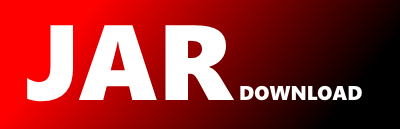
commonMain.com.paoapps.blockedcache.BlockedCacheStreamBuilder.kt Maven / Gradle / Ivy
package com.paoapps.blockedcache
import kotlinx.coroutines.flow.Flow
import kotlinx.coroutines.flow.flowOf
/**
* A builder class for creating instances of [BlockedCacheStream]. This class provides a fluent interface
* to configure and build a stream around a [BlockedCache] with specified fetcher, update, and condition logic.
*
* @param T The type of data managed by the cache.
* @property cache The [BlockedCache] instance to be used for data storage and retrieval.
* @property fetcher A [Fetcher] instance, defining how new data is fetched.
*/
class BlockedCacheStreamBuilder(
private var cache: BlockedCache,
private var fetcher: Fetcher,
) {
private var updateData: ((BlockedCacheData) -> Unit) = { }
private var condition: Flow = flowOf(true)
/**
* Represents a fetching logic encapsulating a suspend function to fetch data.
*
* @param T The type of data to be fetched.
* @property fetcher A suspend function that returns a [FetcherResult] representing the fetched data.
*/
data class Fetcher(
val fetcher: suspend () -> FetcherResult,
) {
companion object {
fun ofResult(fetcher: suspend () -> FetcherResult) = Fetcher(fetcher)
fun of(fetcher: suspend () -> T) = Fetcher {
try {
FetcherResult.Data(fetcher())
} catch (e: Exception) {
FetcherResult.Error.Exception(e)
}
}
}
}
/**
* Sets the function to update the cache data.
*
* @param updateData A function to update the cache with new data.
* @return The current instance of [BlockedCacheStreamBuilder] for fluent configuration.
*/
fun updateData(updateData: (BlockedCacheData) -> Unit) = apply {
this.updateData = updateData
}
companion object {
fun from(
cache: BlockedCache,
fetcher: Fetcher
) = BlockedCacheStreamBuilder(cache, fetcher)
}
/**
* Builds and returns a [BlockedCacheStream] with the configured settings.
*
* @return An instance of [BlockedCacheStream] configured with the provided cache, fetcher, and update logic.
*/
fun build(): BlockedCacheStream {
return BlockedCacheStream(
cache = cache,
fetcher = fetcher,
updateData = { updateData(it) },
condition = condition
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy