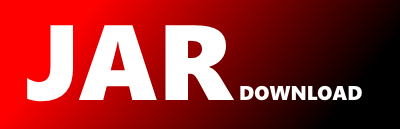
com.google.ortools.sat.ConstraintProtoOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/sat/cp_model.proto
package com.google.ortools.sat;
public interface ConstraintProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:operations_research.sat.ConstraintProto)
com.google.protobuf.MessageOrBuilder {
/**
*
* For debug/logging only. Can be empty.
*
*
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
*
* For debug/logging only. Can be empty.
*
*
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
*
* The constraint will be enforced iff all literals listed here are true. If
* this is empty, then the constraint will always be enforced. An enforced
* constraint must be satisfied, and an un-enforced one will simply be
* ignored.
* This is also called half-reification. To have an equivalence between a
* literal and a constraint (full reification), one must add both a constraint
* (controlled by a literal l) and its negation (controlled by the negation of
* l).
* Important: as of September 2018, only a few constraint support enforcement:
* - bool_or, bool_and, linear: fully supported.
* - interval: only support a single enforcement literal.
* - other: no support (but can be added on a per-demand basis).
*
*
* repeated int32 enforcement_literal = 2;
* @return A list containing the enforcementLiteral.
*/
java.util.List getEnforcementLiteralList();
/**
*
* The constraint will be enforced iff all literals listed here are true. If
* this is empty, then the constraint will always be enforced. An enforced
* constraint must be satisfied, and an un-enforced one will simply be
* ignored.
* This is also called half-reification. To have an equivalence between a
* literal and a constraint (full reification), one must add both a constraint
* (controlled by a literal l) and its negation (controlled by the negation of
* l).
* Important: as of September 2018, only a few constraint support enforcement:
* - bool_or, bool_and, linear: fully supported.
* - interval: only support a single enforcement literal.
* - other: no support (but can be added on a per-demand basis).
*
*
* repeated int32 enforcement_literal = 2;
* @return The count of enforcementLiteral.
*/
int getEnforcementLiteralCount();
/**
*
* The constraint will be enforced iff all literals listed here are true. If
* this is empty, then the constraint will always be enforced. An enforced
* constraint must be satisfied, and an un-enforced one will simply be
* ignored.
* This is also called half-reification. To have an equivalence between a
* literal and a constraint (full reification), one must add both a constraint
* (controlled by a literal l) and its negation (controlled by the negation of
* l).
* Important: as of September 2018, only a few constraint support enforcement:
* - bool_or, bool_and, linear: fully supported.
* - interval: only support a single enforcement literal.
* - other: no support (but can be added on a per-demand basis).
*
*
* repeated int32 enforcement_literal = 2;
* @param index The index of the element to return.
* @return The enforcementLiteral at the given index.
*/
int getEnforcementLiteral(int index);
/**
*
* The bool_or constraint forces at least one literal to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_or = 3;
* @return Whether the boolOr field is set.
*/
boolean hasBoolOr();
/**
*
* The bool_or constraint forces at least one literal to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_or = 3;
* @return The boolOr.
*/
com.google.ortools.sat.BoolArgumentProto getBoolOr();
/**
*
* The bool_or constraint forces at least one literal to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_or = 3;
*/
com.google.ortools.sat.BoolArgumentProtoOrBuilder getBoolOrOrBuilder();
/**
*
* The bool_and constraint forces all of the literals to be true.
* This is a "redundant" constraint in the sense that this can easily be
* encoded with many bool_or. It is just more space efficient and handled
* slightly differently internally.
*
*
* .operations_research.sat.BoolArgumentProto bool_and = 4;
* @return Whether the boolAnd field is set.
*/
boolean hasBoolAnd();
/**
*
* The bool_and constraint forces all of the literals to be true.
* This is a "redundant" constraint in the sense that this can easily be
* encoded with many bool_or. It is just more space efficient and handled
* slightly differently internally.
*
*
* .operations_research.sat.BoolArgumentProto bool_and = 4;
* @return The boolAnd.
*/
com.google.ortools.sat.BoolArgumentProto getBoolAnd();
/**
*
* The bool_and constraint forces all of the literals to be true.
* This is a "redundant" constraint in the sense that this can easily be
* encoded with many bool_or. It is just more space efficient and handled
* slightly differently internally.
*
*
* .operations_research.sat.BoolArgumentProto bool_and = 4;
*/
com.google.ortools.sat.BoolArgumentProtoOrBuilder getBoolAndOrBuilder();
/**
*
* The at_most_one constraint enforces that no more than one literal is
* true at the same time. Note that an at most one constraint of length n
* could be encoded with n bool_and constraint with n-1 term on the right
* hand side. So in a sense, this constraint contribute directly to the
* "implication-graph" or the 2-SAT part of the model.
*
*
* .operations_research.sat.BoolArgumentProto at_most_one = 26;
* @return Whether the atMostOne field is set.
*/
boolean hasAtMostOne();
/**
*
* The at_most_one constraint enforces that no more than one literal is
* true at the same time. Note that an at most one constraint of length n
* could be encoded with n bool_and constraint with n-1 term on the right
* hand side. So in a sense, this constraint contribute directly to the
* "implication-graph" or the 2-SAT part of the model.
*
*
* .operations_research.sat.BoolArgumentProto at_most_one = 26;
* @return The atMostOne.
*/
com.google.ortools.sat.BoolArgumentProto getAtMostOne();
/**
*
* The at_most_one constraint enforces that no more than one literal is
* true at the same time. Note that an at most one constraint of length n
* could be encoded with n bool_and constraint with n-1 term on the right
* hand side. So in a sense, this constraint contribute directly to the
* "implication-graph" or the 2-SAT part of the model.
*
*
* .operations_research.sat.BoolArgumentProto at_most_one = 26;
*/
com.google.ortools.sat.BoolArgumentProtoOrBuilder getAtMostOneOrBuilder();
/**
*
* The bool_xor constraint forces an odd number of the literals to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_xor = 5;
* @return Whether the boolXor field is set.
*/
boolean hasBoolXor();
/**
*
* The bool_xor constraint forces an odd number of the literals to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_xor = 5;
* @return The boolXor.
*/
com.google.ortools.sat.BoolArgumentProto getBoolXor();
/**
*
* The bool_xor constraint forces an odd number of the literals to be true.
*
*
* .operations_research.sat.BoolArgumentProto bool_xor = 5;
*/
com.google.ortools.sat.BoolArgumentProtoOrBuilder getBoolXorOrBuilder();
/**
*
* The int_div constraint forces the target to equal vars[0] / vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_div = 7;
* @return Whether the intDiv field is set.
*/
boolean hasIntDiv();
/**
*
* The int_div constraint forces the target to equal vars[0] / vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_div = 7;
* @return The intDiv.
*/
com.google.ortools.sat.IntegerArgumentProto getIntDiv();
/**
*
* The int_div constraint forces the target to equal vars[0] / vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_div = 7;
*/
com.google.ortools.sat.IntegerArgumentProtoOrBuilder getIntDivOrBuilder();
/**
*
* The int_mod constraint forces the target to equal vars[0] % vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_mod = 8;
* @return Whether the intMod field is set.
*/
boolean hasIntMod();
/**
*
* The int_mod constraint forces the target to equal vars[0] % vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_mod = 8;
* @return The intMod.
*/
com.google.ortools.sat.IntegerArgumentProto getIntMod();
/**
*
* The int_mod constraint forces the target to equal vars[0] % vars[1].
*
*
* .operations_research.sat.IntegerArgumentProto int_mod = 8;
*/
com.google.ortools.sat.IntegerArgumentProtoOrBuilder getIntModOrBuilder();
/**
*
* The int_max constraint forces the target to equal the maximum of all
* variables.
* TODO(user): Remove int_max in favor of lin_max.
*
*
* .operations_research.sat.IntegerArgumentProto int_max = 9;
* @return Whether the intMax field is set.
*/
boolean hasIntMax();
/**
*
* The int_max constraint forces the target to equal the maximum of all
* variables.
* TODO(user): Remove int_max in favor of lin_max.
*
*
* .operations_research.sat.IntegerArgumentProto int_max = 9;
* @return The intMax.
*/
com.google.ortools.sat.IntegerArgumentProto getIntMax();
/**
*
* The int_max constraint forces the target to equal the maximum of all
* variables.
* TODO(user): Remove int_max in favor of lin_max.
*
*
* .operations_research.sat.IntegerArgumentProto int_max = 9;
*/
com.google.ortools.sat.IntegerArgumentProtoOrBuilder getIntMaxOrBuilder();
/**
*
* The lin_max constraint forces the target to equal the maximum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_max = 27;
* @return Whether the linMax field is set.
*/
boolean hasLinMax();
/**
*
* The lin_max constraint forces the target to equal the maximum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_max = 27;
* @return The linMax.
*/
com.google.ortools.sat.LinearArgumentProto getLinMax();
/**
*
* The lin_max constraint forces the target to equal the maximum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_max = 27;
*/
com.google.ortools.sat.LinearArgumentProtoOrBuilder getLinMaxOrBuilder();
/**
*
* The int_min constraint forces the target to equal the minimum of all
* variables.
* TODO(user): Remove int_min in favor of lin_min.
*
*
* .operations_research.sat.IntegerArgumentProto int_min = 10;
* @return Whether the intMin field is set.
*/
boolean hasIntMin();
/**
*
* The int_min constraint forces the target to equal the minimum of all
* variables.
* TODO(user): Remove int_min in favor of lin_min.
*
*
* .operations_research.sat.IntegerArgumentProto int_min = 10;
* @return The intMin.
*/
com.google.ortools.sat.IntegerArgumentProto getIntMin();
/**
*
* The int_min constraint forces the target to equal the minimum of all
* variables.
* TODO(user): Remove int_min in favor of lin_min.
*
*
* .operations_research.sat.IntegerArgumentProto int_min = 10;
*/
com.google.ortools.sat.IntegerArgumentProtoOrBuilder getIntMinOrBuilder();
/**
*
* The lin_min constraint forces the target to equal the minimum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_min = 28;
* @return Whether the linMin field is set.
*/
boolean hasLinMin();
/**
*
* The lin_min constraint forces the target to equal the minimum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_min = 28;
* @return The linMin.
*/
com.google.ortools.sat.LinearArgumentProto getLinMin();
/**
*
* The lin_min constraint forces the target to equal the minimum of all
* linear expressions.
*
*
* .operations_research.sat.LinearArgumentProto lin_min = 28;
*/
com.google.ortools.sat.LinearArgumentProtoOrBuilder getLinMinOrBuilder();
/**
*
* The int_prod constraint forces the target to equal the product of all
* variables.
*
*
* .operations_research.sat.IntegerArgumentProto int_prod = 11;
* @return Whether the intProd field is set.
*/
boolean hasIntProd();
/**
*
* The int_prod constraint forces the target to equal the product of all
* variables.
*
*
* .operations_research.sat.IntegerArgumentProto int_prod = 11;
* @return The intProd.
*/
com.google.ortools.sat.IntegerArgumentProto getIntProd();
/**
*
* The int_prod constraint forces the target to equal the product of all
* variables.
*
*
* .operations_research.sat.IntegerArgumentProto int_prod = 11;
*/
com.google.ortools.sat.IntegerArgumentProtoOrBuilder getIntProdOrBuilder();
/**
*
* The linear constraint enforces a linear inequality among the variables,
* such as 0 <= x + 2y <= 10.
*
*
* .operations_research.sat.LinearConstraintProto linear = 12;
* @return Whether the linear field is set.
*/
boolean hasLinear();
/**
*
* The linear constraint enforces a linear inequality among the variables,
* such as 0 <= x + 2y <= 10.
*
*
* .operations_research.sat.LinearConstraintProto linear = 12;
* @return The linear.
*/
com.google.ortools.sat.LinearConstraintProto getLinear();
/**
*
* The linear constraint enforces a linear inequality among the variables,
* such as 0 <= x + 2y <= 10.
*
*
* .operations_research.sat.LinearConstraintProto linear = 12;
*/
com.google.ortools.sat.LinearConstraintProtoOrBuilder getLinearOrBuilder();
/**
*
* The all_diff constraint forces all variables to take different values.
*
*
* .operations_research.sat.AllDifferentConstraintProto all_diff = 13;
* @return Whether the allDiff field is set.
*/
boolean hasAllDiff();
/**
*
* The all_diff constraint forces all variables to take different values.
*
*
* .operations_research.sat.AllDifferentConstraintProto all_diff = 13;
* @return The allDiff.
*/
com.google.ortools.sat.AllDifferentConstraintProto getAllDiff();
/**
*
* The all_diff constraint forces all variables to take different values.
*
*
* .operations_research.sat.AllDifferentConstraintProto all_diff = 13;
*/
com.google.ortools.sat.AllDifferentConstraintProtoOrBuilder getAllDiffOrBuilder();
/**
*
* The element constraint forces the variable with the given index
* to be equal to the target.
*
*
* .operations_research.sat.ElementConstraintProto element = 14;
* @return Whether the element field is set.
*/
boolean hasElement();
/**
*
* The element constraint forces the variable with the given index
* to be equal to the target.
*
*
* .operations_research.sat.ElementConstraintProto element = 14;
* @return The element.
*/
com.google.ortools.sat.ElementConstraintProto getElement();
/**
*
* The element constraint forces the variable with the given index
* to be equal to the target.
*
*
* .operations_research.sat.ElementConstraintProto element = 14;
*/
com.google.ortools.sat.ElementConstraintProtoOrBuilder getElementOrBuilder();
/**
*
* The circuit constraint takes a graph and forces the arcs present
* (with arc presence indicated by a literal) to form a unique cycle.
*
*
* .operations_research.sat.CircuitConstraintProto circuit = 15;
* @return Whether the circuit field is set.
*/
boolean hasCircuit();
/**
*
* The circuit constraint takes a graph and forces the arcs present
* (with arc presence indicated by a literal) to form a unique cycle.
*
*
* .operations_research.sat.CircuitConstraintProto circuit = 15;
* @return The circuit.
*/
com.google.ortools.sat.CircuitConstraintProto getCircuit();
/**
*
* The circuit constraint takes a graph and forces the arcs present
* (with arc presence indicated by a literal) to form a unique cycle.
*
*
* .operations_research.sat.CircuitConstraintProto circuit = 15;
*/
com.google.ortools.sat.CircuitConstraintProtoOrBuilder getCircuitOrBuilder();
/**
*
* The routes constraint implements the vehicle routing problem.
*
*
* .operations_research.sat.RoutesConstraintProto routes = 23;
* @return Whether the routes field is set.
*/
boolean hasRoutes();
/**
*
* The routes constraint implements the vehicle routing problem.
*
*
* .operations_research.sat.RoutesConstraintProto routes = 23;
* @return The routes.
*/
com.google.ortools.sat.RoutesConstraintProto getRoutes();
/**
*
* The routes constraint implements the vehicle routing problem.
*
*
* .operations_research.sat.RoutesConstraintProto routes = 23;
*/
com.google.ortools.sat.RoutesConstraintProtoOrBuilder getRoutesOrBuilder();
/**
*
* The circuit_covering constraint is similar to the circuit constraint,
* but allows multiple non-overlapping cycles instead of just one.
*
*
* .operations_research.sat.CircuitCoveringConstraintProto circuit_covering = 25;
* @return Whether the circuitCovering field is set.
*/
boolean hasCircuitCovering();
/**
*
* The circuit_covering constraint is similar to the circuit constraint,
* but allows multiple non-overlapping cycles instead of just one.
*
*
* .operations_research.sat.CircuitCoveringConstraintProto circuit_covering = 25;
* @return The circuitCovering.
*/
com.google.ortools.sat.CircuitCoveringConstraintProto getCircuitCovering();
/**
*
* The circuit_covering constraint is similar to the circuit constraint,
* but allows multiple non-overlapping cycles instead of just one.
*
*
* .operations_research.sat.CircuitCoveringConstraintProto circuit_covering = 25;
*/
com.google.ortools.sat.CircuitCoveringConstraintProtoOrBuilder getCircuitCoveringOrBuilder();
/**
*
* The table constraint enforces what values a tuple of variables may
* take.
*
*
* .operations_research.sat.TableConstraintProto table = 16;
* @return Whether the table field is set.
*/
boolean hasTable();
/**
*
* The table constraint enforces what values a tuple of variables may
* take.
*
*
* .operations_research.sat.TableConstraintProto table = 16;
* @return The table.
*/
com.google.ortools.sat.TableConstraintProto getTable();
/**
*
* The table constraint enforces what values a tuple of variables may
* take.
*
*
* .operations_research.sat.TableConstraintProto table = 16;
*/
com.google.ortools.sat.TableConstraintProtoOrBuilder getTableOrBuilder();
/**
*
* The automaton constraint forces a sequence of variables to be accepted
* by an automaton.
*
*
* .operations_research.sat.AutomatonConstraintProto automaton = 17;
* @return Whether the automaton field is set.
*/
boolean hasAutomaton();
/**
*
* The automaton constraint forces a sequence of variables to be accepted
* by an automaton.
*
*
* .operations_research.sat.AutomatonConstraintProto automaton = 17;
* @return The automaton.
*/
com.google.ortools.sat.AutomatonConstraintProto getAutomaton();
/**
*
* The automaton constraint forces a sequence of variables to be accepted
* by an automaton.
*
*
* .operations_research.sat.AutomatonConstraintProto automaton = 17;
*/
com.google.ortools.sat.AutomatonConstraintProtoOrBuilder getAutomatonOrBuilder();
/**
*
* The inverse constraint forces two arrays to be inverses of each other:
* the values of one are the indices of the other, and vice versa.
*
*
* .operations_research.sat.InverseConstraintProto inverse = 18;
* @return Whether the inverse field is set.
*/
boolean hasInverse();
/**
*
* The inverse constraint forces two arrays to be inverses of each other:
* the values of one are the indices of the other, and vice versa.
*
*
* .operations_research.sat.InverseConstraintProto inverse = 18;
* @return The inverse.
*/
com.google.ortools.sat.InverseConstraintProto getInverse();
/**
*
* The inverse constraint forces two arrays to be inverses of each other:
* the values of one are the indices of the other, and vice versa.
*
*
* .operations_research.sat.InverseConstraintProto inverse = 18;
*/
com.google.ortools.sat.InverseConstraintProtoOrBuilder getInverseOrBuilder();
/**
*
* The reservoir constraint forces the sum of a set of active demands
* to always be between a specified minimum and maximum value during
* specific times.
*
*
* .operations_research.sat.ReservoirConstraintProto reservoir = 24;
* @return Whether the reservoir field is set.
*/
boolean hasReservoir();
/**
*
* The reservoir constraint forces the sum of a set of active demands
* to always be between a specified minimum and maximum value during
* specific times.
*
*
* .operations_research.sat.ReservoirConstraintProto reservoir = 24;
* @return The reservoir.
*/
com.google.ortools.sat.ReservoirConstraintProto getReservoir();
/**
*
* The reservoir constraint forces the sum of a set of active demands
* to always be between a specified minimum and maximum value during
* specific times.
*
*
* .operations_research.sat.ReservoirConstraintProto reservoir = 24;
*/
com.google.ortools.sat.ReservoirConstraintProtoOrBuilder getReservoirOrBuilder();
/**
*
* The interval constraint takes a start, end, and size, and forces
* start + size == end.
*
*
* .operations_research.sat.IntervalConstraintProto interval = 19;
* @return Whether the interval field is set.
*/
boolean hasInterval();
/**
*
* The interval constraint takes a start, end, and size, and forces
* start + size == end.
*
*
* .operations_research.sat.IntervalConstraintProto interval = 19;
* @return The interval.
*/
com.google.ortools.sat.IntervalConstraintProto getInterval();
/**
*
* The interval constraint takes a start, end, and size, and forces
* start + size == end.
*
*
* .operations_research.sat.IntervalConstraintProto interval = 19;
*/
com.google.ortools.sat.IntervalConstraintProtoOrBuilder getIntervalOrBuilder();
/**
*
* The no_overlap constraint prevents a set of intervals from
* overlapping; in scheduling, this is called a disjunctive
* constraint.
*
*
* .operations_research.sat.NoOverlapConstraintProto no_overlap = 20;
* @return Whether the noOverlap field is set.
*/
boolean hasNoOverlap();
/**
*
* The no_overlap constraint prevents a set of intervals from
* overlapping; in scheduling, this is called a disjunctive
* constraint.
*
*
* .operations_research.sat.NoOverlapConstraintProto no_overlap = 20;
* @return The noOverlap.
*/
com.google.ortools.sat.NoOverlapConstraintProto getNoOverlap();
/**
*
* The no_overlap constraint prevents a set of intervals from
* overlapping; in scheduling, this is called a disjunctive
* constraint.
*
*
* .operations_research.sat.NoOverlapConstraintProto no_overlap = 20;
*/
com.google.ortools.sat.NoOverlapConstraintProtoOrBuilder getNoOverlapOrBuilder();
/**
*
* The no_overlap_2d constraint prevents a set of boxes from overlapping.
*
*
* .operations_research.sat.NoOverlap2DConstraintProto no_overlap_2d = 21;
* @return Whether the noOverlap2d field is set.
*/
boolean hasNoOverlap2D();
/**
*
* The no_overlap_2d constraint prevents a set of boxes from overlapping.
*
*
* .operations_research.sat.NoOverlap2DConstraintProto no_overlap_2d = 21;
* @return The noOverlap2d.
*/
com.google.ortools.sat.NoOverlap2DConstraintProto getNoOverlap2D();
/**
*
* The no_overlap_2d constraint prevents a set of boxes from overlapping.
*
*
* .operations_research.sat.NoOverlap2DConstraintProto no_overlap_2d = 21;
*/
com.google.ortools.sat.NoOverlap2DConstraintProtoOrBuilder getNoOverlap2DOrBuilder();
/**
*
* The cumulative constraint ensures that for any integer point, the sum
* of the demands of the intervals containing that point does not exceed
* the capacity.
*
*
* .operations_research.sat.CumulativeConstraintProto cumulative = 22;
* @return Whether the cumulative field is set.
*/
boolean hasCumulative();
/**
*
* The cumulative constraint ensures that for any integer point, the sum
* of the demands of the intervals containing that point does not exceed
* the capacity.
*
*
* .operations_research.sat.CumulativeConstraintProto cumulative = 22;
* @return The cumulative.
*/
com.google.ortools.sat.CumulativeConstraintProto getCumulative();
/**
*
* The cumulative constraint ensures that for any integer point, the sum
* of the demands of the intervals containing that point does not exceed
* the capacity.
*
*
* .operations_research.sat.CumulativeConstraintProto cumulative = 22;
*/
com.google.ortools.sat.CumulativeConstraintProtoOrBuilder getCumulativeOrBuilder();
public com.google.ortools.sat.ConstraintProto.ConstraintCase getConstraintCase();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy