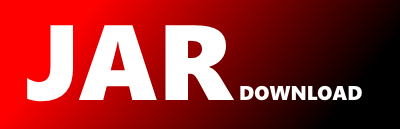
com.google.ortools.linearsolver.MPSolutionResponseOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/linear_solver/linear_solver.proto
package com.google.ortools.linearsolver;
public interface MPSolutionResponseOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.MPSolutionResponse)
com.google.protobuf.MessageOrBuilder {
/**
*
* Result of the optimization.
*
*
* optional .operations_research.MPSolverResponseStatus status = 1 [default = MPSOLVER_UNKNOWN_STATUS];
* @return Whether the status field is set.
*/
boolean hasStatus();
/**
*
* Result of the optimization.
*
*
* optional .operations_research.MPSolverResponseStatus status = 1 [default = MPSOLVER_UNKNOWN_STATUS];
* @return The status.
*/
com.google.ortools.linearsolver.MPSolverResponseStatus getStatus();
/**
*
* Human-readable string giving more details about the status. For example,
* when the status is MPSOLVER_INVALID_MODE, this can hold a description of
* why the model is invalid.
* This isn't always filled: don't depend on its value or even its presence.
*
*
* optional string status_str = 7;
* @return Whether the statusStr field is set.
*/
boolean hasStatusStr();
/**
*
* Human-readable string giving more details about the status. For example,
* when the status is MPSOLVER_INVALID_MODE, this can hold a description of
* why the model is invalid.
* This isn't always filled: don't depend on its value or even its presence.
*
*
* optional string status_str = 7;
* @return The statusStr.
*/
java.lang.String getStatusStr();
/**
*
* Human-readable string giving more details about the status. For example,
* when the status is MPSOLVER_INVALID_MODE, this can hold a description of
* why the model is invalid.
* This isn't always filled: don't depend on its value or even its presence.
*
*
* optional string status_str = 7;
* @return The bytes for statusStr.
*/
com.google.protobuf.ByteString
getStatusStrBytes();
/**
*
* Objective value corresponding to the "variable_value" below, taking into
* account the source "objective_offset" and "objective_coefficient".
* This is set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* optional double objective_value = 2;
* @return Whether the objectiveValue field is set.
*/
boolean hasObjectiveValue();
/**
*
* Objective value corresponding to the "variable_value" below, taking into
* account the source "objective_offset" and "objective_coefficient".
* This is set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* optional double objective_value = 2;
* @return The objectiveValue.
*/
double getObjectiveValue();
/**
*
* This field is only filled for MIP problems. For a minimization problem,
* this is a lower bound on the optimal objective value. For a maximization
* problem, it is an upper bound. It is only filled if the status is OPTIMAL
* or FEASIBLE. In the former case, best_objective_bound should be equal to
* objective_value (modulo numerical errors).
*
*
* optional double best_objective_bound = 5;
* @return Whether the bestObjectiveBound field is set.
*/
boolean hasBestObjectiveBound();
/**
*
* This field is only filled for MIP problems. For a minimization problem,
* this is a lower bound on the optimal objective value. For a maximization
* problem, it is an upper bound. It is only filled if the status is OPTIMAL
* or FEASIBLE. In the former case, best_objective_bound should be equal to
* objective_value (modulo numerical errors).
*
*
* optional double best_objective_bound = 5;
* @return The bestObjectiveBound.
*/
double getBestObjectiveBound();
/**
*
* Variable values in the same order as the MPModelProto::variable field.
* This is a dense representation. These are set iff 'status' is OPTIMAL or
* FEASIBLE.
*
*
* repeated double variable_value = 3 [packed = true];
* @return A list containing the variableValue.
*/
java.util.List getVariableValueList();
/**
*
* Variable values in the same order as the MPModelProto::variable field.
* This is a dense representation. These are set iff 'status' is OPTIMAL or
* FEASIBLE.
*
*
* repeated double variable_value = 3 [packed = true];
* @return The count of variableValue.
*/
int getVariableValueCount();
/**
*
* Variable values in the same order as the MPModelProto::variable field.
* This is a dense representation. These are set iff 'status' is OPTIMAL or
* FEASIBLE.
*
*
* repeated double variable_value = 3 [packed = true];
* @param index The index of the element to return.
* @return The variableValue at the given index.
*/
double getVariableValue(int index);
/**
*
* [Advanced usage.]
* Values of the dual variables values in the same order as the
* MPModelProto::constraint field. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if
* it is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double dual_value = 4 [packed = true];
* @return A list containing the dualValue.
*/
java.util.List getDualValueList();
/**
*
* [Advanced usage.]
* Values of the dual variables values in the same order as the
* MPModelProto::constraint field. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if
* it is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double dual_value = 4 [packed = true];
* @return The count of dualValue.
*/
int getDualValueCount();
/**
*
* [Advanced usage.]
* Values of the dual variables values in the same order as the
* MPModelProto::constraint field. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if
* it is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double dual_value = 4 [packed = true];
* @param index The index of the element to return.
* @return The dualValue at the given index.
*/
double getDualValue(int index);
/**
*
* [Advanced usage.]
* Values of the reduced cost of the variables in the same order as the
* MPModelProto::variable. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if it
* is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double reduced_cost = 6 [packed = true];
* @return A list containing the reducedCost.
*/
java.util.List getReducedCostList();
/**
*
* [Advanced usage.]
* Values of the reduced cost of the variables in the same order as the
* MPModelProto::variable. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if it
* is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double reduced_cost = 6 [packed = true];
* @return The count of reducedCost.
*/
int getReducedCostCount();
/**
*
* [Advanced usage.]
* Values of the reduced cost of the variables in the same order as the
* MPModelProto::variable. This is a dense representation.
* These are not set if the problem was solved with a MIP solver (even if it
* is actually a linear program).
* These are set iff 'status' is OPTIMAL or FEASIBLE.
*
*
* repeated double reduced_cost = 6 [packed = true];
* @param index The index of the element to return.
* @return The reducedCost at the given index.
*/
double getReducedCost(int index);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy