constraint_.set(index, value);
onChanged();
} else {
constraintBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder setConstraint(
int index, com.google.ortools.linearsolver.MPConstraintProto.Builder builderForValue) {
if (constraintBuilder_ == null) {
ensureConstraintIsMutable();
constraint_.set(index, builderForValue.build());
onChanged();
} else {
constraintBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder addConstraint(com.google.ortools.linearsolver.MPConstraintProto value) {
if (constraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstraintIsMutable();
constraint_.add(value);
onChanged();
} else {
constraintBuilder_.addMessage(value);
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder addConstraint(
int index, com.google.ortools.linearsolver.MPConstraintProto value) {
if (constraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstraintIsMutable();
constraint_.add(index, value);
onChanged();
} else {
constraintBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder addConstraint(
com.google.ortools.linearsolver.MPConstraintProto.Builder builderForValue) {
if (constraintBuilder_ == null) {
ensureConstraintIsMutable();
constraint_.add(builderForValue.build());
onChanged();
} else {
constraintBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder addConstraint(
int index, com.google.ortools.linearsolver.MPConstraintProto.Builder builderForValue) {
if (constraintBuilder_ == null) {
ensureConstraintIsMutable();
constraint_.add(index, builderForValue.build());
onChanged();
} else {
constraintBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder addAllConstraint(
java.lang.Iterable extends com.google.ortools.linearsolver.MPConstraintProto> values) {
if (constraintBuilder_ == null) {
ensureConstraintIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, constraint_);
onChanged();
} else {
constraintBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder clearConstraint() {
if (constraintBuilder_ == null) {
constraint_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
constraintBuilder_.clear();
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public Builder removeConstraint(int index) {
if (constraintBuilder_ == null) {
ensureConstraintIsMutable();
constraint_.remove(index);
onChanged();
} else {
constraintBuilder_.remove(index);
}
return this;
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public com.google.ortools.linearsolver.MPConstraintProto.Builder getConstraintBuilder(
int index) {
return getConstraintFieldBuilder().getBuilder(index);
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public com.google.ortools.linearsolver.MPConstraintProtoOrBuilder getConstraintOrBuilder(
int index) {
if (constraintBuilder_ == null) {
return constraint_.get(index); } else {
return constraintBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public java.util.List extends com.google.ortools.linearsolver.MPConstraintProtoOrBuilder>
getConstraintOrBuilderList() {
if (constraintBuilder_ != null) {
return constraintBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(constraint_);
}
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public com.google.ortools.linearsolver.MPConstraintProto.Builder addConstraintBuilder() {
return getConstraintFieldBuilder().addBuilder(
com.google.ortools.linearsolver.MPConstraintProto.getDefaultInstance());
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public com.google.ortools.linearsolver.MPConstraintProto.Builder addConstraintBuilder(
int index) {
return getConstraintFieldBuilder().addBuilder(
index, com.google.ortools.linearsolver.MPConstraintProto.getDefaultInstance());
}
/**
*
* All the constraints appearing in the model.
*
*
* repeated .operations_research.MPConstraintProto constraint = 4;
*/
public java.util.List
getConstraintBuilderList() {
return getConstraintFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPConstraintProto, com.google.ortools.linearsolver.MPConstraintProto.Builder, com.google.ortools.linearsolver.MPConstraintProtoOrBuilder>
getConstraintFieldBuilder() {
if (constraintBuilder_ == null) {
constraintBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPConstraintProto, com.google.ortools.linearsolver.MPConstraintProto.Builder, com.google.ortools.linearsolver.MPConstraintProtoOrBuilder>(
constraint_,
((bitField0_ & 0x00000002) != 0),
getParentForChildren(),
isClean());
constraint_ = null;
}
return constraintBuilder_;
}
private java.util.List generalConstraint_ =
java.util.Collections.emptyList();
private void ensureGeneralConstraintIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
generalConstraint_ = new java.util.ArrayList(generalConstraint_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPGeneralConstraintProto, com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder, com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder> generalConstraintBuilder_;
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public java.util.List getGeneralConstraintList() {
if (generalConstraintBuilder_ == null) {
return java.util.Collections.unmodifiableList(generalConstraint_);
} else {
return generalConstraintBuilder_.getMessageList();
}
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public int getGeneralConstraintCount() {
if (generalConstraintBuilder_ == null) {
return generalConstraint_.size();
} else {
return generalConstraintBuilder_.getCount();
}
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public com.google.ortools.linearsolver.MPGeneralConstraintProto getGeneralConstraint(int index) {
if (generalConstraintBuilder_ == null) {
return generalConstraint_.get(index);
} else {
return generalConstraintBuilder_.getMessage(index);
}
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder setGeneralConstraint(
int index, com.google.ortools.linearsolver.MPGeneralConstraintProto value) {
if (generalConstraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneralConstraintIsMutable();
generalConstraint_.set(index, value);
onChanged();
} else {
generalConstraintBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder setGeneralConstraint(
int index, com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder builderForValue) {
if (generalConstraintBuilder_ == null) {
ensureGeneralConstraintIsMutable();
generalConstraint_.set(index, builderForValue.build());
onChanged();
} else {
generalConstraintBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder addGeneralConstraint(com.google.ortools.linearsolver.MPGeneralConstraintProto value) {
if (generalConstraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneralConstraintIsMutable();
generalConstraint_.add(value);
onChanged();
} else {
generalConstraintBuilder_.addMessage(value);
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder addGeneralConstraint(
int index, com.google.ortools.linearsolver.MPGeneralConstraintProto value) {
if (generalConstraintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGeneralConstraintIsMutable();
generalConstraint_.add(index, value);
onChanged();
} else {
generalConstraintBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder addGeneralConstraint(
com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder builderForValue) {
if (generalConstraintBuilder_ == null) {
ensureGeneralConstraintIsMutable();
generalConstraint_.add(builderForValue.build());
onChanged();
} else {
generalConstraintBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder addGeneralConstraint(
int index, com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder builderForValue) {
if (generalConstraintBuilder_ == null) {
ensureGeneralConstraintIsMutable();
generalConstraint_.add(index, builderForValue.build());
onChanged();
} else {
generalConstraintBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder addAllGeneralConstraint(
java.lang.Iterable extends com.google.ortools.linearsolver.MPGeneralConstraintProto> values) {
if (generalConstraintBuilder_ == null) {
ensureGeneralConstraintIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, generalConstraint_);
onChanged();
} else {
generalConstraintBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder clearGeneralConstraint() {
if (generalConstraintBuilder_ == null) {
generalConstraint_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
generalConstraintBuilder_.clear();
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public Builder removeGeneralConstraint(int index) {
if (generalConstraintBuilder_ == null) {
ensureGeneralConstraintIsMutable();
generalConstraint_.remove(index);
onChanged();
} else {
generalConstraintBuilder_.remove(index);
}
return this;
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder getGeneralConstraintBuilder(
int index) {
return getGeneralConstraintFieldBuilder().getBuilder(index);
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder getGeneralConstraintOrBuilder(
int index) {
if (generalConstraintBuilder_ == null) {
return generalConstraint_.get(index); } else {
return generalConstraintBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public java.util.List extends com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder>
getGeneralConstraintOrBuilderList() {
if (generalConstraintBuilder_ != null) {
return generalConstraintBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(generalConstraint_);
}
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder addGeneralConstraintBuilder() {
return getGeneralConstraintFieldBuilder().addBuilder(
com.google.ortools.linearsolver.MPGeneralConstraintProto.getDefaultInstance());
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder addGeneralConstraintBuilder(
int index) {
return getGeneralConstraintFieldBuilder().addBuilder(
index, com.google.ortools.linearsolver.MPGeneralConstraintProto.getDefaultInstance());
}
/**
*
* All the general constraints appearing in the model. Note that not all
* solvers support all types of general constraints.
*
*
* repeated .operations_research.MPGeneralConstraintProto general_constraint = 7;
*/
public java.util.List
getGeneralConstraintBuilderList() {
return getGeneralConstraintFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPGeneralConstraintProto, com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder, com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder>
getGeneralConstraintFieldBuilder() {
if (generalConstraintBuilder_ == null) {
generalConstraintBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.linearsolver.MPGeneralConstraintProto, com.google.ortools.linearsolver.MPGeneralConstraintProto.Builder, com.google.ortools.linearsolver.MPGeneralConstraintProtoOrBuilder>(
generalConstraint_,
((bitField0_ & 0x00000004) != 0),
getParentForChildren(),
isClean());
generalConstraint_ = null;
}
return generalConstraintBuilder_;
}
private boolean maximize_ ;
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @return Whether the maximize field is set.
*/
@java.lang.Override
public boolean hasMaximize() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @return The maximize.
*/
@java.lang.Override
public boolean getMaximize() {
return maximize_;
}
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @param value The maximize to set.
* @return This builder for chaining.
*/
public Builder setMaximize(boolean value) {
bitField0_ |= 0x00000008;
maximize_ = value;
onChanged();
return this;
}
/**
*
* True if the problem is a maximization problem. Minimize by default.
*
*
* optional bool maximize = 1 [default = false];
* @return This builder for chaining.
*/
public Builder clearMaximize() {
bitField0_ = (bitField0_ & ~0x00000008);
maximize_ = false;
onChanged();
return this;
}
private double objectiveOffset_ ;
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @return Whether the objectiveOffset field is set.
*/
@java.lang.Override
public boolean hasObjectiveOffset() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @return The objectiveOffset.
*/
@java.lang.Override
public double getObjectiveOffset() {
return objectiveOffset_;
}
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @param value The objectiveOffset to set.
* @return This builder for chaining.
*/
public Builder setObjectiveOffset(double value) {
bitField0_ |= 0x00000010;
objectiveOffset_ = value;
onChanged();
return this;
}
/**
*
* Offset for the objective function. Must be finite.
*
*
* optional double objective_offset = 2 [default = 0];
* @return This builder for chaining.
*/
public Builder clearObjectiveOffset() {
bitField0_ = (bitField0_ & ~0x00000010);
objectiveOffset_ = 0D;
onChanged();
return this;
}
private com.google.ortools.linearsolver.MPQuadraticObjective quadraticObjective_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.MPQuadraticObjective, com.google.ortools.linearsolver.MPQuadraticObjective.Builder, com.google.ortools.linearsolver.MPQuadraticObjectiveOrBuilder> quadraticObjectiveBuilder_;
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
* @return Whether the quadraticObjective field is set.
*/
public boolean hasQuadraticObjective() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
* @return The quadraticObjective.
*/
public com.google.ortools.linearsolver.MPQuadraticObjective getQuadraticObjective() {
if (quadraticObjectiveBuilder_ == null) {
return quadraticObjective_ == null ? com.google.ortools.linearsolver.MPQuadraticObjective.getDefaultInstance() : quadraticObjective_;
} else {
return quadraticObjectiveBuilder_.getMessage();
}
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public Builder setQuadraticObjective(com.google.ortools.linearsolver.MPQuadraticObjective value) {
if (quadraticObjectiveBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
quadraticObjective_ = value;
onChanged();
} else {
quadraticObjectiveBuilder_.setMessage(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public Builder setQuadraticObjective(
com.google.ortools.linearsolver.MPQuadraticObjective.Builder builderForValue) {
if (quadraticObjectiveBuilder_ == null) {
quadraticObjective_ = builderForValue.build();
onChanged();
} else {
quadraticObjectiveBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000020;
return this;
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public Builder mergeQuadraticObjective(com.google.ortools.linearsolver.MPQuadraticObjective value) {
if (quadraticObjectiveBuilder_ == null) {
if (((bitField0_ & 0x00000020) != 0) &&
quadraticObjective_ != null &&
quadraticObjective_ != com.google.ortools.linearsolver.MPQuadraticObjective.getDefaultInstance()) {
quadraticObjective_ =
com.google.ortools.linearsolver.MPQuadraticObjective.newBuilder(quadraticObjective_).mergeFrom(value).buildPartial();
} else {
quadraticObjective_ = value;
}
onChanged();
} else {
quadraticObjectiveBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000020;
return this;
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public Builder clearQuadraticObjective() {
if (quadraticObjectiveBuilder_ == null) {
quadraticObjective_ = null;
onChanged();
} else {
quadraticObjectiveBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public com.google.ortools.linearsolver.MPQuadraticObjective.Builder getQuadraticObjectiveBuilder() {
bitField0_ |= 0x00000020;
onChanged();
return getQuadraticObjectiveFieldBuilder().getBuilder();
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
public com.google.ortools.linearsolver.MPQuadraticObjectiveOrBuilder getQuadraticObjectiveOrBuilder() {
if (quadraticObjectiveBuilder_ != null) {
return quadraticObjectiveBuilder_.getMessageOrBuilder();
} else {
return quadraticObjective_ == null ?
com.google.ortools.linearsolver.MPQuadraticObjective.getDefaultInstance() : quadraticObjective_;
}
}
/**
*
* Optionally, a quadratic objective.
* As of 2019/06, only SCIP and Gurobi support quadratic objectives.
*
*
* optional .operations_research.MPQuadraticObjective quadratic_objective = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.MPQuadraticObjective, com.google.ortools.linearsolver.MPQuadraticObjective.Builder, com.google.ortools.linearsolver.MPQuadraticObjectiveOrBuilder>
getQuadraticObjectiveFieldBuilder() {
if (quadraticObjectiveBuilder_ == null) {
quadraticObjectiveBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.MPQuadraticObjective, com.google.ortools.linearsolver.MPQuadraticObjective.Builder, com.google.ortools.linearsolver.MPQuadraticObjectiveOrBuilder>(
getQuadraticObjective(),
getParentForChildren(),
isClean());
quadraticObjective_ = null;
}
return quadraticObjectiveBuilder_;
}
private java.lang.Object name_ = "";
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return Whether the name field is set.
*/
public boolean hasName() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (bs.isValidUtf8()) {
name_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
name_ = value;
onChanged();
return this;
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @return This builder for chaining.
*/
public Builder clearName() {
bitField0_ = (bitField0_ & ~0x00000040);
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
*
* Name of the model.
*
*
* optional string name = 5 [default = ""];
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000040;
name_ = value;
onChanged();
return this;
}
private com.google.ortools.linearsolver.PartialVariableAssignment solutionHint_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.PartialVariableAssignment, com.google.ortools.linearsolver.PartialVariableAssignment.Builder, com.google.ortools.linearsolver.PartialVariableAssignmentOrBuilder> solutionHintBuilder_;
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
* @return Whether the solutionHint field is set.
*/
public boolean hasSolutionHint() {
return ((bitField0_ & 0x00000080) != 0);
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
* @return The solutionHint.
*/
public com.google.ortools.linearsolver.PartialVariableAssignment getSolutionHint() {
if (solutionHintBuilder_ == null) {
return solutionHint_ == null ? com.google.ortools.linearsolver.PartialVariableAssignment.getDefaultInstance() : solutionHint_;
} else {
return solutionHintBuilder_.getMessage();
}
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public Builder setSolutionHint(com.google.ortools.linearsolver.PartialVariableAssignment value) {
if (solutionHintBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
solutionHint_ = value;
onChanged();
} else {
solutionHintBuilder_.setMessage(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public Builder setSolutionHint(
com.google.ortools.linearsolver.PartialVariableAssignment.Builder builderForValue) {
if (solutionHintBuilder_ == null) {
solutionHint_ = builderForValue.build();
onChanged();
} else {
solutionHintBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public Builder mergeSolutionHint(com.google.ortools.linearsolver.PartialVariableAssignment value) {
if (solutionHintBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0) &&
solutionHint_ != null &&
solutionHint_ != com.google.ortools.linearsolver.PartialVariableAssignment.getDefaultInstance()) {
solutionHint_ =
com.google.ortools.linearsolver.PartialVariableAssignment.newBuilder(solutionHint_).mergeFrom(value).buildPartial();
} else {
solutionHint_ = value;
}
onChanged();
} else {
solutionHintBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000080;
return this;
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public Builder clearSolutionHint() {
if (solutionHintBuilder_ == null) {
solutionHint_ = null;
onChanged();
} else {
solutionHintBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public com.google.ortools.linearsolver.PartialVariableAssignment.Builder getSolutionHintBuilder() {
bitField0_ |= 0x00000080;
onChanged();
return getSolutionHintFieldBuilder().getBuilder();
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
public com.google.ortools.linearsolver.PartialVariableAssignmentOrBuilder getSolutionHintOrBuilder() {
if (solutionHintBuilder_ != null) {
return solutionHintBuilder_.getMessageOrBuilder();
} else {
return solutionHint_ == null ?
com.google.ortools.linearsolver.PartialVariableAssignment.getDefaultInstance() : solutionHint_;
}
}
/**
*
* Solution hint.
* If a feasible or almost-feasible solution to the problem is already known,
* it may be helpful to pass it to the solver so that it can be used. A solver
* that supports this feature will try to use this information to create its
* initial feasible solution.
* Note that it may not always be faster to give a hint like this to the
* solver. There is also no guarantee that the solver will use this hint or
* try to return a solution "close" to this assignment in case of multiple
* optimal solutions.
*
*
* optional .operations_research.PartialVariableAssignment solution_hint = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.PartialVariableAssignment, com.google.ortools.linearsolver.PartialVariableAssignment.Builder, com.google.ortools.linearsolver.PartialVariableAssignmentOrBuilder>
getSolutionHintFieldBuilder() {
if (solutionHintBuilder_ == null) {
solutionHintBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.PartialVariableAssignment, com.google.ortools.linearsolver.PartialVariableAssignment.Builder, com.google.ortools.linearsolver.PartialVariableAssignmentOrBuilder>(
getSolutionHint(),
getParentForChildren(),
isClean());
solutionHint_ = null;
}
return solutionHintBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.MPModelProto)
}
// @@protoc_insertion_point(class_scope:operations_research.MPModelProto)
private static final com.google.ortools.linearsolver.MPModelProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.linearsolver.MPModelProto();
}
public static com.google.ortools.linearsolver.MPModelProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MPModelProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MPModelProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPModelProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy