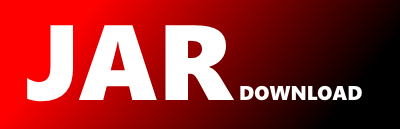
com.google.ortools.linearsolver.MPSolverCommonParameters Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/linear_solver/linear_solver.proto
package com.google.ortools.linearsolver;
/**
*
* MPSolverCommonParameters holds advanced usage parameters that apply to any of
* the solvers we support.
* All of the fields in this proto can have a value of unspecified. In this
* case each inner solver will use their own safe defaults.
* Some values won't be supported by some solvers. The behavior in that case is
* not defined yet.
*
*
* Protobuf type {@code operations_research.MPSolverCommonParameters}
*/
public final class MPSolverCommonParameters extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.MPSolverCommonParameters)
MPSolverCommonParametersOrBuilder {
private static final long serialVersionUID = 0L;
// Use MPSolverCommonParameters.newBuilder() to construct.
private MPSolverCommonParameters(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private MPSolverCommonParameters() {
lpAlgorithm_ = 0;
presolve_ = 0;
scaling_ = 0;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new MPSolverCommonParameters();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private MPSolverCommonParameters(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
com.google.ortools.linearsolver.OptionalDouble.Builder subBuilder = null;
if (((bitField0_ & 0x00000001) != 0)) {
subBuilder = relativeMipGap_.toBuilder();
}
relativeMipGap_ = input.readMessage(com.google.ortools.linearsolver.OptionalDouble.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(relativeMipGap_);
relativeMipGap_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000001;
break;
}
case 18: {
com.google.ortools.linearsolver.OptionalDouble.Builder subBuilder = null;
if (((bitField0_ & 0x00000002) != 0)) {
subBuilder = primalTolerance_.toBuilder();
}
primalTolerance_ = input.readMessage(com.google.ortools.linearsolver.OptionalDouble.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(primalTolerance_);
primalTolerance_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000002;
break;
}
case 26: {
com.google.ortools.linearsolver.OptionalDouble.Builder subBuilder = null;
if (((bitField0_ & 0x00000004) != 0)) {
subBuilder = dualTolerance_.toBuilder();
}
dualTolerance_ = input.readMessage(com.google.ortools.linearsolver.OptionalDouble.PARSER, extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(dualTolerance_);
dualTolerance_ = subBuilder.buildPartial();
}
bitField0_ |= 0x00000004;
break;
}
case 32: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues value = com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(4, rawValue);
} else {
bitField0_ |= 0x00000008;
lpAlgorithm_ = rawValue;
}
break;
}
case 40: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean value = com.google.ortools.util.OptionalBoolean.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(5, rawValue);
} else {
bitField0_ |= 0x00000010;
presolve_ = rawValue;
}
break;
}
case 56: {
int rawValue = input.readEnum();
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean value = com.google.ortools.util.OptionalBoolean.valueOf(rawValue);
if (value == null) {
unknownFields.mergeVarintField(7, rawValue);
} else {
bitField0_ |= 0x00000020;
scaling_ = rawValue;
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPSolverCommonParameters_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPSolverCommonParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.linearsolver.MPSolverCommonParameters.class, com.google.ortools.linearsolver.MPSolverCommonParameters.Builder.class);
}
/**
* Protobuf enum {@code operations_research.MPSolverCommonParameters.LPAlgorithmValues}
*/
public enum LPAlgorithmValues
implements com.google.protobuf.ProtocolMessageEnum {
/**
* LP_ALGO_UNSPECIFIED = 0;
*/
LP_ALGO_UNSPECIFIED(0),
/**
*
* Dual simplex.
*
*
* LP_ALGO_DUAL = 1;
*/
LP_ALGO_DUAL(1),
/**
*
* Primal simplex.
*
*
* LP_ALGO_PRIMAL = 2;
*/
LP_ALGO_PRIMAL(2),
/**
*
* Barrier algorithm.
*
*
* LP_ALGO_BARRIER = 3;
*/
LP_ALGO_BARRIER(3),
;
/**
* LP_ALGO_UNSPECIFIED = 0;
*/
public static final int LP_ALGO_UNSPECIFIED_VALUE = 0;
/**
*
* Dual simplex.
*
*
* LP_ALGO_DUAL = 1;
*/
public static final int LP_ALGO_DUAL_VALUE = 1;
/**
*
* Primal simplex.
*
*
* LP_ALGO_PRIMAL = 2;
*/
public static final int LP_ALGO_PRIMAL_VALUE = 2;
/**
*
* Barrier algorithm.
*
*
* LP_ALGO_BARRIER = 3;
*/
public static final int LP_ALGO_BARRIER_VALUE = 3;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static LPAlgorithmValues valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static LPAlgorithmValues forNumber(int value) {
switch (value) {
case 0: return LP_ALGO_UNSPECIFIED;
case 1: return LP_ALGO_DUAL;
case 2: return LP_ALGO_PRIMAL;
case 3: return LP_ALGO_BARRIER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
LPAlgorithmValues> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public LPAlgorithmValues findValueByNumber(int number) {
return LPAlgorithmValues.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.ortools.linearsolver.MPSolverCommonParameters.getDescriptor().getEnumTypes().get(0);
}
private static final LPAlgorithmValues[] VALUES = values();
public static LPAlgorithmValues valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private LPAlgorithmValues(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:operations_research.MPSolverCommonParameters.LPAlgorithmValues)
}
private int bitField0_;
public static final int RELATIVE_MIP_GAP_FIELD_NUMBER = 1;
private com.google.ortools.linearsolver.OptionalDouble relativeMipGap_;
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
* @return Whether the relativeMipGap field is set.
*/
@java.lang.Override
public boolean hasRelativeMipGap() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
* @return The relativeMipGap.
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDouble getRelativeMipGap() {
return relativeMipGap_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : relativeMipGap_;
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getRelativeMipGapOrBuilder() {
return relativeMipGap_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : relativeMipGap_;
}
public static final int PRIMAL_TOLERANCE_FIELD_NUMBER = 2;
private com.google.ortools.linearsolver.OptionalDouble primalTolerance_;
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
* @return Whether the primalTolerance field is set.
*/
@java.lang.Override
public boolean hasPrimalTolerance() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
* @return The primalTolerance.
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDouble getPrimalTolerance() {
return primalTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : primalTolerance_;
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getPrimalToleranceOrBuilder() {
return primalTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : primalTolerance_;
}
public static final int DUAL_TOLERANCE_FIELD_NUMBER = 3;
private com.google.ortools.linearsolver.OptionalDouble dualTolerance_;
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
* @return Whether the dualTolerance field is set.
*/
@java.lang.Override
public boolean hasDualTolerance() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
* @return The dualTolerance.
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDouble getDualTolerance() {
return dualTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : dualTolerance_;
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
@java.lang.Override
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getDualToleranceOrBuilder() {
return dualTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : dualTolerance_;
}
public static final int LP_ALGORITHM_FIELD_NUMBER = 4;
private int lpAlgorithm_;
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @return Whether the lpAlgorithm field is set.
*/
@java.lang.Override public boolean hasLpAlgorithm() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @return The lpAlgorithm.
*/
@java.lang.Override public com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues getLpAlgorithm() {
@SuppressWarnings("deprecation")
com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues result = com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues.valueOf(lpAlgorithm_);
return result == null ? com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues.LP_ALGO_UNSPECIFIED : result;
}
public static final int PRESOLVE_FIELD_NUMBER = 5;
private int presolve_;
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @return Whether the presolve field is set.
*/
@java.lang.Override public boolean hasPresolve() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @return The presolve.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getPresolve() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(presolve_);
return result == null ? com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED : result;
}
public static final int SCALING_FIELD_NUMBER = 7;
private int scaling_;
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @return Whether the scaling field is set.
*/
@java.lang.Override public boolean hasScaling() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @return The scaling.
*/
@java.lang.Override public com.google.ortools.util.OptionalBoolean getScaling() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(scaling_);
return result == null ? com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(1, getRelativeMipGap());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(2, getPrimalTolerance());
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(3, getDualTolerance());
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeEnum(4, lpAlgorithm_);
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeEnum(5, presolve_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeEnum(7, scaling_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRelativeMipGap());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getPrimalTolerance());
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getDualTolerance());
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, lpAlgorithm_);
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, presolve_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, scaling_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.linearsolver.MPSolverCommonParameters)) {
return super.equals(obj);
}
com.google.ortools.linearsolver.MPSolverCommonParameters other = (com.google.ortools.linearsolver.MPSolverCommonParameters) obj;
if (hasRelativeMipGap() != other.hasRelativeMipGap()) return false;
if (hasRelativeMipGap()) {
if (!getRelativeMipGap()
.equals(other.getRelativeMipGap())) return false;
}
if (hasPrimalTolerance() != other.hasPrimalTolerance()) return false;
if (hasPrimalTolerance()) {
if (!getPrimalTolerance()
.equals(other.getPrimalTolerance())) return false;
}
if (hasDualTolerance() != other.hasDualTolerance()) return false;
if (hasDualTolerance()) {
if (!getDualTolerance()
.equals(other.getDualTolerance())) return false;
}
if (hasLpAlgorithm() != other.hasLpAlgorithm()) return false;
if (hasLpAlgorithm()) {
if (lpAlgorithm_ != other.lpAlgorithm_) return false;
}
if (hasPresolve() != other.hasPresolve()) return false;
if (hasPresolve()) {
if (presolve_ != other.presolve_) return false;
}
if (hasScaling() != other.hasScaling()) return false;
if (hasScaling()) {
if (scaling_ != other.scaling_) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRelativeMipGap()) {
hash = (37 * hash) + RELATIVE_MIP_GAP_FIELD_NUMBER;
hash = (53 * hash) + getRelativeMipGap().hashCode();
}
if (hasPrimalTolerance()) {
hash = (37 * hash) + PRIMAL_TOLERANCE_FIELD_NUMBER;
hash = (53 * hash) + getPrimalTolerance().hashCode();
}
if (hasDualTolerance()) {
hash = (37 * hash) + DUAL_TOLERANCE_FIELD_NUMBER;
hash = (53 * hash) + getDualTolerance().hashCode();
}
if (hasLpAlgorithm()) {
hash = (37 * hash) + LP_ALGORITHM_FIELD_NUMBER;
hash = (53 * hash) + lpAlgorithm_;
}
if (hasPresolve()) {
hash = (37 * hash) + PRESOLVE_FIELD_NUMBER;
hash = (53 * hash) + presolve_;
}
if (hasScaling()) {
hash = (37 * hash) + SCALING_FIELD_NUMBER;
hash = (53 * hash) + scaling_;
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.linearsolver.MPSolverCommonParameters prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* MPSolverCommonParameters holds advanced usage parameters that apply to any of
* the solvers we support.
* All of the fields in this proto can have a value of unspecified. In this
* case each inner solver will use their own safe defaults.
* Some values won't be supported by some solvers. The behavior in that case is
* not defined yet.
*
*
* Protobuf type {@code operations_research.MPSolverCommonParameters}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.MPSolverCommonParameters)
com.google.ortools.linearsolver.MPSolverCommonParametersOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPSolverCommonParameters_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPSolverCommonParameters_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.linearsolver.MPSolverCommonParameters.class, com.google.ortools.linearsolver.MPSolverCommonParameters.Builder.class);
}
// Construct using com.google.ortools.linearsolver.MPSolverCommonParameters.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getRelativeMipGapFieldBuilder();
getPrimalToleranceFieldBuilder();
getDualToleranceFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (relativeMipGapBuilder_ == null) {
relativeMipGap_ = null;
} else {
relativeMipGapBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
if (primalToleranceBuilder_ == null) {
primalTolerance_ = null;
} else {
primalToleranceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
if (dualToleranceBuilder_ == null) {
dualTolerance_ = null;
} else {
dualToleranceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
lpAlgorithm_ = 0;
bitField0_ = (bitField0_ & ~0x00000008);
presolve_ = 0;
bitField0_ = (bitField0_ & ~0x00000010);
scaling_ = 0;
bitField0_ = (bitField0_ & ~0x00000020);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.linearsolver.LinearSolver.internal_static_operations_research_MPSolverCommonParameters_descriptor;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPSolverCommonParameters getDefaultInstanceForType() {
return com.google.ortools.linearsolver.MPSolverCommonParameters.getDefaultInstance();
}
@java.lang.Override
public com.google.ortools.linearsolver.MPSolverCommonParameters build() {
com.google.ortools.linearsolver.MPSolverCommonParameters result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPSolverCommonParameters buildPartial() {
com.google.ortools.linearsolver.MPSolverCommonParameters result = new com.google.ortools.linearsolver.MPSolverCommonParameters(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00000001) != 0)) {
if (relativeMipGapBuilder_ == null) {
result.relativeMipGap_ = relativeMipGap_;
} else {
result.relativeMipGap_ = relativeMipGapBuilder_.build();
}
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
if (primalToleranceBuilder_ == null) {
result.primalTolerance_ = primalTolerance_;
} else {
result.primalTolerance_ = primalToleranceBuilder_.build();
}
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
if (dualToleranceBuilder_ == null) {
result.dualTolerance_ = dualTolerance_;
} else {
result.dualTolerance_ = dualToleranceBuilder_.build();
}
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
to_bitField0_ |= 0x00000008;
}
result.lpAlgorithm_ = lpAlgorithm_;
if (((from_bitField0_ & 0x00000010) != 0)) {
to_bitField0_ |= 0x00000010;
}
result.presolve_ = presolve_;
if (((from_bitField0_ & 0x00000020) != 0)) {
to_bitField0_ |= 0x00000020;
}
result.scaling_ = scaling_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.linearsolver.MPSolverCommonParameters) {
return mergeFrom((com.google.ortools.linearsolver.MPSolverCommonParameters)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.linearsolver.MPSolverCommonParameters other) {
if (other == com.google.ortools.linearsolver.MPSolverCommonParameters.getDefaultInstance()) return this;
if (other.hasRelativeMipGap()) {
mergeRelativeMipGap(other.getRelativeMipGap());
}
if (other.hasPrimalTolerance()) {
mergePrimalTolerance(other.getPrimalTolerance());
}
if (other.hasDualTolerance()) {
mergeDualTolerance(other.getDualTolerance());
}
if (other.hasLpAlgorithm()) {
setLpAlgorithm(other.getLpAlgorithm());
}
if (other.hasPresolve()) {
setPresolve(other.getPresolve());
}
if (other.hasScaling()) {
setScaling(other.getScaling());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.linearsolver.MPSolverCommonParameters parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.linearsolver.MPSolverCommonParameters) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.ortools.linearsolver.OptionalDouble relativeMipGap_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder> relativeMipGapBuilder_;
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
* @return Whether the relativeMipGap field is set.
*/
public boolean hasRelativeMipGap() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
* @return The relativeMipGap.
*/
public com.google.ortools.linearsolver.OptionalDouble getRelativeMipGap() {
if (relativeMipGapBuilder_ == null) {
return relativeMipGap_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : relativeMipGap_;
} else {
return relativeMipGapBuilder_.getMessage();
}
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public Builder setRelativeMipGap(com.google.ortools.linearsolver.OptionalDouble value) {
if (relativeMipGapBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
relativeMipGap_ = value;
onChanged();
} else {
relativeMipGapBuilder_.setMessage(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public Builder setRelativeMipGap(
com.google.ortools.linearsolver.OptionalDouble.Builder builderForValue) {
if (relativeMipGapBuilder_ == null) {
relativeMipGap_ = builderForValue.build();
onChanged();
} else {
relativeMipGapBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public Builder mergeRelativeMipGap(com.google.ortools.linearsolver.OptionalDouble value) {
if (relativeMipGapBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0) &&
relativeMipGap_ != null &&
relativeMipGap_ != com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance()) {
relativeMipGap_ =
com.google.ortools.linearsolver.OptionalDouble.newBuilder(relativeMipGap_).mergeFrom(value).buildPartial();
} else {
relativeMipGap_ = value;
}
onChanged();
} else {
relativeMipGapBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000001;
return this;
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public Builder clearRelativeMipGap() {
if (relativeMipGapBuilder_ == null) {
relativeMipGap_ = null;
onChanged();
} else {
relativeMipGapBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public com.google.ortools.linearsolver.OptionalDouble.Builder getRelativeMipGapBuilder() {
bitField0_ |= 0x00000001;
onChanged();
return getRelativeMipGapFieldBuilder().getBuilder();
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getRelativeMipGapOrBuilder() {
if (relativeMipGapBuilder_ != null) {
return relativeMipGapBuilder_.getMessageOrBuilder();
} else {
return relativeMipGap_ == null ?
com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : relativeMipGap_;
}
}
/**
*
* The solver stops if the relative MIP gap reaches this value or below.
* The relative MIP gap is an upper bound of the relative distance to the
* optimum, and it is defined as:
* abs(best_bound - incumbent) / abs(incumbent) [Gurobi]
* abs(best_bound - incumbent) / min(abs(best_bound), abs(incumbent)) [SCIP]
* where "incumbent" is the objective value of the best solution found so far
* (i.e., lowest when minimizing, highest when maximizing), and "best_bound"
* is the tightest bound of the objective determined so far (i.e., highest
* when minimizing, and lowest when maximizing). The MIP Gap is sensitive to
* objective offset. If the denominator is 0 the MIP Gap is INFINITY for SCIP
* and Gurobi. Of note, "incumbent" and "best bound" are called "primal bound"
* and "dual bound" in SCIP, respectively.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalDouble relative_mip_gap = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>
getRelativeMipGapFieldBuilder() {
if (relativeMipGapBuilder_ == null) {
relativeMipGapBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>(
getRelativeMipGap(),
getParentForChildren(),
isClean());
relativeMipGap_ = null;
}
return relativeMipGapBuilder_;
}
private com.google.ortools.linearsolver.OptionalDouble primalTolerance_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder> primalToleranceBuilder_;
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
* @return Whether the primalTolerance field is set.
*/
public boolean hasPrimalTolerance() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
* @return The primalTolerance.
*/
public com.google.ortools.linearsolver.OptionalDouble getPrimalTolerance() {
if (primalToleranceBuilder_ == null) {
return primalTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : primalTolerance_;
} else {
return primalToleranceBuilder_.getMessage();
}
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public Builder setPrimalTolerance(com.google.ortools.linearsolver.OptionalDouble value) {
if (primalToleranceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
primalTolerance_ = value;
onChanged();
} else {
primalToleranceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public Builder setPrimalTolerance(
com.google.ortools.linearsolver.OptionalDouble.Builder builderForValue) {
if (primalToleranceBuilder_ == null) {
primalTolerance_ = builderForValue.build();
onChanged();
} else {
primalToleranceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public Builder mergePrimalTolerance(com.google.ortools.linearsolver.OptionalDouble value) {
if (primalToleranceBuilder_ == null) {
if (((bitField0_ & 0x00000002) != 0) &&
primalTolerance_ != null &&
primalTolerance_ != com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance()) {
primalTolerance_ =
com.google.ortools.linearsolver.OptionalDouble.newBuilder(primalTolerance_).mergeFrom(value).buildPartial();
} else {
primalTolerance_ = value;
}
onChanged();
} else {
primalToleranceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000002;
return this;
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public Builder clearPrimalTolerance() {
if (primalToleranceBuilder_ == null) {
primalTolerance_ = null;
onChanged();
} else {
primalToleranceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000002);
return this;
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public com.google.ortools.linearsolver.OptionalDouble.Builder getPrimalToleranceBuilder() {
bitField0_ |= 0x00000002;
onChanged();
return getPrimalToleranceFieldBuilder().getBuilder();
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getPrimalToleranceOrBuilder() {
if (primalToleranceBuilder_ != null) {
return primalToleranceBuilder_.getMessageOrBuilder();
} else {
return primalTolerance_ == null ?
com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : primalTolerance_;
}
}
/**
*
* Tolerance for primal feasibility of basic solutions: this is the maximum
* allowed error in constraint satisfiability.
* For SCIP this includes integrality constraints. For Gurobi it does not, you
* need to set the custom parameter IntFeasTol.
*
*
* optional .operations_research.OptionalDouble primal_tolerance = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>
getPrimalToleranceFieldBuilder() {
if (primalToleranceBuilder_ == null) {
primalToleranceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>(
getPrimalTolerance(),
getParentForChildren(),
isClean());
primalTolerance_ = null;
}
return primalToleranceBuilder_;
}
private com.google.ortools.linearsolver.OptionalDouble dualTolerance_;
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder> dualToleranceBuilder_;
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
* @return Whether the dualTolerance field is set.
*/
public boolean hasDualTolerance() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
* @return The dualTolerance.
*/
public com.google.ortools.linearsolver.OptionalDouble getDualTolerance() {
if (dualToleranceBuilder_ == null) {
return dualTolerance_ == null ? com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : dualTolerance_;
} else {
return dualToleranceBuilder_.getMessage();
}
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public Builder setDualTolerance(com.google.ortools.linearsolver.OptionalDouble value) {
if (dualToleranceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
dualTolerance_ = value;
onChanged();
} else {
dualToleranceBuilder_.setMessage(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public Builder setDualTolerance(
com.google.ortools.linearsolver.OptionalDouble.Builder builderForValue) {
if (dualToleranceBuilder_ == null) {
dualTolerance_ = builderForValue.build();
onChanged();
} else {
dualToleranceBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public Builder mergeDualTolerance(com.google.ortools.linearsolver.OptionalDouble value) {
if (dualToleranceBuilder_ == null) {
if (((bitField0_ & 0x00000004) != 0) &&
dualTolerance_ != null &&
dualTolerance_ != com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance()) {
dualTolerance_ =
com.google.ortools.linearsolver.OptionalDouble.newBuilder(dualTolerance_).mergeFrom(value).buildPartial();
} else {
dualTolerance_ = value;
}
onChanged();
} else {
dualToleranceBuilder_.mergeFrom(value);
}
bitField0_ |= 0x00000004;
return this;
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public Builder clearDualTolerance() {
if (dualToleranceBuilder_ == null) {
dualTolerance_ = null;
onChanged();
} else {
dualToleranceBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000004);
return this;
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public com.google.ortools.linearsolver.OptionalDouble.Builder getDualToleranceBuilder() {
bitField0_ |= 0x00000004;
onChanged();
return getDualToleranceFieldBuilder().getBuilder();
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
public com.google.ortools.linearsolver.OptionalDoubleOrBuilder getDualToleranceOrBuilder() {
if (dualToleranceBuilder_ != null) {
return dualToleranceBuilder_.getMessageOrBuilder();
} else {
return dualTolerance_ == null ?
com.google.ortools.linearsolver.OptionalDouble.getDefaultInstance() : dualTolerance_;
}
}
/**
*
* Tolerance for dual feasibility.
* For SCIP and Gurobi this is the feasibility tolerance for reduced costs in
* LP solution: reduced costs must all be smaller than this value in the
* improving direction in order for a model to be declared optimal.
* Not supported for other solvers.
*
*
* optional .operations_research.OptionalDouble dual_tolerance = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>
getDualToleranceFieldBuilder() {
if (dualToleranceBuilder_ == null) {
dualToleranceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
com.google.ortools.linearsolver.OptionalDouble, com.google.ortools.linearsolver.OptionalDouble.Builder, com.google.ortools.linearsolver.OptionalDoubleOrBuilder>(
getDualTolerance(),
getParentForChildren(),
isClean());
dualTolerance_ = null;
}
return dualToleranceBuilder_;
}
private int lpAlgorithm_ = 0;
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @return Whether the lpAlgorithm field is set.
*/
@java.lang.Override public boolean hasLpAlgorithm() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @return The lpAlgorithm.
*/
@java.lang.Override
public com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues getLpAlgorithm() {
@SuppressWarnings("deprecation")
com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues result = com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues.valueOf(lpAlgorithm_);
return result == null ? com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues.LP_ALGO_UNSPECIFIED : result;
}
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @param value The lpAlgorithm to set.
* @return This builder for chaining.
*/
public Builder setLpAlgorithm(com.google.ortools.linearsolver.MPSolverCommonParameters.LPAlgorithmValues value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
lpAlgorithm_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Algorithm to solve linear programs.
* Ask or-core-team@ if you want to know what this does exactly.
*
*
* optional .operations_research.MPSolverCommonParameters.LPAlgorithmValues lp_algorithm = 4 [default = LP_ALGO_UNSPECIFIED];
* @return This builder for chaining.
*/
public Builder clearLpAlgorithm() {
bitField0_ = (bitField0_ & ~0x00000008);
lpAlgorithm_ = 0;
onChanged();
return this;
}
private int presolve_ = 0;
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @return Whether the presolve field is set.
*/
@java.lang.Override public boolean hasPresolve() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @return The presolve.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getPresolve() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(presolve_);
return result == null ? com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED : result;
}
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @param value The presolve to set.
* @return This builder for chaining.
*/
public Builder setPresolve(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000010;
presolve_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Gurobi and SCIP enable presolve by default.
* Ask or-core-team@ for other solvers.
*
*
* optional .operations_research.OptionalBoolean presolve = 5 [default = BOOL_UNSPECIFIED];
* @return This builder for chaining.
*/
public Builder clearPresolve() {
bitField0_ = (bitField0_ & ~0x00000010);
presolve_ = 0;
onChanged();
return this;
}
private int scaling_ = 0;
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @return Whether the scaling field is set.
*/
@java.lang.Override public boolean hasScaling() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @return The scaling.
*/
@java.lang.Override
public com.google.ortools.util.OptionalBoolean getScaling() {
@SuppressWarnings("deprecation")
com.google.ortools.util.OptionalBoolean result = com.google.ortools.util.OptionalBoolean.valueOf(scaling_);
return result == null ? com.google.ortools.util.OptionalBoolean.BOOL_UNSPECIFIED : result;
}
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @param value The scaling to set.
* @return This builder for chaining.
*/
public Builder setScaling(com.google.ortools.util.OptionalBoolean value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
scaling_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Enable automatic scaling of matrix coefficients and objective. Available
* for Gurobi and GLOP.
* Ask or-core-team@ if you want more details.
*
*
* optional .operations_research.OptionalBoolean scaling = 7 [default = BOOL_UNSPECIFIED];
* @return This builder for chaining.
*/
public Builder clearScaling() {
bitField0_ = (bitField0_ & ~0x00000020);
scaling_ = 0;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.MPSolverCommonParameters)
}
// @@protoc_insertion_point(class_scope:operations_research.MPSolverCommonParameters)
private static final com.google.ortools.linearsolver.MPSolverCommonParameters DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.linearsolver.MPSolverCommonParameters();
}
public static com.google.ortools.linearsolver.MPSolverCommonParameters getDefaultInstance() {
return DEFAULT_INSTANCE;
}
@java.lang.Deprecated public static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MPSolverCommonParameters parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new MPSolverCommonParameters(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ortools.linearsolver.MPSolverCommonParameters getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy