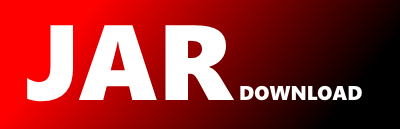
com.google.ortools.sat.CpSolverResponse Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: ortools/sat/cp_model.proto
package com.google.ortools.sat;
/**
*
* The response returned by a solver trying to solve a CpModelProto.
* TODO(user): support returning multiple solutions. Look at the Stubby
* streaming API as we probably wants to get them as they are found.
* Next id: 24
*
*
* Protobuf type {@code operations_research.sat.CpSolverResponse}
*/
public final class CpSolverResponse extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:operations_research.sat.CpSolverResponse)
CpSolverResponseOrBuilder {
private static final long serialVersionUID = 0L;
// Use CpSolverResponse.newBuilder() to construct.
private CpSolverResponse(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CpSolverResponse() {
status_ = 0;
solution_ = emptyLongList();
solutionLowerBounds_ = emptyLongList();
solutionUpperBounds_ = emptyLongList();
tightenedVariables_ = java.util.Collections.emptyList();
sufficientAssumptionsForInfeasibility_ = emptyIntList();
solutionInfo_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CpSolverResponse();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CpSolverResponse(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
status_ = rawValue;
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
solution_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
solution_.addLong(input.readInt64());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) != 0) && input.getBytesUntilLimit() > 0) {
solution_ = newLongList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
solution_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 25: {
objectiveValue_ = input.readDouble();
break;
}
case 33: {
bestObjectiveBound_ = input.readDouble();
break;
}
case 40: {
allSolutionsWereFound_ = input.readBool();
break;
}
case 80: {
numBooleans_ = input.readInt64();
break;
}
case 88: {
numConflicts_ = input.readInt64();
break;
}
case 96: {
numBranches_ = input.readInt64();
break;
}
case 104: {
numBinaryPropagations_ = input.readInt64();
break;
}
case 112: {
numIntegerPropagations_ = input.readInt64();
break;
}
case 121: {
wallTime_ = input.readDouble();
break;
}
case 129: {
userTime_ = input.readDouble();
break;
}
case 137: {
deterministicTime_ = input.readDouble();
break;
}
case 144: {
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
solutionLowerBounds_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
solutionLowerBounds_.addLong(input.readInt64());
break;
}
case 146: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000002) != 0) && input.getBytesUntilLimit() > 0) {
solutionLowerBounds_ = newLongList();
mutable_bitField0_ |= 0x00000002;
}
while (input.getBytesUntilLimit() > 0) {
solutionLowerBounds_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 152: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
solutionUpperBounds_ = newLongList();
mutable_bitField0_ |= 0x00000004;
}
solutionUpperBounds_.addLong(input.readInt64());
break;
}
case 154: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000004) != 0) && input.getBytesUntilLimit() > 0) {
solutionUpperBounds_ = newLongList();
mutable_bitField0_ |= 0x00000004;
}
while (input.getBytesUntilLimit() > 0) {
solutionUpperBounds_.addLong(input.readInt64());
}
input.popLimit(limit);
break;
}
case 162: {
java.lang.String s = input.readStringRequireUtf8();
solutionInfo_ = s;
break;
}
case 170: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
tightenedVariables_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
tightenedVariables_.add(
input.readMessage(com.google.ortools.sat.IntegerVariableProto.parser(), extensionRegistry));
break;
}
case 177: {
primalIntegral_ = input.readDouble();
break;
}
case 184: {
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
sufficientAssumptionsForInfeasibility_ = newIntList();
mutable_bitField0_ |= 0x00000010;
}
sufficientAssumptionsForInfeasibility_.addInt(input.readInt32());
break;
}
case 186: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000010) != 0) && input.getBytesUntilLimit() > 0) {
sufficientAssumptionsForInfeasibility_ = newIntList();
mutable_bitField0_ |= 0x00000010;
}
while (input.getBytesUntilLimit() > 0) {
sufficientAssumptionsForInfeasibility_.addInt(input.readInt32());
}
input.popLimit(limit);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
solution_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
solutionLowerBounds_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
solutionUpperBounds_.makeImmutable(); // C
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
tightenedVariables_ = java.util.Collections.unmodifiableList(tightenedVariables_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
sufficientAssumptionsForInfeasibility_.makeImmutable(); // C
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.sat.CpModelProtobuf.internal_static_operations_research_sat_CpSolverResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.sat.CpModelProtobuf.internal_static_operations_research_sat_CpSolverResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.sat.CpSolverResponse.class, com.google.ortools.sat.CpSolverResponse.Builder.class);
}
public static final int STATUS_FIELD_NUMBER = 1;
private int status_;
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @return The status.
*/
@java.lang.Override public com.google.ortools.sat.CpSolverStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ortools.sat.CpSolverStatus result = com.google.ortools.sat.CpSolverStatus.valueOf(status_);
return result == null ? com.google.ortools.sat.CpSolverStatus.UNRECOGNIZED : result;
}
public static final int SOLUTION_FIELD_NUMBER = 2;
private com.google.protobuf.Internal.LongList solution_;
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @return A list containing the solution.
*/
@java.lang.Override
public java.util.List
getSolutionList() {
return solution_;
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @return The count of solution.
*/
public int getSolutionCount() {
return solution_.size();
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @param index The index of the element to return.
* @return The solution at the given index.
*/
public long getSolution(int index) {
return solution_.getLong(index);
}
private int solutionMemoizedSerializedSize = -1;
public static final int OBJECTIVE_VALUE_FIELD_NUMBER = 3;
private double objectiveValue_;
/**
*
* Only make sense for an optimization problem. The objective value of the
* returned solution if it is non-empty. If there is no solution, then for a
* minimization problem, this will be an upper-bound of the objective of any
* feasible solution, and a lower-bound for a maximization problem.
*
*
* double objective_value = 3;
* @return The objectiveValue.
*/
@java.lang.Override
public double getObjectiveValue() {
return objectiveValue_;
}
public static final int BEST_OBJECTIVE_BOUND_FIELD_NUMBER = 4;
private double bestObjectiveBound_;
/**
*
* Only make sense for an optimization problem. A proven lower-bound on the
* objective for a minimization problem, or a proven upper-bound for a
* maximization problem.
*
*
* double best_objective_bound = 4;
* @return The bestObjectiveBound.
*/
@java.lang.Override
public double getBestObjectiveBound() {
return bestObjectiveBound_;
}
public static final int SOLUTION_LOWER_BOUNDS_FIELD_NUMBER = 18;
private com.google.protobuf.Internal.LongList solutionLowerBounds_;
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @return A list containing the solutionLowerBounds.
*/
@java.lang.Override
public java.util.List
getSolutionLowerBoundsList() {
return solutionLowerBounds_;
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @return The count of solutionLowerBounds.
*/
public int getSolutionLowerBoundsCount() {
return solutionLowerBounds_.size();
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @param index The index of the element to return.
* @return The solutionLowerBounds at the given index.
*/
public long getSolutionLowerBounds(int index) {
return solutionLowerBounds_.getLong(index);
}
private int solutionLowerBoundsMemoizedSerializedSize = -1;
public static final int SOLUTION_UPPER_BOUNDS_FIELD_NUMBER = 19;
private com.google.protobuf.Internal.LongList solutionUpperBounds_;
/**
* repeated int64 solution_upper_bounds = 19;
* @return A list containing the solutionUpperBounds.
*/
@java.lang.Override
public java.util.List
getSolutionUpperBoundsList() {
return solutionUpperBounds_;
}
/**
* repeated int64 solution_upper_bounds = 19;
* @return The count of solutionUpperBounds.
*/
public int getSolutionUpperBoundsCount() {
return solutionUpperBounds_.size();
}
/**
* repeated int64 solution_upper_bounds = 19;
* @param index The index of the element to return.
* @return The solutionUpperBounds at the given index.
*/
public long getSolutionUpperBounds(int index) {
return solutionUpperBounds_.getLong(index);
}
private int solutionUpperBoundsMemoizedSerializedSize = -1;
public static final int TIGHTENED_VARIABLES_FIELD_NUMBER = 21;
private java.util.List tightenedVariables_;
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
@java.lang.Override
public java.util.List getTightenedVariablesList() {
return tightenedVariables_;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
@java.lang.Override
public java.util.List extends com.google.ortools.sat.IntegerVariableProtoOrBuilder>
getTightenedVariablesOrBuilderList() {
return tightenedVariables_;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
@java.lang.Override
public int getTightenedVariablesCount() {
return tightenedVariables_.size();
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
@java.lang.Override
public com.google.ortools.sat.IntegerVariableProto getTightenedVariables(int index) {
return tightenedVariables_.get(index);
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
@java.lang.Override
public com.google.ortools.sat.IntegerVariableProtoOrBuilder getTightenedVariablesOrBuilder(
int index) {
return tightenedVariables_.get(index);
}
public static final int SUFFICIENT_ASSUMPTIONS_FOR_INFEASIBILITY_FIELD_NUMBER = 23;
private com.google.protobuf.Internal.IntList sufficientAssumptionsForInfeasibility_;
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @return A list containing the sufficientAssumptionsForInfeasibility.
*/
@java.lang.Override
public java.util.List
getSufficientAssumptionsForInfeasibilityList() {
return sufficientAssumptionsForInfeasibility_;
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @return The count of sufficientAssumptionsForInfeasibility.
*/
public int getSufficientAssumptionsForInfeasibilityCount() {
return sufficientAssumptionsForInfeasibility_.size();
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @param index The index of the element to return.
* @return The sufficientAssumptionsForInfeasibility at the given index.
*/
public int getSufficientAssumptionsForInfeasibility(int index) {
return sufficientAssumptionsForInfeasibility_.getInt(index);
}
private int sufficientAssumptionsForInfeasibilityMemoizedSerializedSize = -1;
public static final int ALL_SOLUTIONS_WERE_FOUND_FIELD_NUMBER = 5;
private boolean allSolutionsWereFound_;
/**
*
* This will be true iff the solver was asked to find all solutions to a
* satisfiability problem (or all optimal solutions to an optimization
* problem), and it was successful in doing so.
* TODO(user): Remove as we also use the OPTIMAL vs FEASIBLE status for that.
*
*
* bool all_solutions_were_found = 5;
* @return The allSolutionsWereFound.
*/
@java.lang.Override
public boolean getAllSolutionsWereFound() {
return allSolutionsWereFound_;
}
public static final int NUM_BOOLEANS_FIELD_NUMBER = 10;
private long numBooleans_;
/**
*
* Some statistics about the solve.
*
*
* int64 num_booleans = 10;
* @return The numBooleans.
*/
@java.lang.Override
public long getNumBooleans() {
return numBooleans_;
}
public static final int NUM_CONFLICTS_FIELD_NUMBER = 11;
private long numConflicts_;
/**
* int64 num_conflicts = 11;
* @return The numConflicts.
*/
@java.lang.Override
public long getNumConflicts() {
return numConflicts_;
}
public static final int NUM_BRANCHES_FIELD_NUMBER = 12;
private long numBranches_;
/**
* int64 num_branches = 12;
* @return The numBranches.
*/
@java.lang.Override
public long getNumBranches() {
return numBranches_;
}
public static final int NUM_BINARY_PROPAGATIONS_FIELD_NUMBER = 13;
private long numBinaryPropagations_;
/**
* int64 num_binary_propagations = 13;
* @return The numBinaryPropagations.
*/
@java.lang.Override
public long getNumBinaryPropagations() {
return numBinaryPropagations_;
}
public static final int NUM_INTEGER_PROPAGATIONS_FIELD_NUMBER = 14;
private long numIntegerPropagations_;
/**
* int64 num_integer_propagations = 14;
* @return The numIntegerPropagations.
*/
@java.lang.Override
public long getNumIntegerPropagations() {
return numIntegerPropagations_;
}
public static final int WALL_TIME_FIELD_NUMBER = 15;
private double wallTime_;
/**
* double wall_time = 15;
* @return The wallTime.
*/
@java.lang.Override
public double getWallTime() {
return wallTime_;
}
public static final int USER_TIME_FIELD_NUMBER = 16;
private double userTime_;
/**
* double user_time = 16;
* @return The userTime.
*/
@java.lang.Override
public double getUserTime() {
return userTime_;
}
public static final int DETERMINISTIC_TIME_FIELD_NUMBER = 17;
private double deterministicTime_;
/**
* double deterministic_time = 17;
* @return The deterministicTime.
*/
@java.lang.Override
public double getDeterministicTime() {
return deterministicTime_;
}
public static final int PRIMAL_INTEGRAL_FIELD_NUMBER = 22;
private double primalIntegral_;
/**
* double primal_integral = 22;
* @return The primalIntegral.
*/
@java.lang.Override
public double getPrimalIntegral() {
return primalIntegral_;
}
public static final int SOLUTION_INFO_FIELD_NUMBER = 20;
private volatile java.lang.Object solutionInfo_;
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @return The solutionInfo.
*/
@java.lang.Override
public java.lang.String getSolutionInfo() {
java.lang.Object ref = solutionInfo_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
solutionInfo_ = s;
return s;
}
}
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @return The bytes for solutionInfo.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSolutionInfoBytes() {
java.lang.Object ref = solutionInfo_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
solutionInfo_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (status_ != com.google.ortools.sat.CpSolverStatus.UNKNOWN.getNumber()) {
output.writeEnum(1, status_);
}
if (getSolutionList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(solutionMemoizedSerializedSize);
}
for (int i = 0; i < solution_.size(); i++) {
output.writeInt64NoTag(solution_.getLong(i));
}
if (objectiveValue_ != 0D) {
output.writeDouble(3, objectiveValue_);
}
if (bestObjectiveBound_ != 0D) {
output.writeDouble(4, bestObjectiveBound_);
}
if (allSolutionsWereFound_ != false) {
output.writeBool(5, allSolutionsWereFound_);
}
if (numBooleans_ != 0L) {
output.writeInt64(10, numBooleans_);
}
if (numConflicts_ != 0L) {
output.writeInt64(11, numConflicts_);
}
if (numBranches_ != 0L) {
output.writeInt64(12, numBranches_);
}
if (numBinaryPropagations_ != 0L) {
output.writeInt64(13, numBinaryPropagations_);
}
if (numIntegerPropagations_ != 0L) {
output.writeInt64(14, numIntegerPropagations_);
}
if (wallTime_ != 0D) {
output.writeDouble(15, wallTime_);
}
if (userTime_ != 0D) {
output.writeDouble(16, userTime_);
}
if (deterministicTime_ != 0D) {
output.writeDouble(17, deterministicTime_);
}
if (getSolutionLowerBoundsList().size() > 0) {
output.writeUInt32NoTag(146);
output.writeUInt32NoTag(solutionLowerBoundsMemoizedSerializedSize);
}
for (int i = 0; i < solutionLowerBounds_.size(); i++) {
output.writeInt64NoTag(solutionLowerBounds_.getLong(i));
}
if (getSolutionUpperBoundsList().size() > 0) {
output.writeUInt32NoTag(154);
output.writeUInt32NoTag(solutionUpperBoundsMemoizedSerializedSize);
}
for (int i = 0; i < solutionUpperBounds_.size(); i++) {
output.writeInt64NoTag(solutionUpperBounds_.getLong(i));
}
if (!getSolutionInfoBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 20, solutionInfo_);
}
for (int i = 0; i < tightenedVariables_.size(); i++) {
output.writeMessage(21, tightenedVariables_.get(i));
}
if (primalIntegral_ != 0D) {
output.writeDouble(22, primalIntegral_);
}
if (getSufficientAssumptionsForInfeasibilityList().size() > 0) {
output.writeUInt32NoTag(186);
output.writeUInt32NoTag(sufficientAssumptionsForInfeasibilityMemoizedSerializedSize);
}
for (int i = 0; i < sufficientAssumptionsForInfeasibility_.size(); i++) {
output.writeInt32NoTag(sufficientAssumptionsForInfeasibility_.getInt(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (status_ != com.google.ortools.sat.CpSolverStatus.UNKNOWN.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, status_);
}
{
int dataSize = 0;
for (int i = 0; i < solution_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(solution_.getLong(i));
}
size += dataSize;
if (!getSolutionList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
solutionMemoizedSerializedSize = dataSize;
}
if (objectiveValue_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(3, objectiveValue_);
}
if (bestObjectiveBound_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(4, bestObjectiveBound_);
}
if (allSolutionsWereFound_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, allSolutionsWereFound_);
}
if (numBooleans_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, numBooleans_);
}
if (numConflicts_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, numConflicts_);
}
if (numBranches_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, numBranches_);
}
if (numBinaryPropagations_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, numBinaryPropagations_);
}
if (numIntegerPropagations_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(14, numIntegerPropagations_);
}
if (wallTime_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(15, wallTime_);
}
if (userTime_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(16, userTime_);
}
if (deterministicTime_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(17, deterministicTime_);
}
{
int dataSize = 0;
for (int i = 0; i < solutionLowerBounds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(solutionLowerBounds_.getLong(i));
}
size += dataSize;
if (!getSolutionLowerBoundsList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
solutionLowerBoundsMemoizedSerializedSize = dataSize;
}
{
int dataSize = 0;
for (int i = 0; i < solutionUpperBounds_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt64SizeNoTag(solutionUpperBounds_.getLong(i));
}
size += dataSize;
if (!getSolutionUpperBoundsList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
solutionUpperBoundsMemoizedSerializedSize = dataSize;
}
if (!getSolutionInfoBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(20, solutionInfo_);
}
for (int i = 0; i < tightenedVariables_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(21, tightenedVariables_.get(i));
}
if (primalIntegral_ != 0D) {
size += com.google.protobuf.CodedOutputStream
.computeDoubleSize(22, primalIntegral_);
}
{
int dataSize = 0;
for (int i = 0; i < sufficientAssumptionsForInfeasibility_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(sufficientAssumptionsForInfeasibility_.getInt(i));
}
size += dataSize;
if (!getSufficientAssumptionsForInfeasibilityList().isEmpty()) {
size += 2;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
sufficientAssumptionsForInfeasibilityMemoizedSerializedSize = dataSize;
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof com.google.ortools.sat.CpSolverResponse)) {
return super.equals(obj);
}
com.google.ortools.sat.CpSolverResponse other = (com.google.ortools.sat.CpSolverResponse) obj;
if (status_ != other.status_) return false;
if (!getSolutionList()
.equals(other.getSolutionList())) return false;
if (java.lang.Double.doubleToLongBits(getObjectiveValue())
!= java.lang.Double.doubleToLongBits(
other.getObjectiveValue())) return false;
if (java.lang.Double.doubleToLongBits(getBestObjectiveBound())
!= java.lang.Double.doubleToLongBits(
other.getBestObjectiveBound())) return false;
if (!getSolutionLowerBoundsList()
.equals(other.getSolutionLowerBoundsList())) return false;
if (!getSolutionUpperBoundsList()
.equals(other.getSolutionUpperBoundsList())) return false;
if (!getTightenedVariablesList()
.equals(other.getTightenedVariablesList())) return false;
if (!getSufficientAssumptionsForInfeasibilityList()
.equals(other.getSufficientAssumptionsForInfeasibilityList())) return false;
if (getAllSolutionsWereFound()
!= other.getAllSolutionsWereFound()) return false;
if (getNumBooleans()
!= other.getNumBooleans()) return false;
if (getNumConflicts()
!= other.getNumConflicts()) return false;
if (getNumBranches()
!= other.getNumBranches()) return false;
if (getNumBinaryPropagations()
!= other.getNumBinaryPropagations()) return false;
if (getNumIntegerPropagations()
!= other.getNumIntegerPropagations()) return false;
if (java.lang.Double.doubleToLongBits(getWallTime())
!= java.lang.Double.doubleToLongBits(
other.getWallTime())) return false;
if (java.lang.Double.doubleToLongBits(getUserTime())
!= java.lang.Double.doubleToLongBits(
other.getUserTime())) return false;
if (java.lang.Double.doubleToLongBits(getDeterministicTime())
!= java.lang.Double.doubleToLongBits(
other.getDeterministicTime())) return false;
if (java.lang.Double.doubleToLongBits(getPrimalIntegral())
!= java.lang.Double.doubleToLongBits(
other.getPrimalIntegral())) return false;
if (!getSolutionInfo()
.equals(other.getSolutionInfo())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + STATUS_FIELD_NUMBER;
hash = (53 * hash) + status_;
if (getSolutionCount() > 0) {
hash = (37 * hash) + SOLUTION_FIELD_NUMBER;
hash = (53 * hash) + getSolutionList().hashCode();
}
hash = (37 * hash) + OBJECTIVE_VALUE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getObjectiveValue()));
hash = (37 * hash) + BEST_OBJECTIVE_BOUND_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getBestObjectiveBound()));
if (getSolutionLowerBoundsCount() > 0) {
hash = (37 * hash) + SOLUTION_LOWER_BOUNDS_FIELD_NUMBER;
hash = (53 * hash) + getSolutionLowerBoundsList().hashCode();
}
if (getSolutionUpperBoundsCount() > 0) {
hash = (37 * hash) + SOLUTION_UPPER_BOUNDS_FIELD_NUMBER;
hash = (53 * hash) + getSolutionUpperBoundsList().hashCode();
}
if (getTightenedVariablesCount() > 0) {
hash = (37 * hash) + TIGHTENED_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + getTightenedVariablesList().hashCode();
}
if (getSufficientAssumptionsForInfeasibilityCount() > 0) {
hash = (37 * hash) + SUFFICIENT_ASSUMPTIONS_FOR_INFEASIBILITY_FIELD_NUMBER;
hash = (53 * hash) + getSufficientAssumptionsForInfeasibilityList().hashCode();
}
hash = (37 * hash) + ALL_SOLUTIONS_WERE_FOUND_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getAllSolutionsWereFound());
hash = (37 * hash) + NUM_BOOLEANS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumBooleans());
hash = (37 * hash) + NUM_CONFLICTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumConflicts());
hash = (37 * hash) + NUM_BRANCHES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumBranches());
hash = (37 * hash) + NUM_BINARY_PROPAGATIONS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumBinaryPropagations());
hash = (37 * hash) + NUM_INTEGER_PROPAGATIONS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumIntegerPropagations());
hash = (37 * hash) + WALL_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getWallTime()));
hash = (37 * hash) + USER_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getUserTime()));
hash = (37 * hash) + DETERMINISTIC_TIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getDeterministicTime()));
hash = (37 * hash) + PRIMAL_INTEGRAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
java.lang.Double.doubleToLongBits(getPrimalIntegral()));
hash = (37 * hash) + SOLUTION_INFO_FIELD_NUMBER;
hash = (53 * hash) + getSolutionInfo().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.sat.CpSolverResponse parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.ortools.sat.CpSolverResponse parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static com.google.ortools.sat.CpSolverResponse parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.ortools.sat.CpSolverResponse prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* The response returned by a solver trying to solve a CpModelProto.
* TODO(user): support returning multiple solutions. Look at the Stubby
* streaming API as we probably wants to get them as they are found.
* Next id: 24
*
*
* Protobuf type {@code operations_research.sat.CpSolverResponse}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:operations_research.sat.CpSolverResponse)
com.google.ortools.sat.CpSolverResponseOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.ortools.sat.CpModelProtobuf.internal_static_operations_research_sat_CpSolverResponse_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.ortools.sat.CpModelProtobuf.internal_static_operations_research_sat_CpSolverResponse_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.ortools.sat.CpSolverResponse.class, com.google.ortools.sat.CpSolverResponse.Builder.class);
}
// Construct using com.google.ortools.sat.CpSolverResponse.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getTightenedVariablesFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
status_ = 0;
solution_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
objectiveValue_ = 0D;
bestObjectiveBound_ = 0D;
solutionLowerBounds_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
solutionUpperBounds_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000004);
if (tightenedVariablesBuilder_ == null) {
tightenedVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
tightenedVariablesBuilder_.clear();
}
sufficientAssumptionsForInfeasibility_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000010);
allSolutionsWereFound_ = false;
numBooleans_ = 0L;
numConflicts_ = 0L;
numBranches_ = 0L;
numBinaryPropagations_ = 0L;
numIntegerPropagations_ = 0L;
wallTime_ = 0D;
userTime_ = 0D;
deterministicTime_ = 0D;
primalIntegral_ = 0D;
solutionInfo_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.ortools.sat.CpModelProtobuf.internal_static_operations_research_sat_CpSolverResponse_descriptor;
}
@java.lang.Override
public com.google.ortools.sat.CpSolverResponse getDefaultInstanceForType() {
return com.google.ortools.sat.CpSolverResponse.getDefaultInstance();
}
@java.lang.Override
public com.google.ortools.sat.CpSolverResponse build() {
com.google.ortools.sat.CpSolverResponse result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public com.google.ortools.sat.CpSolverResponse buildPartial() {
com.google.ortools.sat.CpSolverResponse result = new com.google.ortools.sat.CpSolverResponse(this);
int from_bitField0_ = bitField0_;
result.status_ = status_;
if (((bitField0_ & 0x00000001) != 0)) {
solution_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.solution_ = solution_;
result.objectiveValue_ = objectiveValue_;
result.bestObjectiveBound_ = bestObjectiveBound_;
if (((bitField0_ & 0x00000002) != 0)) {
solutionLowerBounds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.solutionLowerBounds_ = solutionLowerBounds_;
if (((bitField0_ & 0x00000004) != 0)) {
solutionUpperBounds_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.solutionUpperBounds_ = solutionUpperBounds_;
if (tightenedVariablesBuilder_ == null) {
if (((bitField0_ & 0x00000008) != 0)) {
tightenedVariables_ = java.util.Collections.unmodifiableList(tightenedVariables_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.tightenedVariables_ = tightenedVariables_;
} else {
result.tightenedVariables_ = tightenedVariablesBuilder_.build();
}
if (((bitField0_ & 0x00000010) != 0)) {
sufficientAssumptionsForInfeasibility_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.sufficientAssumptionsForInfeasibility_ = sufficientAssumptionsForInfeasibility_;
result.allSolutionsWereFound_ = allSolutionsWereFound_;
result.numBooleans_ = numBooleans_;
result.numConflicts_ = numConflicts_;
result.numBranches_ = numBranches_;
result.numBinaryPropagations_ = numBinaryPropagations_;
result.numIntegerPropagations_ = numIntegerPropagations_;
result.wallTime_ = wallTime_;
result.userTime_ = userTime_;
result.deterministicTime_ = deterministicTime_;
result.primalIntegral_ = primalIntegral_;
result.solutionInfo_ = solutionInfo_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.ortools.sat.CpSolverResponse) {
return mergeFrom((com.google.ortools.sat.CpSolverResponse)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.ortools.sat.CpSolverResponse other) {
if (other == com.google.ortools.sat.CpSolverResponse.getDefaultInstance()) return this;
if (other.status_ != 0) {
setStatusValue(other.getStatusValue());
}
if (!other.solution_.isEmpty()) {
if (solution_.isEmpty()) {
solution_ = other.solution_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSolutionIsMutable();
solution_.addAll(other.solution_);
}
onChanged();
}
if (other.getObjectiveValue() != 0D) {
setObjectiveValue(other.getObjectiveValue());
}
if (other.getBestObjectiveBound() != 0D) {
setBestObjectiveBound(other.getBestObjectiveBound());
}
if (!other.solutionLowerBounds_.isEmpty()) {
if (solutionLowerBounds_.isEmpty()) {
solutionLowerBounds_ = other.solutionLowerBounds_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSolutionLowerBoundsIsMutable();
solutionLowerBounds_.addAll(other.solutionLowerBounds_);
}
onChanged();
}
if (!other.solutionUpperBounds_.isEmpty()) {
if (solutionUpperBounds_.isEmpty()) {
solutionUpperBounds_ = other.solutionUpperBounds_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSolutionUpperBoundsIsMutable();
solutionUpperBounds_.addAll(other.solutionUpperBounds_);
}
onChanged();
}
if (tightenedVariablesBuilder_ == null) {
if (!other.tightenedVariables_.isEmpty()) {
if (tightenedVariables_.isEmpty()) {
tightenedVariables_ = other.tightenedVariables_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureTightenedVariablesIsMutable();
tightenedVariables_.addAll(other.tightenedVariables_);
}
onChanged();
}
} else {
if (!other.tightenedVariables_.isEmpty()) {
if (tightenedVariablesBuilder_.isEmpty()) {
tightenedVariablesBuilder_.dispose();
tightenedVariablesBuilder_ = null;
tightenedVariables_ = other.tightenedVariables_;
bitField0_ = (bitField0_ & ~0x00000008);
tightenedVariablesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getTightenedVariablesFieldBuilder() : null;
} else {
tightenedVariablesBuilder_.addAllMessages(other.tightenedVariables_);
}
}
}
if (!other.sufficientAssumptionsForInfeasibility_.isEmpty()) {
if (sufficientAssumptionsForInfeasibility_.isEmpty()) {
sufficientAssumptionsForInfeasibility_ = other.sufficientAssumptionsForInfeasibility_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureSufficientAssumptionsForInfeasibilityIsMutable();
sufficientAssumptionsForInfeasibility_.addAll(other.sufficientAssumptionsForInfeasibility_);
}
onChanged();
}
if (other.getAllSolutionsWereFound() != false) {
setAllSolutionsWereFound(other.getAllSolutionsWereFound());
}
if (other.getNumBooleans() != 0L) {
setNumBooleans(other.getNumBooleans());
}
if (other.getNumConflicts() != 0L) {
setNumConflicts(other.getNumConflicts());
}
if (other.getNumBranches() != 0L) {
setNumBranches(other.getNumBranches());
}
if (other.getNumBinaryPropagations() != 0L) {
setNumBinaryPropagations(other.getNumBinaryPropagations());
}
if (other.getNumIntegerPropagations() != 0L) {
setNumIntegerPropagations(other.getNumIntegerPropagations());
}
if (other.getWallTime() != 0D) {
setWallTime(other.getWallTime());
}
if (other.getUserTime() != 0D) {
setUserTime(other.getUserTime());
}
if (other.getDeterministicTime() != 0D) {
setDeterministicTime(other.getDeterministicTime());
}
if (other.getPrimalIntegral() != 0D) {
setPrimalIntegral(other.getPrimalIntegral());
}
if (!other.getSolutionInfo().isEmpty()) {
solutionInfo_ = other.solutionInfo_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.ortools.sat.CpSolverResponse parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.ortools.sat.CpSolverResponse) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int status_ = 0;
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @return The enum numeric value on the wire for status.
*/
@java.lang.Override public int getStatusValue() {
return status_;
}
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @param value The enum numeric value on the wire for status to set.
* @return This builder for chaining.
*/
public Builder setStatusValue(int value) {
status_ = value;
onChanged();
return this;
}
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @return The status.
*/
@java.lang.Override
public com.google.ortools.sat.CpSolverStatus getStatus() {
@SuppressWarnings("deprecation")
com.google.ortools.sat.CpSolverStatus result = com.google.ortools.sat.CpSolverStatus.valueOf(status_);
return result == null ? com.google.ortools.sat.CpSolverStatus.UNRECOGNIZED : result;
}
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @param value The status to set.
* @return This builder for chaining.
*/
public Builder setStatus(com.google.ortools.sat.CpSolverStatus value) {
if (value == null) {
throw new NullPointerException();
}
status_ = value.getNumber();
onChanged();
return this;
}
/**
*
* The status of the solve.
*
*
* .operations_research.sat.CpSolverStatus status = 1;
* @return This builder for chaining.
*/
public Builder clearStatus() {
status_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList solution_ = emptyLongList();
private void ensureSolutionIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
solution_ = mutableCopy(solution_);
bitField0_ |= 0x00000001;
}
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @return A list containing the solution.
*/
public java.util.List
getSolutionList() {
return ((bitField0_ & 0x00000001) != 0) ?
java.util.Collections.unmodifiableList(solution_) : solution_;
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @return The count of solution.
*/
public int getSolutionCount() {
return solution_.size();
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @param index The index of the element to return.
* @return The solution at the given index.
*/
public long getSolution(int index) {
return solution_.getLong(index);
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @param index The index to set the value at.
* @param value The solution to set.
* @return This builder for chaining.
*/
public Builder setSolution(
int index, long value) {
ensureSolutionIsMutable();
solution_.setLong(index, value);
onChanged();
return this;
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @param value The solution to add.
* @return This builder for chaining.
*/
public Builder addSolution(long value) {
ensureSolutionIsMutable();
solution_.addLong(value);
onChanged();
return this;
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @param values The solution to add.
* @return This builder for chaining.
*/
public Builder addAllSolution(
java.lang.Iterable extends java.lang.Long> values) {
ensureSolutionIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, solution_);
onChanged();
return this;
}
/**
*
* A feasible solution to the given problem. Depending on the returned status
* it may be optimal or just feasible. This is in one-to-one correspondence
* with a CpModelProto::variables repeated field and list the values of all
* the variables.
*
*
* repeated int64 solution = 2;
* @return This builder for chaining.
*/
public Builder clearSolution() {
solution_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private double objectiveValue_ ;
/**
*
* Only make sense for an optimization problem. The objective value of the
* returned solution if it is non-empty. If there is no solution, then for a
* minimization problem, this will be an upper-bound of the objective of any
* feasible solution, and a lower-bound for a maximization problem.
*
*
* double objective_value = 3;
* @return The objectiveValue.
*/
@java.lang.Override
public double getObjectiveValue() {
return objectiveValue_;
}
/**
*
* Only make sense for an optimization problem. The objective value of the
* returned solution if it is non-empty. If there is no solution, then for a
* minimization problem, this will be an upper-bound of the objective of any
* feasible solution, and a lower-bound for a maximization problem.
*
*
* double objective_value = 3;
* @param value The objectiveValue to set.
* @return This builder for chaining.
*/
public Builder setObjectiveValue(double value) {
objectiveValue_ = value;
onChanged();
return this;
}
/**
*
* Only make sense for an optimization problem. The objective value of the
* returned solution if it is non-empty. If there is no solution, then for a
* minimization problem, this will be an upper-bound of the objective of any
* feasible solution, and a lower-bound for a maximization problem.
*
*
* double objective_value = 3;
* @return This builder for chaining.
*/
public Builder clearObjectiveValue() {
objectiveValue_ = 0D;
onChanged();
return this;
}
private double bestObjectiveBound_ ;
/**
*
* Only make sense for an optimization problem. A proven lower-bound on the
* objective for a minimization problem, or a proven upper-bound for a
* maximization problem.
*
*
* double best_objective_bound = 4;
* @return The bestObjectiveBound.
*/
@java.lang.Override
public double getBestObjectiveBound() {
return bestObjectiveBound_;
}
/**
*
* Only make sense for an optimization problem. A proven lower-bound on the
* objective for a minimization problem, or a proven upper-bound for a
* maximization problem.
*
*
* double best_objective_bound = 4;
* @param value The bestObjectiveBound to set.
* @return This builder for chaining.
*/
public Builder setBestObjectiveBound(double value) {
bestObjectiveBound_ = value;
onChanged();
return this;
}
/**
*
* Only make sense for an optimization problem. A proven lower-bound on the
* objective for a minimization problem, or a proven upper-bound for a
* maximization problem.
*
*
* double best_objective_bound = 4;
* @return This builder for chaining.
*/
public Builder clearBestObjectiveBound() {
bestObjectiveBound_ = 0D;
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList solutionLowerBounds_ = emptyLongList();
private void ensureSolutionLowerBoundsIsMutable() {
if (!((bitField0_ & 0x00000002) != 0)) {
solutionLowerBounds_ = mutableCopy(solutionLowerBounds_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @return A list containing the solutionLowerBounds.
*/
public java.util.List
getSolutionLowerBoundsList() {
return ((bitField0_ & 0x00000002) != 0) ?
java.util.Collections.unmodifiableList(solutionLowerBounds_) : solutionLowerBounds_;
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @return The count of solutionLowerBounds.
*/
public int getSolutionLowerBoundsCount() {
return solutionLowerBounds_.size();
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @param index The index of the element to return.
* @return The solutionLowerBounds at the given index.
*/
public long getSolutionLowerBounds(int index) {
return solutionLowerBounds_.getLong(index);
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @param index The index to set the value at.
* @param value The solutionLowerBounds to set.
* @return This builder for chaining.
*/
public Builder setSolutionLowerBounds(
int index, long value) {
ensureSolutionLowerBoundsIsMutable();
solutionLowerBounds_.setLong(index, value);
onChanged();
return this;
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @param value The solutionLowerBounds to add.
* @return This builder for chaining.
*/
public Builder addSolutionLowerBounds(long value) {
ensureSolutionLowerBoundsIsMutable();
solutionLowerBounds_.addLong(value);
onChanged();
return this;
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @param values The solutionLowerBounds to add.
* @return This builder for chaining.
*/
public Builder addAllSolutionLowerBounds(
java.lang.Iterable extends java.lang.Long> values) {
ensureSolutionLowerBoundsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, solutionLowerBounds_);
onChanged();
return this;
}
/**
*
* Advanced usage.
* If the problem has some variables that are not fixed at the end of the
* search (because of a particular search strategy in the CpModelProto) then
* this will be used instead of filling the solution above. The two fields
* will then contains the lower and upper bounds of each variable as they were
* when the best "solution" was found.
*
*
* repeated int64 solution_lower_bounds = 18;
* @return This builder for chaining.
*/
public Builder clearSolutionLowerBounds() {
solutionLowerBounds_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
private com.google.protobuf.Internal.LongList solutionUpperBounds_ = emptyLongList();
private void ensureSolutionUpperBoundsIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
solutionUpperBounds_ = mutableCopy(solutionUpperBounds_);
bitField0_ |= 0x00000004;
}
}
/**
* repeated int64 solution_upper_bounds = 19;
* @return A list containing the solutionUpperBounds.
*/
public java.util.List
getSolutionUpperBoundsList() {
return ((bitField0_ & 0x00000004) != 0) ?
java.util.Collections.unmodifiableList(solutionUpperBounds_) : solutionUpperBounds_;
}
/**
* repeated int64 solution_upper_bounds = 19;
* @return The count of solutionUpperBounds.
*/
public int getSolutionUpperBoundsCount() {
return solutionUpperBounds_.size();
}
/**
* repeated int64 solution_upper_bounds = 19;
* @param index The index of the element to return.
* @return The solutionUpperBounds at the given index.
*/
public long getSolutionUpperBounds(int index) {
return solutionUpperBounds_.getLong(index);
}
/**
* repeated int64 solution_upper_bounds = 19;
* @param index The index to set the value at.
* @param value The solutionUpperBounds to set.
* @return This builder for chaining.
*/
public Builder setSolutionUpperBounds(
int index, long value) {
ensureSolutionUpperBoundsIsMutable();
solutionUpperBounds_.setLong(index, value);
onChanged();
return this;
}
/**
* repeated int64 solution_upper_bounds = 19;
* @param value The solutionUpperBounds to add.
* @return This builder for chaining.
*/
public Builder addSolutionUpperBounds(long value) {
ensureSolutionUpperBoundsIsMutable();
solutionUpperBounds_.addLong(value);
onChanged();
return this;
}
/**
* repeated int64 solution_upper_bounds = 19;
* @param values The solutionUpperBounds to add.
* @return This builder for chaining.
*/
public Builder addAllSolutionUpperBounds(
java.lang.Iterable extends java.lang.Long> values) {
ensureSolutionUpperBoundsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, solutionUpperBounds_);
onChanged();
return this;
}
/**
* repeated int64 solution_upper_bounds = 19;
* @return This builder for chaining.
*/
public Builder clearSolutionUpperBounds() {
solutionUpperBounds_ = emptyLongList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
private java.util.List tightenedVariables_ =
java.util.Collections.emptyList();
private void ensureTightenedVariablesIsMutable() {
if (!((bitField0_ & 0x00000008) != 0)) {
tightenedVariables_ = new java.util.ArrayList(tightenedVariables_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.sat.IntegerVariableProto, com.google.ortools.sat.IntegerVariableProto.Builder, com.google.ortools.sat.IntegerVariableProtoOrBuilder> tightenedVariablesBuilder_;
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public java.util.List getTightenedVariablesList() {
if (tightenedVariablesBuilder_ == null) {
return java.util.Collections.unmodifiableList(tightenedVariables_);
} else {
return tightenedVariablesBuilder_.getMessageList();
}
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public int getTightenedVariablesCount() {
if (tightenedVariablesBuilder_ == null) {
return tightenedVariables_.size();
} else {
return tightenedVariablesBuilder_.getCount();
}
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public com.google.ortools.sat.IntegerVariableProto getTightenedVariables(int index) {
if (tightenedVariablesBuilder_ == null) {
return tightenedVariables_.get(index);
} else {
return tightenedVariablesBuilder_.getMessage(index);
}
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder setTightenedVariables(
int index, com.google.ortools.sat.IntegerVariableProto value) {
if (tightenedVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTightenedVariablesIsMutable();
tightenedVariables_.set(index, value);
onChanged();
} else {
tightenedVariablesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder setTightenedVariables(
int index, com.google.ortools.sat.IntegerVariableProto.Builder builderForValue) {
if (tightenedVariablesBuilder_ == null) {
ensureTightenedVariablesIsMutable();
tightenedVariables_.set(index, builderForValue.build());
onChanged();
} else {
tightenedVariablesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder addTightenedVariables(com.google.ortools.sat.IntegerVariableProto value) {
if (tightenedVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTightenedVariablesIsMutable();
tightenedVariables_.add(value);
onChanged();
} else {
tightenedVariablesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder addTightenedVariables(
int index, com.google.ortools.sat.IntegerVariableProto value) {
if (tightenedVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureTightenedVariablesIsMutable();
tightenedVariables_.add(index, value);
onChanged();
} else {
tightenedVariablesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder addTightenedVariables(
com.google.ortools.sat.IntegerVariableProto.Builder builderForValue) {
if (tightenedVariablesBuilder_ == null) {
ensureTightenedVariablesIsMutable();
tightenedVariables_.add(builderForValue.build());
onChanged();
} else {
tightenedVariablesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder addTightenedVariables(
int index, com.google.ortools.sat.IntegerVariableProto.Builder builderForValue) {
if (tightenedVariablesBuilder_ == null) {
ensureTightenedVariablesIsMutable();
tightenedVariables_.add(index, builderForValue.build());
onChanged();
} else {
tightenedVariablesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder addAllTightenedVariables(
java.lang.Iterable extends com.google.ortools.sat.IntegerVariableProto> values) {
if (tightenedVariablesBuilder_ == null) {
ensureTightenedVariablesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, tightenedVariables_);
onChanged();
} else {
tightenedVariablesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder clearTightenedVariables() {
if (tightenedVariablesBuilder_ == null) {
tightenedVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
tightenedVariablesBuilder_.clear();
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public Builder removeTightenedVariables(int index) {
if (tightenedVariablesBuilder_ == null) {
ensureTightenedVariablesIsMutable();
tightenedVariables_.remove(index);
onChanged();
} else {
tightenedVariablesBuilder_.remove(index);
}
return this;
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public com.google.ortools.sat.IntegerVariableProto.Builder getTightenedVariablesBuilder(
int index) {
return getTightenedVariablesFieldBuilder().getBuilder(index);
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public com.google.ortools.sat.IntegerVariableProtoOrBuilder getTightenedVariablesOrBuilder(
int index) {
if (tightenedVariablesBuilder_ == null) {
return tightenedVariables_.get(index); } else {
return tightenedVariablesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public java.util.List extends com.google.ortools.sat.IntegerVariableProtoOrBuilder>
getTightenedVariablesOrBuilderList() {
if (tightenedVariablesBuilder_ != null) {
return tightenedVariablesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(tightenedVariables_);
}
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public com.google.ortools.sat.IntegerVariableProto.Builder addTightenedVariablesBuilder() {
return getTightenedVariablesFieldBuilder().addBuilder(
com.google.ortools.sat.IntegerVariableProto.getDefaultInstance());
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public com.google.ortools.sat.IntegerVariableProto.Builder addTightenedVariablesBuilder(
int index) {
return getTightenedVariablesFieldBuilder().addBuilder(
index, com.google.ortools.sat.IntegerVariableProto.getDefaultInstance());
}
/**
*
* Advanced usage.
* If the option fill_tightened_domains_in_response is set, then this field
* will be a copy of the CpModelProto.variables where each domain has been
* reduced using the information the solver was able to derive. Note that this
* is only filled with the info derived during a normal search and we do not
* have any dedicated algorithm to improve it.
* If the problem is a feasibility problem, then these bounds will be valid
* for any feasible solution. If the problem is an optimization problem, then
* these bounds will only be valid for any OPTIMAL solutions, it can exclude
* sub-optimal feasible ones.
*
*
* repeated .operations_research.sat.IntegerVariableProto tightened_variables = 21;
*/
public java.util.List
getTightenedVariablesBuilderList() {
return getTightenedVariablesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.sat.IntegerVariableProto, com.google.ortools.sat.IntegerVariableProto.Builder, com.google.ortools.sat.IntegerVariableProtoOrBuilder>
getTightenedVariablesFieldBuilder() {
if (tightenedVariablesBuilder_ == null) {
tightenedVariablesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
com.google.ortools.sat.IntegerVariableProto, com.google.ortools.sat.IntegerVariableProto.Builder, com.google.ortools.sat.IntegerVariableProtoOrBuilder>(
tightenedVariables_,
((bitField0_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
tightenedVariables_ = null;
}
return tightenedVariablesBuilder_;
}
private com.google.protobuf.Internal.IntList sufficientAssumptionsForInfeasibility_ = emptyIntList();
private void ensureSufficientAssumptionsForInfeasibilityIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
sufficientAssumptionsForInfeasibility_ = mutableCopy(sufficientAssumptionsForInfeasibility_);
bitField0_ |= 0x00000010;
}
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @return A list containing the sufficientAssumptionsForInfeasibility.
*/
public java.util.List
getSufficientAssumptionsForInfeasibilityList() {
return ((bitField0_ & 0x00000010) != 0) ?
java.util.Collections.unmodifiableList(sufficientAssumptionsForInfeasibility_) : sufficientAssumptionsForInfeasibility_;
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @return The count of sufficientAssumptionsForInfeasibility.
*/
public int getSufficientAssumptionsForInfeasibilityCount() {
return sufficientAssumptionsForInfeasibility_.size();
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @param index The index of the element to return.
* @return The sufficientAssumptionsForInfeasibility at the given index.
*/
public int getSufficientAssumptionsForInfeasibility(int index) {
return sufficientAssumptionsForInfeasibility_.getInt(index);
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @param index The index to set the value at.
* @param value The sufficientAssumptionsForInfeasibility to set.
* @return This builder for chaining.
*/
public Builder setSufficientAssumptionsForInfeasibility(
int index, int value) {
ensureSufficientAssumptionsForInfeasibilityIsMutable();
sufficientAssumptionsForInfeasibility_.setInt(index, value);
onChanged();
return this;
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @param value The sufficientAssumptionsForInfeasibility to add.
* @return This builder for chaining.
*/
public Builder addSufficientAssumptionsForInfeasibility(int value) {
ensureSufficientAssumptionsForInfeasibilityIsMutable();
sufficientAssumptionsForInfeasibility_.addInt(value);
onChanged();
return this;
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @param values The sufficientAssumptionsForInfeasibility to add.
* @return This builder for chaining.
*/
public Builder addAllSufficientAssumptionsForInfeasibility(
java.lang.Iterable extends java.lang.Integer> values) {
ensureSufficientAssumptionsForInfeasibilityIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sufficientAssumptionsForInfeasibility_);
onChanged();
return this;
}
/**
*
* A subset of the model "assumptions" field. This will only be filled if the
* status is INFEASIBLE. This subset of assumption will be enough to still get
* an infeasible problem.
* This is related to what is called the irreducible inconsistent subsystem or
* IIS. Except one is only concerned by the provided assumptions. There is
* also no guarantee that we return an irreducible (aka minimal subset).
* However, this is based on SAT explanation and there is a good chance it is
* not too large.
* If you really want a minimal subset, a possible way to get one is by
* changing your model to minimize the number of assumptions at false, but
* this is likely an harder problem to solve.
* TODO(user): Allows for returning multiple core at once.
*
*
* repeated int32 sufficient_assumptions_for_infeasibility = 23;
* @return This builder for chaining.
*/
public Builder clearSufficientAssumptionsForInfeasibility() {
sufficientAssumptionsForInfeasibility_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
private boolean allSolutionsWereFound_ ;
/**
*
* This will be true iff the solver was asked to find all solutions to a
* satisfiability problem (or all optimal solutions to an optimization
* problem), and it was successful in doing so.
* TODO(user): Remove as we also use the OPTIMAL vs FEASIBLE status for that.
*
*
* bool all_solutions_were_found = 5;
* @return The allSolutionsWereFound.
*/
@java.lang.Override
public boolean getAllSolutionsWereFound() {
return allSolutionsWereFound_;
}
/**
*
* This will be true iff the solver was asked to find all solutions to a
* satisfiability problem (or all optimal solutions to an optimization
* problem), and it was successful in doing so.
* TODO(user): Remove as we also use the OPTIMAL vs FEASIBLE status for that.
*
*
* bool all_solutions_were_found = 5;
* @param value The allSolutionsWereFound to set.
* @return This builder for chaining.
*/
public Builder setAllSolutionsWereFound(boolean value) {
allSolutionsWereFound_ = value;
onChanged();
return this;
}
/**
*
* This will be true iff the solver was asked to find all solutions to a
* satisfiability problem (or all optimal solutions to an optimization
* problem), and it was successful in doing so.
* TODO(user): Remove as we also use the OPTIMAL vs FEASIBLE status for that.
*
*
* bool all_solutions_were_found = 5;
* @return This builder for chaining.
*/
public Builder clearAllSolutionsWereFound() {
allSolutionsWereFound_ = false;
onChanged();
return this;
}
private long numBooleans_ ;
/**
*
* Some statistics about the solve.
*
*
* int64 num_booleans = 10;
* @return The numBooleans.
*/
@java.lang.Override
public long getNumBooleans() {
return numBooleans_;
}
/**
*
* Some statistics about the solve.
*
*
* int64 num_booleans = 10;
* @param value The numBooleans to set.
* @return This builder for chaining.
*/
public Builder setNumBooleans(long value) {
numBooleans_ = value;
onChanged();
return this;
}
/**
*
* Some statistics about the solve.
*
*
* int64 num_booleans = 10;
* @return This builder for chaining.
*/
public Builder clearNumBooleans() {
numBooleans_ = 0L;
onChanged();
return this;
}
private long numConflicts_ ;
/**
* int64 num_conflicts = 11;
* @return The numConflicts.
*/
@java.lang.Override
public long getNumConflicts() {
return numConflicts_;
}
/**
* int64 num_conflicts = 11;
* @param value The numConflicts to set.
* @return This builder for chaining.
*/
public Builder setNumConflicts(long value) {
numConflicts_ = value;
onChanged();
return this;
}
/**
* int64 num_conflicts = 11;
* @return This builder for chaining.
*/
public Builder clearNumConflicts() {
numConflicts_ = 0L;
onChanged();
return this;
}
private long numBranches_ ;
/**
* int64 num_branches = 12;
* @return The numBranches.
*/
@java.lang.Override
public long getNumBranches() {
return numBranches_;
}
/**
* int64 num_branches = 12;
* @param value The numBranches to set.
* @return This builder for chaining.
*/
public Builder setNumBranches(long value) {
numBranches_ = value;
onChanged();
return this;
}
/**
* int64 num_branches = 12;
* @return This builder for chaining.
*/
public Builder clearNumBranches() {
numBranches_ = 0L;
onChanged();
return this;
}
private long numBinaryPropagations_ ;
/**
* int64 num_binary_propagations = 13;
* @return The numBinaryPropagations.
*/
@java.lang.Override
public long getNumBinaryPropagations() {
return numBinaryPropagations_;
}
/**
* int64 num_binary_propagations = 13;
* @param value The numBinaryPropagations to set.
* @return This builder for chaining.
*/
public Builder setNumBinaryPropagations(long value) {
numBinaryPropagations_ = value;
onChanged();
return this;
}
/**
* int64 num_binary_propagations = 13;
* @return This builder for chaining.
*/
public Builder clearNumBinaryPropagations() {
numBinaryPropagations_ = 0L;
onChanged();
return this;
}
private long numIntegerPropagations_ ;
/**
* int64 num_integer_propagations = 14;
* @return The numIntegerPropagations.
*/
@java.lang.Override
public long getNumIntegerPropagations() {
return numIntegerPropagations_;
}
/**
* int64 num_integer_propagations = 14;
* @param value The numIntegerPropagations to set.
* @return This builder for chaining.
*/
public Builder setNumIntegerPropagations(long value) {
numIntegerPropagations_ = value;
onChanged();
return this;
}
/**
* int64 num_integer_propagations = 14;
* @return This builder for chaining.
*/
public Builder clearNumIntegerPropagations() {
numIntegerPropagations_ = 0L;
onChanged();
return this;
}
private double wallTime_ ;
/**
* double wall_time = 15;
* @return The wallTime.
*/
@java.lang.Override
public double getWallTime() {
return wallTime_;
}
/**
* double wall_time = 15;
* @param value The wallTime to set.
* @return This builder for chaining.
*/
public Builder setWallTime(double value) {
wallTime_ = value;
onChanged();
return this;
}
/**
* double wall_time = 15;
* @return This builder for chaining.
*/
public Builder clearWallTime() {
wallTime_ = 0D;
onChanged();
return this;
}
private double userTime_ ;
/**
* double user_time = 16;
* @return The userTime.
*/
@java.lang.Override
public double getUserTime() {
return userTime_;
}
/**
* double user_time = 16;
* @param value The userTime to set.
* @return This builder for chaining.
*/
public Builder setUserTime(double value) {
userTime_ = value;
onChanged();
return this;
}
/**
* double user_time = 16;
* @return This builder for chaining.
*/
public Builder clearUserTime() {
userTime_ = 0D;
onChanged();
return this;
}
private double deterministicTime_ ;
/**
* double deterministic_time = 17;
* @return The deterministicTime.
*/
@java.lang.Override
public double getDeterministicTime() {
return deterministicTime_;
}
/**
* double deterministic_time = 17;
* @param value The deterministicTime to set.
* @return This builder for chaining.
*/
public Builder setDeterministicTime(double value) {
deterministicTime_ = value;
onChanged();
return this;
}
/**
* double deterministic_time = 17;
* @return This builder for chaining.
*/
public Builder clearDeterministicTime() {
deterministicTime_ = 0D;
onChanged();
return this;
}
private double primalIntegral_ ;
/**
* double primal_integral = 22;
* @return The primalIntegral.
*/
@java.lang.Override
public double getPrimalIntegral() {
return primalIntegral_;
}
/**
* double primal_integral = 22;
* @param value The primalIntegral to set.
* @return This builder for chaining.
*/
public Builder setPrimalIntegral(double value) {
primalIntegral_ = value;
onChanged();
return this;
}
/**
* double primal_integral = 22;
* @return This builder for chaining.
*/
public Builder clearPrimalIntegral() {
primalIntegral_ = 0D;
onChanged();
return this;
}
private java.lang.Object solutionInfo_ = "";
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @return The solutionInfo.
*/
public java.lang.String getSolutionInfo() {
java.lang.Object ref = solutionInfo_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
solutionInfo_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @return The bytes for solutionInfo.
*/
public com.google.protobuf.ByteString
getSolutionInfoBytes() {
java.lang.Object ref = solutionInfo_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
solutionInfo_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @param value The solutionInfo to set.
* @return This builder for chaining.
*/
public Builder setSolutionInfo(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
solutionInfo_ = value;
onChanged();
return this;
}
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @return This builder for chaining.
*/
public Builder clearSolutionInfo() {
solutionInfo_ = getDefaultInstance().getSolutionInfo();
onChanged();
return this;
}
/**
*
* Additional information about how the solution was found.
*
*
* string solution_info = 20;
* @param value The bytes for solutionInfo to set.
* @return This builder for chaining.
*/
public Builder setSolutionInfoBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
solutionInfo_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:operations_research.sat.CpSolverResponse)
}
// @@protoc_insertion_point(class_scope:operations_research.sat.CpSolverResponse)
private static final com.google.ortools.sat.CpSolverResponse DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.ortools.sat.CpSolverResponse();
}
public static com.google.ortools.sat.CpSolverResponse getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CpSolverResponse parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CpSolverResponse(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public com.google.ortools.sat.CpSolverResponse getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy