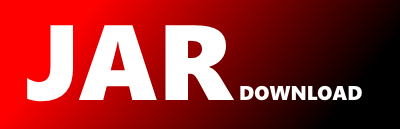
com.google.ortools.linearsolver.MPQuadraticConstraintOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/linear_solver/linear_solver.proto
package com.google.ortools.linearsolver;
public interface MPQuadraticConstraintOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.MPQuadraticConstraint)
com.google.protobuf.MessageOrBuilder {
/**
*
* Sparse representation of linear terms in the quadratic constraint, where
* term i is var_index[i] * coefficient[i].
* `var_index` are variable indices w.r.t the "variable" field in
* MPModelProto, and should be unique.
*
*
* repeated int32 var_index = 1;
* @return A list containing the varIndex.
*/
java.util.List getVarIndexList();
/**
*
* Sparse representation of linear terms in the quadratic constraint, where
* term i is var_index[i] * coefficient[i].
* `var_index` are variable indices w.r.t the "variable" field in
* MPModelProto, and should be unique.
*
*
* repeated int32 var_index = 1;
* @return The count of varIndex.
*/
int getVarIndexCount();
/**
*
* Sparse representation of linear terms in the quadratic constraint, where
* term i is var_index[i] * coefficient[i].
* `var_index` are variable indices w.r.t the "variable" field in
* MPModelProto, and should be unique.
*
*
* repeated int32 var_index = 1;
* @param index The index of the element to return.
* @return The varIndex at the given index.
*/
int getVarIndex(int index);
/**
*
* Must be finite.
*
*
* repeated double coefficient = 2;
* @return A list containing the coefficient.
*/
java.util.List getCoefficientList();
/**
*
* Must be finite.
*
*
* repeated double coefficient = 2;
* @return The count of coefficient.
*/
int getCoefficientCount();
/**
*
* Must be finite.
*
*
* repeated double coefficient = 2;
* @param index The index of the element to return.
* @return The coefficient at the given index.
*/
double getCoefficient(int index);
/**
*
* Sparse representation of quadratic terms in the quadratic constraint, where
* term i is qvar1_index[i] * qvar2_index[i] * qcoefficient[i].
* `qvar1_index` and `qvar2_index` are variable indices w.r.t the "variable"
* field in MPModelProto.
* `qvar1_index`, `qvar2_index` and `coefficients` must have the same size.
* If the same unordered pair (qvar1_index, qvar2_index) appears several
* times, the sum of all of the associated coefficients will be applied.
*
*
* repeated int32 qvar1_index = 3;
* @return A list containing the qvar1Index.
*/
java.util.List getQvar1IndexList();
/**
*
* Sparse representation of quadratic terms in the quadratic constraint, where
* term i is qvar1_index[i] * qvar2_index[i] * qcoefficient[i].
* `qvar1_index` and `qvar2_index` are variable indices w.r.t the "variable"
* field in MPModelProto.
* `qvar1_index`, `qvar2_index` and `coefficients` must have the same size.
* If the same unordered pair (qvar1_index, qvar2_index) appears several
* times, the sum of all of the associated coefficients will be applied.
*
*
* repeated int32 qvar1_index = 3;
* @return The count of qvar1Index.
*/
int getQvar1IndexCount();
/**
*
* Sparse representation of quadratic terms in the quadratic constraint, where
* term i is qvar1_index[i] * qvar2_index[i] * qcoefficient[i].
* `qvar1_index` and `qvar2_index` are variable indices w.r.t the "variable"
* field in MPModelProto.
* `qvar1_index`, `qvar2_index` and `coefficients` must have the same size.
* If the same unordered pair (qvar1_index, qvar2_index) appears several
* times, the sum of all of the associated coefficients will be applied.
*
*
* repeated int32 qvar1_index = 3;
* @param index The index of the element to return.
* @return The qvar1Index at the given index.
*/
int getQvar1Index(int index);
/**
* repeated int32 qvar2_index = 4;
* @return A list containing the qvar2Index.
*/
java.util.List getQvar2IndexList();
/**
* repeated int32 qvar2_index = 4;
* @return The count of qvar2Index.
*/
int getQvar2IndexCount();
/**
* repeated int32 qvar2_index = 4;
* @param index The index of the element to return.
* @return The qvar2Index at the given index.
*/
int getQvar2Index(int index);
/**
*
* Must be finite.
*
*
* repeated double qcoefficient = 5;
* @return A list containing the qcoefficient.
*/
java.util.List getQcoefficientList();
/**
*
* Must be finite.
*
*
* repeated double qcoefficient = 5;
* @return The count of qcoefficient.
*/
int getQcoefficientCount();
/**
*
* Must be finite.
*
*
* repeated double qcoefficient = 5;
* @param index The index of the element to return.
* @return The qcoefficient at the given index.
*/
double getQcoefficient(int index);
/**
*
* lower_bound must be <= upper_bound.
*
*
* optional double lower_bound = 6 [default = -inf];
* @return Whether the lowerBound field is set.
*/
boolean hasLowerBound();
/**
*
* lower_bound must be <= upper_bound.
*
*
* optional double lower_bound = 6 [default = -inf];
* @return The lowerBound.
*/
double getLowerBound();
/**
* optional double upper_bound = 7 [default = inf];
* @return Whether the upperBound field is set.
*/
boolean hasUpperBound();
/**
* optional double upper_bound = 7 [default = inf];
* @return The upperBound.
*/
double getUpperBound();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy