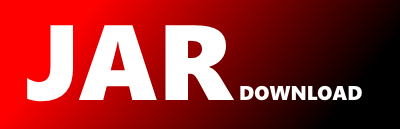
com.google.ortools.linearsolver.MPSolverResponseStatus Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/linear_solver/linear_solver.proto
package com.google.ortools.linearsolver;
/**
*
* Status returned by the solver. They follow a hierarchical nomenclature, to
* allow us to add more enum values in the future. Clients should use
* InCategory() to match these enums, with the following C++ pseudo-code:
* bool InCategory(MPSolverResponseStatus status, MPSolverResponseStatus cat) {
* if (cat == MPSOLVER_OPTIMAL) return status == MPSOLVER_OPTIMAL;
* while (status > cat) status >>= 4;
* return status == cat;
* }
*
*
* Protobuf enum {@code operations_research.MPSolverResponseStatus}
*/
public enum MPSolverResponseStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* The solver found the proven optimal solution. This is what should be
* returned in most cases.
* WARNING: for historical reason, the value is zero, which means that this
* value can't have any subcategories.
*
*
* MPSOLVER_OPTIMAL = 0;
*/
MPSOLVER_OPTIMAL(0),
/**
*
* The solver had enough time to find some solution that satisfies all
* constraints, but it did not prove optimality (which means it may or may
* not have reached the optimal).
* This can happen for large LP models (Linear Programming), and is a frequent
* response for time-limited MIPs (Mixed Integer Programming). In the MIP
* case, the difference between the solution 'objective_value' and
* 'best_objective_bound' fields of the MPSolutionResponse will give an
* indication of how far this solution is from the optimal one.
*
*
* MPSOLVER_FEASIBLE = 1;
*/
MPSOLVER_FEASIBLE(1),
/**
*
* The model does not have any solution, according to the solver (which
* "proved" it, with the caveat that numerical proofs aren't actual proofs),
* or based on trivial considerations (eg. a variable whose lower bound is
* strictly greater than its upper bound).
*
*
* MPSOLVER_INFEASIBLE = 2;
*/
MPSOLVER_INFEASIBLE(2),
/**
*
* There exist solutions that make the magnitude of the objective value
* as large as wanted (i.e. -infinity (resp. +infinity) for a minimization
* (resp. maximization) problem.
*
*
* MPSOLVER_UNBOUNDED = 3;
*/
MPSOLVER_UNBOUNDED(3),
/**
*
* An error (most probably numerical) occurred.
* One likely cause for such errors is a large numerical range among variable
* coefficients (eg. 1e-16, 1e20), in which case one should try to shrink it.
*
*
* MPSOLVER_ABNORMAL = 4;
*/
MPSOLVER_ABNORMAL(4),
/**
*
* The solver did not have a chance to diagnose the model in one of the
* categories above.
*
*
* MPSOLVER_NOT_SOLVED = 6;
*/
MPSOLVER_NOT_SOLVED(6),
/**
*
* Like "NOT_SOLVED", but typically used by model validation functions
* returning a "model status", to enhance readability of the client code.
*
*
* MPSOLVER_MODEL_IS_VALID = 97;
*/
MPSOLVER_MODEL_IS_VALID(97),
/**
*
* Special value: the solver status could not be properly translated and is
* unknown.
*
*
* MPSOLVER_UNKNOWN_STATUS = 99;
*/
MPSOLVER_UNKNOWN_STATUS(99),
/**
*
* Model errors. These are always deterministic and repeatable.
* They should be accompanied with a string description of the error.
*
*
* MPSOLVER_MODEL_INVALID = 5;
*/
MPSOLVER_MODEL_INVALID(5),
/**
*
* Something is wrong with the fields "solution_hint_var_index" and/or
* "solution_hint_var_value".
*
*
* MPSOLVER_MODEL_INVALID_SOLUTION_HINT = 84;
*/
MPSOLVER_MODEL_INVALID_SOLUTION_HINT(84),
/**
*
* Something is wrong with the solver_specific_parameters request field.
*
*
* MPSOLVER_MODEL_INVALID_SOLVER_PARAMETERS = 85;
*/
MPSOLVER_MODEL_INVALID_SOLVER_PARAMETERS(85),
/**
*
* Implementation error: the requested solver implementation is not
* available (see MPModelRequest.solver_type).
* The linear solver binary was probably not linked with the required library,
* eg //ortools/linear_solver:linear_solver_scip for SCIP.
*
*
* MPSOLVER_SOLVER_TYPE_UNAVAILABLE = 7;
*/
MPSOLVER_SOLVER_TYPE_UNAVAILABLE(7),
;
/**
*
* The solver found the proven optimal solution. This is what should be
* returned in most cases.
* WARNING: for historical reason, the value is zero, which means that this
* value can't have any subcategories.
*
*
* MPSOLVER_OPTIMAL = 0;
*/
public static final int MPSOLVER_OPTIMAL_VALUE = 0;
/**
*
* The solver had enough time to find some solution that satisfies all
* constraints, but it did not prove optimality (which means it may or may
* not have reached the optimal).
* This can happen for large LP models (Linear Programming), and is a frequent
* response for time-limited MIPs (Mixed Integer Programming). In the MIP
* case, the difference between the solution 'objective_value' and
* 'best_objective_bound' fields of the MPSolutionResponse will give an
* indication of how far this solution is from the optimal one.
*
*
* MPSOLVER_FEASIBLE = 1;
*/
public static final int MPSOLVER_FEASIBLE_VALUE = 1;
/**
*
* The model does not have any solution, according to the solver (which
* "proved" it, with the caveat that numerical proofs aren't actual proofs),
* or based on trivial considerations (eg. a variable whose lower bound is
* strictly greater than its upper bound).
*
*
* MPSOLVER_INFEASIBLE = 2;
*/
public static final int MPSOLVER_INFEASIBLE_VALUE = 2;
/**
*
* There exist solutions that make the magnitude of the objective value
* as large as wanted (i.e. -infinity (resp. +infinity) for a minimization
* (resp. maximization) problem.
*
*
* MPSOLVER_UNBOUNDED = 3;
*/
public static final int MPSOLVER_UNBOUNDED_VALUE = 3;
/**
*
* An error (most probably numerical) occurred.
* One likely cause for such errors is a large numerical range among variable
* coefficients (eg. 1e-16, 1e20), in which case one should try to shrink it.
*
*
* MPSOLVER_ABNORMAL = 4;
*/
public static final int MPSOLVER_ABNORMAL_VALUE = 4;
/**
*
* The solver did not have a chance to diagnose the model in one of the
* categories above.
*
*
* MPSOLVER_NOT_SOLVED = 6;
*/
public static final int MPSOLVER_NOT_SOLVED_VALUE = 6;
/**
*
* Like "NOT_SOLVED", but typically used by model validation functions
* returning a "model status", to enhance readability of the client code.
*
*
* MPSOLVER_MODEL_IS_VALID = 97;
*/
public static final int MPSOLVER_MODEL_IS_VALID_VALUE = 97;
/**
*
* Special value: the solver status could not be properly translated and is
* unknown.
*
*
* MPSOLVER_UNKNOWN_STATUS = 99;
*/
public static final int MPSOLVER_UNKNOWN_STATUS_VALUE = 99;
/**
*
* Model errors. These are always deterministic and repeatable.
* They should be accompanied with a string description of the error.
*
*
* MPSOLVER_MODEL_INVALID = 5;
*/
public static final int MPSOLVER_MODEL_INVALID_VALUE = 5;
/**
*
* Something is wrong with the fields "solution_hint_var_index" and/or
* "solution_hint_var_value".
*
*
* MPSOLVER_MODEL_INVALID_SOLUTION_HINT = 84;
*/
public static final int MPSOLVER_MODEL_INVALID_SOLUTION_HINT_VALUE = 84;
/**
*
* Something is wrong with the solver_specific_parameters request field.
*
*
* MPSOLVER_MODEL_INVALID_SOLVER_PARAMETERS = 85;
*/
public static final int MPSOLVER_MODEL_INVALID_SOLVER_PARAMETERS_VALUE = 85;
/**
*
* Implementation error: the requested solver implementation is not
* available (see MPModelRequest.solver_type).
* The linear solver binary was probably not linked with the required library,
* eg //ortools/linear_solver:linear_solver_scip for SCIP.
*
*
* MPSOLVER_SOLVER_TYPE_UNAVAILABLE = 7;
*/
public static final int MPSOLVER_SOLVER_TYPE_UNAVAILABLE_VALUE = 7;
public final int getNumber() {
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static MPSolverResponseStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static MPSolverResponseStatus forNumber(int value) {
switch (value) {
case 0: return MPSOLVER_OPTIMAL;
case 1: return MPSOLVER_FEASIBLE;
case 2: return MPSOLVER_INFEASIBLE;
case 3: return MPSOLVER_UNBOUNDED;
case 4: return MPSOLVER_ABNORMAL;
case 6: return MPSOLVER_NOT_SOLVED;
case 97: return MPSOLVER_MODEL_IS_VALID;
case 99: return MPSOLVER_UNKNOWN_STATUS;
case 5: return MPSOLVER_MODEL_INVALID;
case 84: return MPSOLVER_MODEL_INVALID_SOLUTION_HINT;
case 85: return MPSOLVER_MODEL_INVALID_SOLVER_PARAMETERS;
case 7: return MPSOLVER_SOLVER_TYPE_UNAVAILABLE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
MPSolverResponseStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public MPSolverResponseStatus findValueByNumber(int number) {
return MPSolverResponseStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return com.google.ortools.linearsolver.LinearSolver.getDescriptor().getEnumTypes().get(0);
}
private static final MPSolverResponseStatus[] VALUES = values();
public static MPSolverResponseStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
return VALUES[desc.getIndex()];
}
private final int value;
private MPSolverResponseStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:operations_research.MPSolverResponseStatus)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy